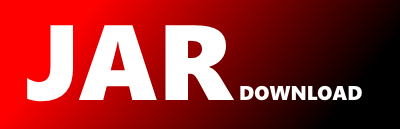
org.lwjgl.openxr.XrSceneMarkerMSFT Maven / Gradle / Ivy
Show all versions of lwjgl-openxr Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.openxr;
import org.jspecify.annotations.*;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* The properties of a scene marker.
*
* Description
*
* The {@link XrSceneMarkerMSFT} structure is an element in the array of {@link XrSceneMarkersMSFT}{@code ::sceneMarkers}.
*
* Refer to the QR code convention for an example of marker’s center and size in the context of a QR code.
*
* When the runtime updates the location or properties of an observed marker, the runtime must set the {@link XrSceneMarkerMSFT}{@code ::lastSeenTime} to the new timestamp of the update.
*
* When the runtime cannot observe a previously observed {@link XrSceneMarkerMSFT}, the runtime must keep the previous {@code lastSeenTime} for the marker. Hence, the application can use the {@code lastSeenTime} to know how fresh the tracking information is for a given marker.
*
* The {@code center} and {@code size} are measured in meters, relative to the {@link XrPosef} of the marker for the visual bound of the marker in XY plane, regardless of the marker type.
*
* Valid Usage (Implicit)
*
*
* - The {@link MSFTSceneMarker XR_MSFT_scene_marker} extension must be enabled prior to using {@link XrSceneMarkerMSFT}
*
*
* See Also
*
* {@link XrExtent2Df}, {@link XrOffset2Df}, {@link XrSceneMarkersMSFT}
*
* Layout
*
*
* struct XrSceneMarkerMSFT {
* XrSceneMarkerTypeMSFT {@link #markerType};
* XrTime {@link #lastSeenTime};
* {@link XrOffset2Df XrOffset2Df} {@link #center};
* {@link XrExtent2Df XrExtent2Df} {@link #size};
* }
*/
public class XrSceneMarkerMSFT extends Struct {
/** The struct size in bytes. */
public static final int SIZEOF;
/** The struct alignment in bytes. */
public static final int ALIGNOF;
/** The struct member offsets. */
public static final int
MARKERTYPE,
LASTSEENTIME,
CENTER,
SIZE;
static {
Layout layout = __struct(
__member(4),
__member(8),
__member(XrOffset2Df.SIZEOF, XrOffset2Df.ALIGNOF),
__member(XrExtent2Df.SIZEOF, XrExtent2Df.ALIGNOF)
);
SIZEOF = layout.getSize();
ALIGNOF = layout.getAlignment();
MARKERTYPE = layout.offsetof(0);
LASTSEENTIME = layout.offsetof(1);
CENTER = layout.offsetof(2);
SIZE = layout.offsetof(3);
}
protected XrSceneMarkerMSFT(long address, @Nullable ByteBuffer container) {
super(address, container);
}
@Override
protected XrSceneMarkerMSFT create(long address, @Nullable ByteBuffer container) {
return new XrSceneMarkerMSFT(address, container);
}
/**
* Creates a {@code XrSceneMarkerMSFT} instance at the current position of the specified {@link ByteBuffer} container. Changes to the buffer's content will be
* visible to the struct instance and vice versa.
*
* The created instance holds a strong reference to the container object.
*/
public XrSceneMarkerMSFT(ByteBuffer container) {
super(memAddress(container), __checkContainer(container, SIZEOF));
}
@Override
public int sizeof() { return SIZEOF; }
/** an {@code XrSceneMarkerTypeMSFT} indicating the type of the marker. */
@NativeType("XrSceneMarkerTypeMSFT")
public int markerType() { return nmarkerType(address()); }
/** an {@code XrTime} indicating when the marker was seen last. */
@NativeType("XrTime")
public long lastSeenTime() { return nlastSeenTime(address()); }
/** an {@link XrOffset2Df} structure representing the location of the center of the axis-aligned bounding box of the marker in the XY plane of the marker’s coordinate system. */
public XrOffset2Df center() { return ncenter(address()); }
/** an {@link XrExtent2Df} structure representing the width and height of the axis-aligned bounding box of the marker in the XY plane of the marker’s coordinate system. */
public XrExtent2Df size() { return nsize(address()); }
// -----------------------------------
/** Returns a new {@code XrSceneMarkerMSFT} instance for the specified memory address. */
public static XrSceneMarkerMSFT create(long address) {
return new XrSceneMarkerMSFT(address, null);
}
/** Like {@link #create(long) create}, but returns {@code null} if {@code address} is {@code NULL}. */
public static @Nullable XrSceneMarkerMSFT createSafe(long address) {
return address == NULL ? null : new XrSceneMarkerMSFT(address, null);
}
/**
* Create a {@link XrSceneMarkerMSFT.Buffer} instance at the specified memory.
*
* @param address the memory address
* @param capacity the buffer capacity
*/
public static XrSceneMarkerMSFT.Buffer create(long address, int capacity) {
return new Buffer(address, capacity);
}
/** Like {@link #create(long, int) create}, but returns {@code null} if {@code address} is {@code NULL}. */
public static XrSceneMarkerMSFT.@Nullable Buffer createSafe(long address, int capacity) {
return address == NULL ? null : new Buffer(address, capacity);
}
// -----------------------------------
/** Unsafe version of {@link #markerType}. */
public static int nmarkerType(long struct) { return memGetInt(struct + XrSceneMarkerMSFT.MARKERTYPE); }
/** Unsafe version of {@link #lastSeenTime}. */
public static long nlastSeenTime(long struct) { return memGetLong(struct + XrSceneMarkerMSFT.LASTSEENTIME); }
/** Unsafe version of {@link #center}. */
public static XrOffset2Df ncenter(long struct) { return XrOffset2Df.create(struct + XrSceneMarkerMSFT.CENTER); }
/** Unsafe version of {@link #size}. */
public static XrExtent2Df nsize(long struct) { return XrExtent2Df.create(struct + XrSceneMarkerMSFT.SIZE); }
// -----------------------------------
/** An array of {@link XrSceneMarkerMSFT} structs. */
public static class Buffer extends StructBuffer {
private static final XrSceneMarkerMSFT ELEMENT_FACTORY = XrSceneMarkerMSFT.create(-1L);
/**
* Creates a new {@code XrSceneMarkerMSFT.Buffer} instance backed by the specified container.
*
* Changes to the container's content will be visible to the struct buffer instance and vice versa. The two buffers' position, limit, and mark values
* will be independent. The new buffer's position will be zero, its capacity and its limit will be the number of bytes remaining in this buffer divided
* by {@link XrSceneMarkerMSFT#SIZEOF}, and its mark will be undefined.
*
* The created buffer instance holds a strong reference to the container object.
*/
public Buffer(ByteBuffer container) {
super(container, container.remaining() / SIZEOF);
}
public Buffer(long address, int cap) {
super(address, null, -1, 0, cap, cap);
}
Buffer(long address, @Nullable ByteBuffer container, int mark, int pos, int lim, int cap) {
super(address, container, mark, pos, lim, cap);
}
@Override
protected Buffer self() {
return this;
}
@Override
protected Buffer create(long address, @Nullable ByteBuffer container, int mark, int position, int limit, int capacity) {
return new Buffer(address, container, mark, position, limit, capacity);
}
@Override
protected XrSceneMarkerMSFT getElementFactory() {
return ELEMENT_FACTORY;
}
/** @return the value of the {@link XrSceneMarkerMSFT#markerType} field. */
@NativeType("XrSceneMarkerTypeMSFT")
public int markerType() { return XrSceneMarkerMSFT.nmarkerType(address()); }
/** @return the value of the {@link XrSceneMarkerMSFT#lastSeenTime} field. */
@NativeType("XrTime")
public long lastSeenTime() { return XrSceneMarkerMSFT.nlastSeenTime(address()); }
/** @return a {@link XrOffset2Df} view of the {@link XrSceneMarkerMSFT#center} field. */
public XrOffset2Df center() { return XrSceneMarkerMSFT.ncenter(address()); }
/** @return a {@link XrExtent2Df} view of the {@link XrSceneMarkerMSFT#size} field. */
public XrExtent2Df size() { return XrSceneMarkerMSFT.nsize(address()); }
}
}