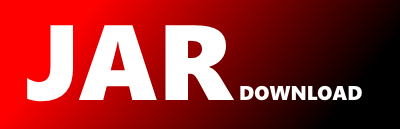
org.lwjgl.openxr.OCULUSExternalCamera Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwjgl-openxr Show documentation
Show all versions of lwjgl-openxr Show documentation
A royalty-free, open standard that provides high-performance access to Augmented Reality (AR) and Virtual Reality (VR)—collectively known as XR—platforms and devices.
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The XR_OCULUS_external_camera extension.
*
* This extension enables the querying of external camera information for a session. This extension is intended to enable mixed reality capture support for applications.
*
* This extension does not provide a mechanism for supplying external camera information to the runtime. If external camera information is not supplied to the runtime before using this extension, no camera information will be returned.
*
* This API supports returning camera intrinsics and extrinsics:
*
*
* - Camera intrinsics are the attributes of the camera: resolution, field of view, etc.
* - Camera extrinsics are everything external to the camera: relative pose, attached to, etc.
* - We do not expect the camera intrinsics to change frequently. We expect the camera extrinsics to change frequently.
*
*/
public class OCULUSExternalCamera {
/** The extension specification version. */
public static final int XR_OCULUS_external_camera_SPEC_VERSION = 1;
/** The extension name. */
public static final String XR_OCULUS_EXTERNAL_CAMERA_EXTENSION_NAME = "XR_OCULUS_external_camera";
/** Extends {@code XrStructureType}. */
public static final int XR_TYPE_EXTERNAL_CAMERA_OCULUS = 1000226000;
/** XR_MAX_EXTERNAL_CAMERA_NAME_SIZE_OCULUS */
public static final int XR_MAX_EXTERNAL_CAMERA_NAME_SIZE_OCULUS = 32;
/**
* XrExternalCameraAttachedToDeviceOCULUS - XrExternalCameraAttachedToDeviceOCULUS
*
* Description
*
*
* Enum Description
*
* {@link #XR_EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_NONE_OCULUS EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_NONE_OCULUS} External camera is at a fixed point in LOCAL space
* {@link #XR_EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_HMD_OCULUS EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_HMD_OCULUS} External camera is attached to the HMD
* {@link #XR_EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_LTOUCH_OCULUS EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_LTOUCH_OCULUS} External camera is attached to a left Touch controller
* {@link #XR_EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_RTOUCH_OCULUS EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_RTOUCH_OCULUS} External camera is attached to a right Touch controller
*
*
*
* See Also
*
* {@link XrExternalCameraExtrinsicsOCULUS}
*/
public static final int
XR_EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_NONE_OCULUS = 0,
XR_EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_HMD_OCULUS = 1,
XR_EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_LTOUCH_OCULUS = 2,
XR_EXTERNAL_CAMERA_ATTACHED_TO_DEVICE_RTOUCH_OCULUS = 3;
/**
* XrExternalCameraStatusFlagBitsOCULUS - XrExternalCameraStatusFlagBitsOCULUS
*
* Flag Descriptions
*
*
* - {@link #XR_EXTERNAL_CAMERA_STATUS_CONNECTED_BIT_OCULUS EXTERNAL_CAMERA_STATUS_CONNECTED_BIT_OCULUS} — External camera is connected
* - {@link #XR_EXTERNAL_CAMERA_STATUS_CALIBRATING_BIT_OCULUS EXTERNAL_CAMERA_STATUS_CALIBRATING_BIT_OCULUS} — External camera is undergoing calibration
* - {@link #XR_EXTERNAL_CAMERA_STATUS_CALIBRATION_FAILED_BIT_OCULUS EXTERNAL_CAMERA_STATUS_CALIBRATION_FAILED_BIT_OCULUS} — External camera has tried and failed calibration
* - {@link #XR_EXTERNAL_CAMERA_STATUS_CALIBRATED_BIT_OCULUS EXTERNAL_CAMERA_STATUS_CALIBRATED_BIT_OCULUS} — External camera has tried and passed calibration
* - {@link #XR_EXTERNAL_CAMERA_STATUS_CAPTURING_BIT_OCULUS EXTERNAL_CAMERA_STATUS_CAPTURING_BIT_OCULUS} — External camera is capturing
*
*/
public static final int
XR_EXTERNAL_CAMERA_STATUS_CONNECTED_BIT_OCULUS = 0x1,
XR_EXTERNAL_CAMERA_STATUS_CALIBRATING_BIT_OCULUS = 0x2,
XR_EXTERNAL_CAMERA_STATUS_CALIBRATION_FAILED_BIT_OCULUS = 0x4,
XR_EXTERNAL_CAMERA_STATUS_CALIBRATED_BIT_OCULUS = 0x8,
XR_EXTERNAL_CAMERA_STATUS_CAPTURING_BIT_OCULUS = 0x10;
protected OCULUSExternalCamera() {
throw new UnsupportedOperationException();
}
// --- [ xrEnumerateExternalCamerasOCULUS ] ---
/**
* Unsafe version of: {@link #xrEnumerateExternalCamerasOCULUS EnumerateExternalCamerasOCULUS}
*
* @param cameraCapacityInput the capacity of the {@code cameras} array, or 0 to indicate a request to retrieve the required capacity.
*/
public static int nxrEnumerateExternalCamerasOCULUS(XrSession session, int cameraCapacityInput, long cameraCountOutput, long cameras) {
long __functionAddress = session.getCapabilities().xrEnumerateExternalCamerasOCULUS;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(session.address(), cameraCapacityInput, cameraCountOutput, cameras, __functionAddress);
}
/**
* Enumerates all the external cameras that are supported by the runtime.
*
* C Specification
*
* The {@link #xrEnumerateExternalCamerasOCULUS EnumerateExternalCamerasOCULUS} function enumerates all the external cameras that are supported by the runtime, it is defined as:
*
*
* XrResult xrEnumerateExternalCamerasOCULUS(
* XrSession session,
* uint32_t cameraCapacityInput,
* uint32_t* cameraCountOutput,
* XrExternalCameraOCULUS* cameras);
*
* Valid Usage (Implicit)
*
*
* - The {@link OCULUSExternalCamera XR_OCULUS_external_camera} extension must be enabled prior to calling {@link #xrEnumerateExternalCamerasOCULUS EnumerateExternalCamerasOCULUS}
* - {@code session} must be a valid {@code XrSession} handle
* - {@code cameraCountOutput} must be a pointer to a {@code uint32_t} value
* - If {@code cameraCapacityInput} is not 0, {@code cameras} must be a pointer to an array of {@code cameraCapacityInput} {@link XrExternalCameraOCULUS} structures
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link XR10#XR_SUCCESS SUCCESS}
* - {@link XR10#XR_SESSION_LOSS_PENDING SESSION_LOSS_PENDING}
*
* - On failure, this command returns
*
* - {@link XR10#XR_ERROR_FUNCTION_UNSUPPORTED ERROR_FUNCTION_UNSUPPORTED}
* - {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}
* - {@link XR10#XR_ERROR_RUNTIME_FAILURE ERROR_RUNTIME_FAILURE}
* - {@link XR10#XR_ERROR_HANDLE_INVALID ERROR_HANDLE_INVALID}
* - {@link XR10#XR_ERROR_INSTANCE_LOST ERROR_INSTANCE_LOST}
* - {@link XR10#XR_ERROR_SESSION_LOST ERROR_SESSION_LOST}
* - {@link XR10#XR_ERROR_OUT_OF_MEMORY ERROR_OUT_OF_MEMORY}
* - {@link XR10#XR_ERROR_SIZE_INSUFFICIENT ERROR_SIZE_INSUFFICIENT}
*
*
*
* See Also
*
* {@link XrExternalCameraOCULUS}
*
* @param session the {@code XrSession} to query the external cameras in
* @param cameraCountOutput filled in by the runtime with the count of {@code cameras} written or the required capacity in the case that {@code cameraCapacityInput} is insufficient.
* @param cameras an array of {@link XrExternalCameraOCULUS} filled in by the runtime which contains all the available external cameras, but can be {@code NULL} if {@code cameraCapacityInput} is 0.
*/
@NativeType("XrResult")
public static int xrEnumerateExternalCamerasOCULUS(XrSession session, @NativeType("uint32_t *") IntBuffer cameraCountOutput, @NativeType("XrExternalCameraOCULUS *") XrExternalCameraOCULUS.@Nullable Buffer cameras) {
if (CHECKS) {
check(cameraCountOutput, 1);
}
return nxrEnumerateExternalCamerasOCULUS(session, remainingSafe(cameras), memAddress(cameraCountOutput), memAddressSafe(cameras));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy