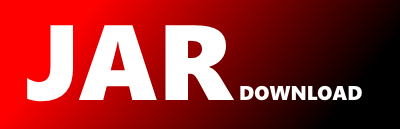
org.lwjgl.vulkan.EXTExtendedDynamicState2 Maven / Gradle / Ivy
Show all versions of lwjgl-vulkan Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.vulkan;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
/**
* This extension adds some more dynamic state to support applications that need to reduce the number of pipeline state objects they compile and bind.
*
* VK_EXT_extended_dynamic_state2
*
*
* - Name String
* - {@code VK_EXT_extended_dynamic_state2}
* - Extension Type
* - Device extension
* - Registered Extension Number
* - 378
* - Revision
* - 1
* - Extension and Version Dependencies
*
* - Requires Vulkan 1.0
* - Requires {@link KHRGetPhysicalDeviceProperties2 VK_KHR_get_physical_device_properties2}
*
* - Contact
*
* - Vikram Kushwaha vkushwaha-nv
*
*
*
* Other Extension Metadata
*
*
* - Last Modified Date
* - 2021-04-12
* - IP Status
* - No known IP claims.
* - Contributors
*
* - Vikram Kushwaha, NVIDIA
* - Piers Daniell, NVIDIA
* - Jeff Bolz, NVIDIA
*
*
*/
public class EXTExtendedDynamicState2 {
/** The extension specification version. */
public static final int VK_EXT_EXTENDED_DYNAMIC_STATE_2_SPEC_VERSION = 1;
/** The extension name. */
public static final String VK_EXT_EXTENDED_DYNAMIC_STATE_2_EXTENSION_NAME = "VK_EXT_extended_dynamic_state2";
/** Extends {@code VkStructureType}. */
public static final int VK_STRUCTURE_TYPE_PHYSICAL_DEVICE_EXTENDED_DYNAMIC_STATE_2_FEATURES_EXT = 1000377000;
/**
* Extends {@code VkDynamicState}.
*
* Enum values:
*
*
* - {@link #VK_DYNAMIC_STATE_PATCH_CONTROL_POINTS_EXT DYNAMIC_STATE_PATCH_CONTROL_POINTS_EXT}
* - {@link #VK_DYNAMIC_STATE_RASTERIZER_DISCARD_ENABLE_EXT DYNAMIC_STATE_RASTERIZER_DISCARD_ENABLE_EXT}
* - {@link #VK_DYNAMIC_STATE_DEPTH_BIAS_ENABLE_EXT DYNAMIC_STATE_DEPTH_BIAS_ENABLE_EXT}
* - {@link #VK_DYNAMIC_STATE_LOGIC_OP_EXT DYNAMIC_STATE_LOGIC_OP_EXT}
* - {@link #VK_DYNAMIC_STATE_PRIMITIVE_RESTART_ENABLE_EXT DYNAMIC_STATE_PRIMITIVE_RESTART_ENABLE_EXT}
*
*/
public static final int
VK_DYNAMIC_STATE_PATCH_CONTROL_POINTS_EXT = 1000377000,
VK_DYNAMIC_STATE_RASTERIZER_DISCARD_ENABLE_EXT = 1000377001,
VK_DYNAMIC_STATE_DEPTH_BIAS_ENABLE_EXT = 1000377002,
VK_DYNAMIC_STATE_LOGIC_OP_EXT = 1000377003,
VK_DYNAMIC_STATE_PRIMITIVE_RESTART_ENABLE_EXT = 1000377004;
protected EXTExtendedDynamicState2() {
throw new UnsupportedOperationException();
}
// --- [ vkCmdSetPatchControlPointsEXT ] ---
/**
* Specify the number of control points per patch dynamically for a command buffer.
*
* C Specification
*
* To dynamically set the number of control points per patch, call:
*
*
* void vkCmdSetPatchControlPointsEXT(
* VkCommandBuffer commandBuffer,
* uint32_t patchControlPoints);
*
* Description
*
* This command sets the number of control points per patch for subsequent drawing commands when the graphics pipeline is created with {@link #VK_DYNAMIC_STATE_PATCH_CONTROL_POINTS_EXT DYNAMIC_STATE_PATCH_CONTROL_POINTS_EXT} set in {@link VkPipelineDynamicStateCreateInfo}{@code ::pDynamicStates}. Otherwise, this state is specified by the {@link VkPipelineTessellationStateCreateInfo}{@code ::patchControlPoints} value used to create the currently active pipeline.
*
* Valid Usage
*
*
* - The extendedDynamicState2PatchControlPoints feature must be enabled
* - {@code patchControlPoints} must be greater than zero and less than or equal to {@link VkPhysicalDeviceLimits}{@code ::maxTessellationPatchSize}
*
*
* Valid Usage (Implicit)
*
*
* - {@code commandBuffer} must be a valid {@code VkCommandBuffer} handle
* - {@code commandBuffer} must be in the recording state
* - The {@code VkCommandPool} that {@code commandBuffer} was allocated from must support graphics operations
*
*
* Host Synchronization
*
*
* - Host access to {@code commandBuffer} must be externally synchronized
* - Host access to the {@code VkCommandPool} that {@code commandBuffer} was allocated from must be externally synchronized
*
*
* Command Properties
*
*
* Command Buffer Levels Render Pass Scope Supported Queue Types
* Primary Secondary Both Graphics
*
*
* @param commandBuffer the command buffer into which the command will be recorded.
* @param patchControlPoints specifies the number of control points per patch.
*/
public static void vkCmdSetPatchControlPointsEXT(VkCommandBuffer commandBuffer, @NativeType("uint32_t") int patchControlPoints) {
long __functionAddress = commandBuffer.getCapabilities().vkCmdSetPatchControlPointsEXT;
if (CHECKS) {
check(__functionAddress);
}
callPV(commandBuffer.address(), patchControlPoints, __functionAddress);
}
// --- [ vkCmdSetRasterizerDiscardEnableEXT ] ---
/**
* Control whether primitives are discarded before the rasterization stage dynamically for a command buffer.
*
* C Specification
*
* To dynamically enable whether primitives are discarded before the rasterization stage, call:
*
*
* void vkCmdSetRasterizerDiscardEnableEXT(
* VkCommandBuffer commandBuffer,
* VkBool32 rasterizerDiscardEnable);
*
* Description
*
* This command sets the discard enable for subsequent drawing commands when the graphics pipeline is created with {@link #VK_DYNAMIC_STATE_RASTERIZER_DISCARD_ENABLE_EXT DYNAMIC_STATE_RASTERIZER_DISCARD_ENABLE_EXT} set in {@link VkPipelineDynamicStateCreateInfo}{@code ::pDynamicStates}. Otherwise, this state is specified by the {@link VkPipelineRasterizationStateCreateInfo}{@code ::rasterizerDiscardEnable} value used to create the currently active pipeline.
*
* Valid Usage
*
*
* - The extendedDynamicState2 feature must be enabled
*
*
* Valid Usage (Implicit)
*
*
* - {@code commandBuffer} must be a valid {@code VkCommandBuffer} handle
* - {@code commandBuffer} must be in the recording state
* - The {@code VkCommandPool} that {@code commandBuffer} was allocated from must support graphics operations
*
*
* Host Synchronization
*
*
* - Host access to {@code commandBuffer} must be externally synchronized
* - Host access to the {@code VkCommandPool} that {@code commandBuffer} was allocated from must be externally synchronized
*
*
* Command Properties
*
*
* Command Buffer Levels Render Pass Scope Supported Queue Types
* Primary Secondary Both Graphics
*
*
* @param commandBuffer the command buffer into which the command will be recorded.
* @param rasterizerDiscardEnable controls whether primitives are discarded immediately before the rasterization stage.
*/
public static void vkCmdSetRasterizerDiscardEnableEXT(VkCommandBuffer commandBuffer, @NativeType("VkBool32") boolean rasterizerDiscardEnable) {
long __functionAddress = commandBuffer.getCapabilities().vkCmdSetRasterizerDiscardEnableEXT;
if (CHECKS) {
check(__functionAddress);
}
callPV(commandBuffer.address(), rasterizerDiscardEnable ? 1 : 0, __functionAddress);
}
// --- [ vkCmdSetDepthBiasEnableEXT ] ---
/**
* Control whether to bias fragment depth values dynamically for a command buffer.
*
* C Specification
*
* To dynamically enable whether to bias fragment depth values, call:
*
*
* void vkCmdSetDepthBiasEnableEXT(
* VkCommandBuffer commandBuffer,
* VkBool32 depthBiasEnable);
*
* Description
*
* This command sets the depth bias enable for subsequent drawing commands when the graphics pipeline is created with {@link #VK_DYNAMIC_STATE_DEPTH_BIAS_ENABLE_EXT DYNAMIC_STATE_DEPTH_BIAS_ENABLE_EXT} set in {@link VkPipelineDynamicStateCreateInfo}{@code ::pDynamicStates}. Otherwise, this state is specified by the {@link VkPipelineRasterizationStateCreateInfo}{@code ::depthBiasEnable} value used to create the currently active pipeline.
*
* Valid Usage
*
*
* - The extendedDynamicState2 feature must be enabled
*
*
* Valid Usage (Implicit)
*
*
* - {@code commandBuffer} must be a valid {@code VkCommandBuffer} handle
* - {@code commandBuffer} must be in the recording state
* - The {@code VkCommandPool} that {@code commandBuffer} was allocated from must support graphics operations
*
*
* Host Synchronization
*
*
* - Host access to {@code commandBuffer} must be externally synchronized
* - Host access to the {@code VkCommandPool} that {@code commandBuffer} was allocated from must be externally synchronized
*
*
* Command Properties
*
*
* Command Buffer Levels Render Pass Scope Supported Queue Types
* Primary Secondary Both Graphics
*
*
* @param commandBuffer the command buffer into which the command will be recorded.
* @param depthBiasEnable controls whether to bias fragment depth values.
*/
public static void vkCmdSetDepthBiasEnableEXT(VkCommandBuffer commandBuffer, @NativeType("VkBool32") boolean depthBiasEnable) {
long __functionAddress = commandBuffer.getCapabilities().vkCmdSetDepthBiasEnableEXT;
if (CHECKS) {
check(__functionAddress);
}
callPV(commandBuffer.address(), depthBiasEnable ? 1 : 0, __functionAddress);
}
// --- [ vkCmdSetLogicOpEXT ] ---
/**
* Select which logical operation to apply for blend state dynamically for a command buffer.
*
* C Specification
*
* To dynamically set the logical operation to apply for blend state, call:
*
*
* void vkCmdSetLogicOpEXT(
* VkCommandBuffer commandBuffer,
* VkLogicOp logicOp);
*
* Description
*
* This command sets the logical operation for blend state for subsequent drawing commands when the graphics pipeline is created with {@link #VK_DYNAMIC_STATE_LOGIC_OP_EXT DYNAMIC_STATE_LOGIC_OP_EXT} set in {@link VkPipelineDynamicStateCreateInfo}{@code ::pDynamicStates}. Otherwise, this state is specified by the {@link VkPipelineColorBlendStateCreateInfo}{@code ::logicOp} value used to create the currently active pipeline.
*
* Valid Usage
*
*
* - The extendedDynamicState2LogicOp feature must be enabled
*
*
* Valid Usage (Implicit)
*
*
* - {@code commandBuffer} must be a valid {@code VkCommandBuffer} handle
* - {@code logicOp} must be a valid {@code VkLogicOp} value
* - {@code commandBuffer} must be in the recording state
* - The {@code VkCommandPool} that {@code commandBuffer} was allocated from must support graphics operations
*
*
* Host Synchronization
*
*
* - Host access to {@code commandBuffer} must be externally synchronized
* - Host access to the {@code VkCommandPool} that {@code commandBuffer} was allocated from must be externally synchronized
*
*
* Command Properties
*
*
* Command Buffer Levels Render Pass Scope Supported Queue Types
* Primary Secondary Both Graphics
*
*
* @param commandBuffer the command buffer into which the command will be recorded.
* @param logicOp specifies the logical operation to apply for blend state.
*/
public static void vkCmdSetLogicOpEXT(VkCommandBuffer commandBuffer, @NativeType("VkLogicOp") int logicOp) {
long __functionAddress = commandBuffer.getCapabilities().vkCmdSetLogicOpEXT;
if (CHECKS) {
check(__functionAddress);
}
callPV(commandBuffer.address(), logicOp, __functionAddress);
}
// --- [ vkCmdSetPrimitiveRestartEnableEXT ] ---
/**
* Set primitive assembly restart state dynamically for a command buffer.
*
* C Specification
*
* To dynamically control whether a special vertex index value is treated as restarting the assembly of primitives, call:
*
*
* void vkCmdSetPrimitiveRestartEnableEXT(
* VkCommandBuffer commandBuffer,
* VkBool32 primitiveRestartEnable);
*
* Description
*
* This command sets the primitive restart enable for subsequent drawing commands when the graphics pipeline is created with {@link #VK_DYNAMIC_STATE_PRIMITIVE_RESTART_ENABLE_EXT DYNAMIC_STATE_PRIMITIVE_RESTART_ENABLE_EXT} set in {@link VkPipelineDynamicStateCreateInfo}{@code ::pDynamicStates}. Otherwise, this state is specified by the {@link VkPipelineInputAssemblyStateCreateInfo}{@code ::primitiveRestartEnable} value used to create the currently active pipeline.
*
* Valid Usage
*
*
* - The extendedDynamicState2 feature must be enabled
*
*
* Valid Usage (Implicit)
*
*
* - {@code commandBuffer} must be a valid {@code VkCommandBuffer} handle
* - {@code commandBuffer} must be in the recording state
* - The {@code VkCommandPool} that {@code commandBuffer} was allocated from must support graphics operations
*
*
* Host Synchronization
*
*
* - Host access to {@code commandBuffer} must be externally synchronized
* - Host access to the {@code VkCommandPool} that {@code commandBuffer} was allocated from must be externally synchronized
*
*
* Command Properties
*
*
* Command Buffer Levels Render Pass Scope Supported Queue Types
* Primary Secondary Both Graphics
*
*
* @param commandBuffer the command buffer into which the command will be recorded.
* @param primitiveRestartEnable controls whether a special vertex index value is treated as restarting the assembly of primitives. It behaves in the same way as {@link VkPipelineInputAssemblyStateCreateInfo}{@code ::primitiveRestartEnable}
*/
public static void vkCmdSetPrimitiveRestartEnableEXT(VkCommandBuffer commandBuffer, @NativeType("VkBool32") boolean primitiveRestartEnable) {
long __functionAddress = commandBuffer.getCapabilities().vkCmdSetPrimitiveRestartEnableEXT;
if (CHECKS) {
check(__functionAddress);
}
callPV(commandBuffer.address(), primitiveRestartEnable ? 1 : 0, __functionAddress);
}
}