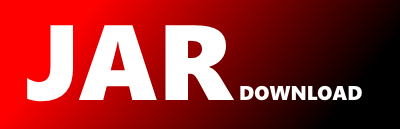
org.lwjgl.openxr.MSFTSceneUnderstanding Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_MSFT_scene_understanding extension.
*
* Scene understanding provides applications with a structured, high-level representation of the planes, meshes, and objects in the user’s environment, enabling the development of spatially-aware applications.
*
* The application requests computation of a scene, receiving the list of scene components observed in the environment around the user. These scene components contain information such as:
*
*
* - The type of the discovered objects (wall, floor, ceiling, or other surface type).
* - The planes and their bounds that represent the object.
* - The visual and collider triangle meshes that represent the object.
*
*
* The application can use this information to reason about the structure and location of the environment, to place holograms on surfaces, or render clues for grounding objects.
*
* An application typically uses this extension in the following steps:
*
*
* - Create an {@code XrSceneObserverMSFT} handle to manage the system resource of the scene understanding compute.
* - Start the scene compute by calling {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} with {@link XrSceneBoundsMSFT} to specify the scan range and a list of {@code XrSceneComputeFeatureMSFT} features.
* - Inspect the completion of computation by polling {@link #xrGetSceneComputeStateMSFT GetSceneComputeStateMSFT}.
* - Once compute is completed, create an {@code XrSceneMSFT} handle to the result by calling {@link #xrCreateSceneMSFT CreateSceneMSFT}.
* - Get properties of scene components using {@link #xrGetSceneComponentsMSFT GetSceneComponentsMSFT}.
* - Locate scene components using {@link #xrLocateSceneComponentsMSFT LocateSceneComponentsMSFT}.
*
*/
public class MSFTSceneUnderstanding {
/** The extension specification version. */
public static final int XR_MSFT_scene_understanding_SPEC_VERSION = 2;
/** The extension name. */
public static final String XR_MSFT_SCENE_UNDERSTANDING_EXTENSION_NAME = "XR_MSFT_scene_understanding";
/**
* Extends {@code XrObjectType}.
*
* Enum values:
*
*
* - {@link #XR_OBJECT_TYPE_SCENE_OBSERVER_MSFT OBJECT_TYPE_SCENE_OBSERVER_MSFT}
* - {@link #XR_OBJECT_TYPE_SCENE_MSFT OBJECT_TYPE_SCENE_MSFT}
*
*/
public static final int
XR_OBJECT_TYPE_SCENE_OBSERVER_MSFT = 1000097000,
XR_OBJECT_TYPE_SCENE_MSFT = 1000097001;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SCENE_OBSERVER_CREATE_INFO_MSFT TYPE_SCENE_OBSERVER_CREATE_INFO_MSFT}
* - {@link #XR_TYPE_SCENE_CREATE_INFO_MSFT TYPE_SCENE_CREATE_INFO_MSFT}
* - {@link #XR_TYPE_NEW_SCENE_COMPUTE_INFO_MSFT TYPE_NEW_SCENE_COMPUTE_INFO_MSFT}
* - {@link #XR_TYPE_VISUAL_MESH_COMPUTE_LOD_INFO_MSFT TYPE_VISUAL_MESH_COMPUTE_LOD_INFO_MSFT}
* - {@link #XR_TYPE_SCENE_COMPONENTS_MSFT TYPE_SCENE_COMPONENTS_MSFT}
* - {@link #XR_TYPE_SCENE_COMPONENTS_GET_INFO_MSFT TYPE_SCENE_COMPONENTS_GET_INFO_MSFT}
* - {@link #XR_TYPE_SCENE_COMPONENT_LOCATIONS_MSFT TYPE_SCENE_COMPONENT_LOCATIONS_MSFT}
* - {@link #XR_TYPE_SCENE_COMPONENTS_LOCATE_INFO_MSFT TYPE_SCENE_COMPONENTS_LOCATE_INFO_MSFT}
* - {@link #XR_TYPE_SCENE_OBJECTS_MSFT TYPE_SCENE_OBJECTS_MSFT}
* - {@link #XR_TYPE_SCENE_COMPONENT_PARENT_FILTER_INFO_MSFT TYPE_SCENE_COMPONENT_PARENT_FILTER_INFO_MSFT}
* - {@link #XR_TYPE_SCENE_OBJECT_TYPES_FILTER_INFO_MSFT TYPE_SCENE_OBJECT_TYPES_FILTER_INFO_MSFT}
* - {@link #XR_TYPE_SCENE_PLANES_MSFT TYPE_SCENE_PLANES_MSFT}
* - {@link #XR_TYPE_SCENE_PLANE_ALIGNMENT_FILTER_INFO_MSFT TYPE_SCENE_PLANE_ALIGNMENT_FILTER_INFO_MSFT}
* - {@link #XR_TYPE_SCENE_MESHES_MSFT TYPE_SCENE_MESHES_MSFT}
* - {@link #XR_TYPE_SCENE_MESH_BUFFERS_GET_INFO_MSFT TYPE_SCENE_MESH_BUFFERS_GET_INFO_MSFT}
* - {@link #XR_TYPE_SCENE_MESH_BUFFERS_MSFT TYPE_SCENE_MESH_BUFFERS_MSFT}
* - {@link #XR_TYPE_SCENE_MESH_VERTEX_BUFFER_MSFT TYPE_SCENE_MESH_VERTEX_BUFFER_MSFT}
* - {@link #XR_TYPE_SCENE_MESH_INDICES_UINT32_MSFT TYPE_SCENE_MESH_INDICES_UINT32_MSFT}
* - {@link #XR_TYPE_SCENE_MESH_INDICES_UINT16_MSFT TYPE_SCENE_MESH_INDICES_UINT16_MSFT}
*
*/
public static final int
XR_TYPE_SCENE_OBSERVER_CREATE_INFO_MSFT = 1000097000,
XR_TYPE_SCENE_CREATE_INFO_MSFT = 1000097001,
XR_TYPE_NEW_SCENE_COMPUTE_INFO_MSFT = 1000097002,
XR_TYPE_VISUAL_MESH_COMPUTE_LOD_INFO_MSFT = 1000097003,
XR_TYPE_SCENE_COMPONENTS_MSFT = 1000097004,
XR_TYPE_SCENE_COMPONENTS_GET_INFO_MSFT = 1000097005,
XR_TYPE_SCENE_COMPONENT_LOCATIONS_MSFT = 1000097006,
XR_TYPE_SCENE_COMPONENTS_LOCATE_INFO_MSFT = 1000097007,
XR_TYPE_SCENE_OBJECTS_MSFT = 1000097008,
XR_TYPE_SCENE_COMPONENT_PARENT_FILTER_INFO_MSFT = 1000097009,
XR_TYPE_SCENE_OBJECT_TYPES_FILTER_INFO_MSFT = 1000097010,
XR_TYPE_SCENE_PLANES_MSFT = 1000097011,
XR_TYPE_SCENE_PLANE_ALIGNMENT_FILTER_INFO_MSFT = 1000097012,
XR_TYPE_SCENE_MESHES_MSFT = 1000097013,
XR_TYPE_SCENE_MESH_BUFFERS_GET_INFO_MSFT = 1000097014,
XR_TYPE_SCENE_MESH_BUFFERS_MSFT = 1000097015,
XR_TYPE_SCENE_MESH_VERTEX_BUFFER_MSFT = 1000097016,
XR_TYPE_SCENE_MESH_INDICES_UINT32_MSFT = 1000097017,
XR_TYPE_SCENE_MESH_INDICES_UINT16_MSFT = 1000097018;
/**
* Extends {@code XrResult}.
*
* Enum values:
*
*
* - {@link #XR_ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT}
* - {@link #XR_ERROR_SCENE_COMPONENT_ID_INVALID_MSFT ERROR_SCENE_COMPONENT_ID_INVALID_MSFT}
* - {@link #XR_ERROR_SCENE_COMPONENT_TYPE_MISMATCH_MSFT ERROR_SCENE_COMPONENT_TYPE_MISMATCH_MSFT}
* - {@link #XR_ERROR_SCENE_MESH_BUFFER_ID_INVALID_MSFT ERROR_SCENE_MESH_BUFFER_ID_INVALID_MSFT}
* - {@link #XR_ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT}
* - {@link #XR_ERROR_SCENE_COMPUTE_CONSISTENCY_MISMATCH_MSFT ERROR_SCENE_COMPUTE_CONSISTENCY_MISMATCH_MSFT}
*
*/
public static final int
XR_ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT = -1000097000,
XR_ERROR_SCENE_COMPONENT_ID_INVALID_MSFT = -1000097001,
XR_ERROR_SCENE_COMPONENT_TYPE_MISMATCH_MSFT = -1000097002,
XR_ERROR_SCENE_MESH_BUFFER_ID_INVALID_MSFT = -1000097003,
XR_ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT = -1000097004,
XR_ERROR_SCENE_COMPUTE_CONSISTENCY_MISMATCH_MSFT = -1000097005;
/**
* XrSceneComputeFeatureMSFT - Scene compute feature
*
* Enumerant Descriptions
*
*
* - {@link #XR_SCENE_COMPUTE_FEATURE_PLANE_MSFT SCENE_COMPUTE_FEATURE_PLANE_MSFT} specifies that plane data for objects should be included in the resulting scene.
* - {@link #XR_SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT} specifies that planar meshes for objects should be included in the resulting scene.
* - {@link #XR_SCENE_COMPUTE_FEATURE_VISUAL_MESH_MSFT SCENE_COMPUTE_FEATURE_VISUAL_MESH_MSFT} specifies that 3D visualization meshes for objects should be included in the resulting scene.
* - {@link #XR_SCENE_COMPUTE_FEATURE_COLLIDER_MESH_MSFT SCENE_COMPUTE_FEATURE_COLLIDER_MESH_MSFT} specifies that 3D collider meshes for objects should be included in the resulting scene.
*
*
* Note
*
* Applications wanting to use the scene for analysis, or in a physics simulation should set {@code consistency} to {@link #XR_SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_COMPLETE_MSFT SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_COMPLETE_MSFT} in order to avoid physics objects falling through the gaps and escaping the scene.
*
* Setting {@code consistency} to {@link #XR_SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_INCOMPLETE_FAST_MSFT SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_INCOMPLETE_FAST_MSFT} might speed up the compute but it will result in gaps in the scene.
*
* Setting {@code consistency} to {@link #XR_SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT} should be done when the resulting mesh will only be used to occlude virtual objects that are behind real-world surfaces. This mode will be most efficient and have the lowest-latency, but will return meshes less suitable for analysis or visualization.
*
*
* See Also
*
* {@link XrNewSceneComputeInfoMSFT}, {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT}, {@link #xrEnumerateSceneComputeFeaturesMSFT EnumerateSceneComputeFeaturesMSFT}
*/
public static final int
XR_SCENE_COMPUTE_FEATURE_PLANE_MSFT = 1,
XR_SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT = 2,
XR_SCENE_COMPUTE_FEATURE_VISUAL_MESH_MSFT = 3,
XR_SCENE_COMPUTE_FEATURE_COLLIDER_MESH_MSFT = 4;
/**
* XrSceneComputeConsistencyMSFT - Scene compute consistency
*
* Enumerant Descriptions
*
*
* - {@link #XR_SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_COMPLETE_MSFT SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_COMPLETE_MSFT}. The runtime must return a scene that is a consistent and complete snapshot of the environment, inferring the size and shape of objects as needed where the objects were not directly observed, in order to generate a watertight representation of the scene.
* - {@link #XR_SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_INCOMPLETE_FAST_MSFT SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_INCOMPLETE_FAST_MSFT}. The runtime must return a consistent snapshot of the scene with meshes that do not overlap adjacent meshes at their edges, but may skip returning objects with {@code XrSceneObjectTypeMSFT} of {@link #XR_SCENE_OBJECT_TYPE_INFERRED_MSFT SCENE_OBJECT_TYPE_INFERRED_MSFT} in order to return the scene faster.
* - {@link #XR_SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT}. The runtime may react to this value by computing scenes more quickly and reusing existing mesh buffer IDs more often to minimize app overhead, with potential tradeoffs such as returning meshes that are not watertight, meshes that overlap adjacent meshes at their edges to allow partial updates in the future, or other reductions in mesh quality that are less observable when mesh is used for occlusion only.
*
*
* See Also
*
* {@link XrNewSceneComputeInfoMSFT}, {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT}
*/
public static final int
XR_SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_COMPLETE_MSFT = 1,
XR_SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_INCOMPLETE_FAST_MSFT = 2,
XR_SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT = 3;
/**
* XrMeshComputeLodMSFT - Mesh compute level of detail enumeration
*
* Enumerant Descriptions
*
*
* - {@link #XR_MESH_COMPUTE_LOD_COARSE_MSFT MESH_COMPUTE_LOD_COARSE_MSFT}. Coarse mesh compute level of detail will generate roughly 100 triangles per cubic meter.
* - {@link #XR_MESH_COMPUTE_LOD_MEDIUM_MSFT MESH_COMPUTE_LOD_MEDIUM_MSFT}. Medium mesh compute level of detail will generate roughly 400 triangles per cubic meter.
* - {@link #XR_MESH_COMPUTE_LOD_FINE_MSFT MESH_COMPUTE_LOD_FINE_MSFT}. Fine mesh compute level of detail will generate roughly 2000 triangles per cubic meter.
* - {@link #XR_MESH_COMPUTE_LOD_UNLIMITED_MSFT MESH_COMPUTE_LOD_UNLIMITED_MSFT}. Unlimited mesh compute level of detail. There is no guarantee as to the number of triangles returned.
*
*
* See Also
*
* {@link XrVisualMeshComputeLodInfoMSFT}
*/
public static final int
XR_MESH_COMPUTE_LOD_COARSE_MSFT = 1,
XR_MESH_COMPUTE_LOD_MEDIUM_MSFT = 2,
XR_MESH_COMPUTE_LOD_FINE_MSFT = 3,
XR_MESH_COMPUTE_LOD_UNLIMITED_MSFT = 4;
/**
* XrSceneComponentTypeMSFT - Scene component type
*
* Enumerant Descriptions
*
*
* - {@link #XR_SCENE_COMPONENT_TYPE_INVALID_MSFT SCENE_COMPONENT_TYPE_INVALID_MSFT} indicates an invalid scene component type.
* - {@link #XR_SCENE_COMPONENT_TYPE_OBJECT_MSFT SCENE_COMPONENT_TYPE_OBJECT_MSFT} indicates a discrete object detected in the world, such as a wall, floor, ceiling or table. Scene objects then provide their geometric representations such as planes and meshes as child scene components with the types below.
* - {@link #XR_SCENE_COMPONENT_TYPE_PLANE_MSFT SCENE_COMPONENT_TYPE_PLANE_MSFT} indicates a flat 2D representation of a surface in the world, such as a wall, floor, ceiling or table.
* - {@link #XR_SCENE_COMPONENT_TYPE_VISUAL_MESH_MSFT SCENE_COMPONENT_TYPE_VISUAL_MESH_MSFT} indicates a visual mesh representation of an object in the world, optimized for visual quality when directly rendering a wireframe or other mesh visualization to the user. Visual mesh can also be used for rendering the silhouettes of objects. Applications can request varying levels of detail for visual meshes when calling {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} using {@link XrVisualMeshComputeLodInfoMSFT}.
* - {@link #XR_SCENE_COMPONENT_TYPE_COLLIDER_MESH_MSFT SCENE_COMPONENT_TYPE_COLLIDER_MESH_MSFT} indicates a collider mesh representation of an object in the world, optimized to maintain the silhouette of an object while reducing detail on mostly-flat surfaces. Collider mesh is useful when calculating physics collisions or when rendering silhouettes of objects for occlusion.
*
*
* See Also
*
* {@link XrSceneComponentMSFT}, {@link XrSceneComponentsGetInfoMSFT}
*/
public static final int
XR_SCENE_COMPONENT_TYPE_INVALID_MSFT = -1,
XR_SCENE_COMPONENT_TYPE_OBJECT_MSFT = 1,
XR_SCENE_COMPONENT_TYPE_PLANE_MSFT = 2,
XR_SCENE_COMPONENT_TYPE_VISUAL_MESH_MSFT = 3,
XR_SCENE_COMPONENT_TYPE_COLLIDER_MESH_MSFT = 4;
/**
* XrSceneObjectTypeMSFT - Scene object type
*
* Enumerant Descriptions
*
*
* - {@link #XR_SCENE_OBJECT_TYPE_UNCATEGORIZED_MSFT SCENE_OBJECT_TYPE_UNCATEGORIZED_MSFT}. This scene object has yet to be classified and assigned a type. This should not be confused with background, as this object could be anything; the system has just not come up with a strong enough classification for it yet.
* - {@link #XR_SCENE_OBJECT_TYPE_BACKGROUND_MSFT SCENE_OBJECT_TYPE_BACKGROUND_MSFT}. The scene object is known to be not one of the other recognized types of scene object. This class should not be confused with uncategorized where background is known not to be wall/floor/ceiling etc. while uncategorized is not yet categorized.
* - {@link #XR_SCENE_OBJECT_TYPE_WALL_MSFT SCENE_OBJECT_TYPE_WALL_MSFT}. A physical wall. Walls are assumed to be immovable environmental structures.
* - {@link #XR_SCENE_OBJECT_TYPE_FLOOR_MSFT SCENE_OBJECT_TYPE_FLOOR_MSFT}. Floors are any surfaces on which one can walk. Note: stairs are not floors. Also note, that floors assume any walkable surface and therefore there is no explicit assumption of a singular floor. Multi-level structures, ramps, etc. should all classify as floor.
* - {@link #XR_SCENE_OBJECT_TYPE_CEILING_MSFT SCENE_OBJECT_TYPE_CEILING_MSFT}. The upper surface of a room.
* - {@link #XR_SCENE_OBJECT_TYPE_PLATFORM_MSFT SCENE_OBJECT_TYPE_PLATFORM_MSFT}. A large flat surface on which you could place holograms. These tend to represent tables, countertops, and other large horizontal surfaces.
* - {@link #XR_SCENE_OBJECT_TYPE_INFERRED_MSFT SCENE_OBJECT_TYPE_INFERRED_MSFT}. An imaginary object that was added to the scene in order to make the scene watertight and avoid gaps.
*
*
* See Also
*
* {@link XrNewSceneComputeInfoMSFT}, {@link XrSceneObjectMSFT}, {@link XrSceneObjectTypesFilterInfoMSFT}
*/
public static final int
XR_SCENE_OBJECT_TYPE_UNCATEGORIZED_MSFT = -1,
XR_SCENE_OBJECT_TYPE_BACKGROUND_MSFT = 1,
XR_SCENE_OBJECT_TYPE_WALL_MSFT = 2,
XR_SCENE_OBJECT_TYPE_FLOOR_MSFT = 3,
XR_SCENE_OBJECT_TYPE_CEILING_MSFT = 4,
XR_SCENE_OBJECT_TYPE_PLATFORM_MSFT = 5,
XR_SCENE_OBJECT_TYPE_INFERRED_MSFT = 6;
/**
* XrScenePlaneAlignmentTypeMSFT - Scene plane alignment type
*
* Enumerant Descriptions
*
*
* - {@link #XR_SCENE_PLANE_ALIGNMENT_TYPE_NON_ORTHOGONAL_MSFT SCENE_PLANE_ALIGNMENT_TYPE_NON_ORTHOGONAL_MSFT} means the plane’s normal is not orthogonal or parallel to the gravity direction.
* - {@link #XR_SCENE_PLANE_ALIGNMENT_TYPE_HORIZONTAL_MSFT SCENE_PLANE_ALIGNMENT_TYPE_HORIZONTAL_MSFT} means the plane’s normal is roughly parallel to the gravity direction.
* - {@link #XR_SCENE_PLANE_ALIGNMENT_TYPE_VERTICAL_MSFT SCENE_PLANE_ALIGNMENT_TYPE_VERTICAL_MSFT} means the plane’s normal is roughly orthogonal to the gravity direction.
*
*
* See Also
*
* {@link XrScenePlaneAlignmentFilterInfoMSFT}, {@link XrScenePlaneMSFT}
*/
public static final int
XR_SCENE_PLANE_ALIGNMENT_TYPE_NON_ORTHOGONAL_MSFT = 0,
XR_SCENE_PLANE_ALIGNMENT_TYPE_HORIZONTAL_MSFT = 1,
XR_SCENE_PLANE_ALIGNMENT_TYPE_VERTICAL_MSFT = 2;
/**
* XrSceneComputeStateMSFT - Scene compute state type
*
* Enumerant Descriptions
*
*
* - {@link #XR_SCENE_COMPUTE_STATE_NONE_MSFT SCENE_COMPUTE_STATE_NONE_MSFT} indicates that no scene is available, and that a scene is not being computed. The application may call {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} to start computing a scene.
* - {@link #XR_SCENE_COMPUTE_STATE_UPDATING_MSFT SCENE_COMPUTE_STATE_UPDATING_MSFT} indicates that a new scene is being computed. Calling {@link #xrCreateSceneMSFT CreateSceneMSFT} or {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} must return the error {@link #XR_ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT}.
* - {@link #XR_SCENE_COMPUTE_STATE_COMPLETED_MSFT SCENE_COMPUTE_STATE_COMPLETED_MSFT} indicates that a new scene has completed computing. The application may call {@link #xrCreateSceneMSFT CreateSceneMSFT} to get the results of the query or the application may call {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} to start computing a new scene.
* - {@link #XR_SCENE_COMPUTE_STATE_COMPLETED_WITH_ERROR_MSFT SCENE_COMPUTE_STATE_COMPLETED_WITH_ERROR_MSFT} indicates that the new scene computation completed with an error. Calling {@link #xrCreateSceneMSFT CreateSceneMSFT} must return a valid {@code XrSceneMSFT} handle but calling {@link #xrGetSceneComponentsMSFT GetSceneComponentsMSFT} with that handle must return zero scene components. The runtime must allow the application to call {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} to try computing a scene again, even if the last call to {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} resulted in {@link #XR_SCENE_COMPUTE_STATE_COMPLETED_WITH_ERROR_MSFT SCENE_COMPUTE_STATE_COMPLETED_WITH_ERROR_MSFT}.
*
*
* See Also
*
* {@link #xrGetSceneComputeStateMSFT GetSceneComputeStateMSFT}
*/
public static final int
XR_SCENE_COMPUTE_STATE_NONE_MSFT = 0,
XR_SCENE_COMPUTE_STATE_UPDATING_MSFT = 1,
XR_SCENE_COMPUTE_STATE_COMPLETED_MSFT = 2,
XR_SCENE_COMPUTE_STATE_COMPLETED_WITH_ERROR_MSFT = 3;
protected MSFTSceneUnderstanding() {
throw new UnsupportedOperationException();
}
// --- [ xrEnumerateSceneComputeFeaturesMSFT ] ---
/**
* Unsafe version of: {@link #xrEnumerateSceneComputeFeaturesMSFT EnumerateSceneComputeFeaturesMSFT}
*
* @param featureCapacityInput the capacity of the array, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrEnumerateSceneComputeFeaturesMSFT(XrInstance instance, long systemId, int featureCapacityInput, long featureCountOutput, long features) {
long __functionAddress = instance.getCapabilities().xrEnumerateSceneComputeFeaturesMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPJPPI(instance.address(), systemId, featureCapacityInput, featureCountOutput, features, __functionAddress);
}
/**
* Enumerates scene compute features.
*
* C Specification
*
* The {@link #xrEnumerateSceneComputeFeaturesMSFT EnumerateSceneComputeFeaturesMSFT} function enumerates the supported scene compute features of the given system.
*
* This function follows the two-call idiom for filling the {@code features} array.
*
*
* XrResult xrEnumerateSceneComputeFeaturesMSFT(
* XrInstance instance,
* XrSystemId systemId,
* uint32_t featureCapacityInput,
* uint32_t* featureCountOutput,
* XrSceneComputeFeatureMSFT* features);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} extension must be enabled prior to calling {@link #xrEnumerateSceneComputeFeaturesMSFT EnumerateSceneComputeFeaturesMSFT}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code featureCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code featureCapacityInput} is not 0, {@code features} must be a pointer to an array of {@code featureCapacityInput} {@code XrSceneComputeFeatureMSFT} values
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link XR10#XR_ERROR_SYSTEM_INVALID ERROR_SYSTEM_INVALID}
*
*
*
* See Also
*
* {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT}
*
* @param instance a handle to an {@code XrInstance}.
* @param systemId the {@code XrSystemId} whose scene compute features will be enumerated.
* @param featureCountOutput a pointer to the count of scene compute features, or a pointer to the required capacity in the case that {@code featureCapacityInput} is insufficient.
* @param features an array of {@code XrSceneComputeFeatureMSFT}.
*/
@NativeType("XrResult")
public static int xrEnumerateSceneComputeFeaturesMSFT(XrInstance instance, @NativeType("XrSystemId") long systemId, @NativeType("uint32_t *") IntBuffer featureCountOutput, @NativeType("XrSceneComputeFeatureMSFT *") @Nullable IntBuffer features) {
if (CHECKS) {
check(featureCountOutput, 1);
}
return nxrEnumerateSceneComputeFeaturesMSFT(instance, systemId, remainingSafe(features), memAddress(featureCountOutput), memAddressSafe(features));
}
// --- [ xrCreateSceneObserverMSFT ] ---
/** Unsafe version of: {@link #xrCreateSceneObserverMSFT CreateSceneObserverMSFT} */
public static int nxrCreateSceneObserverMSFT(XrSession session, long createInfo, long sceneObserver) {
long __functionAddress = session.getCapabilities().xrCreateSceneObserverMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), createInfo, sceneObserver, __functionAddress);
}
/**
* Create a scene observer handle.
*
* C Specification
*
* An {@code XrSceneObserverMSFT} handle is created using {@link #xrCreateSceneObserverMSFT CreateSceneObserverMSFT}.
*
*
* XrResult xrCreateSceneObserverMSFT(
* XrSession session,
* const XrSceneObserverCreateInfoMSFT* createInfo,
* XrSceneObserverMSFT* sceneObserver);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} extension must be enabled prior to calling {@link #xrCreateSceneObserverMSFT CreateSceneObserverMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - If {@code createInfo} is not {@code NULL}, {@code createInfo} must be a pointer to a valid {@link XrSceneObserverCreateInfoMSFT} structure
* - {@code sceneObserver} must be a pointer to an {@code XrSceneObserverMSFT} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* See Also
*
* {@link XrSceneObserverCreateInfoMSFT}, {@link #xrDestroySceneObserverMSFT DestroySceneObserverMSFT}
*
* @param session an {@code XrSession} in which the scene observer will be active.
* @param createInfo exists for extensibility purposes, it is {@code NULL} or a pointer to a valid {@link XrSceneObserverCreateInfoMSFT} structure.
* @param sceneObserver the returned {@code XrSceneObserverMSFT} handle.
*/
@NativeType("XrResult")
public static int xrCreateSceneObserverMSFT(XrSession session, @NativeType("XrSceneObserverCreateInfoMSFT const *") @Nullable XrSceneObserverCreateInfoMSFT createInfo, @NativeType("XrSceneObserverMSFT *") PointerBuffer sceneObserver) {
if (CHECKS) {
check(sceneObserver, 1);
}
return nxrCreateSceneObserverMSFT(session, memAddressSafe(createInfo), memAddress(sceneObserver));
}
// --- [ xrDestroySceneObserverMSFT ] ---
/**
* Destroy a scene observer handle.
*
* C Specification
*
* The {@link #xrDestroySceneObserverMSFT DestroySceneObserverMSFT} function releases the {@code sceneObserver} and the underlying resources.
*
*
* XrResult xrDestroySceneObserverMSFT(
* XrSceneObserverMSFT sceneObserver);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} extension must be enabled prior to calling {@link #xrDestroySceneObserverMSFT DestroySceneObserverMSFT}
* - {@code sceneObserver} must be a valid {@code XrSceneObserverMSFT} handle
*
*
* Thread Safety
*
*
* - Access to {@code sceneObserver}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* See Also
*
* {@link #xrCreateSceneObserverMSFT CreateSceneObserverMSFT}
*
* @param sceneObserver an {@code XrSceneObserverMSFT} previously created by {@link #xrCreateSceneObserverMSFT CreateSceneObserverMSFT}.
*/
@NativeType("XrResult")
public static int xrDestroySceneObserverMSFT(XrSceneObserverMSFT sceneObserver) {
long __functionAddress = sceneObserver.getCapabilities().xrDestroySceneObserverMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPI(sceneObserver.address(), __functionAddress);
}
// --- [ xrCreateSceneMSFT ] ---
/** Unsafe version of: {@link #xrCreateSceneMSFT CreateSceneMSFT} */
public static int nxrCreateSceneMSFT(XrSceneObserverMSFT sceneObserver, long createInfo, long scene) {
long __functionAddress = sceneObserver.getCapabilities().xrCreateSceneMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(sceneObserver.address(), createInfo, scene, __functionAddress);
}
/**
* Create a scene handle.
*
* C Specification
*
* The {@link #xrCreateSceneMSFT CreateSceneMSFT} functions creates an {@code XrSceneMSFT} handle. It can only be called after {@link #xrGetSceneComputeStateMSFT GetSceneComputeStateMSFT} returns {@link #XR_SCENE_COMPUTE_STATE_COMPLETED_MSFT SCENE_COMPUTE_STATE_COMPLETED_MSFT} to indicate that the asynchronous operation has completed. The {@code XrSceneMSFT} handle manages the collection of scene components that represents the detected objects found during the query.
*
* After an {@code XrSceneMSFT} handle is created, the handle and associated data must remain valid until destroyed, even after {@link #xrCreateSceneMSFT CreateSceneMSFT} is called again to create the next scene. The runtime must keep alive any component data and mesh buffers relating to this historical scene until its handle is destroyed.
*
*
* XrResult xrCreateSceneMSFT(
* XrSceneObserverMSFT sceneObserver,
* const XrSceneCreateInfoMSFT* createInfo,
* XrSceneMSFT* scene);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} extension must be enabled prior to calling {@link #xrCreateSceneMSFT CreateSceneMSFT}
* - {@code sceneObserver} must be a valid {@code XrSceneObserverMSFT} handle
* - If {@code createInfo} is not {@code NULL}, {@code createInfo} must be a pointer to a valid {@link XrSceneCreateInfoMSFT} structure
* - {@code scene} must be a pointer to an {@code XrSceneMSFT} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link #XR_ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT}
*
*
*
* Calling {@link #xrCreateSceneMSFT CreateSceneMSFT} when {@link #xrGetSceneComputeStateMSFT GetSceneComputeStateMSFT} returns {@link #XR_SCENE_COMPUTE_STATE_NONE_MSFT SCENE_COMPUTE_STATE_NONE_MSFT} or {@link #XR_SCENE_COMPUTE_STATE_UPDATING_MSFT SCENE_COMPUTE_STATE_UPDATING_MSFT} must return the error {@link #XR_ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT}.
*
* See Also
*
* {@link XrSceneCreateInfoMSFT}, {@link #xrDestroySceneMSFT DestroySceneMSFT}
*
* @param sceneObserver a handle to an {@code XrSceneObserverMSFT}.
* @param createInfo exists for extensibility purposes, it is {@code NULL} or a pointer to a valid {@link XrSceneCreateInfoMSFT} structure.
* @param scene the returned {@code XrSceneMSFT} handle.
*/
@NativeType("XrResult")
public static int xrCreateSceneMSFT(XrSceneObserverMSFT sceneObserver, @NativeType("XrSceneCreateInfoMSFT const *") @Nullable XrSceneCreateInfoMSFT createInfo, @NativeType("XrSceneMSFT *") PointerBuffer scene) {
if (CHECKS) {
check(scene, 1);
}
return nxrCreateSceneMSFT(sceneObserver, memAddressSafe(createInfo), memAddress(scene));
}
// --- [ xrDestroySceneMSFT ] ---
/**
* Destroy a scene handle.
*
* C Specification
*
* The {@link #xrDestroySceneMSFT DestroySceneMSFT} function releases the {@code scene} and the underlying resources.
*
*
* XrResult xrDestroySceneMSFT(
* XrSceneMSFT scene);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} extension must be enabled prior to calling {@link #xrDestroySceneMSFT DestroySceneMSFT}
* - {@code scene} must be a valid {@code XrSceneMSFT} handle
*
*
* Thread Safety
*
*
* - Access to {@code scene}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* See Also
*
* {@link #xrCreateSceneMSFT CreateSceneMSFT}
*
* @param scene an {@code XrSceneMSFT} previously created by {@link #xrCreateSceneMSFT CreateSceneMSFT}.
*/
@NativeType("XrResult")
public static int xrDestroySceneMSFT(XrSceneMSFT scene) {
long __functionAddress = scene.getCapabilities().xrDestroySceneMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPI(scene.address(), __functionAddress);
}
// --- [ xrComputeNewSceneMSFT ] ---
/** Unsafe version of: {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} */
public static int nxrComputeNewSceneMSFT(XrSceneObserverMSFT sceneObserver, long computeInfo) {
long __functionAddress = sceneObserver.getCapabilities().xrComputeNewSceneMSFT;
if (CHECKS) {
check(__functionAddress);
XrNewSceneComputeInfoMSFT.validate(computeInfo);
}
return callPPI(sceneObserver.address(), computeInfo, __functionAddress);
}
/**
* Compute new scene.
*
* C Specification
*
* The {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} function begins the compute of a new scene and the runtime must return quickly without waiting for the compute to complete. The application should use {@link #xrGetSceneComputeStateMSFT GetSceneComputeStateMSFT} to inspect the compute status.
*
* The application can control the compute features by passing a list of {@code XrSceneComputeFeatureMSFT} via {@link XrNewSceneComputeInfoMSFT}{@code ::requestedFeatures}.
*
*
* - If {@link #XR_SCENE_COMPUTE_FEATURE_PLANE_MSFT SCENE_COMPUTE_FEATURE_PLANE_MSFT} is passed, but {@link #XR_SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT} is not passed, then:
*
*
* - The application may be able to read {@link #XR_SCENE_COMPONENT_TYPE_PLANE_MSFT SCENE_COMPONENT_TYPE_PLANE_MSFT} and {@link #XR_SCENE_COMPONENT_TYPE_OBJECT_MSFT SCENE_COMPONENT_TYPE_OBJECT_MSFT} scene components from the resulting {@code XrSceneMSFT} handle.
* - {@link XrScenePlaneMSFT}{@code ::meshBufferId} must be zero to indicate that the plane scene component does not have a mesh buffer available to read.
*
*
* - If {@link #XR_SCENE_COMPUTE_FEATURE_PLANE_MSFT SCENE_COMPUTE_FEATURE_PLANE_MSFT} and {@link #XR_SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT} are passed, then:
*
*
* - the application may be able to read {@link #XR_SCENE_COMPONENT_TYPE_PLANE_MSFT SCENE_COMPONENT_TYPE_PLANE_MSFT} and {@link #XR_SCENE_COMPONENT_TYPE_OBJECT_MSFT SCENE_COMPONENT_TYPE_OBJECT_MSFT} scene components from the resulting {@code XrSceneMSFT} handle
* - {@link XrScenePlaneMSFT}{@code ::meshBufferId} may contain a non-zero mesh buffer identifier to indicate that the plane scene component has a mesh buffer available to read.
*
*
* - If {@link #XR_SCENE_COMPUTE_FEATURE_VISUAL_MESH_MSFT SCENE_COMPUTE_FEATURE_VISUAL_MESH_MSFT} is passed then:
*
*
* - the application may be able to read {@link #XR_SCENE_COMPONENT_TYPE_VISUAL_MESH_MSFT SCENE_COMPONENT_TYPE_VISUAL_MESH_MSFT} and {@link #XR_SCENE_COMPONENT_TYPE_OBJECT_MSFT SCENE_COMPONENT_TYPE_OBJECT_MSFT} scene components from the resulting {@code XrSceneMSFT} handle.
*
*
* - If {@link #XR_SCENE_COMPUTE_FEATURE_COLLIDER_MESH_MSFT SCENE_COMPUTE_FEATURE_COLLIDER_MESH_MSFT} is passed then:
*
*
* - the application may be able to read {@link #XR_SCENE_COMPONENT_TYPE_COLLIDER_MESH_MSFT SCENE_COMPONENT_TYPE_COLLIDER_MESH_MSFT} and {@link #XR_SCENE_COMPONENT_TYPE_OBJECT_MSFT SCENE_COMPONENT_TYPE_OBJECT_MSFT} scene components from the resulting {@code XrSceneMSFT} handle.
*
*
*
*
*
* XrResult xrComputeNewSceneMSFT(
* XrSceneObserverMSFT sceneObserver,
* const XrNewSceneComputeInfoMSFT* computeInfo);
*
* Description
*
* The runtime must return {@link #XR_ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT} if incompatible features were passed or no compatible features were passed.
*
* The runtime must return {@link #XR_ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT} if {@link #XR_SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT} was passed but {@link #XR_SCENE_COMPUTE_FEATURE_PLANE_MSFT SCENE_COMPUTE_FEATURE_PLANE_MSFT} was not passed.
*
* The runtime must return {@link #XR_ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT} if {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} is called while the scene computation is in progress.
*
* An application that wishes to use {@link #XR_SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT} must create an {@code XrSceneObserverMSFT} handle that passes neither {@link #XR_SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_COMPLETE_MSFT SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_COMPLETE_MSFT} nor {@link #XR_SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_INCOMPLETE_FAST_MSFT SCENE_COMPUTE_CONSISTENCY_SNAPSHOT_INCOMPLETE_FAST_MSFT} to {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} for the lifetime of that {@code XrSceneObserverMSFT} handle. This allows the runtime to return occlusion mesh at a different cadence than non-occlusion mesh or planes.
*
*
* - The runtime must return {@link #XR_ERROR_SCENE_COMPUTE_CONSISTENCY_MISMATCH_MSFT ERROR_SCENE_COMPUTE_CONSISTENCY_MISMATCH_MSFT} if:
*
*
* - {@link #XR_SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT} is passed to {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} and
* - a previous call to {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} did not pass {@link #XR_SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT} for the same {@code XrSceneObserverMSFT} handle.
*
*
* - The runtime must return {@link #XR_ERROR_SCENE_COMPUTE_CONSISTENCY_MISMATCH_MSFT ERROR_SCENE_COMPUTE_CONSISTENCY_MISMATCH_MSFT} if:
*
*
* - {@link #XR_SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT} is not passed to {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} and
* - a previous call to {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} did pass {@link #XR_SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT} for the same {@code XrSceneObserverMSFT} handle.
*
*
* - The runtime must return {@link #XR_ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT} if:
*
*
* - {@link #XR_SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT} is passed to {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} and
* - neither {@link #XR_SCENE_COMPUTE_FEATURE_VISUAL_MESH_MSFT SCENE_COMPUTE_FEATURE_VISUAL_MESH_MSFT} nor {@link #XR_SCENE_COMPUTE_FEATURE_COLLIDER_MESH_MSFT SCENE_COMPUTE_FEATURE_COLLIDER_MESH_MSFT} are also passed.
*
*
* - The runtime must return {@link #XR_ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT} if:
*
*
* - {@link #XR_SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT SCENE_COMPUTE_CONSISTENCY_OCCLUSION_OPTIMIZED_MSFT} is passed to {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT} and
* - at least one of {@link MSFTSceneUnderstandingSerialization#XR_SCENE_COMPUTE_FEATURE_SERIALIZE_SCENE_MSFT SCENE_COMPUTE_FEATURE_SERIALIZE_SCENE_MSFT}, {@link #XR_SCENE_COMPUTE_FEATURE_PLANE_MSFT SCENE_COMPUTE_FEATURE_PLANE_MSFT}, {@link #XR_SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT SCENE_COMPUTE_FEATURE_PLANE_MESH_MSFT}, or {@link MSFTSceneUnderstandingSerialization#XR_SCENE_COMPUTE_FEATURE_SERIALIZE_SCENE_MSFT SCENE_COMPUTE_FEATURE_SERIALIZE_SCENE_MSFT} are also passed.
*
*
*
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} extension must be enabled prior to calling {@link #xrComputeNewSceneMSFT ComputeNewSceneMSFT}
* - {@code sceneObserver} must be a valid {@code XrSceneObserverMSFT} handle
* - {@code computeInfo} must be a pointer to a valid {@link XrNewSceneComputeInfoMSFT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
* - {@link #XR_ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT ERROR_SCENE_COMPUTE_FEATURE_INCOMPATIBLE_MSFT}
* - {@link #XR_ERROR_SCENE_COMPUTE_CONSISTENCY_MISMATCH_MSFT ERROR_SCENE_COMPUTE_CONSISTENCY_MISMATCH_MSFT}
* - {@link XR10#XR_ERROR_POSE_INVALID ERROR_POSE_INVALID}
* - {@link #XR_ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT ERROR_COMPUTE_NEW_SCENE_NOT_COMPLETED_MSFT}
*
*
*
* See Also
*
* {@link XrNewSceneComputeInfoMSFT}, {@link #xrEnumerateSceneComputeFeaturesMSFT EnumerateSceneComputeFeaturesMSFT}
*
* @param sceneObserver a handle to an {@code XrSceneObserverMSFT}.
* @param computeInfo a pointer to an {@link XrNewSceneComputeInfoMSFT} structure.
*/
@NativeType("XrResult")
public static int xrComputeNewSceneMSFT(XrSceneObserverMSFT sceneObserver, @NativeType("XrNewSceneComputeInfoMSFT const *") XrNewSceneComputeInfoMSFT computeInfo) {
return nxrComputeNewSceneMSFT(sceneObserver, computeInfo.address());
}
// --- [ xrGetSceneComputeStateMSFT ] ---
/** Unsafe version of: {@link #xrGetSceneComputeStateMSFT GetSceneComputeStateMSFT} */
public static int nxrGetSceneComputeStateMSFT(XrSceneObserverMSFT sceneObserver, long state) {
long __functionAddress = sceneObserver.getCapabilities().xrGetSceneComputeStateMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(sceneObserver.address(), state, __functionAddress);
}
/**
* Get the scene compute state.
*
* C Specification
*
* An application can inspect the completion of the compute by polling {@link #xrGetSceneComputeStateMSFT GetSceneComputeStateMSFT}. This function should typically be called once per frame per {@code XrSceneObserverMSFT}.
*
*
* XrResult xrGetSceneComputeStateMSFT(
* XrSceneObserverMSFT sceneObserver,
* XrSceneComputeStateMSFT* state);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} extension must be enabled prior to calling {@link #xrGetSceneComputeStateMSFT GetSceneComputeStateMSFT}
* - {@code sceneObserver} must be a valid {@code XrSceneObserverMSFT} handle
* - {@code state} must be a pointer to an {@code XrSceneComputeStateMSFT} value
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
*
*
*
* @param sceneObserver a handle to an {@code XrSceneObserverMSFT}.
* @param state the returned {@code XrSceneComputeStateMSFT} value.
*/
@NativeType("XrResult")
public static int xrGetSceneComputeStateMSFT(XrSceneObserverMSFT sceneObserver, @NativeType("XrSceneComputeStateMSFT *") IntBuffer state) {
if (CHECKS) {
check(state, 1);
}
return nxrGetSceneComputeStateMSFT(sceneObserver, memAddress(state));
}
// --- [ xrGetSceneComponentsMSFT ] ---
/** Unsafe version of: {@link #xrGetSceneComponentsMSFT GetSceneComponentsMSFT} */
public static int nxrGetSceneComponentsMSFT(XrSceneMSFT scene, long getInfo, long components) {
long __functionAddress = scene.getCapabilities().xrGetSceneComponentsMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(scene.address(), getInfo, components, __functionAddress);
}
/**
* Get scene components from a scene.
*
* C Specification
*
* Scene components are read from an {@code XrSceneMSFT} using {@link #xrGetSceneComponentsMSFT GetSceneComponentsMSFT} and passing one {@code XrSceneComponentTypeMSFT}. This function follows the two-call idiom for filling multiple buffers in a struct. Different scene component types may have additional properties that can be read by chaining additional structures to {@link XrSceneComponentsMSFT}. Those additional structures must have an array size that is at least as large as {@link XrSceneComponentsMSFT}::componentCapacityInput, otherwise the runtime must return {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}.
*
*
* - If {@link #XR_SCENE_COMPONENT_TYPE_OBJECT_MSFT SCENE_COMPONENT_TYPE_OBJECT_MSFT} is passed to {@link #xrGetSceneComponentsMSFT GetSceneComponentsMSFT}, then {@link XrSceneObjectsMSFT} may be included in the {@link XrSceneComponentsMSFT}{@code ::next} chain.
* - If {@link #XR_SCENE_COMPONENT_TYPE_PLANE_MSFT SCENE_COMPONENT_TYPE_PLANE_MSFT} is passed to {@link #xrGetSceneComponentsMSFT GetSceneComponentsMSFT}, then {@link XrScenePlanesMSFT} may be included in the {@link XrSceneComponentsMSFT}{@code ::next} chain.
* - If {@link #XR_SCENE_COMPONENT_TYPE_VISUAL_MESH_MSFT SCENE_COMPONENT_TYPE_VISUAL_MESH_MSFT} or {@link #XR_SCENE_COMPONENT_TYPE_COLLIDER_MESH_MSFT SCENE_COMPONENT_TYPE_COLLIDER_MESH_MSFT} are passed to {@link #xrGetSceneComponentsMSFT GetSceneComponentsMSFT}, then {@link XrSceneMeshesMSFT} may be included in the {@link XrSceneComponentsMSFT}{@code ::next} chain.
*
*
*
* XrResult xrGetSceneComponentsMSFT(
* XrSceneMSFT scene,
* const XrSceneComponentsGetInfoMSFT* getInfo,
* XrSceneComponentsMSFT* components);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} extension must be enabled prior to calling {@link #xrGetSceneComponentsMSFT GetSceneComponentsMSFT}
* - {@code scene} must be a valid {@code XrSceneMSFT} handle
* - {@code getInfo} must be a pointer to a valid {@link XrSceneComponentsGetInfoMSFT} structure
* - {@code components} must be a pointer to an {@link XrSceneComponentsMSFT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link #XR_ERROR_SCENE_COMPONENT_TYPE_MISMATCH_MSFT ERROR_SCENE_COMPONENT_TYPE_MISMATCH_MSFT}
*
*
*
* See Also
*
* {@link XrSceneComponentsGetInfoMSFT}, {@link XrSceneComponentsMSFT}
*
* @param scene an {@code XrSceneMSFT} previously created by {@link #xrCreateSceneMSFT CreateSceneMSFT}.
* @param getInfo a pointer to an {@link XrSceneComponentsGetInfoMSFT} structure.
* @param components the {@link XrSceneComponentsMSFT} output structure.
*/
@NativeType("XrResult")
public static int xrGetSceneComponentsMSFT(XrSceneMSFT scene, @NativeType("XrSceneComponentsGetInfoMSFT const *") XrSceneComponentsGetInfoMSFT getInfo, @NativeType("XrSceneComponentsMSFT *") XrSceneComponentsMSFT components) {
return nxrGetSceneComponentsMSFT(scene, getInfo.address(), components.address());
}
// --- [ xrLocateSceneComponentsMSFT ] ---
/** Unsafe version of: {@link #xrLocateSceneComponentsMSFT LocateSceneComponentsMSFT} */
public static int nxrLocateSceneComponentsMSFT(XrSceneMSFT scene, long locateInfo, long locations) {
long __functionAddress = scene.getCapabilities().xrLocateSceneComponentsMSFT;
if (CHECKS) {
check(__functionAddress);
XrSceneComponentsLocateInfoMSFT.validate(locateInfo);
}
return callPPPI(scene.address(), locateInfo, locations, __functionAddress);
}
/**
* Locate scene components.
*
* C Specification
*
* The {@link #xrLocateSceneComponentsMSFT LocateSceneComponentsMSFT} function locates an array of scene components to a base space at a given time.
*
*
* XrResult xrLocateSceneComponentsMSFT(
* XrSceneMSFT scene,
* const XrSceneComponentsLocateInfoMSFT* locateInfo,
* XrSceneComponentLocationsMSFT* locations);
*
* Description
*
* The runtime must return {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT} if {@link XrSceneComponentLocationsMSFT}{@code ::locationCount} is less than {@link XrSceneComponentsLocateInfoMSFT}{@code ::componentIdCount}.
*
* Note
*
* Similar to {@link XR10#xrLocateSpace LocateSpace}, apps should call {@link #xrLocateSceneComponentsMSFT LocateSceneComponentsMSFT} each frame because the location returned by {@link #xrLocateSceneComponentsMSFT LocateSceneComponentsMSFT} in later frames may change over time as the target space or the scene components may refine their locations.
*
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} extension must be enabled prior to calling {@link #xrLocateSceneComponentsMSFT LocateSceneComponentsMSFT}
* - {@code scene} must be a valid {@code XrSceneMSFT} handle
* - {@code locateInfo} must be a pointer to a valid {@link XrSceneComponentsLocateInfoMSFT} structure
* - {@code locations} must be a pointer to an {@link XrSceneComponentLocationsMSFT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
*
*
*
* See Also
*
* {@link XrSceneComponentLocationsMSFT}, {@link XrSceneComponentsLocateInfoMSFT}
*
* @param scene a handle to an {@code XrSceneMSFT}.
* @param locateInfo a pointer to {@link XrSceneComponentsLocateInfoMSFT} describing information to locate scene components.
* @param locations a pointer to {@link XrSceneComponentLocationsMSFT} receiving the returned scene component locations.
*/
@NativeType("XrResult")
public static int xrLocateSceneComponentsMSFT(XrSceneMSFT scene, @NativeType("XrSceneComponentsLocateInfoMSFT const *") XrSceneComponentsLocateInfoMSFT locateInfo, @NativeType("XrSceneComponentLocationsMSFT *") XrSceneComponentLocationsMSFT locations) {
return nxrLocateSceneComponentsMSFT(scene, locateInfo.address(), locations.address());
}
// --- [ xrGetSceneMeshBuffersMSFT ] ---
/** Unsafe version of: {@link #xrGetSceneMeshBuffersMSFT GetSceneMeshBuffersMSFT} */
public static int nxrGetSceneMeshBuffersMSFT(XrSceneMSFT scene, long getInfo, long buffers) {
long __functionAddress = scene.getCapabilities().xrGetSceneMeshBuffersMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(scene.address(), getInfo, buffers, __functionAddress);
}
/**
* Get scene mesh buffers.
*
* C Specification
*
* The {@link #xrGetSceneMeshBuffersMSFT GetSceneMeshBuffersMSFT} function retrieves the scene mesh vertex buffer and index buffer for the given scene mesh buffer identifier.
*
* Note
*
* Applications may use the scene mesh buffer identifier as a key to cache the vertices and indices of a mesh for reuse within an {@code XrSceneMSFT} or across multiple {@code XrSceneMSFT} for the same {@code XrSession}.
*
* Applications can avoid unnecessarily calling {@link #xrGetSceneMeshBuffersMSFT GetSceneMeshBuffersMSFT} for a scene component if {@link XrSceneComponentMSFT}{@code ::updateTime} is equal to the {@link XrSceneComponentMSFT}{@code ::updateTime} value in the previous {@code XrSceneMSFT}. A scene component is uniquely identified by {@link XrUuidMSFT}.
*
*
* This function follows the two-call idiom for filling multiple buffers in a struct.
*
* The {@link #xrGetSceneMeshBuffersMSFT GetSceneMeshBuffersMSFT} function is defined as:
*
*
* XrResult xrGetSceneMeshBuffersMSFT(
* XrSceneMSFT scene,
* const XrSceneMeshBuffersGetInfoMSFT* getInfo,
* XrSceneMeshBuffersMSFT* buffers);
*
* Description
*
* Applications can request the vertex buffer of the mesh by including {@link XrSceneMeshVertexBufferMSFT} in the {@link XrSceneMeshBuffersMSFT}{@code ::next} chain. Runtimes must support requesting a 32-bit index buffer and may support requesting a 16-bit index buffer. Applications can request a 32-bit index buffer by including {@link XrSceneMeshIndicesUint32MSFT} in the {@link XrSceneMeshBuffersMSFT}{@code ::next} chain. Applications can request a 16-bit index buffer by including {@link XrSceneMeshIndicesUint16MSFT} in the {@link XrSceneMeshBuffersMSFT}{@code ::next} chain. If the runtime for the given scene mesh buffer does not support requesting a 16-bit index buffer then {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} must be returned. The runtime must support reading a 16-bit index buffer for the given scene mesh buffer if {@link XrScenePlaneMSFT}:supportsIndicesUint16 or {@link XrSceneMeshMSFT}:supportsIndicesUint16 are {@link XR10#XR_TRUE TRUE} for the scene component that contained that scene mesh buffer identifier.
*
* The runtime must return {@link #XR_ERROR_SCENE_MESH_BUFFER_ID_INVALID_MSFT ERROR_SCENE_MESH_BUFFER_ID_INVALID_MSFT} if none of the scene components in the given {@code XrSceneMSFT} contain {@link XrSceneMeshBuffersGetInfoMSFT}{@code ::meshBufferId}. The runtime must return {@link #XR_ERROR_SCENE_MESH_BUFFER_ID_INVALID_MSFT ERROR_SCENE_MESH_BUFFER_ID_INVALID_MSFT} if {@link XrSceneMeshBuffersGetInfoMSFT}{@code ::meshBufferId} is zero. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if both {@link XrSceneMeshIndicesUint32MSFT} and {@link XrSceneMeshIndicesUint16MSFT} are included in the {@link XrSceneMeshBuffersMSFT}{@code ::next} chain. The runtime must return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE} if the {@link XrSceneMeshBuffersMSFT}{@code ::next} does not contain at least one of {@link XrSceneMeshVertexBufferMSFT}, {@link XrSceneMeshIndicesUint32MSFT} or {@link XrSceneMeshIndicesUint16MSFT}.
*
* The runtime must return the same vertices and indices for a given scene mesh buffer identifier and {@code XrSession}. A runtime may return zero vertices and indices if the underlying mesh data is no longer available.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneUnderstanding XR_MSFT_scene_understanding} extension must be enabled prior to calling {@link #xrGetSceneMeshBuffersMSFT GetSceneMeshBuffersMSFT}
* - {@code scene} must be a valid {@code XrSceneMSFT} handle
* - {@code getInfo} must be a pointer to a valid {@link XrSceneMeshBuffersGetInfoMSFT} structure
* - {@code buffers} must be a pointer to an {@link XrSceneMeshBuffersMSFT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link #XR_ERROR_SCENE_MESH_BUFFER_ID_INVALID_MSFT ERROR_SCENE_MESH_BUFFER_ID_INVALID_MSFT}
* - {@link #XR_ERROR_SCENE_COMPONENT_ID_INVALID_MSFT ERROR_SCENE_COMPONENT_ID_INVALID_MSFT}
*
*
*
* See Also
*
* {@link XrSceneMeshBuffersGetInfoMSFT}, {@link XrSceneMeshBuffersMSFT}
*
* @param scene an {@code XrSceneMSFT} previously created by {@link #xrCreateSceneMSFT CreateSceneMSFT}.
* @param getInfo a pointer to an {@link XrSceneMeshBuffersGetInfoMSFT} structure.
* @param buffers a pointer to an {@link XrSceneMeshBuffersMSFT} structure for reading a scene mesh buffer.
*/
@NativeType("XrResult")
public static int xrGetSceneMeshBuffersMSFT(XrSceneMSFT scene, @NativeType("XrSceneMeshBuffersGetInfoMSFT const *") XrSceneMeshBuffersGetInfoMSFT getInfo, @NativeType("XrSceneMeshBuffersMSFT *") XrSceneMeshBuffersMSFT buffers) {
return nxrGetSceneMeshBuffersMSFT(scene, getInfo.address(), buffers.address());
}
}