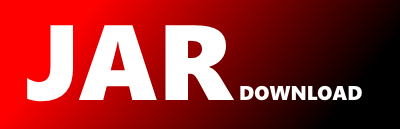
org.lwjgl.openxr.MLLocalizationMap Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_ML_localization_map extension.
*
* A Magic Leap localization map is a container that holds metadata about the scanned environment. It is a digital copy of a physical place. A localization map holds spatial anchors, dense mesh, planes, feature points, and positional data.
*
*
* - Spatial anchors - Used for persistent placement of content.
* - Dense mesh - 3D triangulated geometry representing Magic Leap device understanding of the real-world geometry of an area.
* - Planes - Large, flat surfaces derived from dense mesh data.
*
*
* Localization maps can be created on device or in the Magic Leap AR Cloud. There are two types - "On Device" and "Cloud".
*
*
* - "On Device" for OpenXR (local space for MagicLeap) - are for a single device and can be shared via the export/import mechanism.
* - "Cloud" for OpenXR (shared space for MagicLeap) - can be shared across multiple MagicLeap devices in the AR Cloud.
*
*
* Note
*
* Localization Maps are called Spaces in the Magic Leap C-API.
*
*
* Permissions
*
* Android applications must have the com.magicleap.permission.SPACE_MANAGER permission listed in their manifest to use these functions:
*
*
* - {@link #xrQueryLocalizationMapsML QueryLocalizationMapsML}
* - {@link #xrRequestMapLocalizationML RequestMapLocalizationML}
*
*
* (protection level: normal)
*
* Android applications must have the com.magicleap.permission.SPACE_IMPORT_EXPORT permission listed in their manifest and granted to use these functions:
*
*
* - {@link #xrImportLocalizationMapML ImportLocalizationMapML}
* - {@link #xrCreateExportedLocalizationMapML CreateExportedLocalizationMapML}
*
*
* (protection level: dangerous)
*/
public class MLLocalizationMap {
/** The extension specification version. */
public static final int XR_ML_localization_map_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_ML_LOCALIZATION_MAP_EXTENSION_NAME = "XR_ML_localization_map";
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_EXPORTED_LOCALIZATION_MAP_ML = 1000139000;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_LOCALIZATION_MAP_ML TYPE_LOCALIZATION_MAP_ML}
* - {@link #XR_TYPE_EVENT_DATA_LOCALIZATION_CHANGED_ML TYPE_EVENT_DATA_LOCALIZATION_CHANGED_ML}
* - {@link #XR_TYPE_MAP_LOCALIZATION_REQUEST_INFO_ML TYPE_MAP_LOCALIZATION_REQUEST_INFO_ML}
* - {@link #XR_TYPE_LOCALIZATION_MAP_IMPORT_INFO_ML TYPE_LOCALIZATION_MAP_IMPORT_INFO_ML}
* - {@link #XR_TYPE_LOCALIZATION_ENABLE_EVENTS_INFO_ML TYPE_LOCALIZATION_ENABLE_EVENTS_INFO_ML}
*
*/
public static final int
XR_TYPE_LOCALIZATION_MAP_ML = 1000139000,
XR_TYPE_EVENT_DATA_LOCALIZATION_CHANGED_ML = 1000139001,
XR_TYPE_MAP_LOCALIZATION_REQUEST_INFO_ML = 1000139002,
XR_TYPE_LOCALIZATION_MAP_IMPORT_INFO_ML = 1000139003,
XR_TYPE_LOCALIZATION_ENABLE_EVENTS_INFO_ML = 1000139004;
/**
* Extends {@code XrResult}.
*
* Enum values:
*
*
* - {@link #XR_ERROR_LOCALIZATION_MAP_INCOMPATIBLE_ML ERROR_LOCALIZATION_MAP_INCOMPATIBLE_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_UNAVAILABLE_ML ERROR_LOCALIZATION_MAP_UNAVAILABLE_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_FAIL_ML ERROR_LOCALIZATION_MAP_FAIL_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_IMPORT_EXPORT_PERMISSION_DENIED_ML ERROR_LOCALIZATION_MAP_IMPORT_EXPORT_PERMISSION_DENIED_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_PERMISSION_DENIED_ML ERROR_LOCALIZATION_MAP_PERMISSION_DENIED_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_ALREADY_EXISTS_ML ERROR_LOCALIZATION_MAP_ALREADY_EXISTS_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_CANNOT_EXPORT_CLOUD_MAP_ML ERROR_LOCALIZATION_MAP_CANNOT_EXPORT_CLOUD_MAP_ML}
*
*/
public static final int
XR_ERROR_LOCALIZATION_MAP_INCOMPATIBLE_ML = -1000139000,
XR_ERROR_LOCALIZATION_MAP_UNAVAILABLE_ML = -1000139001,
XR_ERROR_LOCALIZATION_MAP_FAIL_ML = -1000139002,
XR_ERROR_LOCALIZATION_MAP_IMPORT_EXPORT_PERMISSION_DENIED_ML = -1000139003,
XR_ERROR_LOCALIZATION_MAP_PERMISSION_DENIED_ML = -1000139004,
XR_ERROR_LOCALIZATION_MAP_ALREADY_EXISTS_ML = -1000139005,
XR_ERROR_LOCALIZATION_MAP_CANNOT_EXPORT_CLOUD_MAP_ML = -1000139006;
/** Extends {@code XrReferenceSpaceType}. */
public static final int XR_REFERENCE_SPACE_TYPE_LOCALIZATION_MAP_ML = 1000139000;
/** XR_MAX_LOCALIZATION_MAP_NAME_LENGTH_ML */
public static final int XR_MAX_LOCALIZATION_MAP_NAME_LENGTH_ML = 64;
/**
* XrLocalizationMapStateML - Localization Map State
*
* Description
*
*
* Enum Description
*
* {@link #XR_LOCALIZATION_MAP_STATE_NOT_LOCALIZED_ML LOCALIZATION_MAP_STATE_NOT_LOCALIZED_ML} The system is not localized into a map. Features like Spatial Anchors relying on localization will not work.
* {@link #XR_LOCALIZATION_MAP_STATE_LOCALIZED_ML LOCALIZATION_MAP_STATE_LOCALIZED_ML} The system is localized into a map.
* {@link #XR_LOCALIZATION_MAP_STATE_LOCALIZATION_PENDING_ML LOCALIZATION_MAP_STATE_LOCALIZATION_PENDING_ML} The system is localizing into a map.
* {@link #XR_LOCALIZATION_MAP_STATE_LOCALIZATION_SLEEPING_BEFORE_RETRY_ML LOCALIZATION_MAP_STATE_LOCALIZATION_SLEEPING_BEFORE_RETRY_ML} Initial localization failed, the system will retry localization.
*
*
*
* See Also
*
* {@link XrEventDataLocalizationChangedML}
*/
public static final int
XR_LOCALIZATION_MAP_STATE_NOT_LOCALIZED_ML = 0,
XR_LOCALIZATION_MAP_STATE_LOCALIZED_ML = 1,
XR_LOCALIZATION_MAP_STATE_LOCALIZATION_PENDING_ML = 2,
XR_LOCALIZATION_MAP_STATE_LOCALIZATION_SLEEPING_BEFORE_RETRY_ML = 3;
/**
* XrLocalizationMapTypeML - Localization Map Type
*
* Description
*
*
* Enum Description
*
* {@link #XR_LOCALIZATION_MAP_TYPE_ON_DEVICE_ML LOCALIZATION_MAP_TYPE_ON_DEVICE_ML} The system is localized into an On-Device map, published anchors are not shared between different devices.
* {@link #XR_LOCALIZATION_MAP_TYPE_CLOUD_ML LOCALIZATION_MAP_TYPE_CLOUD_ML} The system is localized into a Cloud Map, anchors are shared per cloud account settings.
*
*
*
* See Also
*
* {@link XrLocalizationMapML}
*/
public static final int
XR_LOCALIZATION_MAP_TYPE_ON_DEVICE_ML = 0,
XR_LOCALIZATION_MAP_TYPE_CLOUD_ML = 1;
/**
* XrLocalizationMapConfidenceML - Localization Confidence
*
* Description
*
*
* Enum Description
*
* {@link #XR_LOCALIZATION_MAP_CONFIDENCE_POOR_ML LOCALIZATION_MAP_CONFIDENCE_POOR_ML} The localization map has poor confidence, systems relying on the localization map are likely to have poor performance.
* {@link #XR_LOCALIZATION_MAP_CONFIDENCE_FAIR_ML LOCALIZATION_MAP_CONFIDENCE_FAIR_ML} The confidence is fair, current environmental conditions may adversely affect localization.
* {@link #XR_LOCALIZATION_MAP_CONFIDENCE_GOOD_ML LOCALIZATION_MAP_CONFIDENCE_GOOD_ML} The confidence is high, persistent content should be stable.
* {@link #XR_LOCALIZATION_MAP_CONFIDENCE_EXCELLENT_ML LOCALIZATION_MAP_CONFIDENCE_EXCELLENT_ML} This is a very high-confidence localization, persistent content will be very stable.
*
*
*
* See Also
*
* {@link XrEventDataLocalizationChangedML}
*/
public static final int
XR_LOCALIZATION_MAP_CONFIDENCE_POOR_ML = 0,
XR_LOCALIZATION_MAP_CONFIDENCE_FAIR_ML = 1,
XR_LOCALIZATION_MAP_CONFIDENCE_GOOD_ML = 2,
XR_LOCALIZATION_MAP_CONFIDENCE_EXCELLENT_ML = 3;
/**
* XrLocalizationMapErrorFlagBitsML - The world meshing detector flags.
*
* Description
*
* The flag bits have the following meanings:
*
* Flag Descriptions
*
*
* - {@link #XR_LOCALIZATION_MAP_ERROR_UNKNOWN_BIT_ML LOCALIZATION_MAP_ERROR_UNKNOWN_BIT_ML} — Localization failed for an unknown reason.
* - {@link #XR_LOCALIZATION_MAP_ERROR_OUT_OF_MAPPED_AREA_BIT_ML LOCALIZATION_MAP_ERROR_OUT_OF_MAPPED_AREA_BIT_ML} — Localization failed because the user is outside of the mapped area.
* - {@link #XR_LOCALIZATION_MAP_ERROR_LOW_FEATURE_COUNT_BIT_ML LOCALIZATION_MAP_ERROR_LOW_FEATURE_COUNT_BIT_ML} — There are not enough features in the environment to successfully localize.
* - {@link #XR_LOCALIZATION_MAP_ERROR_EXCESSIVE_MOTION_BIT_ML LOCALIZATION_MAP_ERROR_EXCESSIVE_MOTION_BIT_ML} — Localization failed due to excessive motion.
* - {@link #XR_LOCALIZATION_MAP_ERROR_LOW_LIGHT_BIT_ML LOCALIZATION_MAP_ERROR_LOW_LIGHT_BIT_ML} — Localization failed because the lighting levels are too low in the environment.
* - {@link #XR_LOCALIZATION_MAP_ERROR_HEADPOSE_BIT_ML LOCALIZATION_MAP_ERROR_HEADPOSE_BIT_ML} — A headpose failure caused localization to be unsuccessful.
*
*/
public static final int
XR_LOCALIZATION_MAP_ERROR_UNKNOWN_BIT_ML = 0x1,
XR_LOCALIZATION_MAP_ERROR_OUT_OF_MAPPED_AREA_BIT_ML = 0x2,
XR_LOCALIZATION_MAP_ERROR_LOW_FEATURE_COUNT_BIT_ML = 0x4,
XR_LOCALIZATION_MAP_ERROR_EXCESSIVE_MOTION_BIT_ML = 0x8,
XR_LOCALIZATION_MAP_ERROR_LOW_LIGHT_BIT_ML = 0x10,
XR_LOCALIZATION_MAP_ERROR_HEADPOSE_BIT_ML = 0x20;
protected MLLocalizationMap() {
throw new UnsupportedOperationException();
}
// --- [ xrEnableLocalizationEventsML ] ---
/** Unsafe version of: {@link #xrEnableLocalizationEventsML EnableLocalizationEventsML} */
public static int nxrEnableLocalizationEventsML(XrSession session, long info) {
long __functionAddress = session.getCapabilities().xrEnableLocalizationEventsML;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(session.address(), info, __functionAddress);
}
/**
* Retrieve the current localization status.
*
* C Specification
*
* The {@link #xrEnableLocalizationEventsML EnableLocalizationEventsML} function is defined as:
*
*
* XrResult xrEnableLocalizationEventsML(
* XrSession session,
* const XrLocalizationEnableEventsInfoML * info);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLLocalizationMap XR_ML_localization_map} extension must be enabled prior to calling {@link #xrEnableLocalizationEventsML EnableLocalizationEventsML}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code info} must be a pointer to a valid {@link XrLocalizationEnableEventsInfoML} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link #XR_ERROR_LOCALIZATION_MAP_PERMISSION_DENIED_ML ERROR_LOCALIZATION_MAP_PERMISSION_DENIED_ML}
*
*
*
* See Also
*
* {@link XrEventDataLocalizationChangedML}, {@link XrLocalizationEnableEventsInfoML}
*
* @param session a handle to an {@code XrSession} previously created with {@link XR10#xrCreateSession CreateSession}.
* @param info a pointer to an {@link XrLocalizationEnableEventsInfoML} structure.
*/
@NativeType("XrResult")
public static int xrEnableLocalizationEventsML(XrSession session, @NativeType("XrLocalizationEnableEventsInfoML const *") XrLocalizationEnableEventsInfoML info) {
return nxrEnableLocalizationEventsML(session, info.address());
}
// --- [ xrQueryLocalizationMapsML ] ---
/**
* Unsafe version of: {@link #xrQueryLocalizationMapsML QueryLocalizationMapsML}
*
* @param mapCapacityInput the capacity of the maps array, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrQueryLocalizationMapsML(XrSession session, long queryInfo, int mapCapacityInput, long mapCountOutput, long maps) {
long __functionAddress = session.getCapabilities().xrQueryLocalizationMapsML;
if (CHECKS) {
check(__functionAddress);
}
return callPPPPI(session.address(), queryInfo, mapCapacityInput, mapCountOutput, maps, __functionAddress);
}
/**
* Query the available localization maps.
*
* C Specification
*
* The {@link #xrQueryLocalizationMapsML QueryLocalizationMapsML} function is defined as:
*
*
* XrResult xrQueryLocalizationMapsML(
* XrSession session,
* const XrLocalizationMapQueryInfoBaseHeaderML* queryInfo,
* uint32_t mapCapacityInput,
* uint32_t * mapCountOutput,
* XrLocalizationMapML* maps);
*
* Description
*
* The list of localization maps returned will depend on the current device mapping mode. Only the localization maps associated with the current mapping mode will be returned by this call. Device mapping mode (e.g. {@link #XR_LOCALIZATION_MAP_TYPE_ON_DEVICE_ML LOCALIZATION_MAP_TYPE_ON_DEVICE_ML} or {@link #XR_LOCALIZATION_MAP_TYPE_CLOUD_ML LOCALIZATION_MAP_TYPE_CLOUD_ML}) can only be changed via the system application(s).
*
* The list of maps known to the runtime may change between the two calls to {@link #xrQueryLocalizationMapsML QueryLocalizationMapsML}. This is however a rare occurrence and the application may retry the call again if it receives {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLLocalizationMap XR_ML_localization_map} extension must be enabled prior to calling {@link #xrQueryLocalizationMapsML QueryLocalizationMapsML}
* - {@code session} must be a valid {@code XrSession} handle
* - If {@code queryInfo} is not {@code NULL}, {@code queryInfo} must be a pointer to a valid {@link XrLocalizationMapQueryInfoBaseHeaderML}-based structure
* - {@code mapCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code mapCapacityInput} is not 0, {@code maps} must be a pointer to an array of {@code mapCapacityInput} {@link XrLocalizationMapML} structures
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link #XR_ERROR_LOCALIZATION_MAP_PERMISSION_DENIED_ML ERROR_LOCALIZATION_MAP_PERMISSION_DENIED_ML}
*
*
*
* See Also
*
* {@link XrLocalizationMapML}, {@link XrLocalizationMapQueryInfoBaseHeaderML}
*
* @param session a handle to an {@code XrSession} previously created with {@link XR10#xrCreateSession CreateSession}.
* @param queryInfo an optional enumeration filter based on {@link XrLocalizationMapQueryInfoBaseHeaderML} to use.
* @param mapCountOutput filled in by the runtime with the count of {@code maps} written or the required capacity in the case that {@code mapCapacityInput} is insufficient.
* @param maps an array of {@link XrLocalizationMapML} filled in by the runtime, but can be {@code NULL} if {@code mapCapacityInput} is 0.
*/
@NativeType("XrResult")
public static int xrQueryLocalizationMapsML(XrSession session, @NativeType("XrLocalizationMapQueryInfoBaseHeaderML const *") @Nullable XrLocalizationMapQueryInfoBaseHeaderML queryInfo, @NativeType("uint32_t *") IntBuffer mapCountOutput, @NativeType("XrLocalizationMapML *") XrLocalizationMapML.@Nullable Buffer maps) {
if (CHECKS) {
check(mapCountOutput, 1);
}
return nxrQueryLocalizationMapsML(session, memAddressSafe(queryInfo), remainingSafe(maps), memAddress(mapCountOutput), memAddressSafe(maps));
}
// --- [ xrRequestMapLocalizationML ] ---
/** Unsafe version of: {@link #xrRequestMapLocalizationML RequestMapLocalizationML} */
public static int nxrRequestMapLocalizationML(XrSession session, long requestInfo) {
long __functionAddress = session.getCapabilities().xrRequestMapLocalizationML;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(session.address(), requestInfo, __functionAddress);
}
/**
* Requests a localization map.
*
* C Specification
*
* The {@link #xrRequestMapLocalizationML RequestMapLocalizationML} function is defined as:
*
*
* XrResult xrRequestMapLocalizationML(
* XrSession session,
* const XrMapLocalizationRequestInfoML* requestInfo);
*
* Description
*
* This is an asynchronous request. Listen for {@link XrEventDataLocalizationChangedML} events to get the results of the localization. A new request for localization will override all the past requests for localization that are yet to be completed.
*
* The runtime must return {@link #XR_ERROR_LOCALIZATION_MAP_UNAVAILABLE_ML ERROR_LOCALIZATION_MAP_UNAVAILABLE_ML} if the requested is not a map known to the runtime.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLLocalizationMap XR_ML_localization_map} extension must be enabled prior to calling {@link #xrRequestMapLocalizationML RequestMapLocalizationML}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code requestInfo} must be a pointer to a valid {@link XrMapLocalizationRequestInfoML} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link #XR_ERROR_LOCALIZATION_MAP_UNAVAILABLE_ML ERROR_LOCALIZATION_MAP_UNAVAILABLE_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_PERMISSION_DENIED_ML ERROR_LOCALIZATION_MAP_PERMISSION_DENIED_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_FAIL_ML ERROR_LOCALIZATION_MAP_FAIL_ML}
*
*
*
* See Also
*
* {@link XrMapLocalizationRequestInfoML}
*
* @param session a handle to an {@code XrSession} previously created with {@link XR10#xrCreateSession CreateSession}.
* @param requestInfo contains {@link XrMapLocalizationRequestInfoML} on the localization map to request.
*/
@NativeType("XrResult")
public static int xrRequestMapLocalizationML(XrSession session, @NativeType("XrMapLocalizationRequestInfoML const *") XrMapLocalizationRequestInfoML requestInfo) {
return nxrRequestMapLocalizationML(session, requestInfo.address());
}
// --- [ xrImportLocalizationMapML ] ---
/** Unsafe version of: {@link #xrImportLocalizationMapML ImportLocalizationMapML} */
public static int nxrImportLocalizationMapML(XrSession session, long importInfo, long mapUuid) {
long __functionAddress = session.getCapabilities().xrImportLocalizationMapML;
if (CHECKS) {
check(__functionAddress);
XrLocalizationMapImportInfoML.validate(importInfo);
}
return callPPPI(session.address(), importInfo, mapUuid, __functionAddress);
}
/**
* Imports a localization map.
*
* C Specification
*
* The {@link #xrImportLocalizationMapML ImportLocalizationMapML} function is defined as:
*
*
* XrResult xrImportLocalizationMapML(
* XrSession session,
* const XrLocalizationMapImportInfoML* importInfo,
* XrUuidEXT* mapUuid);
*
* Description
*
* The runtime must return {@link #XR_ERROR_LOCALIZATION_MAP_ALREADY_EXISTS_ML ERROR_LOCALIZATION_MAP_ALREADY_EXISTS_ML} if the map that is being imported already exists. The runtime must return {@link #XR_ERROR_LOCALIZATION_MAP_INCOMPATIBLE_ML ERROR_LOCALIZATION_MAP_INCOMPATIBLE_ML} if the map being imported is not compatible.
*
* {@link #xrImportLocalizationMapML ImportLocalizationMapML} may take a long time to complete; as such applications should not call this from the frame loop.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLLocalizationMap XR_ML_localization_map} extension must be enabled prior to calling {@link #xrImportLocalizationMapML ImportLocalizationMapML}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code importInfo} must be a pointer to a valid {@link XrLocalizationMapImportInfoML} structure
* - If {@code mapUuid} is not {@code NULL}, {@code mapUuid} must be a pointer to an {@link XrUuidEXT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link #XR_ERROR_LOCALIZATION_MAP_INCOMPATIBLE_ML ERROR_LOCALIZATION_MAP_INCOMPATIBLE_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_IMPORT_EXPORT_PERMISSION_DENIED_ML ERROR_LOCALIZATION_MAP_IMPORT_EXPORT_PERMISSION_DENIED_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_ALREADY_EXISTS_ML ERROR_LOCALIZATION_MAP_ALREADY_EXISTS_ML}
*
*
*
* See Also
*
* {@link XrLocalizationMapImportInfoML}, {@link XrUuidEXT}
*
* @param session a handle to an {@code XrSession} previously created with {@link XR10#xrCreateSession CreateSession}.
* @param importInfo contains {@link XrLocalizationMapImportInfoML} on the localization map to import.
* @param mapUuid the {@link XrUuidEXT} of the newly imported localization map filled in by the runtime.
*/
@NativeType("XrResult")
public static int xrImportLocalizationMapML(XrSession session, @NativeType("XrLocalizationMapImportInfoML const *") XrLocalizationMapImportInfoML importInfo, @NativeType("XrUuidEXT *") @Nullable XrUuidEXT mapUuid) {
return nxrImportLocalizationMapML(session, importInfo.address(), memAddressSafe(mapUuid));
}
// --- [ xrCreateExportedLocalizationMapML ] ---
/** Unsafe version of: {@link #xrCreateExportedLocalizationMapML CreateExportedLocalizationMapML} */
public static int nxrCreateExportedLocalizationMapML(XrSession session, long mapUuid, long map) {
long __functionAddress = session.getCapabilities().xrCreateExportedLocalizationMapML;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), mapUuid, map, __functionAddress);
}
/**
* Creates an exported localization map handle.
*
* C Specification
*
* The {@link #xrCreateExportedLocalizationMapML CreateExportedLocalizationMapML} function is defined as:
*
*
* XrResult xrCreateExportedLocalizationMapML(
* XrSession session,
* const XrUuidEXT* mapUuid,
* XrExportedLocalizationMapML* map);
*
* Description
*
* {@link #xrCreateExportedLocalizationMapML CreateExportedLocalizationMapML} creates a frozen copy of the {@code mapUuid} localization map that can be exported using {@link #xrGetExportedLocalizationMapDataML GetExportedLocalizationMapDataML}. Applications should call {@link #xrDestroyExportedLocalizationMapML DestroyExportedLocalizationMapML} once they are done with the data.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLLocalizationMap XR_ML_localization_map} extension must be enabled prior to calling {@link #xrCreateExportedLocalizationMapML CreateExportedLocalizationMapML}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code mapUuid} must be a pointer to a valid {@link XrUuidEXT} structure
* - {@code map} must be a pointer to an {@code XrExportedLocalizationMapML} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link #XR_ERROR_LOCALIZATION_MAP_UNAVAILABLE_ML ERROR_LOCALIZATION_MAP_UNAVAILABLE_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_IMPORT_EXPORT_PERMISSION_DENIED_ML ERROR_LOCALIZATION_MAP_IMPORT_EXPORT_PERMISSION_DENIED_ML}
* - {@link #XR_ERROR_LOCALIZATION_MAP_CANNOT_EXPORT_CLOUD_MAP_ML ERROR_LOCALIZATION_MAP_CANNOT_EXPORT_CLOUD_MAP_ML}
*
*
*
* See Also
*
* {@link XrUuidEXT}, {@link #xrGetExportedLocalizationMapDataML GetExportedLocalizationMapDataML}
*
* @param session a handle to an {@code XrSession} previously created with {@link XR10#xrCreateSession CreateSession}.
* @param mapUuid a pointer to the uuid of the map to export.
* @param map a pointer to a map handle filled in by the runtime.
*/
@NativeType("XrResult")
public static int xrCreateExportedLocalizationMapML(XrSession session, @NativeType("XrUuidEXT const *") XrUuidEXT mapUuid, @NativeType("XrExportedLocalizationMapML *") PointerBuffer map) {
if (CHECKS) {
check(map, 1);
}
return nxrCreateExportedLocalizationMapML(session, mapUuid.address(), memAddress(map));
}
// --- [ xrDestroyExportedLocalizationMapML ] ---
/**
* Destroys an exported localization map.
*
* C Specification
*
* The {@link #xrDestroyExportedLocalizationMapML DestroyExportedLocalizationMapML} function is defined as:
*
*
* XrResult xrDestroyExportedLocalizationMapML(
* XrExportedLocalizationMapML map);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLLocalizationMap XR_ML_localization_map} extension must be enabled prior to calling {@link #xrDestroyExportedLocalizationMapML DestroyExportedLocalizationMapML}
* - {@code map} must be a valid {@code XrExportedLocalizationMapML} handle
*
*
* Thread Safety
*
*
* - Access to {@code map}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* See Also
*
* {@link #xrCreateExportedLocalizationMapML CreateExportedLocalizationMapML}
*
* @param map the map to destroy.
*/
@NativeType("XrResult")
public static int xrDestroyExportedLocalizationMapML(XrExportedLocalizationMapML map) {
long __functionAddress = map.getCapabilities().xrDestroyExportedLocalizationMapML;
if (CHECKS) {
check(__functionAddress);
}
return callPI(map.address(), __functionAddress);
}
// --- [ xrGetExportedLocalizationMapDataML ] ---
/**
* Unsafe version of: {@link #xrGetExportedLocalizationMapDataML GetExportedLocalizationMapDataML}
*
* @param bufferCapacityInput the capacity of the buffer array, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrGetExportedLocalizationMapDataML(XrExportedLocalizationMapML map, int bufferCapacityInput, long bufferCountOutput, long buffer) {
long __functionAddress = map.getCapabilities().xrGetExportedLocalizationMapDataML;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(map.address(), bufferCapacityInput, bufferCountOutput, buffer, __functionAddress);
}
/**
* Returns the exported localization map data.
*
* C Specification
*
* The {@link #xrGetExportedLocalizationMapDataML GetExportedLocalizationMapDataML} function is defined as:
*
*
* XrResult xrGetExportedLocalizationMapDataML(
* XrExportedLocalizationMapML map,
* uint32_t bufferCapacityInput,
* uint32_t* bufferCountOutput,
* char* buffer);
*
* Description
*
* {@link #xrGetExportedLocalizationMapDataML GetExportedLocalizationMapDataML} may take a long time to complete; as such applications should not call this from the frame loop.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLLocalizationMap XR_ML_localization_map} extension must be enabled prior to calling {@link #xrGetExportedLocalizationMapDataML GetExportedLocalizationMapDataML}
* - {@code map} must be a valid {@code XrExportedLocalizationMapML} handle
* - {@code bufferCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code bufferCapacityInput} is not 0, {@code buffer} must be a pointer to an array of {@code bufferCapacityInput} char values
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* See Also
*
* {@link #xrCreateExportedLocalizationMapML CreateExportedLocalizationMapML}
*
* @param map the map to export.
* @param bufferCountOutput filled in by the runtime with the count of bytes written or the required capacity in the case that bufferCapacityInput is insufficient.
* @param buffer an array of bytes filled in by the runtime.
*/
@NativeType("XrResult")
public static int xrGetExportedLocalizationMapDataML(XrExportedLocalizationMapML map, @NativeType("uint32_t *") IntBuffer bufferCountOutput, @NativeType("char *") @Nullable ByteBuffer buffer) {
if (CHECKS) {
check(bufferCountOutput, 1);
}
return nxrGetExportedLocalizationMapDataML(map, remainingSafe(buffer), memAddress(bufferCountOutput), memAddressSafe(buffer));
}
}