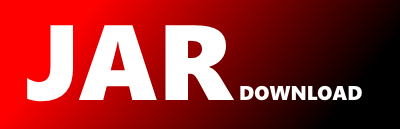
org.lwjgl.vulkan.HUAWEIInvocationMask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lwjgl-vulkan Show documentation
Show all versions of lwjgl-vulkan Show documentation
A new generation graphics and compute API that provides high-efficiency, cross-platform access to modern GPUs used in a wide variety of devices from PCs and consoles to mobile phones and embedded platforms.
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.vulkan;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
/**
* The rays to trace may be sparse in some use cases. For example, the scene only have a few regions to reflect. Providing an invocation mask image to the ray tracing commands could potentially give the hardware the hint to do certain optimization without invoking an additional pass to compact the ray buffer.
*
* Examples
*
* RT mask is updated before each traceRay.
*
* Step 1. Generate InvocationMask.
*
*
* //the rt mask image bind as color attachment in the fragment shader
* Layout(location = 2) out vec4 outRTmask
* vec4 mask = vec4(x,x,x,x);
* outRTmask = mask;
*
* Step 2. traceRay with InvocationMask
*
*
* vkCmdBindPipeline(
* commandBuffers[imageIndex],
* VK_PIPELINE_BIND_POINT_RAY_TRACING_KHR, m_rtPipeline);
* vkCmdBindDescriptorSets(commandBuffers[imageIndex],
* VK_PIPELINE_BIND_POINT_RAY_TRACING_NV,
* m_rtPipelineLayout, 0, 1, &m_rtDescriptorSet,
* 0, nullptr);
*
* vkCmdBindInvocationMaskHUAWEI(
* commandBuffers[imageIndex],
* InvocationMaskimageView,
* InvocationMaskimageLayout);
* vkCmdTraceRaysKHR(commandBuffers[imageIndex],
* pRaygenShaderBindingTable,
* pMissShaderBindingTable,
* swapChainExtent.width,
* swapChainExtent.height, 1);
*
* VK_HUAWEI_invocation_mask
*
*
* - Name String
* - {@code VK_HUAWEI_invocation_mask}
* - Extension Type
* - Device extension
* - Registered Extension Number
* - 371
* - Revision
* - 1
* - Extension and Version Dependencies
*
* - Requires Vulkan 1.0
* - Requires {@link KHRRayTracingPipeline VK_KHR_ray_tracing_pipeline}
* - Requires {@link KHRSynchronization2 VK_KHR_synchronization2}
*
* - Contact
*
* - Yunpeng Zhu yunxingzhu
*
* - Extension Proposal
* - VK_HUAWEI_invocation_mask
*
*
* Other Extension Metadata
*
*
* - Last Modified Date
* - 2021-05-27
* - Interactions and External Dependencies
*
* - This extension requires {@link KHRRayTracingPipeline VK_KHR_ray_tracing_pipeline}, which allow to bind an invocation mask image before the ray tracing command
* - This extension requires {@link KHRSynchronization2 VK_KHR_synchronization2}, which allows new pipeline stage for the invocation mask image
*
* - Contributors
*
* - Yunpeng Zhu, HuaWei
*
*
*/
public class HUAWEIInvocationMask {
/** The extension specification version. */
public static final int VK_HUAWEI_INVOCATION_MASK_SPEC_VERSION = 1;
/** The extension name. */
public static final String VK_HUAWEI_INVOCATION_MASK_EXTENSION_NAME = "VK_HUAWEI_invocation_mask";
/** Extends {@code VkStructureType}. */
public static final int VK_STRUCTURE_TYPE_PHYSICAL_DEVICE_INVOCATION_MASK_FEATURES_HUAWEI = 1000370000;
/** Extends {@code VkAccessFlagBits2KHR}. */
public static final long VK_ACCESS_2_INVOCATION_MASK_READ_BIT_HUAWEI = 0x8000000000L;
/** Extends {@code VkImageUsageFlagBits}. */
public static final int VK_IMAGE_USAGE_INVOCATION_MASK_BIT_HUAWEI = 0x40000;
/** Extends {@code VkPipelineStageFlagBits2KHR}. */
public static final long VK_PIPELINE_STAGE_2_INVOCATION_MASK_BIT_HUAWEI = 0x10000000000L;
protected HUAWEIInvocationMask() {
throw new UnsupportedOperationException();
}
// --- [ vkCmdBindInvocationMaskHUAWEI ] ---
/**
* Bind an invocation mask image on a command buffer.
*
* C Specification
*
* When invocation mask image usage is enabled in the bound ray tracing pipeline, the pipeline uses an invocation mask image specified by the command:
*
*
* void vkCmdBindInvocationMaskHUAWEI(
* VkCommandBuffer commandBuffer,
* VkImageView imageView,
* VkImageLayout imageLayout);
*
* Valid Usage
*
*
* - The invocation mask image feature must be enabled
* - If {@code imageView} is not {@link VK10#VK_NULL_HANDLE NULL_HANDLE}, it must be a valid {@code VkImageView} handle of type {@link VK10#VK_IMAGE_VIEW_TYPE_2D IMAGE_VIEW_TYPE_2D}
* - If {@code imageView} is not {@link VK10#VK_NULL_HANDLE NULL_HANDLE}, it must have a format of {@link VK10#VK_FORMAT_R8_UINT FORMAT_R8_UINT}
* - If {@code imageView} is not {@link VK10#VK_NULL_HANDLE NULL_HANDLE}, it must have been created with {@link #VK_IMAGE_USAGE_INVOCATION_MASK_BIT_HUAWEI IMAGE_USAGE_INVOCATION_MASK_BIT_HUAWEI} set
* - If {@code imageView} is not {@link VK10#VK_NULL_HANDLE NULL_HANDLE}, {@code imageLayout} must be {@link VK10#VK_IMAGE_LAYOUT_GENERAL IMAGE_LAYOUT_GENERAL}
* - Thread mask image resolution must match the {@code width} and {@code height} in {@link KHRRayTracingPipeline#vkCmdTraceRaysKHR CmdTraceRaysKHR}
* - Each element in the invocation mask image must have the value 0 or 1. The value 1 means the invocation is active
* - {@code width} in {@link KHRRayTracingPipeline#vkCmdTraceRaysKHR CmdTraceRaysKHR} should be 1
*
*
* Valid Usage (Implicit)
*
*
* - {@code commandBuffer} must be a valid {@code VkCommandBuffer} handle
* - If {@code imageView} is not {@link VK10#VK_NULL_HANDLE NULL_HANDLE}, {@code imageView} must be a valid {@code VkImageView} handle
* - {@code imageLayout} must be a valid {@code VkImageLayout} value
* - {@code commandBuffer} must be in the recording state
* - The {@code VkCommandPool} that {@code commandBuffer} was allocated from must support compute operations
* - This command must only be called outside of a render pass instance
* - Both of {@code commandBuffer}, and {@code imageView} that are valid handles of non-ignored parameters must have been created, allocated, or retrieved from the same {@code VkDevice}
*
*
* Host Synchronization
*
*
* - Host access to {@code commandBuffer} must be externally synchronized
* - Host access to the {@code VkCommandPool} that {@code commandBuffer} was allocated from must be externally synchronized
*
*
* Command Properties
*
*
* Command Buffer Levels Render Pass Scope Supported Queue Types
* Primary Secondary Outside Compute
*
*
* @param commandBuffer the command buffer into which the command will be recorded
* @param imageView an image view handle that specifies the invocation mask image {@code imageView} may be set to {@link VK10#VK_NULL_HANDLE NULL_HANDLE}, which is equivalent to specifying a view of an image filled with ones value.
* @param imageLayout the layout that the image subresources accessible from {@code imageView} will be in when the invocation mask image is accessed
*/
public static void vkCmdBindInvocationMaskHUAWEI(VkCommandBuffer commandBuffer, @NativeType("VkImageView") long imageView, @NativeType("VkImageLayout") int imageLayout) {
long __functionAddress = commandBuffer.getCapabilities().vkCmdBindInvocationMaskHUAWEI;
if (CHECKS) {
check(__functionAddress);
}
callPJV(commandBuffer.address(), imageView, imageLayout, __functionAddress);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy