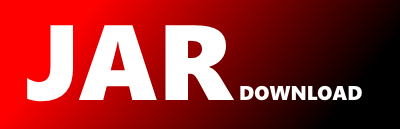
org.lwjgl.openxr.MSFTSpatialAnchor Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_MSFT_spatial_anchor extension.
*
* This extension allows an application to create a spatial anchor, an arbitrary freespace point in the user’s physical environment that will then be tracked by the runtime. The runtime should then adjust the position and orientation of that anchor’s origin over time as needed, independently of all other spaces and anchors, to ensure that it maintains its original mapping to the real world.
*/
public class MSFTSpatialAnchor {
/** The extension specification version. */
public static final int XR_MSFT_spatial_anchor_SPEC_VERSION = 2;
/** The extension name. */
public static final String XR_MSFT_SPATIAL_ANCHOR_EXTENSION_NAME = "XR_MSFT_spatial_anchor";
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SPATIAL_ANCHOR_CREATE_INFO_MSFT TYPE_SPATIAL_ANCHOR_CREATE_INFO_MSFT}
* - {@link #XR_TYPE_SPATIAL_ANCHOR_SPACE_CREATE_INFO_MSFT TYPE_SPATIAL_ANCHOR_SPACE_CREATE_INFO_MSFT}
*
*/
public static final int
XR_TYPE_SPATIAL_ANCHOR_CREATE_INFO_MSFT = 1000039000,
XR_TYPE_SPATIAL_ANCHOR_SPACE_CREATE_INFO_MSFT = 1000039001;
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_SPATIAL_ANCHOR_MSFT = 1000039000;
/** Extends {@code XrResult}. */
public static final int XR_ERROR_CREATE_SPATIAL_ANCHOR_FAILED_MSFT = -1000039001;
protected MSFTSpatialAnchor() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateSpatialAnchorMSFT ] ---
/** Unsafe version of: {@link #xrCreateSpatialAnchorMSFT CreateSpatialAnchorMSFT} */
public static int nxrCreateSpatialAnchorMSFT(XrSession session, long createInfo, long anchor) {
long __functionAddress = session.getCapabilities().xrCreateSpatialAnchorMSFT;
if (CHECKS) {
check(__functionAddress);
XrSpatialAnchorCreateInfoMSFT.validate(createInfo);
}
return callPPPI(session.address(), createInfo, anchor, __functionAddress);
}
/**
* Creates a spatial anchor.
*
* C Specification
*
* The {@link #xrCreateSpatialAnchorMSFT CreateSpatialAnchorMSFT} function is defined as:
*
*
* XrResult xrCreateSpatialAnchorMSFT(
* XrSession session,
* const XrSpatialAnchorCreateInfoMSFT* createInfo,
* XrSpatialAnchorMSFT* anchor);
*
* Description
*
* Creates an {@code XrSpatialAnchorMSFT} handle representing a spatial anchor that will track a fixed location in the physical world over time. That real-world location is specified by the position and orientation of the specified {@link XrSpatialAnchorCreateInfoMSFT}{@code ::pose} within {@link XrSpatialAnchorCreateInfoMSFT}{@code ::space} at {@link XrSpatialAnchorCreateInfoMSFT}{@code ::time}.
*
* The runtime must avoid long blocking operations such as networking or disk operations for {@link #xrCreateSpatialAnchorMSFT CreateSpatialAnchorMSFT} function. The application may safely use this function in UI thread. Though, the created anchor handle may not be ready immediately for certain operations yet. For example, the corresponding anchor space may not return valid location, or its location may not be successfully saved in anchor store.
*
* If {@link XrSpatialAnchorCreateInfoMSFT}{@code ::space} cannot be located relative to the environment at the moment of the call to {@link #xrCreateSpatialAnchorMSFT CreateSpatialAnchorMSFT}, the runtime must return {@link #XR_ERROR_CREATE_SPATIAL_ANCHOR_FAILED_MSFT ERROR_CREATE_SPATIAL_ANCHOR_FAILED_MSFT}.
*
* After the anchor is created, the runtime should then adjust its position and orientation over time relative to other spaces so as to maintain maximum alignment to its original real-world location, even if that changes the anchor’s relationship to the original {@link XrSpatialAnchorCreateInfoMSFT}{@code ::space} used to initialize it.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSpatialAnchor XR_MSFT_spatial_anchor} extension must be enabled prior to calling {@link #xrCreateSpatialAnchorMSFT CreateSpatialAnchorMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrSpatialAnchorCreateInfoMSFT} structure
* - {@code anchor} must be a pointer to an {@code XrSpatialAnchorMSFT} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_TIME_INVALID ERROR_TIME_INVALID}
* - {@link XR10#XR_ERROR_POSE_INVALID ERROR_POSE_INVALID}
* - {@link #XR_ERROR_CREATE_SPATIAL_ANCHOR_FAILED_MSFT ERROR_CREATE_SPATIAL_ANCHOR_FAILED_MSFT}
*
*
*
* See Also
*
* {@link XrSpatialAnchorCreateInfoMSFT}
*
* @param session a handle to an {@code XrSession}.
* @param createInfo a pointer to an {@link XrSpatialAnchorCreateInfoMSFT} structure containing information about how to create the anchor.
* @param anchor a pointer to a handle in which the created {@code XrSpatialAnchorMSFT} is returned.
*/
@NativeType("XrResult")
public static int xrCreateSpatialAnchorMSFT(XrSession session, @NativeType("XrSpatialAnchorCreateInfoMSFT const *") XrSpatialAnchorCreateInfoMSFT createInfo, @NativeType("XrSpatialAnchorMSFT *") PointerBuffer anchor) {
if (CHECKS) {
check(anchor, 1);
}
return nxrCreateSpatialAnchorMSFT(session, createInfo.address(), memAddress(anchor));
}
// --- [ xrCreateSpatialAnchorSpaceMSFT ] ---
/** Unsafe version of: {@link #xrCreateSpatialAnchorSpaceMSFT CreateSpatialAnchorSpaceMSFT} */
public static int nxrCreateSpatialAnchorSpaceMSFT(XrSession session, long createInfo, long space) {
long __functionAddress = session.getCapabilities().xrCreateSpatialAnchorSpaceMSFT;
if (CHECKS) {
check(__functionAddress);
XrSpatialAnchorSpaceCreateInfoMSFT.validate(createInfo);
}
return callPPPI(session.address(), createInfo, space, __functionAddress);
}
/**
* Creates a space from a spatial anchor.
*
* C Specification
*
* The {@link #xrCreateSpatialAnchorSpaceMSFT CreateSpatialAnchorSpaceMSFT} function is defined as:
*
*
* XrResult xrCreateSpatialAnchorSpaceMSFT(
* XrSession session,
* const XrSpatialAnchorSpaceCreateInfoMSFT* createInfo,
* XrSpace* space);
*
* Description
*
* Creates an {@code XrSpace} handle based on a spatial anchor. Application can provide an {@link XrPosef} to define the position and orientation of the new space’s origin relative to the anchor’s natural origin.
*
* Multiple {@code XrSpace} handles may exist for a given {@code XrSpatialAnchorMSFT} simultaneously, up to some limit imposed by the runtime. The {@code XrSpace} handle must be eventually freed via the {@link XR10#xrDestroySpace DestroySpace} function or by destroying the parent {@code XrSpatialAnchorMSFT} handle.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSpatialAnchor XR_MSFT_spatial_anchor} extension must be enabled prior to calling {@link #xrCreateSpatialAnchorSpaceMSFT CreateSpatialAnchorSpaceMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code createInfo} must be a pointer to a valid {@link XrSpatialAnchorSpaceCreateInfoMSFT} structure
* - {@code space} must be a pointer to an {@code XrSpace} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link XR10#XR_ERROR_POSE_INVALID ERROR_POSE_INVALID}
*
*
*
* See Also
*
* {@link XrSpatialAnchorSpaceCreateInfoMSFT}
*
* @param session a handle to an {@code XrSession}.
* @param createInfo a pointer to an {@link XrSpatialAnchorSpaceCreateInfoMSFT} structure containing information about how to create the anchor.
* @param space a pointer to a handle in which the created {@code XrSpace} is returned.
*/
@NativeType("XrResult")
public static int xrCreateSpatialAnchorSpaceMSFT(XrSession session, @NativeType("XrSpatialAnchorSpaceCreateInfoMSFT const *") XrSpatialAnchorSpaceCreateInfoMSFT createInfo, @NativeType("XrSpace *") PointerBuffer space) {
if (CHECKS) {
check(space, 1);
}
return nxrCreateSpatialAnchorSpaceMSFT(session, createInfo.address(), memAddress(space));
}
// --- [ xrDestroySpatialAnchorMSFT ] ---
/**
* Destroys a spatial anchor.
*
* C Specification
*
* The {@link #xrDestroySpatialAnchorMSFT DestroySpatialAnchorMSFT} function is defined as:
*
*
* XrResult xrDestroySpatialAnchorMSFT(
* XrSpatialAnchorMSFT anchor);
*
* Description
*
* {@code XrSpatialAnchorMSFT} handles are destroyed using {@link #xrDestroySpatialAnchorMSFT DestroySpatialAnchorMSFT}. By destroying an anchor, the runtime can stop spending resources used to maintain tracking for that anchor’s origin.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSpatialAnchor XR_MSFT_spatial_anchor} extension must be enabled prior to calling {@link #xrDestroySpatialAnchorMSFT DestroySpatialAnchorMSFT}
* - {@code anchor} must be a valid {@code XrSpatialAnchorMSFT} handle
*
*
* Thread Safety
*
*
* - Access to {@code anchor}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
*
*
*
* See Also
*
* {@link #xrCreateSpatialAnchorMSFT CreateSpatialAnchorMSFT}
*
* @param anchor a handle to an {@code XrSpatialAnchorMSFT} previously created by {@link #xrCreateSpatialAnchorMSFT CreateSpatialAnchorMSFT}.
*/
@NativeType("XrResult")
public static int xrDestroySpatialAnchorMSFT(XrSpatialAnchorMSFT anchor) {
long __functionAddress = anchor.getCapabilities().xrDestroySpatialAnchorMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPI(anchor.address(), __functionAddress);
}
}