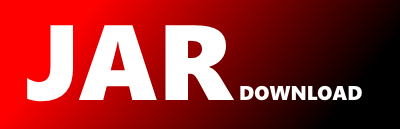
org.lwjgl.openxr.MLCompat Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_ML_compat extension.
*
* This extension provides functionality to facilitate transitioning from Magic Leap SDK to OpenXR SDK, most notably interoperability between Coordinate Frame UUIDs and {@code XrSpace}.
*/
public class MLCompat {
/** The extension specification version. */
public static final int XR_ML_compat_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_ML_COMPAT_EXTENSION_NAME = "XR_ML_compat";
/** Extends {@code XrStructureType}. */
public static final int XR_TYPE_COORDINATE_SPACE_CREATE_INFO_ML = 1000137000;
protected MLCompat() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateSpaceFromCoordinateFrameUIDML ] ---
/** Unsafe version of: {@link #xrCreateSpaceFromCoordinateFrameUIDML CreateSpaceFromCoordinateFrameUIDML} */
public static int nxrCreateSpaceFromCoordinateFrameUIDML(XrSession session, long createInfo, long space) {
long __functionAddress = session.getCapabilities().xrCreateSpaceFromCoordinateFrameUIDML;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), createInfo, space, __functionAddress);
}
/**
* Creates a space based on a cfuid.
*
* C Specification
*
* The {@link #xrCreateSpaceFromCoordinateFrameUIDML CreateSpaceFromCoordinateFrameUIDML} function is defined as:
*
*
* XrResult xrCreateSpaceFromCoordinateFrameUIDML(
* XrSession session,
* const XrCoordinateSpaceCreateInfoML * createInfo,
* XrSpace* space);
*
* Description
*
* The service that created the underlying {@link XrCoordinateSpaceCreateInfoML}{@code ::cfuid} must remain active for the lifetime of the {@code XrSpace}. If {@link XR10#xrLocateSpace LocateSpace} is called on a space created from an {@link XrCoordinateSpaceCreateInfoML}{@code ::cfuid} from a no-longer-active service, the runtime may set {@link XrSpaceLocation}{@code ::locationFlags} to 0.
*
* {@code XrSpace} handles are destroyed using {@link XR10#xrDestroySpace DestroySpace}.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLCompat XR_ML_compat} extension must be enabled prior to calling {@link #xrCreateSpaceFromCoordinateFrameUIDML CreateSpaceFromCoordinateFrameUIDML}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrCoordinateSpaceCreateInfoML} structure
* - {@code space} must be a pointer to an {@code XrSpace} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_POSE_INVALID ERROR_POSE_INVALID}
*
*
*
* See Also
*
* {@link XrCoordinateSpaceCreateInfoML}
*
* @param session a handle to an {@code XrSession} previously created with {@link XR10#xrCreateSession CreateSession}.
* @param createInfo the {@link XrCoordinateSpaceCreateInfoML} used to specify the space.
* @param space the returned space handle.
*/
@NativeType("XrResult")
public static int xrCreateSpaceFromCoordinateFrameUIDML(XrSession session, @NativeType("XrCoordinateSpaceCreateInfoML const *") XrCoordinateSpaceCreateInfoML createInfo, @NativeType("XrSpace *") PointerBuffer space) {
if (CHECKS) {
check(space, 1);
}
return nxrCreateSpaceFromCoordinateFrameUIDML(session, createInfo.address(), memAddress(space));
}
}