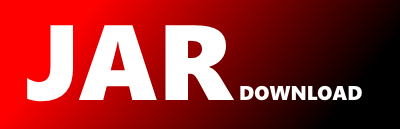
org.lwjgl.openxr.MLSpatialAnchors Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_ML_spatial_anchors extension.
*
* Spatial anchors are {@code XrSpace} entities tied to a physical location. This allows the developer to place virtual content in real world locations.
*
* The runtime should then adjust the {@code XrSpace} over time as needed, independently of all other spaces and anchors, to ensure that it maintains its original mapping to the real world.
*
* Caution
*
* If head pose is lost and regained, spatial anchors may also be lost. It is therefore strongly recommended that once an anchor is created, it is also persisted using the {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension.
*
* Permissions
*
* Android applications must have the com.magicleap.permission.SPATIAL_ANCHOR permission listed in their manifest to use this extension. (protection level: normal)
*/
public class MLSpatialAnchors {
/** The extension specification version. */
public static final int XR_ML_spatial_anchors_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_ML_SPATIAL_ANCHORS_EXTENSION_NAME = "XR_ML_spatial_anchors";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SPATIAL_ANCHORS_CREATE_INFO_FROM_POSE_ML TYPE_SPATIAL_ANCHORS_CREATE_INFO_FROM_POSE_ML}
* - {@link #XR_TYPE_CREATE_SPATIAL_ANCHORS_COMPLETION_ML TYPE_CREATE_SPATIAL_ANCHORS_COMPLETION_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHOR_STATE_ML TYPE_SPATIAL_ANCHOR_STATE_ML}
*
*/
public static final int
XR_TYPE_SPATIAL_ANCHORS_CREATE_INFO_FROM_POSE_ML = 1000140000,
XR_TYPE_CREATE_SPATIAL_ANCHORS_COMPLETION_ML = 1000140001,
XR_TYPE_SPATIAL_ANCHOR_STATE_ML = 1000140002;
/**
* Extends {@code XrResult}.
*
* Enum values:
*
*
* - {@link #XR_ERROR_SPATIAL_ANCHORS_PERMISSION_DENIED_ML ERROR_SPATIAL_ANCHORS_PERMISSION_DENIED_ML}
* - {@link #XR_ERROR_SPATIAL_ANCHORS_NOT_LOCALIZED_ML ERROR_SPATIAL_ANCHORS_NOT_LOCALIZED_ML}
* - {@link #XR_ERROR_SPATIAL_ANCHORS_OUT_OF_MAP_BOUNDS_ML ERROR_SPATIAL_ANCHORS_OUT_OF_MAP_BOUNDS_ML}
* - {@link #XR_ERROR_SPATIAL_ANCHORS_SPACE_NOT_LOCATABLE_ML ERROR_SPATIAL_ANCHORS_SPACE_NOT_LOCATABLE_ML}
*
*/
public static final int
XR_ERROR_SPATIAL_ANCHORS_PERMISSION_DENIED_ML = -1000140000,
XR_ERROR_SPATIAL_ANCHORS_NOT_LOCALIZED_ML = -1000140001,
XR_ERROR_SPATIAL_ANCHORS_OUT_OF_MAP_BOUNDS_ML = -1000140002,
XR_ERROR_SPATIAL_ANCHORS_SPACE_NOT_LOCATABLE_ML = -1000140003;
/**
* XrSpatialAnchorConfidenceML - Spatial anchor confidence
*
* Description
*
*
* Enum Description
*
* {@link #XR_SPATIAL_ANCHOR_CONFIDENCE_LOW_ML SPATIAL_ANCHOR_CONFIDENCE_LOW_ML} Low quality, this anchor can be expected to move significantly.
* {@link #XR_SPATIAL_ANCHOR_CONFIDENCE_MEDIUM_ML SPATIAL_ANCHOR_CONFIDENCE_MEDIUM_ML} Medium quality, this anchor may move slightly.
* {@link #XR_SPATIAL_ANCHOR_CONFIDENCE_HIGH_ML SPATIAL_ANCHOR_CONFIDENCE_HIGH_ML} High quality, this anchor is stable and suitable for digital content attachment.
*
*
*
* See Also
*
* {@link XrSpatialAnchorStateML}
*/
public static final int
XR_SPATIAL_ANCHOR_CONFIDENCE_LOW_ML = 0,
XR_SPATIAL_ANCHOR_CONFIDENCE_MEDIUM_ML = 1,
XR_SPATIAL_ANCHOR_CONFIDENCE_HIGH_ML = 2;
protected MLSpatialAnchors() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateSpatialAnchorsAsyncML ] ---
/** Unsafe version of: {@link #xrCreateSpatialAnchorsAsyncML CreateSpatialAnchorsAsyncML} */
public static int nxrCreateSpatialAnchorsAsyncML(XrSession session, long createInfo, long future) {
long __functionAddress = session.getCapabilities().xrCreateSpatialAnchorsAsyncML;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), createInfo, future, __functionAddress);
}
/**
* Create spatial anchors.
*
* C Specification
*
* The {@link #xrCreateSpatialAnchorsAsyncML CreateSpatialAnchorsAsyncML} function is defined as:
*
*
* XrResult xrCreateSpatialAnchorsAsyncML(
* XrSession session,
* const XrSpatialAnchorsCreateInfoBaseHeaderML* createInfo,
* XrFutureEXT* future);
*
* Description
*
* This function starts an asynchronous spatial anchor creation. Call one of the {@link EXTFuture#xrPollFutureEXT PollFutureEXT} functions to check the ready state on the future. Once the future is in ready state, call {@link #xrCreateSpatialAnchorsCompleteML CreateSpatialAnchorsCompleteML} to retrieve the results.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchors XR_ML_spatial_anchors} extension must be enabled prior to calling {@link #xrCreateSpatialAnchorsAsyncML CreateSpatialAnchorsAsyncML}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrSpatialAnchorsCreateInfoBaseHeaderML}-based structure. See also: {@link XrSpatialAnchorsCreateInfoFromPoseML}, {@link XrSpatialAnchorsCreateInfoFromUuidsML}
* - {@code future} must be a pointer to an {@code XrFutureEXT} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
* - {@link #XR_ERROR_SPATIAL_ANCHORS_SPACE_NOT_LOCATABLE_ML ERROR_SPATIAL_ANCHORS_SPACE_NOT_LOCATABLE_ML}
* - {@link #XR_ERROR_SPATIAL_ANCHORS_PERMISSION_DENIED_ML ERROR_SPATIAL_ANCHORS_PERMISSION_DENIED_ML}
* - {@link XR10#XR_ERROR_POSE_INVALID ERROR_POSE_INVALID}
*
*
*
* See Also
*
* {@link XrSpatialAnchorsCreateInfoBaseHeaderML}, {@link XrSpatialAnchorsCreateInfoFromPoseML}, {@link #xrCreateSpatialAnchorsCompleteML CreateSpatialAnchorsCompleteML}
*
* @param session a handle to an {@code XrSession} previously created with {@link XR10#xrCreateSession CreateSession}.
* @param createInfo a pointer to an {@link XrSpatialAnchorsCreateInfoBaseHeaderML} derived structure.
* @param future a pointer to an {@code XrFutureEXT}.
*/
@NativeType("XrResult")
public static int xrCreateSpatialAnchorsAsyncML(XrSession session, @NativeType("XrSpatialAnchorsCreateInfoBaseHeaderML const *") XrSpatialAnchorsCreateInfoBaseHeaderML createInfo, @NativeType("XrFutureEXT *") LongBuffer future) {
if (CHECKS) {
check(future, 1);
}
return nxrCreateSpatialAnchorsAsyncML(session, createInfo.address(), memAddress(future));
}
// --- [ xrCreateSpatialAnchorsCompleteML ] ---
/** Unsafe version of: {@link #xrCreateSpatialAnchorsCompleteML CreateSpatialAnchorsCompleteML} */
public static int nxrCreateSpatialAnchorsCompleteML(XrSession session, long future, long completion) {
long __functionAddress = session.getCapabilities().xrCreateSpatialAnchorsCompleteML;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(session.address(), future, completion, __functionAddress);
}
/**
* Completion function for spatial anchor creation.
*
* C Specification
*
* The {@link #xrCreateSpatialAnchorsCompleteML CreateSpatialAnchorsCompleteML} function is defined as:
*
*
* XrResult xrCreateSpatialAnchorsCompleteML(
* XrSession session,
* XrFutureEXT future,
* XrCreateSpatialAnchorsCompletionML* completion);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchors XR_ML_spatial_anchors} extension must be enabled prior to calling {@link #xrCreateSpatialAnchorsCompleteML CreateSpatialAnchorsCompleteML}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code completion} must be a pointer to an {@link XrCreateSpatialAnchorsCompletionML} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link EXTFuture#XR_ERROR_FUTURE_PENDING_EXT ERROR_FUTURE_PENDING_EXT}
* - {@link EXTFuture#XR_ERROR_FUTURE_INVALID_EXT ERROR_FUTURE_INVALID_EXT}
*
*
*
* See Also
*
* {@link XrCreateSpatialAnchorsCompletionML}, {@link #xrCreateSpatialAnchorsAsyncML CreateSpatialAnchorsAsyncML}
*
* @param session a handle to an {@code XrSession} previously created with {@link XR10#xrCreateSession CreateSession}.
* @param future the {@code XrFutureEXT} to complete.
* @param completion a pointer to an {@link XrCreateSpatialAnchorsCompletionML} filled in by the runtime.
*/
@NativeType("XrResult")
public static int xrCreateSpatialAnchorsCompleteML(XrSession session, @NativeType("XrFutureEXT") long future, @NativeType("XrCreateSpatialAnchorsCompletionML *") XrCreateSpatialAnchorsCompletionML completion) {
return nxrCreateSpatialAnchorsCompleteML(session, future, completion.address());
}
// --- [ xrGetSpatialAnchorStateML ] ---
/** Unsafe version of: {@link #xrGetSpatialAnchorStateML GetSpatialAnchorStateML} */
public static int nxrGetSpatialAnchorStateML(XrSpace anchor, long state) {
long __functionAddress = anchor.getCapabilities().xrGetSpatialAnchorStateML;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(anchor.address(), state, __functionAddress);
}
/**
* Retrieve spatial anchor state.
*
* C Specification
*
* The {@link #xrGetSpatialAnchorStateML GetSpatialAnchorStateML} function is defined as:
*
*
* XrResult xrGetSpatialAnchorStateML(
* XrSpace anchor,
* XrSpatialAnchorStateML* state);
*
* Description
*
* The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if the {@code XrSpace} is not an spatial anchor.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchors XR_ML_spatial_anchors} extension must be enabled prior to calling {@link #xrGetSpatialAnchorStateML GetSpatialAnchorStateML}
* - {@code anchor} must be a valid {@code XrSpace} handle
* - {@code state} must be a pointer to an {@link XrSpatialAnchorStateML} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* See Also
*
* {@link XrCreateSpatialAnchorsCompletionML}, {@link XrSpatialAnchorStateML}, {@link #xrCreateSpatialAnchorsAsyncML CreateSpatialAnchorsAsyncML}
*
* @param anchor a handle to an {@code XrSpace} corresponding to a spatial anchor.
* @param state a pointer to an {@link XrSpatialAnchorStateML} structure to populate with the spatial anchor state.
*/
@NativeType("XrResult")
public static int xrGetSpatialAnchorStateML(XrSpace anchor, @NativeType("XrSpatialAnchorStateML *") XrSpatialAnchorStateML state) {
return nxrGetSpatialAnchorStateML(anchor, state.address());
}
}