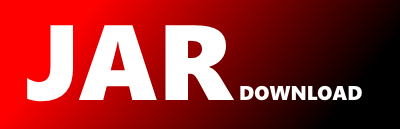
org.madlonkay.supertmxmerge.gui.ProgressWindow Maven / Gradle / Ivy
/*
* Copyright (C) 2013 Aaron Madlon-Kay .
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston,
* MA 02110-1301 USA
*/
package org.madlonkay.supertmxmerge.gui;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JFrame;
import javax.swing.SwingUtilities;
import javax.swing.Timer;
import org.madlonkay.supertmxmerge.util.GuiUtil;
import org.madlonkay.supertmxmerge.util.LocString;
/**
*
* @author Aaron Madlon-Kay
*/
public class ProgressWindow extends javax.swing.JFrame implements ActionListener {
private final Timer timer;
private final int millisToPopup = 2000;
private final int millisToDecidePopup = 500;
private boolean mustPopUp = false;
private boolean maxIsSet = false;
/**
* Creates new form ProgressWindow
*/
public ProgressWindow() {
initComponents();
timer = new Timer(millisToDecidePopup, this);
timer.setRepeats(false);
timer.start();
}
public void setMaximum(int max) {
maxIsSet = true;
progressBar.setMaximum(max);
}
public void setValue(int value) {
if (!maxIsSet) {
throw new UnsupportedOperationException("Must set maximum before setting value.");
}
progressBar.setIndeterminate(false);
progressBar.setValue(value);
}
public void setMustPopup(boolean mustPopUp) {
this.mustPopUp = mustPopUp;
}
public void setMessage(String text) {
label.setText(text);
}
@Override
public void actionPerformed(ActionEvent e) {
// Returning from the timer will be on a different thread,
// so queue this up so as to prevent spurious exceptions.
final JFrame popup = this;
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
if (shouldShowPopup()) {
GuiUtil.displayWindowCentered(popup);
}
}
});
}
private boolean shouldShowPopup() {
if (mustPopUp || progressBar.isIndeterminate() || progressBar.getValue() == 0) {
return true;
}
if (progressBar.getValue() == progressBar.getMaximum()) {
return false;
}
int min = progressBar.getMinimum();
int max = progressBar.getMaximum();
int current = progressBar.getValue();
int required = (max - min) / (current - min) * timer.getInitialDelay();
return required > millisToPopup;
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
filler1 = new javax.swing.Box.Filler(new java.awt.Dimension(20, 0), new java.awt.Dimension(20, 0), new java.awt.Dimension(20, 0));
filler3 = new javax.swing.Box.Filler(new java.awt.Dimension(0, 10), new java.awt.Dimension(0, 10), new java.awt.Dimension(0, 10));
filler2 = new javax.swing.Box.Filler(new java.awt.Dimension(20, 0), new java.awt.Dimension(20, 0), new java.awt.Dimension(20, 0));
filler4 = new javax.swing.Box.Filler(new java.awt.Dimension(0, 10), new java.awt.Dimension(0, 10), new java.awt.Dimension(0, 10));
jPanel1 = new javax.swing.JPanel();
label = new javax.swing.JLabel();
progressBar = new javax.swing.JProgressBar();
setDefaultCloseOperation(javax.swing.WindowConstants.DO_NOTHING_ON_CLOSE);
setTitle(LocString.get("STM_PROGRESS_WINDOW_TITLE")); // NOI18N
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
formWindowClosing(evt);
}
});
getContentPane().add(filler1, java.awt.BorderLayout.WEST);
getContentPane().add(filler3, java.awt.BorderLayout.NORTH);
getContentPane().add(filler2, java.awt.BorderLayout.EAST);
getContentPane().add(filler4, java.awt.BorderLayout.PAGE_END);
jPanel1.setLayout(new java.awt.GridLayout(0, 1));
label.setText(LocString.get("STM_PROGRESS_WINDOW_LABEL")); // NOI18N
jPanel1.add(label);
progressBar.setIndeterminate(true);
progressBar.setPreferredSize(new java.awt.Dimension(300, 14));
jPanel1.add(progressBar);
getContentPane().add(jPanel1, java.awt.BorderLayout.CENTER);
pack();
}// //GEN-END:initComponents
private void formWindowClosing(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_formWindowClosing
// Do nothing. Don't allow user to close.
}//GEN-LAST:event_formWindowClosing
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.Box.Filler filler1;
private javax.swing.Box.Filler filler2;
private javax.swing.Box.Filler filler3;
private javax.swing.Box.Filler filler4;
private javax.swing.JPanel jPanel1;
private javax.swing.JLabel label;
private javax.swing.JProgressBar progressBar;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy