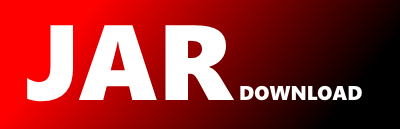
org.macrocloud.kernel.toolkit.tuple.KeyPair Maven / Gradle / Ivy
package org.macrocloud.kernel.toolkit.tuple;
import lombok.RequiredArgsConstructor;
import org.macrocloud.kernel.toolkit.utils.RsaUtil;
import java.security.PrivateKey;
import java.security.PublicKey;
// TODO: Auto-generated Javadoc
/**
* rsa 的 key pair 封装.
*
* @author macro
*/
@RequiredArgsConstructor
public class KeyPair {
/** The key pair. */
private final java.security.KeyPair keyPair;
/**
* Gets the public.
*
* @return PublicKey
*/
public PublicKey getPublic() {
return keyPair.getPublic();
}
/**
* Gets the private.
*
* @return PrivateKey
*/
public PrivateKey getPrivate() {
return keyPair.getPrivate();
}
/**
* Gets the public bytes.
*
* @return byte[]
*/
public byte[] getPublicBytes() {
return this.getPublic().getEncoded();
}
/**
* Gets the private bytes.
*
* @return byte[]
*/
public byte[] getPrivateBytes() {
return this.getPrivate().getEncoded();
}
/**
* Gets the public base 64.
*
* @return String
*/
public String getPublicBase64() {
return RsaUtil.getKeyString(this.getPublic());
}
/**
* Gets the private base 64.
*
* @return String
*/
public String getPrivateBase64() {
return RsaUtil.getKeyString(this.getPrivate());
}
/**
* Title: toString
* Description:
* @return String
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return "PublicKey=" + this.getPublicBase64() + '\n' + "PrivateKey=" + this.getPrivateBase64();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy