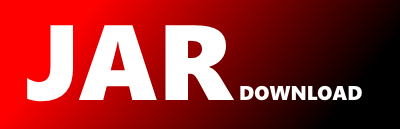
org.macrocloud.kernel.toolkit.utils.Unchecked Maven / Gradle / Ivy
package org.macrocloud.kernel.toolkit.utils;
import org.macrocloud.kernel.toolkit.function.*;
import java.util.Comparator;
import java.util.Objects;
import java.util.concurrent.Callable;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* Lambda 受检异常处理
*
*
* https://segmentfault.com/a/1190000007832130
* https://github.com/jOOQ/jOOL
*
*
* @author macro
*/
public class Unchecked {
/**
* Function.
*
* @param the generic type
* @param the generic type
* @param mapper the mapper
* @return the function
*/
public static Function function(CheckedFunction mapper) {
Objects.requireNonNull(mapper);
return t -> {
try {
return mapper.apply(t);
} catch (Throwable e) {
throw Exceptions.unchecked(e);
}
};
}
/**
* Consumer.
*
* @param the generic type
* @param mapper the mapper
* @return the consumer
*/
public static Consumer consumer(CheckedConsumer mapper) {
Objects.requireNonNull(mapper);
return t -> {
try {
mapper.accept(t);
} catch (Throwable e) {
throw Exceptions.unchecked(e);
}
};
}
/**
* Supplier.
*
* @param the generic type
* @param mapper the mapper
* @return the supplier
*/
public static Supplier supplier(CheckedSupplier mapper) {
Objects.requireNonNull(mapper);
return () -> {
try {
return mapper.get();
} catch (Throwable e) {
throw Exceptions.unchecked(e);
}
};
}
/**
* Runnable.
*
* @param runnable the runnable
* @return the runnable
*/
public static Runnable runnable(CheckedRunnable runnable) {
Objects.requireNonNull(runnable);
return () -> {
try {
runnable.run();
} catch (Throwable e) {
throw Exceptions.unchecked(e);
}
};
}
/**
* Callable.
*
* @param the generic type
* @param callable the callable
* @return the callable
*/
public static Callable callable(CheckedCallable callable) {
Objects.requireNonNull(callable);
return () -> {
try {
return callable.call();
} catch (Throwable e) {
throw Exceptions.unchecked(e);
}
};
}
/**
* Comparator.
*
* @param the generic type
* @param comparator the comparator
* @return the comparator
*/
public static Comparator comparator(CheckedComparator comparator) {
Objects.requireNonNull(comparator);
return (T o1, T o2) -> {
try {
return comparator.compare(o1, o2);
} catch (Throwable e) {
throw Exceptions.unchecked(e);
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy