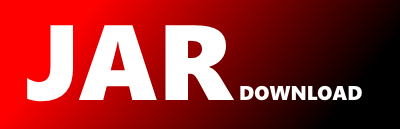
org.lwjgl.openxr.OCULUSAudioDeviceGuid Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwjgl-openxr Show documentation
Show all versions of lwjgl-openxr Show documentation
A royalty-free, open standard that provides high-performance access to Augmented Reality (AR) and Virtual Reality (VR)—collectively known as XR—platforms and devices.
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_OCULUS_audio_device_guid extension.
*
* This extension enables the querying of audio device information associated with an OpenXR instance.
*
* On Windows, there may be multiple audio devices available on the system. This extensions allows applications to query the runtime for the appropriate audio devices for the active HMD.
*/
public class OCULUSAudioDeviceGuid {
/** The extension specification version. */
public static final int XR_OCULUS_audio_device_guid_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_OCULUS_AUDIO_DEVICE_GUID_EXTENSION_NAME = "XR_OCULUS_audio_device_guid";
/** XR_MAX_AUDIO_DEVICE_STR_SIZE_OCULUS */
public static final int XR_MAX_AUDIO_DEVICE_STR_SIZE_OCULUS = 128;
protected OCULUSAudioDeviceGuid() {
throw new UnsupportedOperationException();
}
// --- [ xrGetAudioOutputDeviceGuidOculus ] ---
/** Unsafe version of: {@link #xrGetAudioOutputDeviceGuidOculus GetAudioOutputDeviceGuidOculus} */
public static int nxrGetAudioOutputDeviceGuidOculus(XrInstance instance, long buffer) {
long __functionAddress = instance.getCapabilities().xrGetAudioOutputDeviceGuidOculus;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(instance.address(), buffer, __functionAddress);
}
/**
* Query the GUID for the active audio output device.
*
* C Specification
*
*
* XrResult xrGetAudioOutputDeviceGuidOculus(
* XrInstance instance,
* wchar_t buffer[XR_MAX_AUDIO_DEVICE_STR_SIZE_OCULUS]);
*
* Valid Usage (Implicit)
*
*
* - The {@link OCULUSAudioDeviceGuid XR_OCULUS_audio_device_guid} extension must be enabled prior to calling {@link #xrGetAudioOutputDeviceGuidOculus GetAudioOutputDeviceGuidOculus}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code buffer} must be a wide character array of length {@link #XR_MAX_AUDIO_DEVICE_STR_SIZE_OCULUS MAX_AUDIO_DEVICE_STR_SIZE_OCULUS}
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link #xrGetAudioInputDeviceGuidOculus GetAudioInputDeviceGuidOculus}
*
* @param instance the {@code XrInstance} to query the audio device state in.
* @param buffer a fixed size buffer which will contain the audio device GUID. The format of this data matches the IMMDevice::GetId API.
*/
@NativeType("XrResult")
public static int xrGetAudioOutputDeviceGuidOculus(XrInstance instance, @NativeType("wchar_t *") ByteBuffer buffer) {
if (CHECKS) {
check(buffer, XR_MAX_AUDIO_DEVICE_STR_SIZE_OCULUS);
}
return nxrGetAudioOutputDeviceGuidOculus(instance, memAddress(buffer));
}
// --- [ xrGetAudioInputDeviceGuidOculus ] ---
/** Unsafe version of: {@link #xrGetAudioInputDeviceGuidOculus GetAudioInputDeviceGuidOculus} */
public static int nxrGetAudioInputDeviceGuidOculus(XrInstance instance, long buffer) {
long __functionAddress = instance.getCapabilities().xrGetAudioInputDeviceGuidOculus;
if (CHECKS) {
check(__functionAddress);
}
return callPPI(instance.address(), buffer, __functionAddress);
}
/**
* Query the GUID for the active audio input device.
*
* C Specification
*
*
* XrResult xrGetAudioInputDeviceGuidOculus(
* XrInstance instance,
* wchar_t buffer[XR_MAX_AUDIO_DEVICE_STR_SIZE_OCULUS]);
*
* Valid Usage (Implicit)
*
*
* - The {@link OCULUSAudioDeviceGuid XR_OCULUS_audio_device_guid} extension must be enabled prior to calling {@link #xrGetAudioInputDeviceGuidOculus GetAudioInputDeviceGuidOculus}
* - {@code instance} must be a valid {@code XrInstance} handle
* - {@code buffer} must be a wide character array of length {@link #XR_MAX_AUDIO_DEVICE_STR_SIZE_OCULUS MAX_AUDIO_DEVICE_STR_SIZE_OCULUS}
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_FEATURE_UNSUPPORTED ERROR_FEATURE_UNSUPPORTED}
*
*
*
* See Also
*
* {@link #xrGetAudioOutputDeviceGuidOculus GetAudioOutputDeviceGuidOculus}
*
* @param instance the {@code XrInstance} to query the audio device state in.
* @param buffer a fixed size buffer which will contain the audio device GUID. The format of this data matches the IMMDevice::GetId API.
*/
@NativeType("XrResult")
public static int xrGetAudioInputDeviceGuidOculus(XrInstance instance, @NativeType("wchar_t *") ByteBuffer buffer) {
if (CHECKS) {
check(buffer, XR_MAX_AUDIO_DEVICE_STR_SIZE_OCULUS);
}
return nxrGetAudioInputDeviceGuidOculus(instance, memAddress(buffer));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy