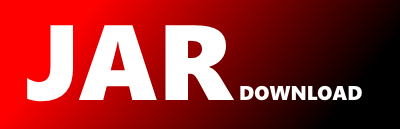
org.macrocloud.kernel.toolkit.beans.BaseBeanMap Maven / Gradle / Ivy
package org.macrocloud.kernel.toolkit.beans;
import org.springframework.asm.ClassVisitor;
import org.springframework.cglib.beans.BeanMap;
import org.springframework.cglib.core.AbstractClassGenerator;
import org.springframework.cglib.core.ReflectUtils;
import java.security.ProtectionDomain;
/**
* 重写 cglib BeanMap,支持链式bean.
*
* @author macro
*/
public abstract class BaseBeanMap extends BeanMap {
/**
* Instantiates a new base bean map.
*/
protected BaseBeanMap() {
}
/**
* Instantiates a new base bean map.
*
* @param bean the bean
*/
protected BaseBeanMap(Object bean) {
super(bean);
}
/**
* Creates the.
*
* @param bean the bean
*/
public static BaseBeanMap create(Object bean) {
BaseGenerator gen = new BaseGenerator();
gen.setBean(bean);
return gen.create();
}
/**
* newInstance.
*
* @param o Object
*/
@Override
public abstract BaseBeanMap newInstance(Object o);
/**
* The Class BaseGenerator.
*/
public static class BaseGenerator extends AbstractClassGenerator {
/** The Constant SOURCE. */
private static final Source SOURCE = new Source(BaseBeanMap.class.getName());
/** The bean. */
private Object bean;
/** The bean class. */
private Class beanClass;
/** The require. */
private int require;
/**
* Instantiates a new base generator.
*/
public BaseGenerator() {
super(SOURCE);
}
/**
* Set the bean that the generated map should reflect. The bean may be swapped
* out for another bean of the same type using {@link #setBean}.
* Calling this method overrides any value previously set using {@link #setBeanClass}.
* You must call either this method or {@link #setBeanClass} before {@link #create}.
*
* @param bean the initial bean
*/
public void setBean(Object bean) {
this.bean = bean;
if (bean != null) {
beanClass = bean.getClass();
}
}
/**
* Set the class of the bean that the generated map should support.
* You must call either this method or {@link #setBeanClass} before {@link #create}.
*
* @param beanClass the class of the bean
*/
public void setBeanClass(Class beanClass) {
this.beanClass = beanClass;
}
/**
* Limit the properties reflected by the generated map.
*
* @param require any combination of {@link #REQUIRE_GETTER} and
* {@link #REQUIRE_SETTER}; default is zero (any property allowed)
*/
public void setRequire(int require) {
this.require = require;
}
/**
* Title: getDefaultClassLoader
* Description:
.
*
* @see org.springframework.cglib.core.AbstractClassGenerator#getDefaultClassLoader()
*/
@Override
protected ClassLoader getDefaultClassLoader() {
return beanClass.getClassLoader();
}
/**
* Title: getProtectionDomain
* Description:
.
*
* @see org.springframework.cglib.core.AbstractClassGenerator#getProtectionDomain()
*/
@Override
protected ProtectionDomain getProtectionDomain() {
return ReflectUtils.getProtectionDomain(beanClass);
}
/**
* Create a new instance of the BeanMap
. An existing
* generated class will be reused if possible.
*
*/
public BaseBeanMap create() {
if (beanClass == null) {
throw new IllegalArgumentException("Class of bean unknown");
}
setNamePrefix(beanClass.getName());
BeanMapKey key = new BeanMapKey(beanClass, require);
return (BaseBeanMap) super.create(key);
}
/**
* Title: generateClass
* Description:
.
*
* @param v the v
* @throws Exception the exception
* @see org.springframework.cglib.core.ClassGenerator#generateClass(org.springframework.asm.ClassVisitor)
*/
@Override
public void generateClass(ClassVisitor v) throws Exception {
new BeanMapEmitter(v, getClassName(), beanClass, require);
}
/**
* Title: firstInstance
* Description:
.
*
* @param type the type
* @see org.springframework.cglib.core.AbstractClassGenerator#firstInstance(java.lang.Class)
*/
@Override
protected Object firstInstance(Class type) {
return ((BeanMap) ReflectUtils.newInstance(type)).newInstance(bean);
}
/**
* Title: nextInstance
* Description:
.
*
* @param instance the instance
* @see org.springframework.cglib.core.AbstractClassGenerator#nextInstance(java.lang.Object)
*/
@Override
protected Object nextInstance(Object instance) {
return ((BeanMap) instance).newInstance(bean);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy