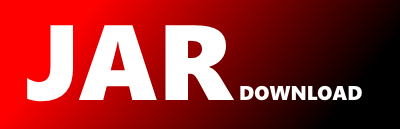
org.lwjgl.openxr.MSFTPerceptionAnchorInterop Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_MSFT_perception_anchor_interop extension.
*
* This extension supports conversion between {@code XrSpatialAnchorMSFT} and Windows.Perception.Spatial.SpatialAnchor. An application can use this extension to persist spatial anchors on the Windows device through SpatialAnchorStore or transfer spatial anchors between devices through SpatialAnchorTransferManager.
*/
public class MSFTPerceptionAnchorInterop {
/** The extension specification version. */
public static final int XR_MSFT_perception_anchor_interop_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_MSFT_PERCEPTION_ANCHOR_INTEROP_EXTENSION_NAME = "XR_MSFT_perception_anchor_interop";
protected MSFTPerceptionAnchorInterop() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateSpatialAnchorFromPerceptionAnchorMSFT ] ---
/** Unsafe version of: {@link #xrCreateSpatialAnchorFromPerceptionAnchorMSFT CreateSpatialAnchorFromPerceptionAnchorMSFT} */
public static int nxrCreateSpatialAnchorFromPerceptionAnchorMSFT(XrSession session, long perceptionAnchor, long anchor) {
long __functionAddress = session.getCapabilities().xrCreateSpatialAnchorFromPerceptionAnchorMSFT;
if (CHECKS) {
check(__functionAddress);
check(perceptionAnchor);
}
return callPPPI(session.address(), perceptionAnchor, anchor, __functionAddress);
}
/**
* Create an {@code XrSpatialAnchorMSFT} from a Windows SpatialAnchor pointer.
*
* C Specification
*
* The {@link #xrCreateSpatialAnchorFromPerceptionAnchorMSFT CreateSpatialAnchorFromPerceptionAnchorMSFT} function creates a {@code XrSpatialAnchorMSFT} handle from an {@code IUnknown} pointer to Windows.Perception.Spatial.SpatialAnchor.
*
*
* XrResult xrCreateSpatialAnchorFromPerceptionAnchorMSFT(
* XrSession session,
* IUnknown* perceptionAnchor,
* XrSpatialAnchorMSFT* anchor);
*
* Description
*
* The input {@code perceptionAnchor} must support successful {@code QueryInterface} to Windows.Perception.Spatial.SpatialAnchor , otherwise the runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}.
*
* If the function successfully returned, the output {@code anchor} must be a valid handle. This also increments the refcount of the {@code perceptionAnchor} object.
*
* When application is done with the {@code anchor} handle, it can be destroyed using {@link MSFTSpatialAnchor#xrDestroySpatialAnchorMSFT DestroySpatialAnchorMSFT} function. This also decrements the refcount of underlying windows perception anchor object.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTPerceptionAnchorInterop XR_MSFT_perception_anchor_interop} extension must be enabled prior to calling {@link #xrCreateSpatialAnchorFromPerceptionAnchorMSFT CreateSpatialAnchorFromPerceptionAnchorMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code perceptionAnchor} must be a pointer to an {@code IUnknown} value
* - {@code anchor} must be a pointer to an {@code XrSpatialAnchorMSFT} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* @param session the specified {@code XrSession}.
* @param perceptionAnchor an IUnknown pointer to a Windows.Perception.Spatial.SpatialAnchor object.
* @param anchor a pointer to {@code XrSpatialAnchorMSFT} to receive the returned anchor handle.
*/
@NativeType("XrResult")
public static int xrCreateSpatialAnchorFromPerceptionAnchorMSFT(XrSession session, @NativeType("IUnknown *") long perceptionAnchor, @NativeType("XrSpatialAnchorMSFT *") PointerBuffer anchor) {
if (CHECKS) {
check(anchor, 1);
}
return nxrCreateSpatialAnchorFromPerceptionAnchorMSFT(session, perceptionAnchor, memAddress(anchor));
}
// --- [ xrTryGetPerceptionAnchorFromSpatialAnchorMSFT ] ---
/** Unsafe version of: {@link #xrTryGetPerceptionAnchorFromSpatialAnchorMSFT TryGetPerceptionAnchorFromSpatialAnchorMSFT} */
public static int nxrTryGetPerceptionAnchorFromSpatialAnchorMSFT(XrSession session, XrSpatialAnchorMSFT anchor, long perceptionAnchor) {
long __functionAddress = session.getCapabilities().xrTryGetPerceptionAnchorFromSpatialAnchorMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), anchor.address(), perceptionAnchor, __functionAddress);
}
/**
* Convert an {@code XrSpatialAnchorMSFT} to a Windows SpatialAnchor.
*
* C Specification
*
* The {@link #xrTryGetPerceptionAnchorFromSpatialAnchorMSFT TryGetPerceptionAnchorFromSpatialAnchorMSFT} function converts a {@code XrSpatialAnchorMSFT} handle into an {@code IUnknown} pointer to Windows.Perception.Spatial.SpatialAnchor.
*
*
* XrResult xrTryGetPerceptionAnchorFromSpatialAnchorMSFT(
* XrSession session,
* XrSpatialAnchorMSFT anchor,
* IUnknown** perceptionAnchor);
*
* Description
*
* If the runtime can convert the {@code anchor} to a Windows.Perception.Spatial.SpatialAnchor object, this function must return {@link XR10#XR_SUCCESS SUCCESS}, and the output {@code IUnknown} in the pointer of {@code perceptionAnchor} must be not {@code NULL}. This also increments the refcount of the object. The application can then use {@code QueryInterface} to get the pointer for Windows.Perception.Spatial.SpatialAnchor object. The application should release the COM pointer after done with the object, or attach it to a smart COM pointer such as {@code winrt::com_ptr}.
*
* If the runtime cannot convert the {@code anchor} to a Windows.Perception.Spatial.SpatialAnchor object, the function must return {@link XR10#XR_SUCCESS SUCCESS}, and the output {@code IUnknown} in the pointer of {@code perceptionAnchor} must be {@code NULL}.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTPerceptionAnchorInterop XR_MSFT_perception_anchor_interop} extension must be enabled prior to calling {@link #xrTryGetPerceptionAnchorFromSpatialAnchorMSFT TryGetPerceptionAnchorFromSpatialAnchorMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code anchor} must be a valid {@code XrSpatialAnchorMSFT} handle
* - {@code perceptionAnchor} must be a pointer to a pointer to an {@code IUnknown} value
* - {@code anchor} must have been created, allocated, or retrieved from {@code session}
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
*
*
*
* @param session the specified {@code XrSession}.
* @param anchor a valid {@code XrSpatialAnchorMSFT} handle.
* @param perceptionAnchor a valid pointer to IUnknown pointer to receive the output Windows.Perception.Spatial.SpatialAnchor object.
*/
@NativeType("XrResult")
public static int xrTryGetPerceptionAnchorFromSpatialAnchorMSFT(XrSession session, XrSpatialAnchorMSFT anchor, @NativeType("IUnknown **") PointerBuffer perceptionAnchor) {
if (CHECKS) {
check(perceptionAnchor, 1);
}
return nxrTryGetPerceptionAnchorFromSpatialAnchorMSFT(session, anchor, memAddress(perceptionAnchor));
}
}