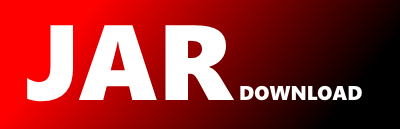
org.lwjgl.openxr.MSFTControllerModel Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_MSFT_controller_model extension.
*
* This extension provides a mechanism to load a GLTF model for controllers. An application can render the controller model using the real time pose input from controller’s grip action pose and animate controller parts representing the user’s interactions, such as pressing a button, or pulling a trigger.
*
* This extension supports any controller interaction profile that supports subpathname:/grip/pose. The returned controller model represents the physical controller held in the user’s hands, and it may be different from the current interaction profile.
*/
public class MSFTControllerModel {
/** The extension specification version. */
public static final int XR_MSFT_controller_model_SPEC_VERSION = 2;
/** The extension name. */
public static final String XR_MSFT_CONTROLLER_MODEL_EXTENSION_NAME = "XR_MSFT_controller_model";
/** XR_MAX_CONTROLLER_MODEL_NODE_NAME_SIZE_MSFT */
public static final int XR_MAX_CONTROLLER_MODEL_NODE_NAME_SIZE_MSFT = 64;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_CONTROLLER_MODEL_KEY_STATE_MSFT TYPE_CONTROLLER_MODEL_KEY_STATE_MSFT}
* - {@link #XR_TYPE_CONTROLLER_MODEL_NODE_PROPERTIES_MSFT TYPE_CONTROLLER_MODEL_NODE_PROPERTIES_MSFT}
* - {@link #XR_TYPE_CONTROLLER_MODEL_PROPERTIES_MSFT TYPE_CONTROLLER_MODEL_PROPERTIES_MSFT}
* - {@link #XR_TYPE_CONTROLLER_MODEL_NODE_STATE_MSFT TYPE_CONTROLLER_MODEL_NODE_STATE_MSFT}
* - {@link #XR_TYPE_CONTROLLER_MODEL_STATE_MSFT TYPE_CONTROLLER_MODEL_STATE_MSFT}
*
*/
public static final int
XR_TYPE_CONTROLLER_MODEL_KEY_STATE_MSFT = 1000055000,
XR_TYPE_CONTROLLER_MODEL_NODE_PROPERTIES_MSFT = 1000055001,
XR_TYPE_CONTROLLER_MODEL_PROPERTIES_MSFT = 1000055002,
XR_TYPE_CONTROLLER_MODEL_NODE_STATE_MSFT = 1000055003,
XR_TYPE_CONTROLLER_MODEL_STATE_MSFT = 1000055004;
/** Extends {@code XrResult}. */
public static final int XR_ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT = -1000055000;
/** API Constants */
public static final long XR_NULL_CONTROLLER_MODEL_KEY_MSFT = 0x0L;
protected MSFTControllerModel() {
throw new UnsupportedOperationException();
}
// --- [ xrGetControllerModelKeyMSFT ] ---
/** Unsafe version of: {@link #xrGetControllerModelKeyMSFT GetControllerModelKeyMSFT} */
public static int nxrGetControllerModelKeyMSFT(XrSession session, long topLevelUserPath, long controllerModelKeyState) {
long __functionAddress = session.getCapabilities().xrGetControllerModelKeyMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(session.address(), topLevelUserPath, controllerModelKeyState, __functionAddress);
}
/**
* Retrieve the model key for the controller.
*
* C Specification
*
* {@link #xrGetControllerModelKeyMSFT GetControllerModelKeyMSFT} retrieves the {@code XrControllerModelKeyMSFT} for a controller. This model key may later be used to retrieve the model data.
*
* The {@link #xrGetControllerModelKeyMSFT GetControllerModelKeyMSFT} function is defined as:
*
*
* XrResult xrGetControllerModelKeyMSFT(
* XrSession session,
* XrPath topLevelUserPath,
* XrControllerModelKeyStateMSFT* controllerModelKeyState);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTControllerModel XR_MSFT_controller_model} extension must be enabled prior to calling {@link #xrGetControllerModelKeyMSFT GetControllerModelKeyMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code controllerModelKeyState} must be a pointer to an {@link XrControllerModelKeyStateMSFT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_PATH_UNSUPPORTED ERROR_PATH_UNSUPPORTED}
* - {@link XR10#XR_ERROR_PATH_INVALID ERROR_PATH_INVALID}
* - {@link #XR_ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT}
*
*
*
* See Also
*
* {@link XrControllerModelKeyStateMSFT}
*
* @param session the specified {@code XrSession}.
* @param topLevelUserPath the top level user path corresponding to the controller render model being queried (e.g. pathname:/user/hand/left or pathname:/user/hand/right).
* @param controllerModelKeyState a pointer to the {@link XrControllerModelKeyStateMSFT} to write the model key state to.
*/
@NativeType("XrResult")
public static int xrGetControllerModelKeyMSFT(XrSession session, @NativeType("XrPath") long topLevelUserPath, @NativeType("XrControllerModelKeyStateMSFT *") XrControllerModelKeyStateMSFT controllerModelKeyState) {
return nxrGetControllerModelKeyMSFT(session, topLevelUserPath, controllerModelKeyState.address());
}
// --- [ xrLoadControllerModelMSFT ] ---
/**
* Unsafe version of: {@link #xrLoadControllerModelMSFT LoadControllerModelMSFT}
*
* @param bufferCapacityInput the capacity of the {@code buffer} array, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrLoadControllerModelMSFT(XrSession session, long modelKey, int bufferCapacityInput, long bufferCountOutput, long buffer) {
long __functionAddress = session.getCapabilities().xrLoadControllerModelMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPJPPI(session.address(), modelKey, bufferCapacityInput, bufferCountOutput, buffer, __functionAddress);
}
/**
* Load controller render model.
*
* C Specification
*
* The {@link #xrLoadControllerModelMSFT LoadControllerModelMSFT} function loads the controller model as a byte buffer containing a binary form of glTF (a.k.a GLB file format) for the controller. The binary glTF data must conform to glTF 2.0 format defined at https://registry.khronos.org/glTF/specs/2.0/glTF-2.0.html.
*
*
* XrResult xrLoadControllerModelMSFT(
* XrSession session,
* XrControllerModelKeyMSFT modelKey,
* uint32_t bufferCapacityInput,
* uint32_t* bufferCountOutput,
* uint8_t* buffer);
*
* Description
*
* The {@link #xrLoadControllerModelMSFT LoadControllerModelMSFT} function may be a slow operation and therefore should be invoked from a non-timing critical thread.
*
* If the input {@code modelKey} is invalid, i.e. it is {@link #XR_NULL_CONTROLLER_MODEL_KEY_MSFT NULL_CONTROLLER_MODEL_KEY_MSFT} or not a key returned from {@link XrControllerModelKeyStateMSFT}, the runtime must return {@link #XR_ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT}.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTControllerModel XR_MSFT_controller_model} extension must be enabled prior to calling {@link #xrLoadControllerModelMSFT LoadControllerModelMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code bufferCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code bufferCapacityInput} is not 0, {@code buffer} must be a pointer to an array of {@code bufferCapacityInput} {@code uint8_t} values
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link #XR_ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT}
*
*
*
* @param session the specified {@code XrSession}.
* @param modelKey the model key corresponding to the controller render model being queried.
* @param bufferCountOutput filled in by the runtime with the count of elements in {@code buffer} array, or returns the required capacity in the case that {@code bufferCapacityInput} is insufficient.
* @param buffer a pointer to an application-allocated array of the model for the device that will be filled with the {@code uint8_t} values by the runtime. It can be {@code NULL} if {@code bufferCapacityInput} is 0.
*/
@NativeType("XrResult")
public static int xrLoadControllerModelMSFT(XrSession session, @NativeType("XrControllerModelKeyMSFT") long modelKey, @NativeType("uint32_t *") IntBuffer bufferCountOutput, @NativeType("uint8_t *") @Nullable ByteBuffer buffer) {
if (CHECKS) {
check(bufferCountOutput, 1);
}
return nxrLoadControllerModelMSFT(session, modelKey, remainingSafe(buffer), memAddress(bufferCountOutput), memAddressSafe(buffer));
}
// --- [ xrGetControllerModelPropertiesMSFT ] ---
/** Unsafe version of: {@link #xrGetControllerModelPropertiesMSFT GetControllerModelPropertiesMSFT} */
public static int nxrGetControllerModelPropertiesMSFT(XrSession session, long modelKey, long properties) {
long __functionAddress = session.getCapabilities().xrGetControllerModelPropertiesMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(session.address(), modelKey, properties, __functionAddress);
}
/**
* Get controller model properties.
*
* C Specification
*
* The {@link #xrGetControllerModelPropertiesMSFT GetControllerModelPropertiesMSFT} function returns the controller model properties for a given {@code modelKey}.
*
*
* XrResult xrGetControllerModelPropertiesMSFT(
* XrSession session,
* XrControllerModelKeyMSFT modelKey,
* XrControllerModelPropertiesMSFT* properties);
*
* Description
*
* The runtime must return the same data in {@link XrControllerModelPropertiesMSFT} for a valid {@code modelKey}. Therefore, the application can cache the returned {@link XrControllerModelPropertiesMSFT} using {@code modelKey} and reuse the data for each frame.
*
* If the input {@code modelKey} is invalid, i.e. it is {@link #XR_NULL_CONTROLLER_MODEL_KEY_MSFT NULL_CONTROLLER_MODEL_KEY_MSFT} or not a key returned from {@link XrControllerModelKeyStateMSFT}, the runtime must return {@link #XR_ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT}.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTControllerModel XR_MSFT_controller_model} extension must be enabled prior to calling {@link #xrGetControllerModelPropertiesMSFT GetControllerModelPropertiesMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code properties} must be a pointer to an {@link XrControllerModelPropertiesMSFT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link #XR_ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT}
*
*
*
* See Also
*
* {@link XrControllerModelPropertiesMSFT}
*
* @param session the specified {@code XrSession}.
* @param modelKey a valid model key obtained from {@link XrControllerModelKeyStateMSFT}
* @param properties an {@link XrControllerModelPropertiesMSFT} returning the properties of the controller model
*/
@NativeType("XrResult")
public static int xrGetControllerModelPropertiesMSFT(XrSession session, @NativeType("XrControllerModelKeyMSFT") long modelKey, @NativeType("XrControllerModelPropertiesMSFT *") XrControllerModelPropertiesMSFT properties) {
return nxrGetControllerModelPropertiesMSFT(session, modelKey, properties.address());
}
// --- [ xrGetControllerModelStateMSFT ] ---
/** Unsafe version of: {@link #xrGetControllerModelStateMSFT GetControllerModelStateMSFT} */
public static int nxrGetControllerModelStateMSFT(XrSession session, long modelKey, long state) {
long __functionAddress = session.getCapabilities().xrGetControllerModelStateMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPJPI(session.address(), modelKey, state, __functionAddress);
}
/**
* Get controller model state.
*
* C Specification
*
* The {@link #xrGetControllerModelStateMSFT GetControllerModelStateMSFT} function returns the current state of the controller model representing user’s interaction to the controller, such as pressing a button or pulling a trigger.
*
*
* XrResult xrGetControllerModelStateMSFT(
* XrSession session,
* XrControllerModelKeyMSFT modelKey,
* XrControllerModelStateMSFT* state);
*
* Description
*
* The runtime may return different state for a model key after each call to {@link XR10#xrSyncActions SyncActions}, which represents the latest state of the user interactions.
*
* If the input {@code modelKey} is invalid, i.e. it is {@link #XR_NULL_CONTROLLER_MODEL_KEY_MSFT NULL_CONTROLLER_MODEL_KEY_MSFT} or not a key returned from {@link XrControllerModelKeyStateMSFT}, the runtime must return {@link #XR_ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT}.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTControllerModel XR_MSFT_controller_model} extension must be enabled prior to calling {@link #xrGetControllerModelStateMSFT GetControllerModelStateMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code state} must be a pointer to an {@link XrControllerModelStateMSFT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link #XR_ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT ERROR_CONTROLLER_MODEL_KEY_INVALID_MSFT}
*
*
*
* See Also
*
* {@link XrControllerModelStateMSFT}
*
* @param session the specified {@code XrSession}.
* @param modelKey the model key corresponding to the controller model being queried.
* @param state a pointer to {@link XrControllerModelNodeStateMSFT} returns the current controller model state.
*/
@NativeType("XrResult")
public static int xrGetControllerModelStateMSFT(XrSession session, @NativeType("XrControllerModelKeyMSFT") long modelKey, @NativeType("XrControllerModelStateMSFT *") XrControllerModelStateMSFT state) {
return nxrGetControllerModelStateMSFT(session, modelKey, state.address());
}
}