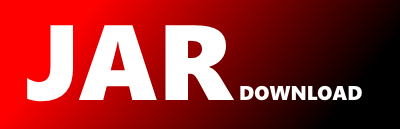
org.lwjgl.openxr.MLSpatialAnchorsStorage Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_ML_spatial_anchors_storage extension.
*
* This extension allows spatial anchors created by {@link MLSpatialAnchors XR_ML_spatial_anchors} to be persisted beyond the head pose session.
*
* Spatial anchor management is closely tied to the selected mapping mode on the device. The modes are mutually exclusive and affect the functionality of these APIs. The available mapping modes are:
*
*
* - On-Device Mode
* - A persistent mode in which anchors are persisted locally and will be available across multiple sessions when localized to the same localization map in which they were published.
* - AR Cloud Mode
* - A persistent mode in which anchors are persisted in the cloud environment and will be available across multiple sessions and across multiple devices that are localized to the same localization map in which they were published.
*
*
* For more details on mapping modes refer to the {@link MLLocalizationMap XR_ML_localization_map} extension.
*
* Permissions
*
* Android applications must have the com.magicleap.permission.SPATIAL_ANCHOR permission listed in their manifest to use this extension. (protection level: normal)
*/
public class MLSpatialAnchorsStorage {
/** The extension specification version. */
public static final int XR_ML_spatial_anchors_storage_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_ML_SPATIAL_ANCHORS_STORAGE_EXTENSION_NAME = "XR_ML_spatial_anchors_storage";
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_SPATIAL_ANCHORS_STORAGE_ML = 1000141000;
/** Extends {@code XrResult}. */
public static final int XR_ERROR_SPATIAL_ANCHORS_ANCHOR_NOT_FOUND_ML = -1000141000;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SPATIAL_ANCHORS_CREATE_STORAGE_INFO_ML TYPE_SPATIAL_ANCHORS_CREATE_STORAGE_INFO_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_QUERY_INFO_RADIUS_ML TYPE_SPATIAL_ANCHORS_QUERY_INFO_RADIUS_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_QUERY_COMPLETION_ML TYPE_SPATIAL_ANCHORS_QUERY_COMPLETION_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_CREATE_INFO_FROM_UUIDS_ML TYPE_SPATIAL_ANCHORS_CREATE_INFO_FROM_UUIDS_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_PUBLISH_INFO_ML TYPE_SPATIAL_ANCHORS_PUBLISH_INFO_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_PUBLISH_COMPLETION_ML TYPE_SPATIAL_ANCHORS_PUBLISH_COMPLETION_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_DELETE_INFO_ML TYPE_SPATIAL_ANCHORS_DELETE_INFO_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_DELETE_COMPLETION_ML TYPE_SPATIAL_ANCHORS_DELETE_COMPLETION_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_UPDATE_EXPIRATION_INFO_ML TYPE_SPATIAL_ANCHORS_UPDATE_EXPIRATION_INFO_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_UPDATE_EXPIRATION_COMPLETION_ML TYPE_SPATIAL_ANCHORS_UPDATE_EXPIRATION_COMPLETION_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_PUBLISH_COMPLETION_DETAILS_ML TYPE_SPATIAL_ANCHORS_PUBLISH_COMPLETION_DETAILS_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_DELETE_COMPLETION_DETAILS_ML TYPE_SPATIAL_ANCHORS_DELETE_COMPLETION_DETAILS_ML}
* - {@link #XR_TYPE_SPATIAL_ANCHORS_UPDATE_EXPIRATION_COMPLETION_DETAILS_ML TYPE_SPATIAL_ANCHORS_UPDATE_EXPIRATION_COMPLETION_DETAILS_ML}
*
*/
public static final int
XR_TYPE_SPATIAL_ANCHORS_CREATE_STORAGE_INFO_ML = 1000141000,
XR_TYPE_SPATIAL_ANCHORS_QUERY_INFO_RADIUS_ML = 1000141001,
XR_TYPE_SPATIAL_ANCHORS_QUERY_COMPLETION_ML = 1000141002,
XR_TYPE_SPATIAL_ANCHORS_CREATE_INFO_FROM_UUIDS_ML = 1000141003,
XR_TYPE_SPATIAL_ANCHORS_PUBLISH_INFO_ML = 1000141004,
XR_TYPE_SPATIAL_ANCHORS_PUBLISH_COMPLETION_ML = 1000141005,
XR_TYPE_SPATIAL_ANCHORS_DELETE_INFO_ML = 1000141006,
XR_TYPE_SPATIAL_ANCHORS_DELETE_COMPLETION_ML = 1000141007,
XR_TYPE_SPATIAL_ANCHORS_UPDATE_EXPIRATION_INFO_ML = 1000141008,
XR_TYPE_SPATIAL_ANCHORS_UPDATE_EXPIRATION_COMPLETION_ML = 1000141009,
XR_TYPE_SPATIAL_ANCHORS_PUBLISH_COMPLETION_DETAILS_ML = 1000141010,
XR_TYPE_SPATIAL_ANCHORS_DELETE_COMPLETION_DETAILS_ML = 1000141011,
XR_TYPE_SPATIAL_ANCHORS_UPDATE_EXPIRATION_COMPLETION_DETAILS_ML = 1000141012;
protected MLSpatialAnchorsStorage() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateSpatialAnchorsStorageML ] ---
/** Unsafe version of: {@link #xrCreateSpatialAnchorsStorageML CreateSpatialAnchorsStorageML} */
public static int nxrCreateSpatialAnchorsStorageML(XrSession session, long createInfo, long storage) {
long __functionAddress = session.getCapabilities().xrCreateSpatialAnchorsStorageML;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), createInfo, storage, __functionAddress);
}
/**
* Create spatial anchors storage connection.
*
* C Specification
*
* The {@link #xrCreateSpatialAnchorsStorageML CreateSpatialAnchorsStorageML} function is defined as:
*
*
* XrResult xrCreateSpatialAnchorsStorageML(
* XrSession session,
* const XrSpatialAnchorsCreateStorageInfoML* createInfo,
* XrSpatialAnchorsStorageML* storage);
*
* Description
*
* The {@link #xrCreateSpatialAnchorsStorageML CreateSpatialAnchorsStorageML} function is used to create a {@code XrSpatialAnchorsStorageML}.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension must be enabled prior to calling {@link #xrCreateSpatialAnchorsStorageML CreateSpatialAnchorsStorageML}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrSpatialAnchorsCreateStorageInfoML} structure
* - {@code storage} must be a pointer to an {@code XrSpatialAnchorsStorageML} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link MLSpatialAnchors#XR_ERROR_SPATIAL_ANCHORS_PERMISSION_DENIED_ML ERROR_SPATIAL_ANCHORS_PERMISSION_DENIED_ML}
*
*
*
* See Also
*
* {@link XrSpatialAnchorsCreateStorageInfoML}
*
* @param session a handle to an {@code XrSession} previously created with {@link XR10#xrCreateSession CreateSession}.
* @param createInfo a pointer to an {@link XrSpatialAnchorsCreateInfoBaseHeaderML} derived structure.
* @param storage a pointer to an {@code XrSpatialAnchorsStorageML} where the created storage is returned.
*/
@NativeType("XrResult")
public static int xrCreateSpatialAnchorsStorageML(XrSession session, @NativeType("XrSpatialAnchorsCreateStorageInfoML const *") XrSpatialAnchorsCreateStorageInfoML createInfo, @NativeType("XrSpatialAnchorsStorageML *") PointerBuffer storage) {
if (CHECKS) {
check(storage, 1);
}
return nxrCreateSpatialAnchorsStorageML(session, createInfo.address(), memAddress(storage));
}
// --- [ xrDestroySpatialAnchorsStorageML ] ---
/**
* Destroy spatial anchors storage.
*
* C Specification
*
* The {@link #xrDestroySpatialAnchorsStorageML DestroySpatialAnchorsStorageML} function is defined as:
*
*
* XrResult xrDestroySpatialAnchorsStorageML(
* XrSpatialAnchorsStorageML storage);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension must be enabled prior to calling {@link #xrDestroySpatialAnchorsStorageML DestroySpatialAnchorsStorageML}
* - {@code storage} must be a valid {@code XrSpatialAnchorsStorageML} handle
*
*
* Thread Safety
*
*
* - Access to {@code storage}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* See Also
*
* {@link #xrCreateSpatialAnchorsStorageML CreateSpatialAnchorsStorageML}
*
* @param storage the {@code XrSpatialAnchorsStorageML} to destroy.
*/
@NativeType("XrResult")
public static int xrDestroySpatialAnchorsStorageML(XrSpatialAnchorsStorageML storage) {
long __functionAddress = storage.getCapabilities().xrDestroySpatialAnchorsStorageML;
if (CHECKS) {
check(__functionAddress);
}
return callPI(storage.address(), __functionAddress);
}
// --- [ xrQuerySpatialAnchorsAsyncML ] ---
/** Unsafe version of: {@link #xrQuerySpatialAnchorsAsyncML QuerySpatialAnchorsAsyncML} */
public static int nxrQuerySpatialAnchorsAsyncML(XrSpatialAnchorsStorageML storage, long queryInfo, long future) {
long __functionAddress = storage.getCapabilities().xrQuerySpatialAnchorsAsyncML;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(storage.address(), queryInfo, future, __functionAddress);
}
/**
* Begin a storage query.
*
* C Specification
*
* The {@link #xrQuerySpatialAnchorsAsyncML QuerySpatialAnchorsAsyncML} function is defined as:
*
*
* XrResult xrQuerySpatialAnchorsAsyncML(
* XrSpatialAnchorsStorageML storage,
* const XrSpatialAnchorsQueryInfoBaseHeaderML* queryInfo,
* XrFutureEXT* future);
*
* Description
*
* If the space was not locatable during the query the runtime must return {@link EXTPlaneDetection#XR_ERROR_SPACE_NOT_LOCATABLE_EXT ERROR_SPACE_NOT_LOCATABLE_EXT} in {@link XrSpatialAnchorsQueryCompletionML}{@code ::futureResult}.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension must be enabled prior to calling {@link #xrQuerySpatialAnchorsAsyncML QuerySpatialAnchorsAsyncML}
* - {@code storage} must be a valid {@code XrSpatialAnchorsStorageML} handle
* - {@code queryInfo} must be a pointer to a valid {@link XrSpatialAnchorsQueryInfoBaseHeaderML}-based structure. See also: {@link XrSpatialAnchorsQueryInfoRadiusML}
* - {@code future} must be a pointer to an {@code XrFutureEXT} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
* - {@link MLSpatialAnchors#XR_ERROR_SPATIAL_ANCHORS_SPACE_NOT_LOCATABLE_ML ERROR_SPATIAL_ANCHORS_SPACE_NOT_LOCATABLE_ML}
*
*
*
* See Also
*
* {@link XrSpatialAnchorsQueryInfoBaseHeaderML}
*
* @param storage the {@code XrSpatialAnchorsStorageML} to use.
* @param queryInfo the {@link XrSpatialAnchorsQueryInfoBaseHeaderML} structure used to define the query. {@link XrSpatialAnchorsQueryInfoRadiusML} allows querying around a pose.
* @param future a pointer the created {@code XrFutureEXT}.
*/
@NativeType("XrResult")
public static int xrQuerySpatialAnchorsAsyncML(XrSpatialAnchorsStorageML storage, @NativeType("XrSpatialAnchorsQueryInfoBaseHeaderML const *") XrSpatialAnchorsQueryInfoBaseHeaderML queryInfo, @NativeType("XrFutureEXT *") LongBuffer future) {
if (CHECKS) {
check(future, 1);
}
return nxrQuerySpatialAnchorsAsyncML(storage, queryInfo.address(), memAddress(future));
}
// --- [ xrQuerySpatialAnchorsCompleteML ] ---
/** Unsafe version of: {@link #xrQuerySpatialAnchorsCompleteML QuerySpatialAnchorsCompleteML} */
public static int nxrQuerySpatialAnchorsCompleteML(XrSpatialAnchorsStorageML storage, long future, long completion) {
long __functionAddress = storage.getCapabilities().xrQuerySpatialAnchorsCompleteML;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(storage.address(), future, completion, __functionAddress);
}
/**
* Complete the asynchronous query operation.
*
* C Specification
*
* The {@link #xrQuerySpatialAnchorsCompleteML QuerySpatialAnchorsCompleteML} function is defined as:
*
*
* XrResult xrQuerySpatialAnchorsCompleteML(
* XrSpatialAnchorsStorageML storage,
* XrFutureEXT future,
* XrSpatialAnchorsQueryCompletionML* completion);
*
* Description
*
* Once the {@code XrFutureEXT} has completed {@link #xrQuerySpatialAnchorsCompleteML QuerySpatialAnchorsCompleteML} must be called to retrieve the {@link XrUuidEXT} values of the found anchors.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension must be enabled prior to calling {@link #xrQuerySpatialAnchorsCompleteML QuerySpatialAnchorsCompleteML}
* - {@code storage} must be a valid {@code XrSpatialAnchorsStorageML} handle
* - {@code completion} must be a pointer to an {@link XrSpatialAnchorsQueryCompletionML} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link EXTFuture#XR_ERROR_FUTURE_PENDING_EXT ERROR_FUTURE_PENDING_EXT}
* - {@link EXTFuture#XR_ERROR_FUTURE_INVALID_EXT ERROR_FUTURE_INVALID_EXT}
*
*
*
* See Also
*
* {@link XrSpatialAnchorsQueryCompletionML}, {@link #xrQuerySpatialAnchorsAsyncML QuerySpatialAnchorsAsyncML}
*
* @param storage the {@code XrSpatialAnchorsStorageML} used to start the query.
* @param future the {@code XrFutureEXT} of the future to complete.
* @param completion a pointer to an {@link XrSpatialAnchorsQueryCompletionML} structure containing the result of the operation.
*/
@NativeType("XrResult")
public static int xrQuerySpatialAnchorsCompleteML(XrSpatialAnchorsStorageML storage, @NativeType("XrFutureEXT") long future, @NativeType("XrSpatialAnchorsQueryCompletionML *") XrSpatialAnchorsQueryCompletionML completion) {
return nxrQuerySpatialAnchorsCompleteML(storage, future, completion.address());
}
// --- [ xrPublishSpatialAnchorsAsyncML ] ---
/** Unsafe version of: {@link #xrPublishSpatialAnchorsAsyncML PublishSpatialAnchorsAsyncML} */
public static int nxrPublishSpatialAnchorsAsyncML(XrSpatialAnchorsStorageML storage, long publishInfo, long future) {
long __functionAddress = storage.getCapabilities().xrPublishSpatialAnchorsAsyncML;
if (CHECKS) {
check(__functionAddress);
XrSpatialAnchorsPublishInfoML.validate(publishInfo);
}
return callPPPI(storage.address(), publishInfo, future, __functionAddress);
}
/**
* Begin a storage query.
*
* C Specification
*
* The {@link #xrPublishSpatialAnchorsAsyncML PublishSpatialAnchorsAsyncML} function is defined as:
*
*
* XrResult xrPublishSpatialAnchorsAsyncML(
* XrSpatialAnchorsStorageML storage,
* const XrSpatialAnchorsPublishInfoML* publishInfo,
* XrFutureEXT* future);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension must be enabled prior to calling {@link #xrPublishSpatialAnchorsAsyncML PublishSpatialAnchorsAsyncML}
* - {@code storage} must be a valid {@code XrSpatialAnchorsStorageML} handle
* - {@code publishInfo} must be a pointer to a valid {@link XrSpatialAnchorsPublishInfoML} structure
* - {@code future} must be a pointer to an {@code XrFutureEXT} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* See Also
*
* {@link XrSpatialAnchorsPublishInfoML}
*
* @param storage the {@code XrSpatialAnchorsStorageML} to use.
* @param publishInfo the {@link XrSpatialAnchorsPublishInfoML} structure use to specify the anchors to publish.
* @param future a pointer the created {@code XrFutureEXT}.
*/
@NativeType("XrResult")
public static int xrPublishSpatialAnchorsAsyncML(XrSpatialAnchorsStorageML storage, @NativeType("XrSpatialAnchorsPublishInfoML const *") XrSpatialAnchorsPublishInfoML publishInfo, @NativeType("XrFutureEXT *") LongBuffer future) {
if (CHECKS) {
check(future, 1);
}
return nxrPublishSpatialAnchorsAsyncML(storage, publishInfo.address(), memAddress(future));
}
// --- [ xrPublishSpatialAnchorsCompleteML ] ---
/** Unsafe version of: {@link #xrPublishSpatialAnchorsCompleteML PublishSpatialAnchorsCompleteML} */
public static int nxrPublishSpatialAnchorsCompleteML(XrSpatialAnchorsStorageML storage, long future, long completion) {
long __functionAddress = storage.getCapabilities().xrPublishSpatialAnchorsCompleteML;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(storage.address(), future, completion, __functionAddress);
}
/**
* Complete the asynchronous publish operation.
*
* C Specification
*
* The {@link #xrPublishSpatialAnchorsCompleteML PublishSpatialAnchorsCompleteML} function is defined as:
*
*
* XrResult xrPublishSpatialAnchorsCompleteML(
* XrSpatialAnchorsStorageML storage,
* XrFutureEXT future,
* XrSpatialAnchorsPublishCompletionML* completion);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension must be enabled prior to calling {@link #xrPublishSpatialAnchorsCompleteML PublishSpatialAnchorsCompleteML}
* - {@code storage} must be a valid {@code XrSpatialAnchorsStorageML} handle
* - {@code completion} must be a pointer to an {@link XrSpatialAnchorsPublishCompletionML} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link EXTFuture#XR_ERROR_FUTURE_PENDING_EXT ERROR_FUTURE_PENDING_EXT}
* - {@link EXTFuture#XR_ERROR_FUTURE_INVALID_EXT ERROR_FUTURE_INVALID_EXT}
*
*
*
* See Also
*
* {@link XrSpatialAnchorsPublishCompletionML}, {@link #xrPublishSpatialAnchorsAsyncML PublishSpatialAnchorsAsyncML}
*
* @param storage the {@code XrSpatialAnchorsStorageML} used to start the publish operation.
* @param future the {@code XrFutureEXT} of the future to complete.
* @param completion a pointer to a {@link XrSpatialAnchorsPublishCompletionML} structure containing the result of the operation.
*/
@NativeType("XrResult")
public static int xrPublishSpatialAnchorsCompleteML(XrSpatialAnchorsStorageML storage, @NativeType("XrFutureEXT") long future, @NativeType("XrSpatialAnchorsPublishCompletionML *") XrSpatialAnchorsPublishCompletionML completion) {
return nxrPublishSpatialAnchorsCompleteML(storage, future, completion.address());
}
// --- [ xrDeleteSpatialAnchorsAsyncML ] ---
/** Unsafe version of: {@link #xrDeleteSpatialAnchorsAsyncML DeleteSpatialAnchorsAsyncML} */
public static int nxrDeleteSpatialAnchorsAsyncML(XrSpatialAnchorsStorageML storage, long deleteInfo, long future) {
long __functionAddress = storage.getCapabilities().xrDeleteSpatialAnchorsAsyncML;
if (CHECKS) {
check(__functionAddress);
XrSpatialAnchorsDeleteInfoML.validate(deleteInfo);
}
return callPPPI(storage.address(), deleteInfo, future, __functionAddress);
}
/**
* Begin a storage query.
*
* C Specification
*
* The {@link #xrDeleteSpatialAnchorsAsyncML DeleteSpatialAnchorsAsyncML} function is defined as:
*
*
* XrResult xrDeleteSpatialAnchorsAsyncML(
* XrSpatialAnchorsStorageML storage,
* const XrSpatialAnchorsDeleteInfoML* deleteInfo,
* XrFutureEXT* future);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension must be enabled prior to calling {@link #xrDeleteSpatialAnchorsAsyncML DeleteSpatialAnchorsAsyncML}
* - {@code storage} must be a valid {@code XrSpatialAnchorsStorageML} handle
* - {@code deleteInfo} must be a pointer to a valid {@link XrSpatialAnchorsDeleteInfoML} structure
* - {@code future} must be a pointer to an {@code XrFutureEXT} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* See Also
*
* {@link XrSpatialAnchorsDeleteInfoML}, {@link #xrDeleteSpatialAnchorsCompleteML DeleteSpatialAnchorsCompleteML}
*
* @param storage the {@code XrSpatialAnchorsStorageML} to use.
* @param deleteInfo the {@link XrSpatialAnchorsDeleteInfoML} structure used to specify the anchors to delete.
* @param future a pointer the created {@code XrFutureEXT}.
*/
@NativeType("XrResult")
public static int xrDeleteSpatialAnchorsAsyncML(XrSpatialAnchorsStorageML storage, @NativeType("XrSpatialAnchorsDeleteInfoML const *") XrSpatialAnchorsDeleteInfoML deleteInfo, @NativeType("XrFutureEXT *") LongBuffer future) {
if (CHECKS) {
check(future, 1);
}
return nxrDeleteSpatialAnchorsAsyncML(storage, deleteInfo.address(), memAddress(future));
}
// --- [ xrDeleteSpatialAnchorsCompleteML ] ---
/** Unsafe version of: {@link #xrDeleteSpatialAnchorsCompleteML DeleteSpatialAnchorsCompleteML} */
public static int nxrDeleteSpatialAnchorsCompleteML(XrSpatialAnchorsStorageML storage, long future, long completion) {
long __functionAddress = storage.getCapabilities().xrDeleteSpatialAnchorsCompleteML;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(storage.address(), future, completion, __functionAddress);
}
/**
* Complete the asynchronous deletion operation.
*
* C Specification
*
* The {@link #xrDeleteSpatialAnchorsCompleteML DeleteSpatialAnchorsCompleteML} function is defined as:
*
*
* XrResult xrDeleteSpatialAnchorsCompleteML(
* XrSpatialAnchorsStorageML storage,
* XrFutureEXT future,
* XrSpatialAnchorsDeleteCompletionML* completion);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension must be enabled prior to calling {@link #xrDeleteSpatialAnchorsCompleteML DeleteSpatialAnchorsCompleteML}
* - {@code storage} must be a valid {@code XrSpatialAnchorsStorageML} handle
* - {@code completion} must be a pointer to an {@link XrSpatialAnchorsDeleteCompletionML} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link EXTFuture#XR_ERROR_FUTURE_PENDING_EXT ERROR_FUTURE_PENDING_EXT}
* - {@link EXTFuture#XR_ERROR_FUTURE_INVALID_EXT ERROR_FUTURE_INVALID_EXT}
*
*
*
* See Also
*
* {@link XrSpatialAnchorsDeleteCompletionML}, {@link #xrDeleteSpatialAnchorsAsyncML DeleteSpatialAnchorsAsyncML}
*
* @param storage the {@code XrSpatialAnchorsStorageML} used to start the publish operation.
* @param future the {@code XrFutureEXT} of the future to complete.
* @param completion a pointer to a {@link XrSpatialAnchorsDeleteCompletionML} structure containing the result of the operation.
*/
@NativeType("XrResult")
public static int xrDeleteSpatialAnchorsCompleteML(XrSpatialAnchorsStorageML storage, @NativeType("XrFutureEXT") long future, @NativeType("XrSpatialAnchorsDeleteCompletionML *") XrSpatialAnchorsDeleteCompletionML completion) {
return nxrDeleteSpatialAnchorsCompleteML(storage, future, completion.address());
}
// --- [ xrUpdateSpatialAnchorsExpirationAsyncML ] ---
/** Unsafe version of: {@link #xrUpdateSpatialAnchorsExpirationAsyncML UpdateSpatialAnchorsExpirationAsyncML} */
public static int nxrUpdateSpatialAnchorsExpirationAsyncML(XrSpatialAnchorsStorageML storage, long updateInfo, long future) {
long __functionAddress = storage.getCapabilities().xrUpdateSpatialAnchorsExpirationAsyncML;
if (CHECKS) {
check(__functionAddress);
XrSpatialAnchorsUpdateExpirationInfoML.validate(updateInfo);
}
return callPPPI(storage.address(), updateInfo, future, __functionAddress);
}
/**
* Update anchors expiration time.
*
* C Specification
*
* The {@link #xrUpdateSpatialAnchorsExpirationAsyncML UpdateSpatialAnchorsExpirationAsyncML} function is defined as:
*
*
* XrResult xrUpdateSpatialAnchorsExpirationAsyncML(
* XrSpatialAnchorsStorageML storage,
* const XrSpatialAnchorsUpdateExpirationInfoML* updateInfo,
* XrFutureEXT* future);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension must be enabled prior to calling {@link #xrUpdateSpatialAnchorsExpirationAsyncML UpdateSpatialAnchorsExpirationAsyncML}
* - {@code storage} must be a valid {@code XrSpatialAnchorsStorageML} handle
* - {@code updateInfo} must be a pointer to a valid {@link XrSpatialAnchorsUpdateExpirationInfoML} structure
* - {@code future} must be a pointer to an {@code XrFutureEXT} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
*
*
*
* See Also
*
* {@link XrSpatialAnchorsUpdateExpirationInfoML}, {@link #xrUpdateSpatialAnchorsExpirationCompleteML UpdateSpatialAnchorsExpirationCompleteML}
*
* @param storage the {@code XrSpatialAnchorsStorageML} to use.
* @param updateInfo a pointer to {@link XrSpatialAnchorsUpdateExpirationInfoML} structure used to specify the anchors to update.
* @param future a pointer to the created {@code XrFutureEXT}.
*/
@NativeType("XrResult")
public static int xrUpdateSpatialAnchorsExpirationAsyncML(XrSpatialAnchorsStorageML storage, @NativeType("XrSpatialAnchorsUpdateExpirationInfoML const *") XrSpatialAnchorsUpdateExpirationInfoML updateInfo, @NativeType("XrFutureEXT *") LongBuffer future) {
if (CHECKS) {
check(future, 1);
}
return nxrUpdateSpatialAnchorsExpirationAsyncML(storage, updateInfo.address(), memAddress(future));
}
// --- [ xrUpdateSpatialAnchorsExpirationCompleteML ] ---
/** Unsafe version of: {@link #xrUpdateSpatialAnchorsExpirationCompleteML UpdateSpatialAnchorsExpirationCompleteML} */
public static int nxrUpdateSpatialAnchorsExpirationCompleteML(XrSpatialAnchorsStorageML storage, long future, long completion) {
long __functionAddress = storage.getCapabilities().xrUpdateSpatialAnchorsExpirationCompleteML;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(storage.address(), future, completion, __functionAddress);
}
/**
* Complete the asynchronous update operation.
*
* C Specification
*
* The {@link #xrUpdateSpatialAnchorsExpirationCompleteML UpdateSpatialAnchorsExpirationCompleteML} function is defined as:
*
*
* XrResult xrUpdateSpatialAnchorsExpirationCompleteML(
* XrSpatialAnchorsStorageML storage,
* XrFutureEXT future,
* XrSpatialAnchorsUpdateExpirationCompletionML* completion);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSpatialAnchorsStorage XR_ML_spatial_anchors_storage} extension must be enabled prior to calling {@link #xrUpdateSpatialAnchorsExpirationCompleteML UpdateSpatialAnchorsExpirationCompleteML}
* - {@code storage} must be a valid {@code XrSpatialAnchorsStorageML} handle
* - {@code completion} must be a pointer to an {@link XrSpatialAnchorsUpdateExpirationCompletionML} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link EXTFuture#XR_ERROR_FUTURE_PENDING_EXT ERROR_FUTURE_PENDING_EXT}
* - {@link EXTFuture#XR_ERROR_FUTURE_INVALID_EXT ERROR_FUTURE_INVALID_EXT}
*
*
*
* See Also
*
* {@link XrSpatialAnchorsUpdateExpirationCompletionML}, {@link #xrUpdateSpatialAnchorsExpirationAsyncML UpdateSpatialAnchorsExpirationAsyncML}
*
* @param storage the {@code XrSpatialAnchorsStorageML} used to start the publish operation.
* @param future the {@code XrFutureEXT} of the future to complete.
* @param completion a pointer to a {@link XrSpatialAnchorsUpdateExpirationCompletionML} structure containing the result of the operation.
*/
@NativeType("XrResult")
public static int xrUpdateSpatialAnchorsExpirationCompleteML(XrSpatialAnchorsStorageML storage, @NativeType("XrFutureEXT") long future, @NativeType("XrSpatialAnchorsUpdateExpirationCompletionML *") XrSpatialAnchorsUpdateExpirationCompletionML completion) {
return nxrUpdateSpatialAnchorsExpirationCompleteML(storage, future, completion.address());
}
}