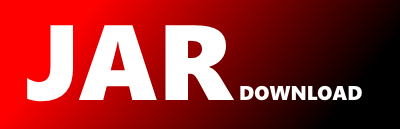
org.macrocloud.kernel.toolkit.support.Try Maven / Gradle / Ivy
package org.macrocloud.kernel.toolkit.support;
import org.macrocloud.kernel.toolkit.utils.Exceptions;
import org.springframework.lang.Nullable;
import java.util.Objects;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* Lambda 受检异常处理
* https://segmentfault.com/a/1190000007832130
*
*/
public class Try {
/**
* Of.
*
* @param the generic type
* @param the generic type
* @param mapper the mapper
* @return the function
*/
public static Function of(UncheckedFunction mapper) {
Objects.requireNonNull(mapper);
return t -> {
try {
return mapper.apply(t);
} catch (Exception e) {
throw Exceptions.unchecked(e);
}
};
}
/**
* Of.
*
* @param the generic type
* @param mapper the mapper
* @return the consumer
*/
public static Consumer of(UncheckedConsumer mapper) {
Objects.requireNonNull(mapper);
return t -> {
try {
mapper.accept(t);
} catch (Exception e) {
throw Exceptions.unchecked(e);
}
};
}
/**
* Of.
*
* @param the generic type
* @param mapper the mapper
* @return the supplier
*/
public static Supplier of(UncheckedSupplier mapper) {
Objects.requireNonNull(mapper);
return () -> {
try {
return mapper.get();
} catch (Exception e) {
throw Exceptions.unchecked(e);
}
};
}
/**
* The Interface UncheckedFunction.
*
* @param the generic type
* @param the generic type
*/
@FunctionalInterface
public interface UncheckedFunction {
/**
* apply.
*
* @param t the t
* @return the r
* @throws Exception the exception
*/
@Nullable
R apply(@Nullable T t) throws Exception;
}
/**
* The Interface UncheckedConsumer.
*
* @param the generic type
*/
@FunctionalInterface
public interface UncheckedConsumer {
/**
* accept.
*
* @param t the t
* @throws Exception the exception
*/
@Nullable
void accept(@Nullable T t) throws Exception;
}
/**
* The Interface UncheckedSupplier.
*
* @param the generic type
*/
@FunctionalInterface
public interface UncheckedSupplier {
/**
* get.
*
* @return the t
* @throws Exception the exception
*/
@Nullable
T get() throws Exception;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy