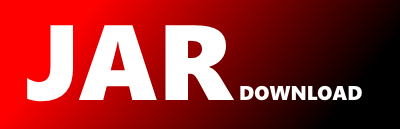
org.lwjgl.openxr.XrDebugUtilsMessengerCallbackEXT Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* Type of callback function invoked by the debug utils.
*
* C Specification
*
*
* typedef XrBool32 (XRAPI_PTR *PFN_xrDebugUtilsMessengerCallbackEXT)(
* XrDebugUtilsMessageSeverityFlagsEXT messageSeverity,
* XrDebugUtilsMessageTypeFlagsEXT messageTypes,
* const XrDebugUtilsMessengerCallbackDataEXT* callbackData,
* void* userData);
*
* Description
*
* The callback must not call {@link EXTDebugUtils#xrDestroyDebugUtilsMessengerEXT DestroyDebugUtilsMessengerEXT}.
*
* The callback returns an {@code XrBool32} that indicates to the calling layer the application’s desire to abort the call. A value of {@link XR10#XR_TRUE TRUE} indicates that the application wants to abort this call. If the application returns {@link XR10#XR_FALSE FALSE}, the function must not be aborted. Applications should always return {@link XR10#XR_FALSE FALSE} so that they see the same behavior with and without validation layers enabled.
*
* If the application returns {@link XR10#XR_TRUE TRUE} from its callback and the OpenXR call being aborted returns an {@code XrResult}, the layer will return {@link XR10#XR_ERROR_VALIDATION_FAILURE ERROR_VALIDATION_FAILURE}.
*
* The object pointed to by {@code callbackData} (and any pointers in it recursively) must be valid during the lifetime of the triggered callback. It may become invalid afterwards.
*
* See Also
*
* {@link XrDebugUtilsMessengerCreateInfoEXT}, {@link EXTDebugUtils#xrCreateDebugUtilsMessengerEXT CreateDebugUtilsMessengerEXT}
*
* Type
*
*
* XrBool32 (*{@link #invoke}) (
* XrDebugUtilsMessageSeverityFlagsEXT messageSeverity,
* XrDebugUtilsMessageTypeFlagsEXT messageTypes,
* XrDebugUtilsMessengerCallbackDataEXT const *callbackData,
* void *userData
* )
*/
public abstract class XrDebugUtilsMessengerCallbackEXT extends Callback implements XrDebugUtilsMessengerCallbackEXTI {
/**
* Creates a {@code XrDebugUtilsMessengerCallbackEXT} instance from the specified function pointer.
*
* @return the new {@code XrDebugUtilsMessengerCallbackEXT}
*/
public static XrDebugUtilsMessengerCallbackEXT create(long functionPointer) {
XrDebugUtilsMessengerCallbackEXTI instance = Callback.get(functionPointer);
return instance instanceof XrDebugUtilsMessengerCallbackEXT
? (XrDebugUtilsMessengerCallbackEXT)instance
: new Container(functionPointer, instance);
}
/** Like {@link #create(long) create}, but returns {@code null} if {@code functionPointer} is {@code NULL}. */
public static @Nullable XrDebugUtilsMessengerCallbackEXT createSafe(long functionPointer) {
return functionPointer == NULL ? null : create(functionPointer);
}
/** Creates a {@code XrDebugUtilsMessengerCallbackEXT} instance that delegates to the specified {@code XrDebugUtilsMessengerCallbackEXTI} instance. */
public static XrDebugUtilsMessengerCallbackEXT create(XrDebugUtilsMessengerCallbackEXTI instance) {
return instance instanceof XrDebugUtilsMessengerCallbackEXT
? (XrDebugUtilsMessengerCallbackEXT)instance
: new Container(instance.address(), instance);
}
protected XrDebugUtilsMessengerCallbackEXT() {
super(CIF);
}
XrDebugUtilsMessengerCallbackEXT(long functionPointer) {
super(functionPointer);
}
private static final class Container extends XrDebugUtilsMessengerCallbackEXT {
private final XrDebugUtilsMessengerCallbackEXTI delegate;
Container(long functionPointer, XrDebugUtilsMessengerCallbackEXTI delegate) {
super(functionPointer);
this.delegate = delegate;
}
@Override
public int invoke(long messageSeverity, long messageTypes, long callbackData, long userData) {
return delegate.invoke(messageSeverity, messageTypes, callbackData, userData);
}
}
}