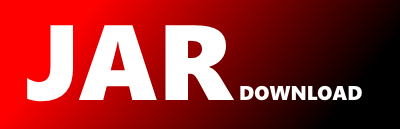
org.lwjgl.vulkan.NVCoverageReductionMode Maven / Gradle / Ivy
Show all versions of lwjgl-vulkan Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.vulkan;
import javax.annotation.*;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.JNI.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* When using a framebuffer with mixed samples, a per-fragment coverage reduction operation is performed which generates color sample coverage from the pixel coverage. This extension defines the following modes to control how this reduction is performed.
*
*
* - Merge: When there are more samples in the pixel coverage than color samples, there is an implementation-dependent association of each pixel coverage sample to a color sample. In the merge mode, the color sample coverage is computed such that only if any associated sample in the pixel coverage is covered, the color sample is covered. This is the default mode.
* - Truncate: When there are more raster samples (N) than color samples(M), there is one to one association of the first M raster samples to the M color samples; other raster samples are ignored.
*
*
* When the number of raster samples is equal to the color samples, there is a one to one mapping between them in either of the above modes.
*
* The new command {@link #vkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV GetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV} can be used to query the various raster, color, depth/stencil sample count and reduction mode combinations that are supported by the implementation. This extension would allow an implementation to support the behavior of both {@code VK_NV_framebuffer_mixed_samples} and {@code VK_AMD_mixed_attachment_samples} extensions simultaneously.
*
* VK_NV_coverage_reduction_mode
*
*
* - Name String
* - {@code VK_NV_coverage_reduction_mode}
* - Extension Type
* - Device extension
* - Registered Extension Number
* - 251
* - Revision
* - 1
* - Extension and Version Dependencies
*
* - Requires Vulkan 1.0
* - Requires {@link NVFramebufferMixedSamples VK_NV_framebuffer_mixed_samples}
*
* - Contact
*
* - Kedarnath Thangudu kthangudu
*
*
*
* Other Extension Metadata
*
*
* - Last Modified Date
* - 2019-01-29
* - Contributors
*
* - Kedarnath Thangudu, NVIDIA
* - Jeff Bolz, NVIDIA
*
*
*/
public class NVCoverageReductionMode {
/** The extension specification version. */
public static final int VK_NV_COVERAGE_REDUCTION_MODE_SPEC_VERSION = 1;
/** The extension name. */
public static final String VK_NV_COVERAGE_REDUCTION_MODE_EXTENSION_NAME = "VK_NV_coverage_reduction_mode";
/**
* Extends {@code VkStructureType}.
*
* Enum values:
*
*
* - {@link #VK_STRUCTURE_TYPE_PHYSICAL_DEVICE_COVERAGE_REDUCTION_MODE_FEATURES_NV STRUCTURE_TYPE_PHYSICAL_DEVICE_COVERAGE_REDUCTION_MODE_FEATURES_NV}
* - {@link #VK_STRUCTURE_TYPE_PIPELINE_COVERAGE_REDUCTION_STATE_CREATE_INFO_NV STRUCTURE_TYPE_PIPELINE_COVERAGE_REDUCTION_STATE_CREATE_INFO_NV}
* - {@link #VK_STRUCTURE_TYPE_FRAMEBUFFER_MIXED_SAMPLES_COMBINATION_NV STRUCTURE_TYPE_FRAMEBUFFER_MIXED_SAMPLES_COMBINATION_NV}
*
*/
public static final int
VK_STRUCTURE_TYPE_PHYSICAL_DEVICE_COVERAGE_REDUCTION_MODE_FEATURES_NV = 1000250000,
VK_STRUCTURE_TYPE_PIPELINE_COVERAGE_REDUCTION_STATE_CREATE_INFO_NV = 1000250001,
VK_STRUCTURE_TYPE_FRAMEBUFFER_MIXED_SAMPLES_COMBINATION_NV = 1000250002;
/**
* VkCoverageReductionModeNV - Specify the coverage reduction mode
*
* Description
*
*
* - {@link #VK_COVERAGE_REDUCTION_MODE_MERGE_NV COVERAGE_REDUCTION_MODE_MERGE_NV} specifies that each color sample will be associated with an implementation-dependent subset of samples in the pixel coverage. If any of those associated samples are covered, the color sample is covered.
* - {@link #VK_COVERAGE_REDUCTION_MODE_TRUNCATE_NV COVERAGE_REDUCTION_MODE_TRUNCATE_NV} specifies that for color samples present in the color attachments, a color sample is covered if the pixel coverage sample with the same sample index
i
is covered; other pixel coverage samples are discarded.
*
*
* See Also
*
* {@link VkFramebufferMixedSamplesCombinationNV}, {@link VkPipelineCoverageReductionStateCreateInfoNV}
*/
public static final int
VK_COVERAGE_REDUCTION_MODE_MERGE_NV = 0,
VK_COVERAGE_REDUCTION_MODE_TRUNCATE_NV = 1;
protected NVCoverageReductionMode() {
throw new UnsupportedOperationException();
}
// --- [ vkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV ] ---
/**
* Unsafe version of: {@link #vkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV GetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV}
*
* @param pCombinationCount a pointer to an integer related to the number of combinations available or queried, as described below.
*/
public static int nvkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV(VkPhysicalDevice physicalDevice, long pCombinationCount, long pCombinations) {
long __functionAddress = physicalDevice.getCapabilities().vkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV;
if (CHECKS) {
check(__functionAddress);
}
return callPPPI(physicalDevice.address(), pCombinationCount, pCombinations, __functionAddress);
}
/**
* Query supported sample count combinations.
*
* C Specification
*
* To query the set of mixed sample combinations of coverage reduction mode, rasterization samples and color, depth, stencil attachment sample counts that are supported by a physical device, call:
*
*
* VkResult vkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV(
* VkPhysicalDevice physicalDevice,
* uint32_t* pCombinationCount,
* VkFramebufferMixedSamplesCombinationNV* pCombinations);
*
* Description
*
* If {@code pCombinations} is {@code NULL}, then the number of supported combinations for the given {@code physicalDevice} is returned in {@code pCombinationCount}. Otherwise, {@code pCombinationCount} must point to a variable set by the user to the number of elements in the {@code pCombinations} array, and on return the variable is overwritten with the number of values actually written to {@code pCombinations}. If the value of {@code pCombinationCount} is less than the number of combinations supported for the given {@code physicalDevice}, at most {@code pCombinationCount} values will be written to {@code pCombinations}, and {@link VK10#VK_INCOMPLETE INCOMPLETE} will be returned instead of {@link VK10#VK_SUCCESS SUCCESS}, to indicate that not all the supported values were returned.
*
* Valid Usage (Implicit)
*
*
* - {@code physicalDevice} must be a valid {@code VkPhysicalDevice} handle
* - {@code pCombinationCount} must be a valid pointer to a {@code uint32_t} value
* - If the value referenced by {@code pCombinationCount} is not 0, and {@code pCombinations} is not {@code NULL}, {@code pCombinations} must be a valid pointer to an array of {@code pCombinationCount} {@link VkFramebufferMixedSamplesCombinationNV} structures
*
*
* Return Codes
*
*
* - On success, this command returns
*
* - {@link VK10#VK_SUCCESS SUCCESS}
* - {@link VK10#VK_INCOMPLETE INCOMPLETE}
*
* - On failure, this command returns
*
* - {@link VK10#VK_ERROR_OUT_OF_HOST_MEMORY ERROR_OUT_OF_HOST_MEMORY}
* - {@link VK10#VK_ERROR_OUT_OF_DEVICE_MEMORY ERROR_OUT_OF_DEVICE_MEMORY}
*
*
*
* See Also
*
* {@link VkFramebufferMixedSamplesCombinationNV}
*
* @param physicalDevice the physical device from which to query the set of combinations.
* @param pCombinationCount a pointer to an integer related to the number of combinations available or queried, as described below.
* @param pCombinations either {@code NULL} or a pointer to an array of {@link VkFramebufferMixedSamplesCombinationNV} values, indicating the supported combinations of coverage reduction mode, rasterization samples, and color, depth, stencil attachment sample counts.
*/
@NativeType("VkResult")
public static int vkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV(VkPhysicalDevice physicalDevice, @NativeType("uint32_t *") IntBuffer pCombinationCount, @Nullable @NativeType("VkFramebufferMixedSamplesCombinationNV *") VkFramebufferMixedSamplesCombinationNV.Buffer pCombinations) {
if (CHECKS) {
check(pCombinationCount, 1);
checkSafe(pCombinations, pCombinationCount.get(pCombinationCount.position()));
}
return nvkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV(physicalDevice, memAddress(pCombinationCount), memAddressSafe(pCombinations));
}
/** Array version of: {@link #vkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV GetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV} */
@NativeType("VkResult")
public static int vkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV(VkPhysicalDevice physicalDevice, @NativeType("uint32_t *") int[] pCombinationCount, @Nullable @NativeType("VkFramebufferMixedSamplesCombinationNV *") VkFramebufferMixedSamplesCombinationNV.Buffer pCombinations) {
long __functionAddress = physicalDevice.getCapabilities().vkGetPhysicalDeviceSupportedFramebufferMixedSamplesCombinationsNV;
if (CHECKS) {
check(__functionAddress);
check(pCombinationCount, 1);
checkSafe(pCombinations, pCombinationCount[0]);
}
return callPPPI(physicalDevice.address(), pCombinationCount, memAddressSafe(pCombinations), __functionAddress);
}
}