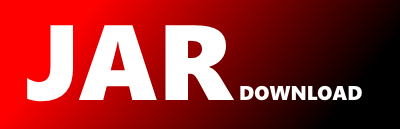
org.mandas.docker.client.messages.ImmutableAttachedNetwork Maven / Gradle / Ivy
package org.mandas.docker.client.messages;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import org.mandas.docker.Nullable;
/**
* Immutable implementation of {@link AttachedNetwork}.
*
* Use the builder to create immutable instances:
* {@code ImmutableAttachedNetwork.builder()}.
*/
@SuppressWarnings({"all"})
final class ImmutableAttachedNetwork implements AttachedNetwork {
private final @Nullable List aliases;
private final @Nullable String networkId;
private final String endpointId;
private final String gateway;
private final String ipAddress;
private final Integer ipPrefixLen;
private final String ipv6Gateway;
private final String globalIPv6Address;
private final Integer globalIPv6PrefixLen;
private final String macAddress;
private ImmutableAttachedNetwork(
@Nullable List aliases,
@Nullable String networkId,
String endpointId,
String gateway,
String ipAddress,
Integer ipPrefixLen,
String ipv6Gateway,
String globalIPv6Address,
Integer globalIPv6PrefixLen,
String macAddress) {
this.aliases = aliases;
this.networkId = networkId;
this.endpointId = endpointId;
this.gateway = gateway;
this.ipAddress = ipAddress;
this.ipPrefixLen = ipPrefixLen;
this.ipv6Gateway = ipv6Gateway;
this.globalIPv6Address = globalIPv6Address;
this.globalIPv6PrefixLen = globalIPv6PrefixLen;
this.macAddress = macAddress;
}
/**
* @return The value of the {@code aliases} attribute
*/
@JsonProperty("Aliases")
@Override
public @Nullable List aliases() {
return aliases;
}
/**
* @return The value of the {@code networkId} attribute
*/
@JsonProperty("NetworkID")
@Override
public @Nullable String networkId() {
return networkId;
}
/**
* @return The value of the {@code endpointId} attribute
*/
@JsonProperty("EndpointID")
@Override
public String endpointId() {
return endpointId;
}
/**
* @return The value of the {@code gateway} attribute
*/
@JsonProperty("Gateway")
@Override
public String gateway() {
return gateway;
}
/**
* @return The value of the {@code ipAddress} attribute
*/
@JsonProperty("IPAddress")
@Override
public String ipAddress() {
return ipAddress;
}
/**
* @return The value of the {@code ipPrefixLen} attribute
*/
@JsonProperty("IPPrefixLen")
@Override
public Integer ipPrefixLen() {
return ipPrefixLen;
}
/**
* @return The value of the {@code ipv6Gateway} attribute
*/
@JsonProperty("IPv6Gateway")
@Override
public String ipv6Gateway() {
return ipv6Gateway;
}
/**
* @return The value of the {@code globalIPv6Address} attribute
*/
@JsonProperty("GlobalIPv6Address")
@Override
public String globalIPv6Address() {
return globalIPv6Address;
}
/**
* @return The value of the {@code globalIPv6PrefixLen} attribute
*/
@JsonProperty("GlobalIPv6PrefixLen")
@Override
public Integer globalIPv6PrefixLen() {
return globalIPv6PrefixLen;
}
/**
* @return The value of the {@code macAddress} attribute
*/
@JsonProperty("MacAddress")
@Override
public String macAddress() {
return macAddress;
}
/**
* Copy the current immutable object with elements that replace the content of {@link AttachedNetwork#aliases() aliases}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAttachedNetwork withAliases(@Nullable String... elements) {
if (elements == null) {
return new ImmutableAttachedNetwork(
null,
this.networkId,
this.endpointId,
this.gateway,
this.ipAddress,
this.ipPrefixLen,
this.ipv6Gateway,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.macAddress);
}
@Nullable List newValue = Arrays.asList(elements) == null ? null : createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableAttachedNetwork(
newValue,
this.networkId,
this.endpointId,
this.gateway,
this.ipAddress,
this.ipPrefixLen,
this.ipv6Gateway,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.macAddress);
}
/**
* Copy the current immutable object with elements that replace the content of {@link AttachedNetwork#aliases() aliases}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of aliases elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableAttachedNetwork withAliases(@Nullable Iterable elements) {
if (this.aliases == elements) return this;
@Nullable List newValue = elements == null ? null : createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableAttachedNetwork(
newValue,
this.networkId,
this.endpointId,
this.gateway,
this.ipAddress,
this.ipPrefixLen,
this.ipv6Gateway,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.macAddress);
}
/**
* Copy the current immutable object by setting a value for the {@link AttachedNetwork#networkId() networkId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for networkId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableAttachedNetwork withNetworkId(@Nullable String value) {
if (Objects.equals(this.networkId, value)) return this;
return new ImmutableAttachedNetwork(
this.aliases,
value,
this.endpointId,
this.gateway,
this.ipAddress,
this.ipPrefixLen,
this.ipv6Gateway,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.macAddress);
}
/**
* Copy the current immutable object by setting a value for the {@link AttachedNetwork#endpointId() endpointId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for endpointId
* @return A modified copy of the {@code this} object
*/
public final ImmutableAttachedNetwork withEndpointId(String value) {
String newValue = Objects.requireNonNull(value, "endpointId");
if (this.endpointId.equals(newValue)) return this;
return new ImmutableAttachedNetwork(
this.aliases,
this.networkId,
newValue,
this.gateway,
this.ipAddress,
this.ipPrefixLen,
this.ipv6Gateway,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.macAddress);
}
/**
* Copy the current immutable object by setting a value for the {@link AttachedNetwork#gateway() gateway} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for gateway
* @return A modified copy of the {@code this} object
*/
public final ImmutableAttachedNetwork withGateway(String value) {
String newValue = Objects.requireNonNull(value, "gateway");
if (this.gateway.equals(newValue)) return this;
return new ImmutableAttachedNetwork(
this.aliases,
this.networkId,
this.endpointId,
newValue,
this.ipAddress,
this.ipPrefixLen,
this.ipv6Gateway,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.macAddress);
}
/**
* Copy the current immutable object by setting a value for the {@link AttachedNetwork#ipAddress() ipAddress} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for ipAddress
* @return A modified copy of the {@code this} object
*/
public final ImmutableAttachedNetwork withIpAddress(String value) {
String newValue = Objects.requireNonNull(value, "ipAddress");
if (this.ipAddress.equals(newValue)) return this;
return new ImmutableAttachedNetwork(
this.aliases,
this.networkId,
this.endpointId,
this.gateway,
newValue,
this.ipPrefixLen,
this.ipv6Gateway,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.macAddress);
}
/**
* Copy the current immutable object by setting a value for the {@link AttachedNetwork#ipPrefixLen() ipPrefixLen} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for ipPrefixLen
* @return A modified copy of the {@code this} object
*/
public final ImmutableAttachedNetwork withIpPrefixLen(Integer value) {
Integer newValue = Objects.requireNonNull(value, "ipPrefixLen");
if (this.ipPrefixLen.equals(newValue)) return this;
return new ImmutableAttachedNetwork(
this.aliases,
this.networkId,
this.endpointId,
this.gateway,
this.ipAddress,
newValue,
this.ipv6Gateway,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.macAddress);
}
/**
* Copy the current immutable object by setting a value for the {@link AttachedNetwork#ipv6Gateway() ipv6Gateway} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for ipv6Gateway
* @return A modified copy of the {@code this} object
*/
public final ImmutableAttachedNetwork withIpv6Gateway(String value) {
String newValue = Objects.requireNonNull(value, "ipv6Gateway");
if (this.ipv6Gateway.equals(newValue)) return this;
return new ImmutableAttachedNetwork(
this.aliases,
this.networkId,
this.endpointId,
this.gateway,
this.ipAddress,
this.ipPrefixLen,
newValue,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.macAddress);
}
/**
* Copy the current immutable object by setting a value for the {@link AttachedNetwork#globalIPv6Address() globalIPv6Address} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for globalIPv6Address
* @return A modified copy of the {@code this} object
*/
public final ImmutableAttachedNetwork withGlobalIPv6Address(String value) {
String newValue = Objects.requireNonNull(value, "globalIPv6Address");
if (this.globalIPv6Address.equals(newValue)) return this;
return new ImmutableAttachedNetwork(
this.aliases,
this.networkId,
this.endpointId,
this.gateway,
this.ipAddress,
this.ipPrefixLen,
this.ipv6Gateway,
newValue,
this.globalIPv6PrefixLen,
this.macAddress);
}
/**
* Copy the current immutable object by setting a value for the {@link AttachedNetwork#globalIPv6PrefixLen() globalIPv6PrefixLen} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for globalIPv6PrefixLen
* @return A modified copy of the {@code this} object
*/
public final ImmutableAttachedNetwork withGlobalIPv6PrefixLen(Integer value) {
Integer newValue = Objects.requireNonNull(value, "globalIPv6PrefixLen");
if (this.globalIPv6PrefixLen.equals(newValue)) return this;
return new ImmutableAttachedNetwork(
this.aliases,
this.networkId,
this.endpointId,
this.gateway,
this.ipAddress,
this.ipPrefixLen,
this.ipv6Gateway,
this.globalIPv6Address,
newValue,
this.macAddress);
}
/**
* Copy the current immutable object by setting a value for the {@link AttachedNetwork#macAddress() macAddress} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for macAddress
* @return A modified copy of the {@code this} object
*/
public final ImmutableAttachedNetwork withMacAddress(String value) {
String newValue = Objects.requireNonNull(value, "macAddress");
if (this.macAddress.equals(newValue)) return this;
return new ImmutableAttachedNetwork(
this.aliases,
this.networkId,
this.endpointId,
this.gateway,
this.ipAddress,
this.ipPrefixLen,
this.ipv6Gateway,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableAttachedNetwork} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableAttachedNetwork
&& equalTo(0, (ImmutableAttachedNetwork) another);
}
private boolean equalTo(int synthetic, ImmutableAttachedNetwork another) {
return Objects.equals(aliases, another.aliases)
&& Objects.equals(networkId, another.networkId)
&& endpointId.equals(another.endpointId)
&& gateway.equals(another.gateway)
&& ipAddress.equals(another.ipAddress)
&& ipPrefixLen.equals(another.ipPrefixLen)
&& ipv6Gateway.equals(another.ipv6Gateway)
&& globalIPv6Address.equals(another.globalIPv6Address)
&& globalIPv6PrefixLen.equals(another.globalIPv6PrefixLen)
&& macAddress.equals(another.macAddress);
}
/**
* Computes a hash code from attributes: {@code aliases}, {@code networkId}, {@code endpointId}, {@code gateway}, {@code ipAddress}, {@code ipPrefixLen}, {@code ipv6Gateway}, {@code globalIPv6Address}, {@code globalIPv6PrefixLen}, {@code macAddress}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(aliases);
h += (h << 5) + Objects.hashCode(networkId);
h += (h << 5) + endpointId.hashCode();
h += (h << 5) + gateway.hashCode();
h += (h << 5) + ipAddress.hashCode();
h += (h << 5) + ipPrefixLen.hashCode();
h += (h << 5) + ipv6Gateway.hashCode();
h += (h << 5) + globalIPv6Address.hashCode();
h += (h << 5) + globalIPv6PrefixLen.hashCode();
h += (h << 5) + macAddress.hashCode();
return h;
}
/**
* Prints the immutable value {@code AttachedNetwork} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "AttachedNetwork{"
+ "aliases=" + aliases
+ ", networkId=" + networkId
+ ", endpointId=" + endpointId
+ ", gateway=" + gateway
+ ", ipAddress=" + ipAddress
+ ", ipPrefixLen=" + ipPrefixLen
+ ", ipv6Gateway=" + ipv6Gateway
+ ", globalIPv6Address=" + globalIPv6Address
+ ", globalIPv6PrefixLen=" + globalIPv6PrefixLen
+ ", macAddress=" + macAddress
+ "}";
}
/**
* Creates an immutable copy of a {@link AttachedNetwork} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable AttachedNetwork instance
*/
public static ImmutableAttachedNetwork copyOf(AttachedNetwork instance) {
if (instance instanceof ImmutableAttachedNetwork) {
return (ImmutableAttachedNetwork) instance;
}
return ImmutableAttachedNetwork.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableAttachedNetwork ImmutableAttachedNetwork}.
*
* ImmutableAttachedNetwork.builder()
* .aliases(List<String> | null) // nullable {@link AttachedNetwork#aliases() aliases}
* .networkId(String | null) // nullable {@link AttachedNetwork#networkId() networkId}
* .endpointId(String) // required {@link AttachedNetwork#endpointId() endpointId}
* .gateway(String) // required {@link AttachedNetwork#gateway() gateway}
* .ipAddress(String) // required {@link AttachedNetwork#ipAddress() ipAddress}
* .ipPrefixLen(Integer) // required {@link AttachedNetwork#ipPrefixLen() ipPrefixLen}
* .ipv6Gateway(String) // required {@link AttachedNetwork#ipv6Gateway() ipv6Gateway}
* .globalIPv6Address(String) // required {@link AttachedNetwork#globalIPv6Address() globalIPv6Address}
* .globalIPv6PrefixLen(Integer) // required {@link AttachedNetwork#globalIPv6PrefixLen() globalIPv6PrefixLen}
* .macAddress(String) // required {@link AttachedNetwork#macAddress() macAddress}
* .build();
*
* @return A new ImmutableAttachedNetwork builder
*/
public static ImmutableAttachedNetwork.Builder builder() {
return new ImmutableAttachedNetwork.Builder();
}
/**
* Builds instances of type {@link ImmutableAttachedNetwork ImmutableAttachedNetwork}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder {
private static final long INIT_BIT_ENDPOINT_ID = 0x1L;
private static final long INIT_BIT_GATEWAY = 0x2L;
private static final long INIT_BIT_IP_ADDRESS = 0x4L;
private static final long INIT_BIT_IP_PREFIX_LEN = 0x8L;
private static final long INIT_BIT_IPV6_GATEWAY = 0x10L;
private static final long INIT_BIT_GLOBAL_I_PV6_ADDRESS = 0x20L;
private static final long INIT_BIT_GLOBAL_I_PV6_PREFIX_LEN = 0x40L;
private static final long INIT_BIT_MAC_ADDRESS = 0x80L;
private long initBits = 0xffL;
private List aliases = null;
private String networkId;
private String endpointId;
private String gateway;
private String ipAddress;
private Integer ipPrefixLen;
private String ipv6Gateway;
private String globalIPv6Address;
private Integer globalIPv6PrefixLen;
private String macAddress;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code AttachedNetwork} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(AttachedNetwork instance) {
Objects.requireNonNull(instance, "instance");
@Nullable List aliasesValue = instance.aliases();
if (aliasesValue != null) {
addAllAliases(aliasesValue);
}
@Nullable String networkIdValue = instance.networkId();
if (networkIdValue != null) {
networkId(networkIdValue);
}
this.endpointId(instance.endpointId());
this.gateway(instance.gateway());
this.ipAddress(instance.ipAddress());
this.ipPrefixLen(instance.ipPrefixLen());
this.ipv6Gateway(instance.ipv6Gateway());
this.globalIPv6Address(instance.globalIPv6Address());
this.globalIPv6PrefixLen(instance.globalIPv6PrefixLen());
this.macAddress(instance.macAddress());
return this;
}
/**
* Adds one element to {@link AttachedNetwork#aliases() aliases} list.
* @param element A aliases element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder aliase(String element) {
if (this.aliases == null) {
this.aliases = new ArrayList();
}
this.aliases.add(Objects.requireNonNull(element, "aliases element"));
return this;
}
/**
* Adds elements to {@link AttachedNetwork#aliases() aliases} list.
* @param elements An array of aliases elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder aliases(String... elements) {
if (this.aliases == null) {
this.aliases = new ArrayList();
}
for (String element : elements) {
this.aliases.add(Objects.requireNonNull(element, "aliases element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link AttachedNetwork#aliases() aliases} list.
* @param elements An iterable of aliases elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Aliases")
public final Builder aliases(@Nullable Iterable elements) {
if (elements == null) {
this.aliases = null;
return this;
}
this.aliases = new ArrayList();
return addAllAliases(elements);
}
/**
* Adds elements to {@link AttachedNetwork#aliases() aliases} list.
* @param elements An iterable of aliases elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllAliases(Iterable elements) {
Objects.requireNonNull(elements, "aliases element");
if (this.aliases == null) {
this.aliases = new ArrayList();
}
for (String element : elements) {
this.aliases.add(Objects.requireNonNull(element, "aliases element"));
}
return this;
}
/**
* Initializes the value for the {@link AttachedNetwork#networkId() networkId} attribute.
* @param networkId The value for networkId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("NetworkID")
public final Builder networkId(@Nullable String networkId) {
this.networkId = networkId;
return this;
}
/**
* Initializes the value for the {@link AttachedNetwork#endpointId() endpointId} attribute.
* @param endpointId The value for endpointId
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("EndpointID")
public final Builder endpointId(String endpointId) {
this.endpointId = Objects.requireNonNull(endpointId, "endpointId");
initBits &= ~INIT_BIT_ENDPOINT_ID;
return this;
}
/**
* Initializes the value for the {@link AttachedNetwork#gateway() gateway} attribute.
* @param gateway The value for gateway
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Gateway")
public final Builder gateway(String gateway) {
this.gateway = Objects.requireNonNull(gateway, "gateway");
initBits &= ~INIT_BIT_GATEWAY;
return this;
}
/**
* Initializes the value for the {@link AttachedNetwork#ipAddress() ipAddress} attribute.
* @param ipAddress The value for ipAddress
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("IPAddress")
public final Builder ipAddress(String ipAddress) {
this.ipAddress = Objects.requireNonNull(ipAddress, "ipAddress");
initBits &= ~INIT_BIT_IP_ADDRESS;
return this;
}
/**
* Initializes the value for the {@link AttachedNetwork#ipPrefixLen() ipPrefixLen} attribute.
* @param ipPrefixLen The value for ipPrefixLen
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("IPPrefixLen")
public final Builder ipPrefixLen(Integer ipPrefixLen) {
this.ipPrefixLen = Objects.requireNonNull(ipPrefixLen, "ipPrefixLen");
initBits &= ~INIT_BIT_IP_PREFIX_LEN;
return this;
}
/**
* Initializes the value for the {@link AttachedNetwork#ipv6Gateway() ipv6Gateway} attribute.
* @param ipv6Gateway The value for ipv6Gateway
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("IPv6Gateway")
public final Builder ipv6Gateway(String ipv6Gateway) {
this.ipv6Gateway = Objects.requireNonNull(ipv6Gateway, "ipv6Gateway");
initBits &= ~INIT_BIT_IPV6_GATEWAY;
return this;
}
/**
* Initializes the value for the {@link AttachedNetwork#globalIPv6Address() globalIPv6Address} attribute.
* @param globalIPv6Address The value for globalIPv6Address
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("GlobalIPv6Address")
public final Builder globalIPv6Address(String globalIPv6Address) {
this.globalIPv6Address = Objects.requireNonNull(globalIPv6Address, "globalIPv6Address");
initBits &= ~INIT_BIT_GLOBAL_I_PV6_ADDRESS;
return this;
}
/**
* Initializes the value for the {@link AttachedNetwork#globalIPv6PrefixLen() globalIPv6PrefixLen} attribute.
* @param globalIPv6PrefixLen The value for globalIPv6PrefixLen
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("GlobalIPv6PrefixLen")
public final Builder globalIPv6PrefixLen(Integer globalIPv6PrefixLen) {
this.globalIPv6PrefixLen = Objects.requireNonNull(globalIPv6PrefixLen, "globalIPv6PrefixLen");
initBits &= ~INIT_BIT_GLOBAL_I_PV6_PREFIX_LEN;
return this;
}
/**
* Initializes the value for the {@link AttachedNetwork#macAddress() macAddress} attribute.
* @param macAddress The value for macAddress
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("MacAddress")
public final Builder macAddress(String macAddress) {
this.macAddress = Objects.requireNonNull(macAddress, "macAddress");
initBits &= ~INIT_BIT_MAC_ADDRESS;
return this;
}
/**
* Builds a new {@link ImmutableAttachedNetwork ImmutableAttachedNetwork}.
* @return An immutable instance of AttachedNetwork
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableAttachedNetwork build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableAttachedNetwork(
aliases == null ? null : createUnmodifiableList(true, aliases),
networkId,
endpointId,
gateway,
ipAddress,
ipPrefixLen,
ipv6Gateway,
globalIPv6Address,
globalIPv6PrefixLen,
macAddress);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_ENDPOINT_ID) != 0) attributes.add("endpointId");
if ((initBits & INIT_BIT_GATEWAY) != 0) attributes.add("gateway");
if ((initBits & INIT_BIT_IP_ADDRESS) != 0) attributes.add("ipAddress");
if ((initBits & INIT_BIT_IP_PREFIX_LEN) != 0) attributes.add("ipPrefixLen");
if ((initBits & INIT_BIT_IPV6_GATEWAY) != 0) attributes.add("ipv6Gateway");
if ((initBits & INIT_BIT_GLOBAL_I_PV6_ADDRESS) != 0) attributes.add("globalIPv6Address");
if ((initBits & INIT_BIT_GLOBAL_I_PV6_PREFIX_LEN) != 0) attributes.add("globalIPv6PrefixLen");
if ((initBits & INIT_BIT_MAC_ADDRESS) != 0) attributes.add("macAddress");
return "Cannot build AttachedNetwork, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}