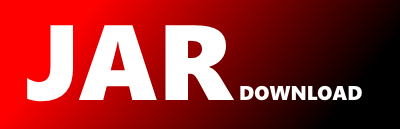
org.lwjgl.openxr.MSFTSceneMarker Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_MSFT_scene_marker extension.
*
* This extension enables the application to observe the tracked markers, such as the QR Code markers in ISO/IEC 18004:2015. This extension also enables future extensions to easily add new types of marker tracking.
*
* The application must enable both {@link MSFTSceneMarker XR_MSFT_scene_marker} and {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} in order to use this extension.
*
* Note
*
* A typical use of this extension is:
*
*
* - Verify if marker detection is supported by calling {@link MSFTSceneUnderstanding#xrEnumerateSceneComputeFeaturesMSFT EnumerateSceneComputeFeaturesMSFT} and validate that the returned supported features include {@link #XR_SCENE_COMPUTE_FEATURE_MARKER_MSFT SCENE_COMPUTE_FEATURE_MARKER_MSFT}.
* - If supported, create an {@code XrSceneObserverMSFT} handle.
* - Pass in {@link #XR_SCENE_COMPUTE_FEATURE_MARKER_MSFT SCENE_COMPUTE_FEATURE_MARKER_MSFT} as requested feature when starting the scene compute by calling {@link MSFTSceneUnderstanding#xrComputeNewSceneMSFT ComputeNewSceneMSFT} function.
* - Inspect the completion of computation by polling {@link MSFTSceneUnderstanding#xrGetSceneComputeStateMSFT GetSceneComputeStateMSFT}.
* - Once compute is successfully completed, create an {@code XrSceneMSFT} handle to the result by calling {@link MSFTSceneUnderstanding#xrCreateSceneMSFT CreateSceneMSFT}.
* - Get the list of detected markers using {@link MSFTSceneUnderstanding#xrGetSceneComponentsMSFT GetSceneComponentsMSFT}:
*
*
* - optionally filter the type of the returned markers using {@link XrSceneMarkerTypeFilterMSFT}.
* - optionally retrieve additional marker properties by chaining {@link XrSceneMarkersMSFT} and/or {@link XrSceneMarkerQRCodesMSFT} to the next pointer of {@link XrSceneComponentsMSFT}.
*
*
* - Get the data encoded in a marker using {@link #xrGetSceneMarkerDecodedStringMSFT GetSceneMarkerDecodedStringMSFT} or {@link #xrGetSceneMarkerRawDataMSFT GetSceneMarkerRawDataMSFT}.
* - Locate markers using {@link MSFTSceneUnderstanding#xrLocateSceneComponentsMSFT LocateSceneComponentsMSFT}.
*
*
*/
public class MSFTSceneMarker {
/** The extension specification version. */
public static final int XR_MSFT_scene_marker_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_MSFT_SCENE_MARKER_EXTENSION_NAME = "XR_MSFT_scene_marker";
/** Extends {@code XrSceneComputeFeatureMSFT}. */
public static final int XR_SCENE_COMPUTE_FEATURE_MARKER_MSFT = 1000147000;
/** Extends {@code XrSceneComponentTypeMSFT}. */
public static final int XR_SCENE_COMPONENT_TYPE_MARKER_MSFT = 1000147000;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SCENE_MARKERS_MSFT TYPE_SCENE_MARKERS_MSFT}
* - {@link #XR_TYPE_SCENE_MARKER_TYPE_FILTER_MSFT TYPE_SCENE_MARKER_TYPE_FILTER_MSFT}
* - {@link #XR_TYPE_SCENE_MARKER_QR_CODES_MSFT TYPE_SCENE_MARKER_QR_CODES_MSFT}
*
*/
public static final int
XR_TYPE_SCENE_MARKERS_MSFT = 1000147000,
XR_TYPE_SCENE_MARKER_TYPE_FILTER_MSFT = 1000147001,
XR_TYPE_SCENE_MARKER_QR_CODES_MSFT = 1000147002;
/** Extends {@code XrResult}. */
public static final int XR_SCENE_MARKER_DATA_NOT_STRING_MSFT = 1000147000;
/**
* XrSceneMarkerTypeMSFT - Marker type
*
* Enumerant Descriptions
*
*
* - {@link #XR_SCENE_MARKER_TYPE_QR_CODE_MSFT SCENE_MARKER_TYPE_QR_CODE_MSFT} represents a marker that follows the ISO standard for QR code in ISO/IEC 18004:2015.
*
*
* See Also
*
* {@link XrSceneMarkerMSFT}, {@link XrSceneMarkerTypeFilterMSFT}
*/
public static final int XR_SCENE_MARKER_TYPE_QR_CODE_MSFT = 1;
/**
* XrSceneMarkerQRCodeSymbolTypeMSFT - QR Code Symbol type
*
* Description
*
* The {@code XrSceneMarkerQRCodeSymbolTypeMSFT} identifies the symbol type of the QR Code.
*
* Enumerant Descriptions
*
*
* - {@link #XR_SCENE_MARKER_QR_CODE_SYMBOL_TYPE_QR_CODE_MSFT SCENE_MARKER_QR_CODE_SYMBOL_TYPE_QR_CODE_MSFT} if the marker is a QR Code.
* - {@link #XR_SCENE_MARKER_QR_CODE_SYMBOL_TYPE_MICRO_QR_CODE_MSFT SCENE_MARKER_QR_CODE_SYMBOL_TYPE_MICRO_QR_CODE_MSFT} if the marker is a Micro QR Code.
*
*
* See Also
*
* {@link XrSceneMarkerQRCodeMSFT}
*/
public static final int
XR_SCENE_MARKER_QR_CODE_SYMBOL_TYPE_QR_CODE_MSFT = 1,
XR_SCENE_MARKER_QR_CODE_SYMBOL_TYPE_MICRO_QR_CODE_MSFT = 2;
protected MSFTSceneMarker() {
throw new UnsupportedOperationException();
}
// --- [ xrGetSceneMarkerRawDataMSFT ] ---
/**
* Unsafe version of: {@link #xrGetSceneMarkerRawDataMSFT GetSceneMarkerRawDataMSFT}
*
* @param bufferCapacityInput the capacity of the buffer, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrGetSceneMarkerRawDataMSFT(XrSceneMSFT scene, long markerId, int bufferCapacityInput, long bufferCountOutput, long buffer) {
long __functionAddress = scene.getCapabilities().xrGetSceneMarkerRawDataMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPPI(scene.address(), markerId, bufferCapacityInput, bufferCountOutput, buffer, __functionAddress);
}
/**
* Get the data stored in the scene marker.
*
* C Specification
*
* The {@link #xrGetSceneMarkerRawDataMSFT GetSceneMarkerRawDataMSFT} function is defined as:
*
*
* XrResult xrGetSceneMarkerRawDataMSFT(
* XrSceneMSFT scene,
* const XrUuidMSFT* markerId,
* uint32_t bufferCapacityInput,
* uint32_t* bufferCountOutput,
* uint8_t* buffer);
*
* Description
*
* The {@link #xrGetSceneMarkerRawDataMSFT GetSceneMarkerRawDataMSFT} function retrieves the data stored in the scene marker.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneMarker XR_MSFT_scene_marker} extension must be enabled prior to calling {@link #xrGetSceneMarkerRawDataMSFT GetSceneMarkerRawDataMSFT}
* - {@code scene} must be a valid {@code XrSceneMSFT} handle
* - {@code markerId} must be a pointer to a valid {@link XrUuidMSFT} structure
* - {@code bufferCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code bufferCapacityInput} is not 0, {@code buffer} must be a pointer to an array of {@code bufferCapacityInput} {@code uint8_t} values
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link MSFTSceneUnderstanding#XR_ERROR_SCENE_COMPONENT_TYPE_MISMATCH_MSFT ERROR_SCENE_COMPONENT_TYPE_MISMATCH_MSFT}
* - {@link MSFTSceneUnderstanding#XR_ERROR_SCENE_COMPONENT_ID_INVALID_MSFT ERROR_SCENE_COMPONENT_ID_INVALID_MSFT}
*
*
*
* See Also
*
* {@link XrUuidMSFT}
*
* @param scene an {@code XrSceneMSFT} previously created by {@link MSFTSceneUnderstanding#xrCreateSceneMSFT CreateSceneMSFT}.
* @param markerId an {@link XrUuidMSFT} identifying the marker, and it is returned previous from {@link XrSceneComponentMSFT} when calling {@link MSFTSceneUnderstanding#xrGetSceneComponentsMSFT GetSceneComponentsMSFT}.
* @param bufferCountOutput a pointer to the count of bytes written, or a pointer to the required capacity in the case that bufferCapacityInput is insufficient.
* @param buffer a pointer to an application-allocated buffer that will be filled with the data stored in the QR Code. It can be NULL if bufferCapacityInput is 0.
*/
@NativeType("XrResult")
public static int xrGetSceneMarkerRawDataMSFT(XrSceneMSFT scene, @NativeType("XrUuidMSFT const *") XrUuidMSFT markerId, @NativeType("uint32_t *") IntBuffer bufferCountOutput, @NativeType("uint8_t *") @Nullable ByteBuffer buffer) {
if (CHECKS) {
check(bufferCountOutput, 1);
}
return nxrGetSceneMarkerRawDataMSFT(scene, markerId.address(), remainingSafe(buffer), memAddress(bufferCountOutput), memAddressSafe(buffer));
}
// --- [ xrGetSceneMarkerDecodedStringMSFT ] ---
/**
* Unsafe version of: {@link #xrGetSceneMarkerDecodedStringMSFT GetSceneMarkerDecodedStringMSFT}
*
* @param bufferCapacityInput the capacity of the string buffer, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrGetSceneMarkerDecodedStringMSFT(XrSceneMSFT scene, long markerId, int bufferCapacityInput, long bufferCountOutput, long buffer) {
long __functionAddress = scene.getCapabilities().xrGetSceneMarkerDecodedStringMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPPI(scene.address(), markerId, bufferCapacityInput, bufferCountOutput, buffer, __functionAddress);
}
/**
* Get the string encoded in the scene marker.
*
* C Specification
*
* The {@link #xrGetSceneMarkerDecodedStringMSFT GetSceneMarkerDecodedStringMSFT} function is defined as:
*
*
* XrResult xrGetSceneMarkerDecodedStringMSFT(
* XrSceneMSFT scene,
* const XrUuidMSFT* markerId,
* uint32_t bufferCapacityInput,
* uint32_t* bufferCountOutput,
* char* buffer);
*
* Description
*
* The {@link #xrGetSceneMarkerDecodedStringMSFT GetSceneMarkerDecodedStringMSFT} function retrieves the string stored in the scene marker as an UTF-8 string, including the terminating '\0'. This function follows the two-call idiom for filling the {@code buffer} array.
*
* If the stored data in the marker is not an encoded string, the runtime must return the success code {@link #XR_SCENE_MARKER_DATA_NOT_STRING_MSFT SCENE_MARKER_DATA_NOT_STRING_MSFT}, set {@code bufferCountOutput} to 1, and make {@code buffer} an empty string.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneMarker XR_MSFT_scene_marker} extension must be enabled prior to calling {@link #xrGetSceneMarkerDecodedStringMSFT GetSceneMarkerDecodedStringMSFT}
* - {@code scene} must be a valid {@code XrSceneMSFT} handle
* - {@code markerId} must be a pointer to a valid {@link XrUuidMSFT} structure
* - {@code bufferCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code bufferCapacityInput} is not 0, {@code buffer} must be a pointer to an array of {@code bufferCapacityInput} char values
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
* - {@link #XR_SCENE_MARKER_DATA_NOT_STRING_MSFT SCENE_MARKER_DATA_NOT_STRING_MSFT}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link MSFTSceneUnderstanding#XR_ERROR_SCENE_COMPONENT_TYPE_MISMATCH_MSFT ERROR_SCENE_COMPONENT_TYPE_MISMATCH_MSFT}
* - {@link MSFTSceneUnderstanding#XR_ERROR_SCENE_COMPONENT_ID_INVALID_MSFT ERROR_SCENE_COMPONENT_ID_INVALID_MSFT}
*
*
*
* See Also
*
* {@link XrUuidMSFT}
*
* @param scene an {@code XrSceneMSFT} previously created by {@link MSFTSceneUnderstanding#xrCreateSceneMSFT CreateSceneMSFT}.
* @param markerId an {@link XrUuidMSFT} identifying the marker, returned previously from {@link XrSceneComponentMSFT}{@code ::id} when calling {@link MSFTSceneUnderstanding#xrGetSceneComponentsMSFT GetSceneComponentsMSFT}.
* @param bufferCountOutput a pointer to the count of characters written (including the terminating '\0'), or a pointer to the required capacity in the case that bufferCapacityInput is insufficient.
* @param buffer a pointer to an application-allocated buffer that will be filled with the string stored in the QR Code. It can be NULL if bufferCapacityInput is 0.
*/
@NativeType("XrResult")
public static int xrGetSceneMarkerDecodedStringMSFT(XrSceneMSFT scene, @NativeType("XrUuidMSFT const *") XrUuidMSFT markerId, @NativeType("uint32_t *") IntBuffer bufferCountOutput, @NativeType("char *") @Nullable ByteBuffer buffer) {
if (CHECKS) {
check(bufferCountOutput, 1);
}
return nxrGetSceneMarkerDecodedStringMSFT(scene, markerId.address(), remainingSafe(buffer), memAddress(bufferCountOutput), memAddressSafe(buffer));
}
}