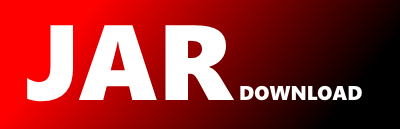
org.lwjgl.openxr.MSFTHandTrackingMesh Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_MSFT_hand_tracking_mesh extension.
*
* This extension enables hand tracking inputs represented as a dynamic hand mesh. It enables applications to render hands in XR experiences and interact with virtual objects using hand meshes.
*
* The application must also enable the {@link EXTHandTracking XR_EXT_hand_tracking} extension in order to use this extension.
*/
public class MSFTHandTrackingMesh {
/** The extension specification version. */
public static final int XR_MSFT_hand_tracking_mesh_SPEC_VERSION = 4;
/** The extension name. */
public static final String XR_MSFT_HAND_TRACKING_MESH_EXTENSION_NAME = "XR_MSFT_hand_tracking_mesh";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SYSTEM_HAND_TRACKING_MESH_PROPERTIES_MSFT TYPE_SYSTEM_HAND_TRACKING_MESH_PROPERTIES_MSFT}
* - {@link #XR_TYPE_HAND_MESH_SPACE_CREATE_INFO_MSFT TYPE_HAND_MESH_SPACE_CREATE_INFO_MSFT}
* - {@link #XR_TYPE_HAND_MESH_UPDATE_INFO_MSFT TYPE_HAND_MESH_UPDATE_INFO_MSFT}
* - {@link #XR_TYPE_HAND_MESH_MSFT TYPE_HAND_MESH_MSFT}
* - {@link #XR_TYPE_HAND_POSE_TYPE_INFO_MSFT TYPE_HAND_POSE_TYPE_INFO_MSFT}
*
*/
public static final int
XR_TYPE_SYSTEM_HAND_TRACKING_MESH_PROPERTIES_MSFT = 1000052000,
XR_TYPE_HAND_MESH_SPACE_CREATE_INFO_MSFT = 1000052001,
XR_TYPE_HAND_MESH_UPDATE_INFO_MSFT = 1000052002,
XR_TYPE_HAND_MESH_MSFT = 1000052003,
XR_TYPE_HAND_POSE_TYPE_INFO_MSFT = 1000052004;
/**
* XrHandPoseTypeMSFT - Describe type of input hand pose
*
* Enumerant Descriptions
*
*
* - {@link #XR_HAND_POSE_TYPE_TRACKED_MSFT HAND_POSE_TYPE_TRACKED_MSFT} represents a hand pose provided by actual tracking of the user’s hand.
* - {@link #XR_HAND_POSE_TYPE_REFERENCE_OPEN_PALM_MSFT HAND_POSE_TYPE_REFERENCE_OPEN_PALM_MSFT} represents a stable reference hand pose in a relaxed open hand shape.
*
*
* The {@link #XR_HAND_POSE_TYPE_TRACKED_MSFT HAND_POSE_TYPE_TRACKED_MSFT} input provides best fidelity to the user’s actual hand motion. When the hand tracking input requires the user to be holding a controller in their hand, the hand tracking input will appear as the user virtually holding the controller. This input can be used to render the hand shape together with the controller in hand.
*
* The {@link #XR_HAND_POSE_TYPE_REFERENCE_OPEN_PALM_MSFT HAND_POSE_TYPE_REFERENCE_OPEN_PALM_MSFT} input does not move with the user’s actual hand. Through this reference hand pose, an application can get a stable hand joint and mesh that has the same mesh topology as the tracked hand mesh using the same {@code XrHandTrackerEXT}, so that the application can apply the data computed from a reference hand pose to the corresponding tracked hand.
*
* Although a reference hand pose does not move with user’s hand motion, the bone length and hand thickness may be updated, for example when tracking result refines, or a different user’s hand is detected. The application should update reference hand joints and meshes when the tracked mesh’s {@code indexBufferKey} is changed or when the {@code isActive} value returned from {@link #xrUpdateHandMeshMSFT UpdateHandMeshMSFT} changes from {@link XR10#XR_FALSE FALSE} to {@link XR10#XR_TRUE TRUE}. It can use the returned {@code indexBufferKey} and {@code vertexUpdateTime} from {@link #xrUpdateHandMeshMSFT UpdateHandMeshMSFT} to avoid unnecessary CPU or GPU work to process the neutral hand inputs.
*
* See Also
*
* {@link XrHandMeshSpaceCreateInfoMSFT}, {@link XrHandMeshUpdateInfoMSFT}, {@link XrHandPoseTypeInfoMSFT}, {@link XrHandTrackerCreateInfoEXT}, {@link EXTHandTracking#xrCreateHandTrackerEXT CreateHandTrackerEXT}
*/
public static final int
XR_HAND_POSE_TYPE_TRACKED_MSFT = 0,
XR_HAND_POSE_TYPE_REFERENCE_OPEN_PALM_MSFT = 1;
protected MSFTHandTrackingMesh() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateHandMeshSpaceMSFT ] ---
/** Unsafe version of: {@link #xrCreateHandMeshSpaceMSFT CreateHandMeshSpaceMSFT} */
public static int nxrCreateHandMeshSpaceMSFT(XrHandTrackerEXT handTracker, long createInfo, long space) {
long __functionAddress = handTracker.getCapabilities().xrCreateHandMeshSpaceMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(handTracker.address(), createInfo, space, __functionAddress);
}
/**
* Create a space for hand mesh tracking.
*
* C Specification
*
* The application creates a hand mesh space using function {@link #xrCreateHandMeshSpaceMSFT CreateHandMeshSpaceMSFT}. The position and normal of hand mesh vertices will be represented in this space.
*
*
* XrResult xrCreateHandMeshSpaceMSFT(
* XrHandTrackerEXT handTracker,
* const XrHandMeshSpaceCreateInfoMSFT* createInfo,
* XrSpace* space);
*
* Description
*
* A hand mesh space location is specified by runtime preference to effectively represent hand mesh vertices without unnecessary transformations. For example, an optical hand tracking system can define the hand mesh space origin at the depth camera’s optical center.
*
* An application should create separate hand mesh space handles for each hand to retrieve the corresponding hand mesh data. The runtime may use the lifetime of this hand mesh space handle to manage the underlying device resources. Therefore, the application should destroy the hand mesh handle after it is finished using the hand mesh.
*
* The hand mesh space can be related to other spaces in the session, such as view reference space, or grip action space from the pathname:/interaction_profiles/khr/simple_controller interaction profile. The hand mesh space may be not locatable when the hand is outside of the tracking range, or if focus is removed from the application. In these cases, the runtime must not set the {@link XR10#XR_SPACE_LOCATION_POSITION_VALID_BIT SPACE_LOCATION_POSITION_VALID_BIT} and {@link XR10#XR_SPACE_LOCATION_ORIENTATION_VALID_BIT SPACE_LOCATION_ORIENTATION_VALID_BIT} bits on calls to {@link XR10#xrLocateSpace LocateSpace} with the hand mesh space, and the application should avoid using the returned poses or query for hand mesh data.
*
* If the underlying {@code XrHandTrackerEXT} is destroyed, the runtime must continue to support {@link XR10#xrLocateSpace LocateSpace} using the hand mesh space, and it must return space location with {@link XR10#XR_SPACE_LOCATION_POSITION_VALID_BIT SPACE_LOCATION_POSITION_VALID_BIT} and {@link XR10#XR_SPACE_LOCATION_ORIENTATION_VALID_BIT SPACE_LOCATION_ORIENTATION_VALID_BIT} unset.
*
* The application may create a mesh space for the reference hand by setting {@link XrHandPoseTypeInfoMSFT}{@code ::handPoseType} to {@link #XR_HAND_POSE_TYPE_REFERENCE_OPEN_PALM_MSFT HAND_POSE_TYPE_REFERENCE_OPEN_PALM_MSFT}. Hand mesh spaces for the reference hand must only be locatable in reference to mesh spaces or joint spaces of the reference hand.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTHandTrackingMesh XR_MSFT_hand_tracking_mesh} extension must be enabled prior to calling {@link #xrCreateHandMeshSpaceMSFT CreateHandMeshSpaceMSFT}
* - {@code handTracker} must be a valid {@code XrHandTrackerEXT} handle
* - {@code createInfo} must be a pointer to a valid {@link XrHandMeshSpaceCreateInfoMSFT} structure
* - {@code space} must be a pointer to an {@code XrSpace} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_POSE_INVALID ERROR_POSE_INVALID}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrHandMeshSpaceCreateInfoMSFT}
*
* @param handTracker an {@code XrHandTrackerEXT} handle previously created with the {@link EXTHandTracking#xrCreateHandTrackerEXT CreateHandTrackerEXT} function.
* @param createInfo the {@link XrHandMeshSpaceCreateInfoMSFT} used to specify the hand mesh space.
* @param space the returned {@code XrSpace} handle of the new hand mesh space.
*/
@NativeType("XrResult")
public static int xrCreateHandMeshSpaceMSFT(XrHandTrackerEXT handTracker, @NativeType("XrHandMeshSpaceCreateInfoMSFT const *") XrHandMeshSpaceCreateInfoMSFT createInfo, @NativeType("XrSpace *") PointerBuffer space) {
if (CHECKS) {
check(space, 1);
}
return nxrCreateHandMeshSpaceMSFT(handTracker, createInfo.address(), memAddress(space));
}
// --- [ xrUpdateHandMeshMSFT ] ---
/** Unsafe version of: {@link #xrUpdateHandMeshMSFT UpdateHandMeshMSFT} */
public static int nxrUpdateHandMeshMSFT(XrHandTrackerEXT handTracker, long updateInfo, long handMesh) {
long __functionAddress = handTracker.getCapabilities().xrUpdateHandMeshMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(handTracker.address(), updateInfo, handMesh, __functionAddress);
}
/**
* Update hand mesh buffers.
*
* C Specification
*
* The application can use the {@link #xrUpdateHandMeshMSFT UpdateHandMeshMSFT} function to retrieve the hand mesh at a given timestamp. The hand mesh’s vertices position and normal are represented in the hand mesh space created by {@link #xrCreateHandMeshSpaceMSFT CreateHandMeshSpaceMSFT} with a same {@code XrHandTrackerEXT}.
*
*
* XrResult xrUpdateHandMeshMSFT(
* XrHandTrackerEXT handTracker,
* const XrHandMeshUpdateInfoMSFT* updateInfo,
* XrHandMeshMSFT* handMesh);
*
* Description
*
* The application should preallocate the index buffer and vertex buffer in {@link XrHandMeshMSFT} using the {@link XrSystemHandTrackingMeshPropertiesMSFT}{@code ::maxHandMeshIndexCount} and {@link XrSystemHandTrackingMeshPropertiesMSFT}{@code ::maxHandMeshVertexCount} from the {@link XrSystemHandTrackingMeshPropertiesMSFT} returned from the {@link XR10#xrGetSystemProperties GetSystemProperties} function.
*
* The application should preallocate the {@link XrHandMeshMSFT} structure and reuse it for each frame so as to reduce the copies of data when underlying tracking data is not changed. The application should use {@link XrHandMeshMSFT}{@code ::indexBufferChanged} and {@link XrHandMeshMSFT}{@code ::vertexBufferChanged} in {@link XrHandMeshMSFT} to detect changes and avoid unnecessary data processing when there is no changes.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTHandTrackingMesh XR_MSFT_hand_tracking_mesh} extension must be enabled prior to calling {@link #xrUpdateHandMeshMSFT UpdateHandMeshMSFT}
* - {@code handTracker} must be a valid {@code XrHandTrackerEXT} handle
* - {@code updateInfo} must be a pointer to a valid {@link XrHandMeshUpdateInfoMSFT} structure
* - {@code handMesh} must be a pointer to an {@link XrHandMeshMSFT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link XrHandMeshMSFT}, {@link XrHandMeshUpdateInfoMSFT}, {@link #xrCreateHandMeshSpaceMSFT CreateHandMeshSpaceMSFT}
*
* @param handTracker an {@code XrHandTrackerEXT} handle previously created with {@link EXTHandTracking#xrCreateHandTrackerEXT CreateHandTrackerEXT}.
* @param updateInfo an {@link XrHandMeshUpdateInfoMSFT} which contains information to query the hand mesh.
* @param handMesh an {@link XrHandMeshMSFT} structure to receive the updates of hand mesh data.
*/
@NativeType("XrResult")
public static int xrUpdateHandMeshMSFT(XrHandTrackerEXT handTracker, @NativeType("XrHandMeshUpdateInfoMSFT const *") XrHandMeshUpdateInfoMSFT updateInfo, @NativeType("XrHandMeshMSFT *") XrHandMeshMSFT handMesh) {
return nxrUpdateHandMeshMSFT(handTracker, updateInfo.address(), handMesh.address());
}
}