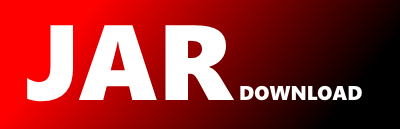
org.macrocloud.kernel.toolkit.utils.IoUtil Maven / Gradle / Ivy
package org.macrocloud.kernel.toolkit.utils;
import org.springframework.lang.Nullable;
import java.io.*;
import java.nio.charset.Charset;
/**
* 流工具类.
*
* @author macro
*/
public class IoUtil extends org.springframework.util.StreamUtils {
/**
* closeQuietly.
*
* @param closeable 自动关闭
*/
public static void closeQuietly(@Nullable Closeable closeable) {
if (closeable == null) {
return;
}
if (closeable instanceof Flushable) {
try {
((Flushable) closeable).flush();
} catch (IOException ignored) {
// ignore
}
}
try {
closeable.close();
} catch (IOException ignored) {
// ignore
}
}
/**
* InputStream to String utf-8.
*
* @param input the InputStream
to read from
* @return the requested String
*/
public static String readToString(InputStream input) {
return readToString(input, Charsets.UTF_8);
}
/**
* InputStream to String.
*
* @param input the InputStream
to read from
* @param charset the Charset
* @return the requested String
*/
public static String readToString(@Nullable InputStream input, Charset charset) {
try {
return IoUtil.copyToString(input, charset);
} catch (IOException e) {
throw Exceptions.unchecked(e);
} finally {
IoUtil.closeQuietly(input);
}
}
/**
* Read to byte array.
*
* @param input the input
* @return the byte[]
*/
public static byte[] readToByteArray(@Nullable InputStream input) {
try {
return IoUtil.copyToByteArray(input);
} catch (IOException e) {
throw Exceptions.unchecked(e);
} finally {
IoUtil.closeQuietly(input);
}
}
/**
* Writes chars from a String
to bytes on an
* OutputStream
using the specified character encoding.
*
* This method uses {@link String#getBytes(String)}.
*
*
* @param data the String
to write, null ignored
* @param output the OutputStream
to write to
* @param encoding the encoding to use, null means platform default
* @throws IOException if an I/O error occurs
* @throws NullPointerException if output is null
*/
public static void write(@Nullable final String data, final OutputStream output, final Charset encoding) throws IOException {
if (data != null) {
output.write(data.getBytes(encoding));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy