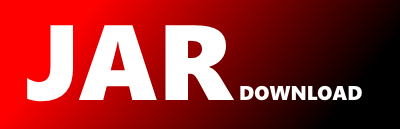
org.lwjgl.util.zstd.Zstd Maven / Gradle / Ivy
Show all versions of lwjgl-zstd Show documentation
/*
* Copyright LWJGL. All rights reserved.
* License terms: https://www.lwjgl.org/license
* MACHINE GENERATED FILE, DO NOT EDIT
*/
package org.lwjgl.util.zstd;
import java.nio.*;
import org.lwjgl.system.*;
import static org.lwjgl.system.Checks.*;
import static org.lwjgl.system.MemoryUtil.*;
/**
* Native bindings to Zstandard (zstd), a fast lossless compression algorithm, targeting real-time
* compression scenarios at zlib-level and better compression ratios.
*
* Introduction
*
* zstd, short for Zstandard, is a fast lossless compression algorithm, targeting real-time compression scenarios at zlib-level and better compression
* ratios. The zstd compression library provides in-memory compression and decompression functions. The library supports compression levels from 1 up to
* {@link #ZSTD_maxCLevel maxCLevel} which is currently 22. Levels ≥ 20, labeled {@code --ultra}, should be used with caution, as they require more memory.
*
* Compression can be done in:
*
*
* - a single step (described as Simple API)
* - a single step, reusing a context (described as Explicit memory management)
* - unbounded multiple steps (described as Streaming compression)
*
*
* The compression ratio achievable on small data can be highly improved using a dictionary in:
*
*
* - a single step (described as Simple dictionary API)
* - a single step, reusing a dictionary (described as Fast dictionary API)
*
*
* Advanced experimental functions can be accessed using {@code #define ZSTD_STATIC_LINKING_ONLY} before including {@code zstd.h}. Advanced experimental
* APIs shall never be used with a dynamic library. They are not "stable", their definition may change in the future. Only static linking is allowed.
*
* Streaming compression - HowTo
*
* A {@code ZSTD_CStream} object is required to track streaming operation.
*
* Use {@link #ZSTD_createCStream createCStream} and {@link #ZSTD_freeCStream freeCStream} to create/release resources. {@code ZSTD_CStream} objects can be reused multiple times on consecutive
* compression operations. It is recommended to re-use {@code ZSTD_CStream} in situations where many streaming operations will be achieved consecutively,
* since it will play nicer with system's memory, by re-using already allocated memory. Use one separate {@code ZSTD_CStream} per thread for parallel
* execution.
*
* Start a new compression by initializing {@code ZSTD_CStream}. Use {@link #ZSTD_initCStream initCStream} to start a new compression operation. Use
* {@code ZSTD_initCStream_usingDict()} or {@code ZSTD_initCStream_usingCDict()} for a compression which requires a dictionary (experimental section).
*
* Use {@link #ZSTD_compressStream compressStream} repetitively to consume input stream. The function will automatically update both {@code pos} fields. Note that it may not
* consume the entire input, in which case {@code pos < size}, and it's up to the caller to present again remaining data.
*
* At any moment, it's possible to flush whatever data remains within internal buffer, using {@link #ZSTD_flushStream flushStream}. {@code output->pos} will be updated. Note
* that some content might still be left within internal buffer if {@code output->size} is too small.
*
* {@link #ZSTD_endStream endStream} instructs to finish a frame. It will perform a flush and write frame epilogue. The epilogue is required for decoders to consider a frame
* completed. {@link #ZSTD_endStream endStream} may not be able to flush full data if {output->size} is too small. In which case, call again {@link #ZSTD_endStream endStream} to complete the
* flush.
*
* Streaming decompression - HowTo
*
* A {@code ZSTD_DStream} object is required to track streaming operations.
*
* Use {@link #ZSTD_createDStream createDStream} and {@link #ZSTD_freeDStream freeDStream} to create/release resources. {@code ZSTD_DStream} objects can be re-used multiple times.
*
* Use {@link #ZSTD_initDStream initDStream} to start a new decompression operation, or {@code ZSTD_initDStream_usingDict()} if decompression requires a dictionary.
*
* Use {@link #ZSTD_decompressStream decompressStream} repetitively to consume your input. The function will update both {@code pos} fields. If {@code input.pos < input.size}, some
* input has not been consumed. It's up to the caller to present again remaining data. If {@code output.pos < output.size}, decoder has flushed everything
* it could.
*/
public class Zstd {
/** Version number part. */
public static final int
ZSTD_VERSION_MAJOR = 1,
ZSTD_VERSION_MINOR = 3,
ZSTD_VERSION_RELEASE = 2;
/** Version number. */
public static final int ZSTD_VERSION_NUMBER = (ZSTD_VERSION_MAJOR *100*100 + ZSTD_VERSION_MINOR *100 + ZSTD_VERSION_RELEASE);
/** Version string. */
public static final String ZSTD_VERSION_STRING = ZSTD_VERSION_MAJOR + "." + ZSTD_VERSION_MINOR + "." + ZSTD_VERSION_RELEASE;
/** Content size. */
public static final long
ZSTD_CONTENTSIZE_UNKNOWN = -1L,
ZSTD_CONTENTSIZE_ERROR = -2L;
/** Default compression level. */
public static final int ZSTD_CLEVEL_DEFAULT = 3;
static { LibZstd.initialize(); }
protected Zstd() {
throw new UnsupportedOperationException();
}
// --- [ ZSTD_versionNumber ] ---
/** Returns the version number. */
@NativeType("unsigned")
public static native int ZSTD_versionNumber();
// --- [ ZSTD_versionString ] ---
/** Unsafe version of: {@link #ZSTD_versionString versionString} */
public static native long nZSTD_versionString();
/** Returns the version string. */
@NativeType("const char *")
public static String ZSTD_versionString() {
long __result = nZSTD_versionString();
return memASCII(__result);
}
// --- [ ZSTD_compress ] ---
/** Unsafe version of: {@link #ZSTD_compress compress} */
public static native long nZSTD_compress(long dst, long dstCapacity, long src, long srcSize, int compressionLevel);
/**
* Compresses {@code src} content as a single zstd compressed frame into already allocated {@code dst}.
*
* Hint: compression runs faster if {@code dstCapacity} ≥ {@link #ZSTD_compressBound compressBound}{@code (srcSize)}
*
* @param dst
* @param src
* @param compressionLevel
*
* @return compressed size written into {@code dst} (≤ {@code dstCapacity}), or an error code if it fails (which can be tested using {@link #ZSTD_isError isError}).
*/
@NativeType("size_t")
public static long ZSTD_compress(@NativeType("void *") ByteBuffer dst, @NativeType("const void *") ByteBuffer src, @NativeType("int") int compressionLevel) {
return nZSTD_compress(memAddress(dst), dst.remaining(), memAddress(src), src.remaining(), compressionLevel);
}
// --- [ ZSTD_decompress ] ---
/**
* Unsafe version of: {@link #ZSTD_decompress decompress}
*
* @param dstCapacity is an upper bound of {@code originalSize} to regenerate. If user cannot imply a maximum upper bound, it's better to use streaming mode to
* decompress data.
* @param compressedSize must be the exact size of some number of compressed and/or skippable frames
*/
public static native long nZSTD_decompress(long dst, long dstCapacity, long src, long compressedSize);
/**
* @param dst
* @param src
*
* @return the number of bytes decompressed into {@code dst} (≤ {@code dstCapacity}), or an {@code errorCode} if it fails (which can be tested using
* {@link #ZSTD_isError isError}).
*/
@NativeType("size_t")
public static long ZSTD_decompress(@NativeType("void *") ByteBuffer dst, @NativeType("const void *") ByteBuffer src) {
return nZSTD_decompress(memAddress(dst), dst.remaining(), memAddress(src), src.remaining());
}
// --- [ ZSTD_getFrameContentSize ] ---
/**
* Unsafe version of: {@link #ZSTD_getFrameContentSize getFrameContentSize}
*
* @param srcSize must be at least as large as the frame header. Hint: any size ≥ {@link ZstdX#ZSTD_FRAMEHEADERSIZE_MAX FRAMEHEADERSIZE_MAX} is large enough.
*/
public static native long nZSTD_getFrameContentSize(long src, long srcSize);
/**
* Notes:
*
*
* - a 0 return value means the frame is valid but "empty"
* - decompressed size is an optional field, it may not be present, typically in streaming mode. When {@code return==ZSTD_CONTENTSIZE_UNKNOWN}, data to
* decompress could be any size. In which case, it's necessary to use streaming mode to decompress data. Optionally, application can rely on some
* implicit limit, as {@link #ZSTD_decompress decompress} only needs an upper bound of decompressed size. (For example, data could be necessarily cut into blocks <= 16 KB).
* - decompressed size is always present when compression is done with {@link #ZSTD_compress compress}
* - decompressed size can be very large (64-bits value), potentially larger than what local system can handle as a single memory segment. In which
* case, it's necessary to use streaming mode to decompress data.
* - If source is untrusted, decompressed size could be wrong or intentionally modified. Always ensure return value fits within application's authorized
* limits. Each application can set its own limits.
*
*
* @param src should point to the start of a ZSTD encoded frame
*
* @return decompressed size of the frame in {@code src}, if known
*
*
* - {@link #ZSTD_CONTENTSIZE_UNKNOWN CONTENTSIZE_UNKNOWN} if the size cannot be determined
* - {@link #ZSTD_CONTENTSIZE_ERROR CONTENTSIZE_ERROR} if an error occurred (e.g. invalid magic number, {@code srcSize} too small)
*
*/
@NativeType("unsigned long long")
public static long ZSTD_getFrameContentSize(@NativeType("const void *") ByteBuffer src) {
return nZSTD_getFrameContentSize(memAddress(src), src.remaining());
}
// --- [ ZSTD_compressBound ] ---
/**
* Returns the maximum compressed size in worst case scenario.
*
* @param srcSize
*/
@NativeType("size_t")
public static native long ZSTD_compressBound(@NativeType("size_t") long srcSize);
// --- [ ZSTD_isError ] ---
/** Unsafe version of: {@link #ZSTD_isError isError} */
public static native int nZSTD_isError(long code);
/**
* Tells if a {@code size_t} function result is an error code.
*
* @param code
*/
@NativeType("unsigned int")
public static boolean ZSTD_isError(@NativeType("size_t") long code) {
return nZSTD_isError(code) != 0;
}
// --- [ ZSTD_getErrorName ] ---
/** Unsafe version of: {@link #ZSTD_getErrorName getErrorName} */
public static native long nZSTD_getErrorName(long code);
/**
* Provides readable string from an error code.
*
* @param code
*/
@NativeType("const char *")
public static String ZSTD_getErrorName(@NativeType("size_t") long code) {
long __result = nZSTD_getErrorName(code);
return memASCII(__result);
}
// --- [ ZSTD_maxCLevel ] ---
/** Returns the maximum compression level available. */
public static native int ZSTD_maxCLevel();
// --- [ ZSTD_createCCtx ] ---
/**
* Creates a compression context.
*
* When compressing many times, it is recommended to allocate a context just once, and re-use it for each successive compression operation. This will make
* workload friendlier for system's memory. Use one context per thread for parallel execution in multi-threaded environments.
*/
@NativeType("ZSTD_CCtx *")
public static native long ZSTD_createCCtx();
// --- [ ZSTD_freeCCtx ] ---
/** Unsafe version of: {@link #ZSTD_freeCCtx freeCCtx} */
public static native long nZSTD_freeCCtx(long cctx);
/**
* Frees memory allocated by {@link #ZSTD_createCCtx createCCtx}.
*
* @param cctx
*/
@NativeType("size_t")
public static long ZSTD_freeCCtx(@NativeType("ZSTD_CCtx *") long cctx) {
if (CHECKS) {
check(cctx);
}
return nZSTD_freeCCtx(cctx);
}
// --- [ ZSTD_compressCCtx ] ---
/** Unsafe version of: {@link #ZSTD_compressCCtx compressCCtx} */
public static native long nZSTD_compressCCtx(long ctx, long dst, long dstCapacity, long src, long srcSize, int compressionLevel);
/**
* Same as {@link #ZSTD_compress compress}, requires an allocated {@code ZSTD_CCtx} (see {@link #ZSTD_createCCtx createCCtx}).
*
* @param ctx
* @param dst
* @param src
* @param compressionLevel
*/
@NativeType("size_t")
public static long ZSTD_compressCCtx(@NativeType("ZSTD_CCtx *") long ctx, @NativeType("void *") ByteBuffer dst, @NativeType("const void *") ByteBuffer src, @NativeType("int") int compressionLevel) {
if (CHECKS) {
check(ctx);
}
return nZSTD_compressCCtx(ctx, memAddress(dst), dst.remaining(), memAddress(src), src.remaining(), compressionLevel);
}
// --- [ ZSTD_createDCtx ] ---
/**
* Creates a decompression context.
*
* When decompressing many times, it is recommended to allocate a context only once, and re-use it for each successive compression operation. This will
* make workload friendlier for system's memory. Use one context per thread for parallel execution.
*/
@NativeType("ZSTD_DCtx *")
public static native long ZSTD_createDCtx();
// --- [ ZSTD_freeDCtx ] ---
/** Unsafe version of: {@link #ZSTD_freeDCtx freeDCtx} */
public static native long nZSTD_freeDCtx(long dctx);
/**
* Frees memory allocated by {@link #ZSTD_createDCtx createDCtx}.
*
* @param dctx
*/
@NativeType("size_t")
public static long ZSTD_freeDCtx(@NativeType("ZSTD_DCtx *") long dctx) {
if (CHECKS) {
check(dctx);
}
return nZSTD_freeDCtx(dctx);
}
// --- [ ZSTD_decompressDCtx ] ---
/** Unsafe version of: {@link #ZSTD_decompressDCtx decompressDCtx} */
public static native long nZSTD_decompressDCtx(long ctx, long dst, long dstCapacity, long src, long srcSize);
/**
* Same as {@link #ZSTD_decompress decompress}, requires an allocated {@code ZSTD_DCtx} (see {@link #ZSTD_createDCtx createDCtx}).
*
* @param ctx
* @param dst
* @param src
*/
@NativeType("size_t")
public static long ZSTD_decompressDCtx(@NativeType("ZSTD_DCtx *") long ctx, @NativeType("void *") ByteBuffer dst, @NativeType("const void *") ByteBuffer src) {
if (CHECKS) {
check(ctx);
}
return nZSTD_decompressDCtx(ctx, memAddress(dst), dst.remaining(), memAddress(src), src.remaining());
}
// --- [ ZSTD_compress_usingDict ] ---
/** Unsafe version of: {@link #ZSTD_compress_usingDict compress_usingDict} */
public static native long nZSTD_compress_usingDict(long ctx, long dst, long dstCapacity, long src, long srcSize, long dict, long dictSize, int compressionLevel);
/**
* Compression using a predefined Dictionary (see {@code dictBuilder/zdict.h}).
*
* This function loads the dictionary, resulting in significant startup delay.
*
* When {@code dict == NULL || dictSize < 8} no dictionary is used.
*
* @param ctx
* @param dst
* @param src
* @param dict
* @param compressionLevel
*/
@NativeType("size_t")
public static long ZSTD_compress_usingDict(@NativeType("ZSTD_CCtx *") long ctx, @NativeType("void *") ByteBuffer dst, @NativeType("const void *") ByteBuffer src, @NativeType("const void *") ByteBuffer dict, @NativeType("int") int compressionLevel) {
if (CHECKS) {
check(ctx);
}
return nZSTD_compress_usingDict(ctx, memAddress(dst), dst.remaining(), memAddress(src), src.remaining(), memAddressSafe(dict), remainingSafe(dict), compressionLevel);
}
// --- [ ZSTD_decompress_usingDict ] ---
/** Unsafe version of: {@link #ZSTD_decompress_usingDict decompress_usingDict} */
public static native long nZSTD_decompress_usingDict(long dctx, long dst, long dstCapacity, long src, long srcSize, long dict, long dictSize);
/**
* Decompression using a predefined Dictionary (see {@code dictBuilder/zdict.h}). Dictionary must be identical to the one used during compression.
*
* This function loads the dictionary, resulting in significant startup delay.
*
* When {@code dict == NULL || dictSize < 8} no dictionary is used.
*
* @param dctx
* @param dst
* @param src
* @param dict
*/
@NativeType("size_t")
public static long ZSTD_decompress_usingDict(@NativeType("ZSTD_DCtx *") long dctx, @NativeType("void *") ByteBuffer dst, @NativeType("const void *") ByteBuffer src, @NativeType("const void *") ByteBuffer dict) {
if (CHECKS) {
check(dctx);
}
return nZSTD_decompress_usingDict(dctx, memAddress(dst), dst.remaining(), memAddress(src), src.remaining(), memAddressSafe(dict), remainingSafe(dict));
}
// --- [ ZSTD_createCDict ] ---
/** Unsafe version of: {@link #ZSTD_createCDict createCDict} */
public static native long nZSTD_createCDict(long dictBuffer, long dictSize, int compressionLevel);
/**
* When compressing multiple messages / blocks with the same dictionary, it's recommended to load it just once.
*
* {@code ZSTD_createCDict()} will create a digested dictionary, ready to start future compression operations without startup delay. {@code ZSTD_CDict}
* can be created once and shared by multiple threads concurrently, since its usage is read-only.
*
* {@code dictBuffer} can be released after {@code ZSTD_CDict} creation, since its content is copied within CDict.
*
* @param dictBuffer
* @param compressionLevel
*/
@NativeType("ZSTD_CDict *")
public static long ZSTD_createCDict(@NativeType("const void *") ByteBuffer dictBuffer, @NativeType("int") int compressionLevel) {
return nZSTD_createCDict(memAddress(dictBuffer), dictBuffer.remaining(), compressionLevel);
}
// --- [ ZSTD_freeCDict ] ---
/** Unsafe version of: {@link #ZSTD_freeCDict freeCDict} */
public static native long nZSTD_freeCDict(long CDict);
/**
* Frees memory allocated by {@link #ZSTD_createCDict createCDict}.
*
* @param CDict
*/
@NativeType("size_t")
public static long ZSTD_freeCDict(@NativeType("ZSTD_CDict *") long CDict) {
if (CHECKS) {
check(CDict);
}
return nZSTD_freeCDict(CDict);
}
// --- [ ZSTD_compress_usingCDict ] ---
/** Unsafe version of: {@link #ZSTD_compress_usingCDict compress_usingCDict} */
public static native long nZSTD_compress_usingCDict(long cctx, long dst, long dstCapacity, long src, long srcSize, long cdict);
/**
* Compression using a digested Dictionary.
*
* Faster startup than {@link #ZSTD_compress_usingDict compress_usingDict}, recommended when same dictionary is used multiple times. Note that compression level is decided during
* dictionary creation. Frame parameters are hardcoded ({@code dictID=yes, contentSize=yes, checksum=no})
*
* @param cctx
* @param dst
* @param src
* @param cdict
*/
@NativeType("size_t")
public static long ZSTD_compress_usingCDict(@NativeType("ZSTD_CCtx *") long cctx, @NativeType("void *") ByteBuffer dst, @NativeType("const void *") ByteBuffer src, @NativeType("const ZSTD_CDict *") long cdict) {
if (CHECKS) {
check(cctx);
check(cdict);
}
return nZSTD_compress_usingCDict(cctx, memAddress(dst), dst.remaining(), memAddress(src), src.remaining(), cdict);
}
// --- [ ZSTD_createDDict ] ---
/** Unsafe version of: {@link #ZSTD_createDDict createDDict} */
public static native long nZSTD_createDDict(long dictBuffer, long dictSize);
/**
* Creates a digested dictionary, ready to start decompression operation without startup delay.
*
* {@code dictBuffer} can be released after {@code DDict} creation, as its content is copied inside {@code DDict}.
*
* @param dictBuffer
*/
@NativeType("ZSTD_DDict *")
public static long ZSTD_createDDict(@NativeType("const void *") ByteBuffer dictBuffer) {
return nZSTD_createDDict(memAddress(dictBuffer), dictBuffer.remaining());
}
// --- [ ZSTD_freeDDict ] ---
/** Unsafe version of: {@link #ZSTD_freeDDict freeDDict} */
public static native long nZSTD_freeDDict(long ddict);
/**
* Frees memory allocated with {@link #ZSTD_createDDict createDDict}.
*
* @param ddict
*/
@NativeType("size_t")
public static long ZSTD_freeDDict(@NativeType("ZSTD_DDict *") long ddict) {
if (CHECKS) {
check(ddict);
}
return nZSTD_freeDDict(ddict);
}
// --- [ ZSTD_decompress_usingDDict ] ---
/** Unsafe version of: {@link #ZSTD_decompress_usingDDict decompress_usingDDict} */
public static native long nZSTD_decompress_usingDDict(long dctx, long dst, long dstCapacity, long src, long srcSize, long ddict);
/**
* Decompression using a digested Dictionary.
*
* Faster startup than {@link #ZSTD_decompress_usingDict decompress_usingDict}, recommended when same dictionary is used multiple times.
*
* @param dctx
* @param dst
* @param src
* @param ddict
*/
@NativeType("size_t")
public static long ZSTD_decompress_usingDDict(@NativeType("ZSTD_DCtx *") long dctx, @NativeType("void *") ByteBuffer dst, @NativeType("const void *") ByteBuffer src, @NativeType("const ZSTD_DDict *") long ddict) {
if (CHECKS) {
check(dctx);
check(ddict);
}
return nZSTD_decompress_usingDDict(dctx, memAddress(dst), dst.remaining(), memAddress(src), src.remaining(), ddict);
}
// --- [ ZSTD_createCStream ] ---
@NativeType("ZSTD_CStream *")
public static native long ZSTD_createCStream();
// --- [ ZSTD_freeCStream ] ---
/** Unsafe version of: {@link #ZSTD_freeCStream freeCStream} */
public static native long nZSTD_freeCStream(long zcs);
/**
* Frees memory allocated by {@link #ZSTD_createCStream createCStream}.
*
* @param zcs
*/
@NativeType("size_t")
public static long ZSTD_freeCStream(@NativeType("ZSTD_CStream *") long zcs) {
if (CHECKS) {
check(zcs);
}
return nZSTD_freeCStream(zcs);
}
// --- [ ZSTD_initCStream ] ---
public static native long nZSTD_initCStream(long zcs, int compressionLevel);
@NativeType("size_t")
public static long ZSTD_initCStream(@NativeType("ZSTD_CStream *") long zcs, int compressionLevel) {
if (CHECKS) {
check(zcs);
}
return nZSTD_initCStream(zcs, compressionLevel);
}
// --- [ ZSTD_compressStream ] ---
/** Unsafe version of: {@link #ZSTD_compressStream compressStream} */
public static native long nZSTD_compressStream(long zcs, long output, long input);
/**
* @param zcs
* @param output
* @param input
*
* @return a size hint, preferred {@code nb} of bytes to use as input for next function call or an error code, which can be tested using {@link #ZSTD_isError isError}.
*
* Notes:
*
*
* - it's just a hint, to help latency a little, any other value will work fine
* - size hint is guaranteed to be ≤ {@link #ZSTD_CStreamInSize CStreamInSize}
*
*/
@NativeType("size_t")
public static long ZSTD_compressStream(@NativeType("ZSTD_CStream *") long zcs, @NativeType("ZSTD_outBuffer *") ZSTDOutBuffer output, @NativeType("ZSTD_inBuffer *") ZSTDInBuffer input) {
if (CHECKS) {
check(zcs);
ZSTDOutBuffer.validate(output.address());
ZSTDInBuffer.validate(input.address());
}
return nZSTD_compressStream(zcs, output.address(), input.address());
}
// --- [ ZSTD_flushStream ] ---
/** Unsafe version of: {@link #ZSTD_flushStream flushStream} */
public static native long nZSTD_flushStream(long zcs, long output);
/**
* @param zcs
* @param output
*
* @return {@code nb} of bytes still present within internal buffer (0 if it's empty) or an error code, which can be tested using {@link #ZSTD_isError isError}
*/
@NativeType("size_t")
public static long ZSTD_flushStream(@NativeType("ZSTD_CStream *") long zcs, @NativeType("ZSTD_outBuffer *") ZSTDOutBuffer output) {
if (CHECKS) {
check(zcs);
ZSTDOutBuffer.validate(output.address());
}
return nZSTD_flushStream(zcs, output.address());
}
// --- [ ZSTD_endStream ] ---
/** Unsafe version of: {@link #ZSTD_endStream endStream} */
public static native long nZSTD_endStream(long zcs, long output);
/**
* @param zcs
* @param output
*
* @return 0 if frame fully completed and fully flushed, or > 0 if some data is still present within internal buffer (value is minimum size estimation for
* remaining data to flush, but it could be more) or an error code, which can be tested using {@link #ZSTD_isError isError}
*/
@NativeType("size_t")
public static long ZSTD_endStream(@NativeType("ZSTD_CStream *") long zcs, @NativeType("ZSTD_outBuffer *") ZSTDOutBuffer output) {
if (CHECKS) {
check(zcs);
ZSTDOutBuffer.validate(output.address());
}
return nZSTD_endStream(zcs, output.address());
}
// --- [ ZSTD_CStreamInSize ] ---
/** Returns the recommended size for input buffer. */
@NativeType("size_t")
public static native long ZSTD_CStreamInSize();
// --- [ ZSTD_CStreamOutSize ] ---
/** Returns the recommended size for output buffer. Guarantee to successfully flush at least one complete compressed block in all circumstances. */
@NativeType("size_t")
public static native long ZSTD_CStreamOutSize();
// --- [ ZSTD_createDStream ] ---
@NativeType("ZSTD_DStream *")
public static native long ZSTD_createDStream();
// --- [ ZSTD_freeDStream ] ---
/** Unsafe version of: {@link #ZSTD_freeDStream freeDStream} */
public static native long nZSTD_freeDStream(long zds);
/**
* Frees memory allocated by {@link #ZSTD_createDStream createDStream}.
*
* @param zds
*/
@NativeType("size_t")
public static long ZSTD_freeDStream(@NativeType("ZSTD_DStream *") long zds) {
if (CHECKS) {
check(zds);
}
return nZSTD_freeDStream(zds);
}
// --- [ ZSTD_initDStream ] ---
/** Unsafe version of: {@link #ZSTD_initDStream initDStream} */
public static native long nZSTD_initDStream(long zds);
/**
* @param zds
*
* @return recommended first input size
*/
@NativeType("size_t")
public static long ZSTD_initDStream(@NativeType("ZSTD_DStream *") long zds) {
if (CHECKS) {
check(zds);
}
return nZSTD_initDStream(zds);
}
// --- [ ZSTD_decompressStream ] ---
/** Unsafe version of: {@link #ZSTD_decompressStream decompressStream} */
public static native long nZSTD_decompressStream(long zds, long output, long input);
/**
* @param zds
* @param output
* @param input
*
* @return 0 when a frame is completely decoded and fully flushed, an error code, which can be tested using {@link #ZSTD_isError isError}, any other value > 0, which means there
* is still some decoding to do to complete current frame. The return value is a suggested next input size (a hint to improve latency) that will never
* load more than the current frame.
*/
@NativeType("size_t")
public static long ZSTD_decompressStream(@NativeType("ZSTD_DStream *") long zds, @NativeType("ZSTD_outBuffer *") ZSTDOutBuffer output, @NativeType("ZSTD_inBuffer *") ZSTDInBuffer input) {
if (CHECKS) {
check(zds);
ZSTDOutBuffer.validate(output.address());
ZSTDInBuffer.validate(input.address());
}
return nZSTD_decompressStream(zds, output.address(), input.address());
}
// --- [ ZSTD_DStreamInSize ] ---
/** Returns the recommended size for input buffer. */
@NativeType("size_t")
public static native long ZSTD_DStreamInSize();
// --- [ ZSTD_DStreamOutSize ] ---
/** Returns the recommended size for output buffer. Guarantee to successfully flush at least one complete compressed block in all circumstances. */
@NativeType("size_t")
public static native long ZSTD_DStreamOutSize();
/** Pure Java version of {@link #ZSTD_compressBound}. */
public static long ZSTD_COMPRESSBOUND(long srcSize) {
/* this formula ensures that bound(A) + bound(B) <= bound(A+B) as long as A and B >= 128 KB */
return srcSize
+ (srcSize >> 8)
+ (srcSize < (128 << 10)
? (128 << 10) - srcSize >> 11 /* margin, from 64 to 0 */
: 0
);
}
}