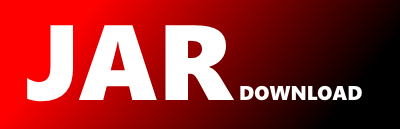
org.lwjgl.openxr.MLSystemNotifications Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
/**
* The XR_ML_system_notifications extension.
*
* This extension provides control over the system notifications. This extension allows system notifications that might obscure the field of view to be disabled.
*
* Note that even when all system notifications have been suppressed, developers can still intercept certain events that allow them to properly react to the underlying reason of system notifications.
*
* Permissions
*
* Android applications must have the com.magicleap.permission.SYSTEM_NOTIFICATION permission listed in their manifest to use this extension. (protection level: normal)
*/
public class MLSystemNotifications {
/** The extension specification version. */
public static final int XR_ML_system_notifications_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_ML_SYSTEM_NOTIFICATIONS_EXTENSION_NAME = "XR_ML_system_notifications";
/** Extends {@code XrStructureType}. */
public static final int XR_TYPE_SYSTEM_NOTIFICATIONS_SET_INFO_ML = 1000473000;
/**
* Extends {@code XrResult}.
*
* Enum values:
*
*
* - {@link #XR_ERROR_SYSTEM_NOTIFICATION_PERMISSION_DENIED_ML ERROR_SYSTEM_NOTIFICATION_PERMISSION_DENIED_ML}
* - {@link #XR_ERROR_SYSTEM_NOTIFICATION_INCOMPATIBLE_SKU_ML ERROR_SYSTEM_NOTIFICATION_INCOMPATIBLE_SKU_ML}
*
*/
public static final int
XR_ERROR_SYSTEM_NOTIFICATION_PERMISSION_DENIED_ML = -1000473000,
XR_ERROR_SYSTEM_NOTIFICATION_INCOMPATIBLE_SKU_ML = -1000473001;
protected MLSystemNotifications() {
throw new UnsupportedOperationException();
}
// --- [ xrSetSystemNotificationsML ] ---
/** Unsafe version of: {@link #xrSetSystemNotificationsML SetSystemNotificationsML} */
public static int nxrSetSystemNotificationsML(XrInstance instance, long info) {
long __functionAddress = instance.getCapabilities().xrSetSystemNotificationsML;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(instance.address(), info, __functionAddress);
}
/**
* Set system notification suppression state.
*
* C Specification
*
* The {@link #xrSetSystemNotificationsML SetSystemNotificationsML} function is defined as:
*
*
* XrResult xrSetSystemNotificationsML(
* XrInstance instance,
* const XrSystemNotificationsSetInfoML* info);
*
* Description
*
* This API will work only on certain SKUs. When called on an incompatible SKU the {@link #XR_ERROR_SYSTEM_NOTIFICATION_INCOMPATIBLE_SKU_ML ERROR_SYSTEM_NOTIFICATION_INCOMPATIBLE_SKU_ML} error must be returned.
*
* If the com.magicleap.permission.SYSTEM_NOTIFICATION permission is not granted, the runtime must return {@link #XR_ERROR_SYSTEM_NOTIFICATION_PERMISSION_DENIED_ML ERROR_SYSTEM_NOTIFICATION_PERMISSION_DENIED_ML}.
*
* Valid Usage (Implicit)
*
*
* - The {@link MLSystemNotifications XR_ML_system_notifications} extension must be enabled prior to calling {@link #xrSetSystemNotificationsML SetSystemNotificationsML}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code info} must be a pointer to a valid {@link XrSystemNotificationsSetInfoML} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link #XR_ERROR_SYSTEM_NOTIFICATION_PERMISSION_DENIED_ML ERROR_SYSTEM_NOTIFICATION_PERMISSION_DENIED_ML}
* - {@link #XR_ERROR_SYSTEM_NOTIFICATION_INCOMPATIBLE_SKU_ML ERROR_SYSTEM_NOTIFICATION_INCOMPATIBLE_SKU_ML}
*
*
*
* See Also
*
* {@link XrSystemNotificationsSetInfoML}
*
* @param instance {@code XrInstance}.
* @param info a pointer to an {@link XrSystemNotificationsSetInfoML} structure.
*/
@NativeType("XrResult")
public static int xrSetSystemNotificationsML(XrInstance instance, @NativeType("XrSystemNotificationsSetInfoML const *") XrSystemNotificationsSetInfoML info) {
return nxrSetSystemNotificationsML(instance, info.address());
}
}