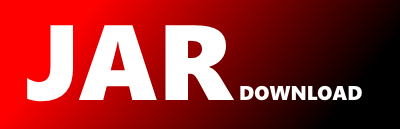
org.macrocloud.kernel.toolkit.convert.StringToEnumConverter Maven / Gradle / Ivy
package org.macrocloud.kernel.toolkit.convert;
import com.fasterxml.jackson.annotation.JsonCreator;
import lombok.extern.slf4j.Slf4j;
import org.macrocloud.kernel.toolkit.utils.ConvertUtil;
import org.macrocloud.kernel.toolkit.utils.StringUtil;
import org.springframework.core.convert.TypeDescriptor;
import org.springframework.core.convert.converter.ConditionalGenericConverter;
import org.springframework.lang.Nullable;
import java.lang.reflect.AccessibleObject;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
/**
* 接收参数 同 jackson String -》 Enum 转换.
*
* @author macro
*/
/** The Constant log. */
/** The Constant log. */
@Slf4j
public class StringToEnumConverter implements ConditionalGenericConverter {
/** 缓存 Enum 类信息,提供性能. */
private static final ConcurrentMap, AccessibleObject> ENUM_CACHE_MAP = new ConcurrentHashMap<>(8);
/**
* Gets the annotation.
*
* @param clazz the clazz
* @return the annotation
*/
@Nullable
private static AccessibleObject getAnnotation(Class> clazz) {
Set accessibleObjects = new HashSet<>();
// JsonCreator METHOD, CONSTRUCTOR
Constructor>[] constructors = clazz.getConstructors();
Collections.addAll(accessibleObjects, constructors);
// methods
Method[] methods = clazz.getDeclaredMethods();
Collections.addAll(accessibleObjects, methods);
for (AccessibleObject accessibleObject : accessibleObjects) {
// 复用 jackson 的 JsonCreator注解
JsonCreator jsonCreator = accessibleObject.getAnnotation(JsonCreator.class);
if (jsonCreator != null && JsonCreator.Mode.DISABLED != jsonCreator.mode()) {
accessibleObject.setAccessible(true);
return accessibleObject;
}
}
return null;
}
/**
* Title: matches
* Description:
.
*
* @param sourceType the source type
* @param targetType the target type
* @return true, if successful
* @see org.springframework.core.convert.converter.ConditionalConverter#matches(org.springframework.core.convert.TypeDescriptor, org.springframework.core.convert.TypeDescriptor)
*/
@Override
public boolean matches(TypeDescriptor sourceType, TypeDescriptor targetType) {
return true;
}
/**
* Title: getConvertibleTypes
* Description:
.
*
* @return the convertible types
* @see org.springframework.core.convert.converter.GenericConverter#getConvertibleTypes()
*/
@Override
public Set getConvertibleTypes() {
return Collections.singleton(new ConvertiblePair(String.class, Enum.class));
}
/**
* Title: convert
* Description:
.
*
* @param source the source
* @param sourceType the source type
* @param targetType the target type
* @return the object
* @see org.springframework.core.convert.converter.GenericConverter#convert(java.lang.Object, org.springframework.core.convert.TypeDescriptor, org.springframework.core.convert.TypeDescriptor)
*/
@Nullable
@Override
public Object convert(@Nullable Object source, TypeDescriptor sourceType, TypeDescriptor targetType) {
if (StringUtil.isBlank((String) source)) {
return null;
}
Class> clazz = targetType.getType();
AccessibleObject accessibleObject = ENUM_CACHE_MAP.computeIfAbsent(clazz, StringToEnumConverter::getAnnotation);
String value = ((String) source).trim();
// 如果为null,走默认的转换
if (accessibleObject == null) {
return valueOf(clazz, value);
}
try {
return StringToEnumConverter.invoke(clazz, accessibleObject, value);
} catch (Exception e) {
log.error(e.getMessage(), e);
}
return null;
}
/**
* Value of.
*
* @param the generic type
* @param clazz the clazz
* @param value the value
* @return the t
*/
@SuppressWarnings("unchecked")
private static > T valueOf(Class> clazz, String value){
return Enum.valueOf((Class) clazz, value);
}
/**
* Invoke.
*
* @param clazz the clazz
* @param accessibleObject the accessible object
* @param value the value
* @return the object
* @throws IllegalAccessException the illegal access exception
* @throws InvocationTargetException the invocation target exception
* @throws InstantiationException the instantiation exception
*/
@Nullable
private static Object invoke(Class> clazz, AccessibleObject accessibleObject, String value)
throws IllegalAccessException, InvocationTargetException, InstantiationException {
if (accessibleObject instanceof Constructor) {
Constructor constructor = (Constructor) accessibleObject;
Class> paramType = constructor.getParameterTypes()[0];
// 类型转换
Object object = ConvertUtil.convert(value, paramType);
return constructor.newInstance(object);
}
if (accessibleObject instanceof Method) {
Method method = (Method) accessibleObject;
Class> paramType = method.getParameterTypes()[0];
// 类型转换
Object object = ConvertUtil.convert(value, paramType);
return method.invoke(clazz, object);
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy