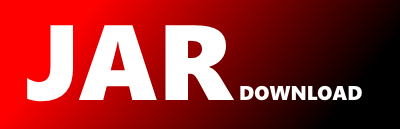
org.lwjgl.openxr.METASpatialEntityMesh Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
/**
* The XR_META_spatial_entity_mesh extension.
*
* This extension expands on the concept of spatial entities to include a way for a spatial entity to represent a triangle mesh that describes 3D geometry of the spatial entity in a scene. Spatial entities are defined in {@link FBSpatialEntity XR_FB_spatial_entity} extension using the Entity-Component System. The triangle mesh is a component type that may be associated to a spatial entity.
*
* In order to enable the functionality of this extension, you must pass the name of the extension into {@link XR10#xrCreateInstance CreateInstance} via the {@link XrInstanceCreateInfo}{@code ::enabledExtensionNames} parameter as indicated in the extension section.
*/
public class METASpatialEntityMesh {
/** The extension specification version. */
public static final int XR_META_spatial_entity_mesh_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_META_SPATIAL_ENTITY_MESH_EXTENSION_NAME = "XR_META_spatial_entity_mesh";
/** Extends {@code XrSpaceComponentTypeFB}. */
public static final int XR_SPACE_COMPONENT_TYPE_TRIANGLE_MESH_META = 1000269000;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SPACE_TRIANGLE_MESH_GET_INFO_META TYPE_SPACE_TRIANGLE_MESH_GET_INFO_META}
* - {@link #XR_TYPE_SPACE_TRIANGLE_MESH_META TYPE_SPACE_TRIANGLE_MESH_META}
*
*/
public static final int
XR_TYPE_SPACE_TRIANGLE_MESH_GET_INFO_META = 1000269001,
XR_TYPE_SPACE_TRIANGLE_MESH_META = 1000269002;
protected METASpatialEntityMesh() {
throw new UnsupportedOperationException();
}
// --- [ xrGetSpaceTriangleMeshMETA ] ---
/** Unsafe version of: {@link #xrGetSpaceTriangleMeshMETA GetSpaceTriangleMeshMETA} */
public static int nxrGetSpaceTriangleMeshMETA(XrSpace space, long getInfo, long triangleMeshOutput) {
long __functionAddress = space.getCapabilities().xrGetSpaceTriangleMeshMETA;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(space.address(), getInfo, triangleMeshOutput, __functionAddress);
}
/**
* Gets an XrSpaceTriangleMeshMETA that represents a triangle mesh.
*
* C Specification
*
* The {@link #xrGetSpaceTriangleMeshMETA GetSpaceTriangleMeshMETA} function is defined as:
*
*
* XrResult xrGetSpaceTriangleMeshMETA(
* XrSpace space,
* const XrSpaceTriangleMeshGetInfoMETA* getInfo,
* XrSpaceTriangleMeshMETA* triangleMeshOutput);
*
* Description
*
* The {@link #xrGetSpaceTriangleMeshMETA GetSpaceTriangleMeshMETA} function is used by the application to perform the two calls required to obtain a triangle mesh associated to a spatial entity specified by {@code space}.
*
* The spatial entity {@code space} must have the {@link #XR_SPACE_COMPONENT_TYPE_TRIANGLE_MESH_META SPACE_COMPONENT_TYPE_TRIANGLE_MESH_META} component type enabled, otherwise this function will return {@link FBSpatialEntity#XR_ERROR_SPACE_COMPONENT_NOT_ENABLED_FB ERROR_SPACE_COMPONENT_NOT_ENABLED_FB}.
*
* Valid Usage (Implicit)
*
*
* - The {@link METASpatialEntityMesh XR_META_spatial_entity_mesh} extension must be enabled prior to calling {@link #xrGetSpaceTriangleMeshMETA GetSpaceTriangleMeshMETA}
* - {@code space} must be a valid {@code XrSpace} handle
* - {@code getInfo} must be a pointer to a valid {@link XrSpaceTriangleMeshGetInfoMETA} structure
* - {@code triangleMeshOutput} must be a pointer to an {@link XrSpaceTriangleMeshMETA} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link FBSpatialEntity#XR_ERROR_SPACE_COMPONENT_NOT_ENABLED_FB ERROR_SPACE_COMPONENT_NOT_ENABLED_FB}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrSpaceTriangleMeshGetInfoMETA}, {@link XrSpaceTriangleMeshMETA}
*
* @param space a handle to an {@code XrSpace}.
* @param getInfo exists for extensibility purposes. It is {@code NULL} or a pointer to a valid {@link XrSpaceTriangleMeshGetInfoMETA}.
* @param triangleMeshOutput the output parameter that points to an {@link XrSpaceTriangleMeshMETA}.
*/
@NativeType("XrResult")
public static int xrGetSpaceTriangleMeshMETA(XrSpace space, @NativeType("XrSpaceTriangleMeshGetInfoMETA const *") XrSpaceTriangleMeshGetInfoMETA getInfo, @NativeType("XrSpaceTriangleMeshMETA *") XrSpaceTriangleMeshMETA triangleMeshOutput) {
return nxrGetSpaceTriangleMeshMETA(space, getInfo.address(), triangleMeshOutput.address());
}
}