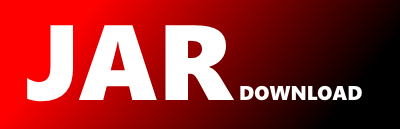
org.lwjgl.openxr.MSFTSpatialAnchorPersistence Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_MSFT_spatial_anchor_persistence extension.
*
* This extension allows persistence and retrieval of spatial anchors sharing and localization across application sessions on a device. Spatial anchors persisted during an application session on a device will only be able to be retrieved during sessions of that same application on the same device. This extension requires {@link MSFTSpatialAnchor XR_MSFT_spatial_anchor} to also be enabled.
*/
public class MSFTSpatialAnchorPersistence {
/** The extension specification version. */
public static final int XR_MSFT_spatial_anchor_persistence_SPEC_VERSION = 2;
/** The extension name. */
public static final String XR_MSFT_SPATIAL_ANCHOR_PERSISTENCE_EXTENSION_NAME = "XR_MSFT_spatial_anchor_persistence";
/** Extends {@code XrObjectType}. */
public static final int XR_OBJECT_TYPE_SPATIAL_ANCHOR_STORE_CONNECTION_MSFT = 1000142000;
/**
* Extends {@code XrStructureType}.
*
* Enum values:
*
*
* - {@link #XR_TYPE_SPATIAL_ANCHOR_PERSISTENCE_INFO_MSFT TYPE_SPATIAL_ANCHOR_PERSISTENCE_INFO_MSFT}
* - {@link #XR_TYPE_SPATIAL_ANCHOR_FROM_PERSISTED_ANCHOR_CREATE_INFO_MSFT TYPE_SPATIAL_ANCHOR_FROM_PERSISTED_ANCHOR_CREATE_INFO_MSFT}
*
*/
public static final int
XR_TYPE_SPATIAL_ANCHOR_PERSISTENCE_INFO_MSFT = 1000142000,
XR_TYPE_SPATIAL_ANCHOR_FROM_PERSISTED_ANCHOR_CREATE_INFO_MSFT = 1000142001;
/**
* Extends {@code XrResult}.
*
* Enum values:
*
*
* - {@link #XR_ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT}
* - {@link #XR_ERROR_SPATIAL_ANCHOR_NAME_INVALID_MSFT ERROR_SPATIAL_ANCHOR_NAME_INVALID_MSFT}
*
*/
public static final int
XR_ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT = -1000142001,
XR_ERROR_SPATIAL_ANCHOR_NAME_INVALID_MSFT = -1000142002;
/** XR_MAX_SPATIAL_ANCHOR_NAME_SIZE_MSFT */
public static final int XR_MAX_SPATIAL_ANCHOR_NAME_SIZE_MSFT = 256;
protected MSFTSpatialAnchorPersistence() {
throw new UnsupportedOperationException();
}
// --- [ xrCreateSpatialAnchorStoreConnectionMSFT ] ---
/** Unsafe version of: {@link #xrCreateSpatialAnchorStoreConnectionMSFT CreateSpatialAnchorStoreConnectionMSFT} */
public static int nxrCreateSpatialAnchorStoreConnectionMSFT(XrSession session, long spatialAnchorStore) {
long __functionAddress = session.getCapabilities().xrCreateSpatialAnchorStoreConnectionMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(session.address(), spatialAnchorStore, __functionAddress);
}
/**
* Create a handle to track a connection to the spatial anchor store.
*
* C Specification
*
* The application can use the {@link #xrCreateSpatialAnchorStoreConnectionMSFT CreateSpatialAnchorStoreConnectionMSFT} function to create an handle to the spatial anchor store. The application can use this handle to interact with the spatial anchor store in order to persist anchors across application sessions.
*
* The {@link #xrCreateSpatialAnchorStoreConnectionMSFT CreateSpatialAnchorStoreConnectionMSFT} function may be a slow operation and therefore should be invoked from a non-timing critical thread.
*
*
* XrResult xrCreateSpatialAnchorStoreConnectionMSFT(
* XrSession session,
* XrSpatialAnchorStoreConnectionMSFT* spatialAnchorStore);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSpatialAnchorPersistence XR_MSFT_spatial_anchor_persistence} extension must be enabled prior to calling {@link #xrCreateSpatialAnchorStoreConnectionMSFT CreateSpatialAnchorStoreConnectionMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code spatialAnchorStore} must be a pointer to an {@code XrSpatialAnchorStoreConnectionMSFT} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
*
*
*
* @param session the {@code XrSession} the anchor was created with.
* @param spatialAnchorStore a pointer to the {@code XrSpatialAnchorStoreConnectionMSFT} handle.
*/
@NativeType("XrResult")
public static int xrCreateSpatialAnchorStoreConnectionMSFT(XrSession session, @NativeType("XrSpatialAnchorStoreConnectionMSFT *") PointerBuffer spatialAnchorStore) {
if (CHECKS) {
check(spatialAnchorStore, 1);
}
return nxrCreateSpatialAnchorStoreConnectionMSFT(session, memAddress(spatialAnchorStore));
}
// --- [ xrDestroySpatialAnchorStoreConnectionMSFT ] ---
/**
* Destroys the anchor store handle.
*
* C Specification
*
* The application can use the {@link #xrDestroySpatialAnchorStoreConnectionMSFT DestroySpatialAnchorStoreConnectionMSFT} function to destroy an anchor store connection.
*
*
* XrResult xrDestroySpatialAnchorStoreConnectionMSFT(
* XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSpatialAnchorPersistence XR_MSFT_spatial_anchor_persistence} extension must be enabled prior to calling {@link #xrDestroySpatialAnchorStoreConnectionMSFT DestroySpatialAnchorStoreConnectionMSFT}
* - {@code spatialAnchorStore} must be a valid {@code XrSpatialAnchorStoreConnectionMSFT} handle
*
*
* Thread Safety
*
*
* - Access to {@code spatialAnchorStore}, and any child handles, must be externally synchronized
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
*
*
*
* @param spatialAnchorStore the {@code XrSpatialAnchorStoreConnectionMSFT} to be destroyed.
*/
@NativeType("XrResult")
public static int xrDestroySpatialAnchorStoreConnectionMSFT(XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore) {
long __functionAddress = spatialAnchorStore.getCapabilities().xrDestroySpatialAnchorStoreConnectionMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPI(spatialAnchorStore.address(), __functionAddress);
}
// --- [ xrPersistSpatialAnchorMSFT ] ---
/** Unsafe version of: {@link #xrPersistSpatialAnchorMSFT PersistSpatialAnchorMSFT} */
public static int nxrPersistSpatialAnchorMSFT(XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore, long spatialAnchorPersistenceInfo) {
long __functionAddress = spatialAnchorStore.getCapabilities().xrPersistSpatialAnchorMSFT;
if (CHECKS) {
check(__functionAddress);
XrSpatialAnchorPersistenceInfoMSFT.validate(spatialAnchorPersistenceInfo);
}
return callPPI(spatialAnchorStore.address(), spatialAnchorPersistenceInfo, __functionAddress);
}
/**
* Persist the spatial anchor in the spatial anchor store.
*
* C Specification
*
* The application can use the {@link #xrPersistSpatialAnchorMSFT PersistSpatialAnchorMSFT} function to persist a spatial anchor in the spatial anchor store for this application. The given {@link XrSpatialAnchorPersistenceInfoMSFT}{@code ::spatialAnchorPersistenceName} will be the string to retrieve the spatial anchor from the Spatial Anchor store or subsequently remove the record of this spatial anchor from the store. This name will uniquely identify the spatial anchor for the current application. If there is already a spatial anchor of the same name persisted in the spatial anchor store, the existing spatial anchor will be replaced and {@link #xrPersistSpatialAnchorMSFT PersistSpatialAnchorMSFT} must return {@link XR10#XR_SUCCESS SUCCESS}.
*
*
* XrResult xrPersistSpatialAnchorMSFT(
* XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore,
* const XrSpatialAnchorPersistenceInfoMSFT* spatialAnchorPersistenceInfo);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSpatialAnchorPersistence XR_MSFT_spatial_anchor_persistence} extension must be enabled prior to calling {@link #xrPersistSpatialAnchorMSFT PersistSpatialAnchorMSFT}
* - {@code spatialAnchorStore} must be a valid {@code XrSpatialAnchorStoreConnectionMSFT} handle
* - {@code spatialAnchorPersistenceInfo} must be a pointer to a valid {@link XrSpatialAnchorPersistenceInfoMSFT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link #XR_ERROR_SPATIAL_ANCHOR_NAME_INVALID_MSFT ERROR_SPATIAL_ANCHOR_NAME_INVALID_MSFT}
*
*
*
* See Also
*
* {@link XrSpatialAnchorPersistenceInfoMSFT}
*
* @param spatialAnchorStore the {@code XrSpatialAnchorStoreConnectionMSFT} with which to persist the {@link XrSpatialAnchorPersistenceInfoMSFT}{@code ::spatialAnchor}.
* @param spatialAnchorPersistenceInfo a pointer to {@link XrSpatialAnchorPersistenceInfoMSFT} structure to specify the anchor and its name to persist.
*/
@NativeType("XrResult")
public static int xrPersistSpatialAnchorMSFT(XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore, @NativeType("XrSpatialAnchorPersistenceInfoMSFT const *") XrSpatialAnchorPersistenceInfoMSFT spatialAnchorPersistenceInfo) {
return nxrPersistSpatialAnchorMSFT(spatialAnchorStore, spatialAnchorPersistenceInfo.address());
}
// --- [ xrEnumeratePersistedSpatialAnchorNamesMSFT ] ---
/**
* Unsafe version of: {@link #xrEnumeratePersistedSpatialAnchorNamesMSFT EnumeratePersistedSpatialAnchorNamesMSFT}
*
* @param spatialAnchorNameCapacityInput the capacity of the {@code spatialAnchorNames} array, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrEnumeratePersistedSpatialAnchorNamesMSFT(XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore, int spatialAnchorNameCapacityInput, long spatialAnchorNameCountOutput, long spatialAnchorNames) {
long __functionAddress = spatialAnchorStore.getCapabilities().xrEnumeratePersistedSpatialAnchorNamesMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(spatialAnchorStore.address(), spatialAnchorNameCapacityInput, spatialAnchorNameCountOutput, spatialAnchorNames, __functionAddress);
}
/**
* Enumerate the names of currently persisted spatial anchors in the spatial anchor store.
*
* C Specification
*
* The application can use the {@link #xrEnumeratePersistedSpatialAnchorNamesMSFT EnumeratePersistedSpatialAnchorNamesMSFT} function to enumerate the names of all spatial anchors currently persisted in the spatial anchor store for this application. This function follows the two-call idiom for filling the {@code spatialAnchorNames}.
*
*
* XrResult xrEnumeratePersistedSpatialAnchorNamesMSFT(
* XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore,
* uint32_t spatialAnchorNameCapacityInput,
* uint32_t* spatialAnchorNameCountOutput,
* XrSpatialAnchorPersistenceNameMSFT* spatialAnchorNames);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSpatialAnchorPersistence XR_MSFT_spatial_anchor_persistence} extension must be enabled prior to calling {@link #xrEnumeratePersistedSpatialAnchorNamesMSFT EnumeratePersistedSpatialAnchorNamesMSFT}
* - {@code spatialAnchorStore} must be a valid {@code XrSpatialAnchorStoreConnectionMSFT} handle
* - {@code spatialAnchorNameCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code spatialAnchorNameCapacityInput} is not 0, {@code spatialAnchorNames} must be a pointer to an array of {@code spatialAnchorNameCapacityInput} {@link XrSpatialAnchorPersistenceNameMSFT} structures
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
*
*
*
* See Also
*
* {@link XrSpatialAnchorPersistenceNameMSFT}
*
* @param spatialAnchorStore the {@code XrSpatialAnchorStoreConnectionMSFT} anchor store to perform the enumeration operation on.
* @param spatialAnchorNameCountOutput filled in by the runtime with the count of anchor names written or the required capacity in the case that {@code spatialAnchorNameCapacityInput} is insufficient.
* @param spatialAnchorNames a pointer to an array of {@link XrSpatialAnchorPersistenceNameMSFT} structures, but can be {@code NULL} if propertyCapacityInput is 0.
*/
@NativeType("XrResult")
public static int xrEnumeratePersistedSpatialAnchorNamesMSFT(XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore, @NativeType("uint32_t *") IntBuffer spatialAnchorNameCountOutput, @NativeType("XrSpatialAnchorPersistenceNameMSFT *") XrSpatialAnchorPersistenceNameMSFT.@Nullable Buffer spatialAnchorNames) {
if (CHECKS) {
check(spatialAnchorNameCountOutput, 1);
}
return nxrEnumeratePersistedSpatialAnchorNamesMSFT(spatialAnchorStore, remainingSafe(spatialAnchorNames), memAddress(spatialAnchorNameCountOutput), memAddressSafe(spatialAnchorNames));
}
// --- [ xrCreateSpatialAnchorFromPersistedNameMSFT ] ---
/** Unsafe version of: {@link #xrCreateSpatialAnchorFromPersistedNameMSFT CreateSpatialAnchorFromPersistedNameMSFT} */
public static int nxrCreateSpatialAnchorFromPersistedNameMSFT(XrSession session, long spatialAnchorCreateInfo, long spatialAnchor) {
long __functionAddress = session.getCapabilities().xrCreateSpatialAnchorFromPersistedNameMSFT;
if (CHECKS) {
check(__functionAddress);
XrSpatialAnchorFromPersistedAnchorCreateInfoMSFT.validate(spatialAnchorCreateInfo);
}
return callPPPI(session.address(), spatialAnchorCreateInfo, spatialAnchor, __functionAddress);
}
/**
* Create a spatial anchor from the spatial anchor store by name.
*
* C Specification
*
* The application can use the {@link #xrCreateSpatialAnchorFromPersistedNameMSFT CreateSpatialAnchorFromPersistedNameMSFT} function to create a {@code XrSpatialAnchorMSFT} from the spatial anchor store. If the {@link XrSpatialAnchorFromPersistedAnchorCreateInfoMSFT}{@code ::spatialAnchorPersistenceName} provided does not correspond to a currently stored anchor (i.e. the list of spatial anchor names returned from {@link #xrEnumeratePersistedSpatialAnchorNamesMSFT EnumeratePersistedSpatialAnchorNamesMSFT}), the function must return {@link #XR_ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT}.
*
*
* XrResult xrCreateSpatialAnchorFromPersistedNameMSFT(
* XrSession session,
* const XrSpatialAnchorFromPersistedAnchorCreateInfoMSFT* spatialAnchorCreateInfo,
* XrSpatialAnchorMSFT* spatialAnchor);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSpatialAnchorPersistence XR_MSFT_spatial_anchor_persistence} extension must be enabled prior to calling {@link #xrCreateSpatialAnchorFromPersistedNameMSFT CreateSpatialAnchorFromPersistedNameMSFT}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code spatialAnchorCreateInfo} must be a pointer to a valid {@link XrSpatialAnchorFromPersistedAnchorCreateInfoMSFT} structure
* - {@code spatialAnchor} must be a pointer to an {@code XrSpatialAnchorMSFT} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_LIMIT_REACHED ERROR_LIMIT_REACHED}
* - {@link #XR_ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT}
* - {@link #XR_ERROR_SPATIAL_ANCHOR_NAME_INVALID_MSFT ERROR_SPATIAL_ANCHOR_NAME_INVALID_MSFT}
*
*
*
* See Also
*
* {@link XrSpatialAnchorFromPersistedAnchorCreateInfoMSFT}
*
* @param session a handle to an {@code XrSession} previously created with {@link XR10#xrCreateSession CreateSession}.
* @param spatialAnchorCreateInfo a pointer to the {@link XrSpatialAnchorFromPersistedAnchorCreateInfoMSFT}.
* @param spatialAnchor a pointer to an {@code XrSpatialAnchorMSFT} handle that will be set by the runtime on successful load.
*/
@NativeType("XrResult")
public static int xrCreateSpatialAnchorFromPersistedNameMSFT(XrSession session, @NativeType("XrSpatialAnchorFromPersistedAnchorCreateInfoMSFT const *") XrSpatialAnchorFromPersistedAnchorCreateInfoMSFT spatialAnchorCreateInfo, @NativeType("XrSpatialAnchorMSFT *") PointerBuffer spatialAnchor) {
if (CHECKS) {
check(spatialAnchor, 1);
}
return nxrCreateSpatialAnchorFromPersistedNameMSFT(session, spatialAnchorCreateInfo.address(), memAddress(spatialAnchor));
}
// --- [ xrUnpersistSpatialAnchorMSFT ] ---
/** Unsafe version of: {@link #xrUnpersistSpatialAnchorMSFT UnpersistSpatialAnchorMSFT} */
public static int nxrUnpersistSpatialAnchorMSFT(XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore, long spatialAnchorPersistenceName) {
long __functionAddress = spatialAnchorStore.getCapabilities().xrUnpersistSpatialAnchorMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(spatialAnchorStore.address(), spatialAnchorPersistenceName, __functionAddress);
}
/**
* Removes an anchor from the store.
*
* C Specification
*
* The application can use the {@link #xrUnpersistSpatialAnchorMSFT UnpersistSpatialAnchorMSFT} function to remove the record of the anchor in the spatial anchor store. This operation will not affect any {@code XrSpatialAnchorMSFT} handles previously created. If the {@code spatialAnchorPersistenceName} provided does not correspond to a currently stored anchor, the function must return {@link #XR_ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT}.
*
*
* XrResult xrUnpersistSpatialAnchorMSFT(
* XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore,
* const XrSpatialAnchorPersistenceNameMSFT* spatialAnchorPersistenceName);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSpatialAnchorPersistence XR_MSFT_spatial_anchor_persistence} extension must be enabled prior to calling {@link #xrUnpersistSpatialAnchorMSFT UnpersistSpatialAnchorMSFT}
* - {@code spatialAnchorStore} must be a valid {@code XrSpatialAnchorStoreConnectionMSFT} handle
* - {@code spatialAnchorPersistenceName} must be a pointer to a valid {@link XrSpatialAnchorPersistenceNameMSFT} structure
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link #XR_ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT ERROR_SPATIAL_ANCHOR_NAME_NOT_FOUND_MSFT}
* - {@link #XR_ERROR_SPATIAL_ANCHOR_NAME_INVALID_MSFT ERROR_SPATIAL_ANCHOR_NAME_INVALID_MSFT}
*
*
*
* See Also
*
* {@link XrSpatialAnchorPersistenceNameMSFT}
*
* @param spatialAnchorStore an {@code XrSpatialAnchorStoreConnectionMSFT} anchor store to perform the unpersist operation on.
* @param spatialAnchorPersistenceName a pointer to the {@link XrSpatialAnchorPersistenceNameMSFT}.
*/
@NativeType("XrResult")
public static int xrUnpersistSpatialAnchorMSFT(XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore, @NativeType("XrSpatialAnchorPersistenceNameMSFT const *") XrSpatialAnchorPersistenceNameMSFT spatialAnchorPersistenceName) {
return nxrUnpersistSpatialAnchorMSFT(spatialAnchorStore, spatialAnchorPersistenceName.address());
}
// --- [ xrClearSpatialAnchorStoreMSFT ] ---
/**
* Clear all spatial anchors from the spatial anchor store.
*
* C Specification
*
* The application can use the {@link #xrClearSpatialAnchorStoreMSFT ClearSpatialAnchorStoreMSFT} function to remove all spatial anchors from the spatial anchor store for this application. The function only removes the record of the spatial anchors in the store but does not affect any {@code XrSpatialAnchorMSFT} handles previously loaded in the current session.
*
*
* XrResult xrClearSpatialAnchorStoreMSFT(
* XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore);
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSpatialAnchorPersistence XR_MSFT_spatial_anchor_persistence} extension must be enabled prior to calling {@link #xrClearSpatialAnchorStoreMSFT ClearSpatialAnchorStoreMSFT}
* - {@code spatialAnchorStore} must be a valid {@code XrSpatialAnchorStoreConnectionMSFT} handle
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
*
*
*
* @param spatialAnchorStore {@code XrSpatialAnchorStoreConnectionMSFT} to perform the clear operation on.
*/
@NativeType("XrResult")
public static int xrClearSpatialAnchorStoreMSFT(XrSpatialAnchorStoreConnectionMSFT spatialAnchorStore) {
long __functionAddress = spatialAnchorStore.getCapabilities().xrClearSpatialAnchorStoreMSFT;
if (CHECKS) {
check(__functionAddress);
}
return callPI(spatialAnchorStore.address(), __functionAddress);
}
}