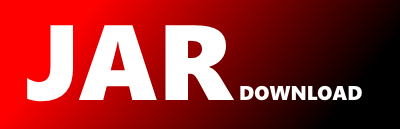
org.lwjgl.openxr.MNDXForceFeedbackCurl Maven / Gradle / Ivy
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
/**
* The XR_MNDX_force_feedback_curl extension.
*
* This extension provides APIs for force feedback devices capable of restricting physical movement in a single direction along a single dimension.
*
* The intended use for this extension is to provide simple force feedback capabilities to restrict finger movement for VR Gloves.
*
* The application must also enable the {@link EXTHandTracking XR_EXT_hand_tracking} extension in order to use this extension.
*/
public class MNDXForceFeedbackCurl {
/** The extension specification version. */
public static final int XR_MNDX_force_feedback_curl_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_MNDX_FORCE_FEEDBACK_CURL_EXTENSION_NAME = "XR_MNDX_force_feedback_curl";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SYSTEM_FORCE_FEEDBACK_CURL_PROPERTIES_MNDX TYPE_SYSTEM_FORCE_FEEDBACK_CURL_PROPERTIES_MNDX}
* - {@link #XR_TYPE_FORCE_FEEDBACK_CURL_APPLY_LOCATIONS_MNDX TYPE_FORCE_FEEDBACK_CURL_APPLY_LOCATIONS_MNDX}
*
*/
public static final int
XR_TYPE_SYSTEM_FORCE_FEEDBACK_CURL_PROPERTIES_MNDX = 1000375000,
XR_TYPE_FORCE_FEEDBACK_CURL_APPLY_LOCATIONS_MNDX = 1000375001;
/**
* XrForceFeedbackCurlLocationMNDX - Describes which location to apply force feedback
*
* Enumerant Descriptions
*
*
* - {@link #XR_FORCE_FEEDBACK_CURL_LOCATION_THUMB_CURL_MNDX FORCE_FEEDBACK_CURL_LOCATION_THUMB_CURL_MNDX} — force feedback for thumb curl
* - {@link #XR_FORCE_FEEDBACK_CURL_LOCATION_INDEX_CURL_MNDX FORCE_FEEDBACK_CURL_LOCATION_INDEX_CURL_MNDX} — force feedback for index finger curl
* - {@link #XR_FORCE_FEEDBACK_CURL_LOCATION_MIDDLE_CURL_MNDX FORCE_FEEDBACK_CURL_LOCATION_MIDDLE_CURL_MNDX} — force feedback for middle finger curl
* - {@link #XR_FORCE_FEEDBACK_CURL_LOCATION_RING_CURL_MNDX FORCE_FEEDBACK_CURL_LOCATION_RING_CURL_MNDX} — force feedback for ring finger curl
* - {@link #XR_FORCE_FEEDBACK_CURL_LOCATION_LITTLE_CURL_MNDX FORCE_FEEDBACK_CURL_LOCATION_LITTLE_CURL_MNDX} — force feedback for little finger curl
*
*
* See Also
*
* {@link XrForceFeedbackCurlApplyLocationMNDX}
*/
public static final int
XR_FORCE_FEEDBACK_CURL_LOCATION_THUMB_CURL_MNDX = 0,
XR_FORCE_FEEDBACK_CURL_LOCATION_INDEX_CURL_MNDX = 1,
XR_FORCE_FEEDBACK_CURL_LOCATION_MIDDLE_CURL_MNDX = 2,
XR_FORCE_FEEDBACK_CURL_LOCATION_RING_CURL_MNDX = 3,
XR_FORCE_FEEDBACK_CURL_LOCATION_LITTLE_CURL_MNDX = 4;
protected MNDXForceFeedbackCurl() {
throw new UnsupportedOperationException();
}
// --- [ xrApplyForceFeedbackCurlMNDX ] ---
/** Unsafe version of: {@link #xrApplyForceFeedbackCurlMNDX ApplyForceFeedbackCurlMNDX} */
public static int nxrApplyForceFeedbackCurlMNDX(XrHandTrackerEXT handTracker, long locations) {
long __functionAddress = handTracker.getCapabilities().xrApplyForceFeedbackCurlMNDX;
if (CHECKS) {
check(__functionAddress);
XrForceFeedbackCurlApplyLocationsMNDX.validate(locations);
}
return callPPI(handTracker.address(), locations, __functionAddress);
}
/**
* Applies force feedback to a set of locations.
*
* C Specification
*
* The {@link #xrApplyForceFeedbackCurlMNDX ApplyForceFeedbackCurlMNDX} function is defined as:
*
*
* XrResult xrApplyForceFeedbackCurlMNDX(
* XrHandTrackerEXT handTracker,
* const XrForceFeedbackCurlApplyLocationsMNDX* locations);
*
* Description
*
* The {@link #xrApplyForceFeedbackCurlMNDX ApplyForceFeedbackCurlMNDX} function applies force feedback to the set locations listed in {@link XrForceFeedbackCurlApplyLocationsMNDX}.
*
* {@link #xrApplyForceFeedbackCurlMNDX ApplyForceFeedbackCurlMNDX} should be called every time an application wishes to update a set of force feedback locations.
*
* Submits a request for force feedback for a set of locations. The runtime should deliver this request to the {@code handTracker} device. If the {@code handTracker} device is not available, the runtime may ignore this request for force feedback.
*
* If the session associated with {@code handTracker} is not focused, the runtime must return {@link XR10#XR_SESSION_NOT_FOCUSED SESSION_NOT_FOCUSED}, and not apply force feedback.
*
* When an application submits force feedback for a set of locations, the runtime must update the set of locations to that specified by the application. A runtime must set any locations not specified by the application when submitting force feedback to 0.
*
* The runtime may discontinue force feedback if the application that set it loses focus. An application should call the function again after regaining focus if force feedback is still desired.
*
* Valid Usage (Implicit)
*
*
* - The {@link MNDXForceFeedbackCurl XR_MNDX_force_feedback_curl} extension must be enabled prior to calling {@link #xrApplyForceFeedbackCurlMNDX ApplyForceFeedbackCurlMNDX}
* - {@code handTracker} must be a valid {@code XrHandTrackerEXT} handle
* - {@code locations} must be a pointer to a valid {@link XrForceFeedbackCurlApplyLocationsMNDX} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
* - {@link XR10#XR_SESSION_NOT_FOCUSED SESSION_NOT_FOCUSED}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
*
*
*
* See Also
*
* {@link XrForceFeedbackCurlApplyLocationsMNDX}
*
* @param handTracker an {@code XrHandTrackerEXT} handle previously created with {@link EXTHandTracking#xrCreateHandTrackerEXT CreateHandTrackerEXT}.
* @param locations an {@link XrForceFeedbackCurlApplyLocationsMNDX} containing a set of locations to apply force feedback to.
*/
@NativeType("XrResult")
public static int xrApplyForceFeedbackCurlMNDX(XrHandTrackerEXT handTracker, @NativeType("XrForceFeedbackCurlApplyLocationsMNDX const *") XrForceFeedbackCurlApplyLocationsMNDX locations) {
return nxrApplyForceFeedbackCurlMNDX(handTracker, locations.address());
}
}