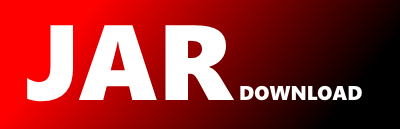
org.lwjgl.openxr.MLUserCalibration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwjgl-openxr Show documentation
Show all versions of lwjgl-openxr Show documentation
A royalty-free, open standard that provides high-performance access to Augmented Reality (AR) and Virtual Reality (VR)—collectively known as XR—platforms and devices.
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
/**
* The XR_ML_user_calibration extension.
*
* This extension can be used to determine how well the device is calibrated for the current user of the device. The extension provides two events for this purpose:
*
*
* - Headset Fit: Provides the quality of the fit of the headset on the user.
* - Eye Calibration: Provides the quality of the user’s eye calibration.
*
*/
public class MLUserCalibration {
/** The extension specification version. */
public static final int XR_ML_user_calibration_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_ML_USER_CALIBRATION_EXTENSION_NAME = "XR_ML_user_calibration";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_EVENT_DATA_HEADSET_FIT_CHANGED_ML TYPE_EVENT_DATA_HEADSET_FIT_CHANGED_ML}
* - {@link #XR_TYPE_EVENT_DATA_EYE_CALIBRATION_CHANGED_ML TYPE_EVENT_DATA_EYE_CALIBRATION_CHANGED_ML}
* - {@link #XR_TYPE_USER_CALIBRATION_ENABLE_EVENTS_INFO_ML TYPE_USER_CALIBRATION_ENABLE_EVENTS_INFO_ML}
*
*/
public static final int
XR_TYPE_EVENT_DATA_HEADSET_FIT_CHANGED_ML = 1000472000,
XR_TYPE_EVENT_DATA_EYE_CALIBRATION_CHANGED_ML = 1000472001,
XR_TYPE_USER_CALIBRATION_ENABLE_EVENTS_INFO_ML = 1000472002;
/**
* XrHeadsetFitStatusML - Headset fit status
*
* Description
*
*
* Enum Description
*
* {@link #XR_HEADSET_FIT_STATUS_UNKNOWN_ML HEADSET_FIT_STATUS_UNKNOWN_ML} Headset fit status not available for unknown reason.
* {@link #XR_HEADSET_FIT_STATUS_NOT_WORN_ML HEADSET_FIT_STATUS_NOT_WORN_ML} Headset not worn.
* {@link #XR_HEADSET_FIT_STATUS_GOOD_FIT_ML HEADSET_FIT_STATUS_GOOD_FIT_ML} Good fit.
* {@link #XR_HEADSET_FIT_STATUS_BAD_FIT_ML HEADSET_FIT_STATUS_BAD_FIT_ML} Bad fit.
*
*
*
* See Also
*
* {@link XrEventDataHeadsetFitChangedML}
*/
public static final int
XR_HEADSET_FIT_STATUS_UNKNOWN_ML = 0,
XR_HEADSET_FIT_STATUS_NOT_WORN_ML = 1,
XR_HEADSET_FIT_STATUS_GOOD_FIT_ML = 2,
XR_HEADSET_FIT_STATUS_BAD_FIT_ML = 3;
/**
* XrEyeCalibrationStatusML - Headset fit status
*
* Description
*
*
* Enum Description
*
* {@link #XR_EYE_CALIBRATION_STATUS_UNKNOWN_ML EYE_CALIBRATION_STATUS_UNKNOWN_ML} Eye calibration status not available for unknown reason.
* {@link #XR_EYE_CALIBRATION_STATUS_NONE_ML EYE_CALIBRATION_STATUS_NONE_ML} User has not performed the eye calibration step. Use system provided app to perform eye calibration.
* {@link #XR_EYE_CALIBRATION_STATUS_COARSE_ML EYE_CALIBRATION_STATUS_COARSE_ML} Eye calibration is of lower accuracy.
* {@link #XR_EYE_CALIBRATION_STATUS_FINE_ML EYE_CALIBRATION_STATUS_FINE_ML} Eye calibration is of higher accuracy.
*
*
*
* See Also
*
* {@link XrEventDataEyeCalibrationChangedML}
*/
public static final int
XR_EYE_CALIBRATION_STATUS_UNKNOWN_ML = 0,
XR_EYE_CALIBRATION_STATUS_NONE_ML = 1,
XR_EYE_CALIBRATION_STATUS_COARSE_ML = 2,
XR_EYE_CALIBRATION_STATUS_FINE_ML = 3;
protected MLUserCalibration() {
throw new UnsupportedOperationException();
}
// --- [ xrEnableUserCalibrationEventsML ] ---
/** Unsafe version of: {@link #xrEnableUserCalibrationEventsML EnableUserCalibrationEventsML} */
public static int nxrEnableUserCalibrationEventsML(XrInstance instance, long enableInfo) {
long __functionAddress = instance.getCapabilities().xrEnableUserCalibrationEventsML;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(instance.address(), enableInfo, __functionAddress);
}
/**
* Enable/disable user calibration events.
*
* C Specification
*
* The {@link #xrEnableUserCalibrationEventsML EnableUserCalibrationEventsML} function is defined as:
*
*
* XrResult xrEnableUserCalibrationEventsML(
* XrInstance instance,
* const XrUserCalibrationEnableEventsInfoML* enableInfo);
*
* Valid Usage (Implicit)
*
*
* - The {@link MLUserCalibration XR_ML_user_calibration} extension must be enabled prior to calling {@link #xrEnableUserCalibrationEventsML EnableUserCalibrationEventsML}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code enableInfo} must be a pointer to a valid {@link XrUserCalibrationEnableEventsInfoML} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
*
*
*
* See Also
*
* {@link XrUserCalibrationEnableEventsInfoML}
*
* @param instance a handle to an {@code XrInstance} previously created with {@link XR10#xrCreateInstance CreateInstance}.
* @param enableInfo the {@link XrUserCalibrationEnableEventsInfoML} that enables or disables user calibration events.
*/
@NativeType("XrResult")
public static int xrEnableUserCalibrationEventsML(XrInstance instance, @NativeType("XrUserCalibrationEnableEventsInfoML const *") XrUserCalibrationEnableEventsInfoML enableInfo) {
return nxrEnableUserCalibrationEventsML(instance, enableInfo.address());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy