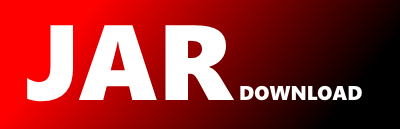
org.mandas.docker.client.messages.ImmutableContainerConfig Maven / Gradle / Ivy
package org.mandas.docker.client.messages;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import org.mandas.docker.Nullable;
import org.mandas.docker.client.ObjectMapperProvider;
/**
* Immutable implementation of {@link ContainerConfig}.
*
* Use the builder to create immutable instances:
* {@code ImmutableContainerConfig.builder()}.
*/
@SuppressWarnings({"all", "deprecation", "removal"})
final class ImmutableContainerConfig implements ContainerConfig {
private final @Nullable String hostname;
private final @Nullable String domainname;
private final @Nullable String user;
private final @Nullable Boolean attachStdin;
private final @Nullable Boolean attachStdout;
private final @Nullable Boolean attachStderr;
private final @Nullable List portSpecs;
private final @Nullable Set exposedPorts;
private final @Nullable Boolean tty;
private final @Nullable Boolean openStdin;
private final @Nullable Boolean stdinOnce;
private final @Nullable List env;
private final @Nullable List cmd;
private final @Nullable String image;
private final @Nullable Set volumes;
private final @Nullable String workingDir;
private final @Nullable List entrypoint;
private final @Nullable Boolean networkDisabled;
private final @Nullable List onBuild;
private final @Nullable Map labels;
private final @Nullable String macAddress;
private final @Nullable HostConfig hostConfig;
private final @Nullable String stopSignal;
private final @Nullable Healthcheck healthcheck;
private final @Nullable NetworkingConfig networkingConfig;
private transient final ContainerConfig.Builder toBuilder;
private ImmutableContainerConfig(
@Nullable String hostname,
@Nullable String domainname,
@Nullable String user,
@Nullable Boolean attachStdin,
@Nullable Boolean attachStdout,
@Nullable Boolean attachStderr,
@Nullable List portSpecs,
@Nullable Set exposedPorts,
@Nullable Boolean tty,
@Nullable Boolean openStdin,
@Nullable Boolean stdinOnce,
@Nullable List env,
@Nullable List cmd,
@Nullable String image,
@Nullable Set volumes,
@Nullable String workingDir,
@Nullable List entrypoint,
@Nullable Boolean networkDisabled,
@Nullable List onBuild,
@Nullable Map labels,
@Nullable String macAddress,
@Nullable HostConfig hostConfig,
@Nullable String stopSignal,
@Nullable Healthcheck healthcheck,
@Nullable NetworkingConfig networkingConfig) {
this.hostname = hostname;
this.domainname = domainname;
this.user = user;
this.attachStdin = attachStdin;
this.attachStdout = attachStdout;
this.attachStderr = attachStderr;
this.portSpecs = portSpecs;
this.exposedPorts = exposedPorts;
this.tty = tty;
this.openStdin = openStdin;
this.stdinOnce = stdinOnce;
this.env = env;
this.cmd = cmd;
this.image = image;
this.volumes = volumes;
this.workingDir = workingDir;
this.entrypoint = entrypoint;
this.networkDisabled = networkDisabled;
this.onBuild = onBuild;
this.labels = labels;
this.macAddress = macAddress;
this.hostConfig = hostConfig;
this.stopSignal = stopSignal;
this.healthcheck = healthcheck;
this.networkingConfig = networkingConfig;
this.toBuilder = Objects.requireNonNull(ContainerConfig.super.toBuilder(), "toBuilder");
}
/**
* @return The value of the {@code hostname} attribute
*/
@JsonProperty("Hostname")
@Override
public @Nullable String hostname() {
return hostname;
}
/**
* @return The value of the {@code domainname} attribute
*/
@JsonProperty("Domainname")
@Override
public @Nullable String domainname() {
return domainname;
}
/**
* @return The value of the {@code user} attribute
*/
@JsonProperty("User")
@Override
public @Nullable String user() {
return user;
}
/**
* @return The value of the {@code attachStdin} attribute
*/
@JsonProperty("AttachStdin")
@Override
public @Nullable Boolean attachStdin() {
return attachStdin;
}
/**
* @return The value of the {@code attachStdout} attribute
*/
@JsonProperty("AttachStdout")
@Override
public @Nullable Boolean attachStdout() {
return attachStdout;
}
/**
* @return The value of the {@code attachStderr} attribute
*/
@JsonProperty("AttachStderr")
@Override
public @Nullable Boolean attachStderr() {
return attachStderr;
}
/**
* @return The value of the {@code portSpecs} attribute
*/
@JsonProperty("PortSpecs")
@Override
public @Nullable List portSpecs() {
return portSpecs;
}
/**
* @return The value of the {@code exposedPorts} attribute
*/
@JsonProperty("ExposedPorts")
@JsonSerialize(using = ObjectMapperProvider.SetSerializer.class)
@JsonDeserialize(using = ObjectMapperProvider.SetDeserializer.class)
@Override
public @Nullable Set exposedPorts() {
return exposedPorts;
}
/**
* @return The value of the {@code tty} attribute
*/
@JsonProperty("Tty")
@Override
public @Nullable Boolean tty() {
return tty;
}
/**
* @return The value of the {@code openStdin} attribute
*/
@JsonProperty("OpenStdin")
@Override
public @Nullable Boolean openStdin() {
return openStdin;
}
/**
* @return The value of the {@code stdinOnce} attribute
*/
@JsonProperty("StdinOnce")
@Override
public @Nullable Boolean stdinOnce() {
return stdinOnce;
}
/**
* @return The value of the {@code env} attribute
*/
@JsonProperty("Env")
@Override
public @Nullable List env() {
return env;
}
/**
* @return The value of the {@code cmd} attribute
*/
@JsonProperty("Cmd")
@Override
public @Nullable List cmd() {
return cmd;
}
/**
* @return The value of the {@code image} attribute
*/
@JsonProperty("Image")
@Override
public @Nullable String image() {
return image;
}
/**
* @return The value of the {@code volumes} attribute
*/
@JsonProperty("Volumes")
@JsonSerialize(using = ObjectMapperProvider.SetSerializer.class)
@JsonDeserialize(using = ObjectMapperProvider.SetDeserializer.class)
@Override
public @Nullable Set volumes() {
return volumes;
}
/**
* @return The value of the {@code workingDir} attribute
*/
@JsonProperty("WorkingDir")
@Override
public @Nullable String workingDir() {
return workingDir;
}
/**
* @return The value of the {@code entrypoint} attribute
*/
@JsonProperty("Entrypoint")
@Override
public @Nullable List entrypoint() {
return entrypoint;
}
/**
* @return The value of the {@code networkDisabled} attribute
*/
@JsonProperty("NetworkDisabled")
@Override
public @Nullable Boolean networkDisabled() {
return networkDisabled;
}
/**
* @return The value of the {@code onBuild} attribute
*/
@JsonProperty("OnBuild")
@Override
public @Nullable List onBuild() {
return onBuild;
}
/**
* @return The value of the {@code labels} attribute
*/
@JsonProperty("Labels")
@Override
public @Nullable Map labels() {
return labels;
}
/**
* @return The value of the {@code macAddress} attribute
*/
@JsonProperty("MacAddress")
@Deprecated
@Override
public @Nullable String macAddress() {
return macAddress;
}
/**
* @return The value of the {@code hostConfig} attribute
*/
@JsonProperty("HostConfig")
@Override
public @Nullable HostConfig hostConfig() {
return hostConfig;
}
/**
* @return The value of the {@code stopSignal} attribute
*/
@JsonProperty("StopSignal")
@Override
public @Nullable String stopSignal() {
return stopSignal;
}
/**
* @return The value of the {@code healthcheck} attribute
*/
@JsonProperty("Healthcheck")
@Override
public @Nullable Healthcheck healthcheck() {
return healthcheck;
}
/**
* @return The value of the {@code networkingConfig} attribute
*/
@JsonProperty("NetworkingConfig")
@Override
public @Nullable NetworkingConfig networkingConfig() {
return networkingConfig;
}
/**
* @return The computed-at-construction value of the {@code toBuilder} attribute
*/
@JsonProperty("toBuilder")
@JsonIgnore
@Override
public ContainerConfig.Builder toBuilder() {
return toBuilder;
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#hostname() hostname} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for hostname (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withHostname(@Nullable String value) {
if (Objects.equals(this.hostname, value)) return this;
return new ImmutableContainerConfig(
value,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#domainname() domainname} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for domainname (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withDomainname(@Nullable String value) {
if (Objects.equals(this.domainname, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
value,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#user() user} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for user (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withUser(@Nullable String value) {
if (Objects.equals(this.user, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
value,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#attachStdin() attachStdin} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for attachStdin (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withAttachStdin(@Nullable Boolean value) {
if (Objects.equals(this.attachStdin, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
value,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#attachStdout() attachStdout} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for attachStdout (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withAttachStdout(@Nullable Boolean value) {
if (Objects.equals(this.attachStdout, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
value,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#attachStderr() attachStderr} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for attachStderr (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withAttachStderr(@Nullable Boolean value) {
if (Objects.equals(this.attachStderr, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
value,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#portSpecs() portSpecs}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withPortSpecs(@Nullable String... elements) {
if (elements == null) {
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
null,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
@Nullable List newValue = Arrays.asList(elements) == null ? null : createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
newValue,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#portSpecs() portSpecs}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of portSpecs elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withPortSpecs(@Nullable Iterable elements) {
if (this.portSpecs == elements) return this;
@Nullable List newValue = elements == null ? null : createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
newValue,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#exposedPorts() exposedPorts}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withExposedPorts(@Nullable String... elements) {
if (elements == null) {
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
null,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
@Nullable Set newValue = Arrays.asList(elements) == null ? null : createUnmodifiableSet(createSafeList(Arrays.asList(elements), true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
newValue,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#exposedPorts() exposedPorts}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of exposedPorts elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withExposedPorts(@Nullable Iterable elements) {
if (this.exposedPorts == elements) return this;
@Nullable Set newValue = elements == null ? null : createUnmodifiableSet(createSafeList(elements, true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
newValue,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#tty() tty} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for tty (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withTty(@Nullable Boolean value) {
if (Objects.equals(this.tty, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
value,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#openStdin() openStdin} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for openStdin (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withOpenStdin(@Nullable Boolean value) {
if (Objects.equals(this.openStdin, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
value,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#stdinOnce() stdinOnce} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for stdinOnce (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withStdinOnce(@Nullable Boolean value) {
if (Objects.equals(this.stdinOnce, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
value,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#env() env}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withEnv(@Nullable String... elements) {
if (elements == null) {
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
null,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
@Nullable List newValue = Arrays.asList(elements) == null ? null : createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
newValue,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#env() env}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of env elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withEnv(@Nullable Iterable elements) {
if (this.env == elements) return this;
@Nullable List newValue = elements == null ? null : createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
newValue,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#cmd() cmd}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withCmd(@Nullable String... elements) {
if (elements == null) {
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
null,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
@Nullable List newValue = Arrays.asList(elements) == null ? null : createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
newValue,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#cmd() cmd}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of cmd elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withCmd(@Nullable Iterable elements) {
if (this.cmd == elements) return this;
@Nullable List newValue = elements == null ? null : createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
newValue,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#image() image} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for image (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withImage(@Nullable String value) {
if (Objects.equals(this.image, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
value,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#volumes() volumes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withVolumes(@Nullable String... elements) {
if (elements == null) {
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
null,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
@Nullable Set newValue = Arrays.asList(elements) == null ? null : createUnmodifiableSet(createSafeList(Arrays.asList(elements), true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
newValue,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#volumes() volumes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of volumes elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withVolumes(@Nullable Iterable elements) {
if (this.volumes == elements) return this;
@Nullable Set newValue = elements == null ? null : createUnmodifiableSet(createSafeList(elements, true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
newValue,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#workingDir() workingDir} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for workingDir (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withWorkingDir(@Nullable String value) {
if (Objects.equals(this.workingDir, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
value,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#entrypoint() entrypoint}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withEntrypoint(@Nullable String... elements) {
if (elements == null) {
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
null,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
@Nullable List newValue = Arrays.asList(elements) == null ? null : createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
newValue,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#entrypoint() entrypoint}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of entrypoint elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withEntrypoint(@Nullable Iterable elements) {
if (this.entrypoint == elements) return this;
@Nullable List newValue = elements == null ? null : createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
newValue,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#networkDisabled() networkDisabled} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for networkDisabled (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withNetworkDisabled(@Nullable Boolean value) {
if (Objects.equals(this.networkDisabled, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
value,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#onBuild() onBuild}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withOnBuild(@Nullable String... elements) {
if (elements == null) {
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
null,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
@Nullable List newValue = Arrays.asList(elements) == null ? null : createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
newValue,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerConfig#onBuild() onBuild}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of onBuild elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withOnBuild(@Nullable Iterable elements) {
if (this.onBuild == elements) return this;
@Nullable List newValue = elements == null ? null : createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
newValue,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by replacing the {@link ContainerConfig#labels() labels} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the labels map
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerConfig withLabels(@Nullable Map entries) {
if (this.labels == entries) return this;
@Nullable Map newValue = entries == null ? null : createUnmodifiableMap(true, false, entries);
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
newValue,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#macAddress() macAddress} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for macAddress (can be {@code null})
* @return A modified copy of the {@code this} object
*/
@Deprecated
public final ImmutableContainerConfig withMacAddress(@Nullable String value) {
if (Objects.equals(this.macAddress, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
value,
this.hostConfig,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#hostConfig() hostConfig} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for hostConfig (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withHostConfig(@Nullable HostConfig value) {
if (this.hostConfig == value) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
value,
this.stopSignal,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#stopSignal() stopSignal} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for stopSignal (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withStopSignal(@Nullable String value) {
if (Objects.equals(this.stopSignal, value)) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
value,
this.healthcheck,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#healthcheck() healthcheck} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for healthcheck (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withHealthcheck(@Nullable Healthcheck value) {
if (this.healthcheck == value) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
value,
this.networkingConfig);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerConfig#networkingConfig() networkingConfig} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for networkingConfig (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerConfig withNetworkingConfig(@Nullable NetworkingConfig value) {
if (this.networkingConfig == value) return this;
return new ImmutableContainerConfig(
this.hostname,
this.domainname,
this.user,
this.attachStdin,
this.attachStdout,
this.attachStderr,
this.portSpecs,
this.exposedPorts,
this.tty,
this.openStdin,
this.stdinOnce,
this.env,
this.cmd,
this.image,
this.volumes,
this.workingDir,
this.entrypoint,
this.networkDisabled,
this.onBuild,
this.labels,
this.macAddress,
this.hostConfig,
this.stopSignal,
this.healthcheck,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableContainerConfig} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableContainerConfig
&& equalTo(0, (ImmutableContainerConfig) another);
}
private boolean equalTo(int synthetic, ImmutableContainerConfig another) {
return Objects.equals(hostname, another.hostname)
&& Objects.equals(domainname, another.domainname)
&& Objects.equals(user, another.user)
&& Objects.equals(attachStdin, another.attachStdin)
&& Objects.equals(attachStdout, another.attachStdout)
&& Objects.equals(attachStderr, another.attachStderr)
&& Objects.equals(portSpecs, another.portSpecs)
&& Objects.equals(exposedPorts, another.exposedPorts)
&& Objects.equals(tty, another.tty)
&& Objects.equals(openStdin, another.openStdin)
&& Objects.equals(stdinOnce, another.stdinOnce)
&& Objects.equals(env, another.env)
&& Objects.equals(cmd, another.cmd)
&& Objects.equals(image, another.image)
&& Objects.equals(volumes, another.volumes)
&& Objects.equals(workingDir, another.workingDir)
&& Objects.equals(entrypoint, another.entrypoint)
&& Objects.equals(networkDisabled, another.networkDisabled)
&& Objects.equals(onBuild, another.onBuild)
&& Objects.equals(labels, another.labels)
&& Objects.equals(macAddress, another.macAddress)
&& Objects.equals(hostConfig, another.hostConfig)
&& Objects.equals(stopSignal, another.stopSignal)
&& Objects.equals(healthcheck, another.healthcheck)
&& Objects.equals(networkingConfig, another.networkingConfig);
}
/**
* Computes a hash code from attributes: {@code hostname}, {@code domainname}, {@code user}, {@code attachStdin}, {@code attachStdout}, {@code attachStderr}, {@code portSpecs}, {@code exposedPorts}, {@code tty}, {@code openStdin}, {@code stdinOnce}, {@code env}, {@code cmd}, {@code image}, {@code volumes}, {@code workingDir}, {@code entrypoint}, {@code networkDisabled}, {@code onBuild}, {@code labels}, {@code macAddress}, {@code hostConfig}, {@code stopSignal}, {@code healthcheck}, {@code networkingConfig}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(hostname);
h += (h << 5) + Objects.hashCode(domainname);
h += (h << 5) + Objects.hashCode(user);
h += (h << 5) + Objects.hashCode(attachStdin);
h += (h << 5) + Objects.hashCode(attachStdout);
h += (h << 5) + Objects.hashCode(attachStderr);
h += (h << 5) + Objects.hashCode(portSpecs);
h += (h << 5) + Objects.hashCode(exposedPorts);
h += (h << 5) + Objects.hashCode(tty);
h += (h << 5) + Objects.hashCode(openStdin);
h += (h << 5) + Objects.hashCode(stdinOnce);
h += (h << 5) + Objects.hashCode(env);
h += (h << 5) + Objects.hashCode(cmd);
h += (h << 5) + Objects.hashCode(image);
h += (h << 5) + Objects.hashCode(volumes);
h += (h << 5) + Objects.hashCode(workingDir);
h += (h << 5) + Objects.hashCode(entrypoint);
h += (h << 5) + Objects.hashCode(networkDisabled);
h += (h << 5) + Objects.hashCode(onBuild);
h += (h << 5) + Objects.hashCode(labels);
h += (h << 5) + Objects.hashCode(macAddress);
h += (h << 5) + Objects.hashCode(hostConfig);
h += (h << 5) + Objects.hashCode(stopSignal);
h += (h << 5) + Objects.hashCode(healthcheck);
h += (h << 5) + Objects.hashCode(networkingConfig);
return h;
}
/**
* Prints the immutable value {@code ContainerConfig} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "ContainerConfig{"
+ "hostname=" + hostname
+ ", domainname=" + domainname
+ ", user=" + user
+ ", attachStdin=" + attachStdin
+ ", attachStdout=" + attachStdout
+ ", attachStderr=" + attachStderr
+ ", portSpecs=" + portSpecs
+ ", exposedPorts=" + exposedPorts
+ ", tty=" + tty
+ ", openStdin=" + openStdin
+ ", stdinOnce=" + stdinOnce
+ ", env=" + env
+ ", cmd=" + cmd
+ ", image=" + image
+ ", volumes=" + volumes
+ ", workingDir=" + workingDir
+ ", entrypoint=" + entrypoint
+ ", networkDisabled=" + networkDisabled
+ ", onBuild=" + onBuild
+ ", labels=" + labels
+ ", macAddress=" + macAddress
+ ", hostConfig=" + hostConfig
+ ", stopSignal=" + stopSignal
+ ", healthcheck=" + healthcheck
+ ", networkingConfig=" + networkingConfig
+ "}";
}
/**
* Creates an immutable copy of a {@link ContainerConfig} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ContainerConfig instance
*/
public static ImmutableContainerConfig copyOf(ContainerConfig instance) {
if (instance instanceof ImmutableContainerConfig) {
return (ImmutableContainerConfig) instance;
}
return ImmutableContainerConfig.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableContainerConfig ImmutableContainerConfig}.
*
* ImmutableContainerConfig.builder()
* .hostname(String | null) // nullable {@link ContainerConfig#hostname() hostname}
* .domainname(String | null) // nullable {@link ContainerConfig#domainname() domainname}
* .user(String | null) // nullable {@link ContainerConfig#user() user}
* .attachStdin(Boolean | null) // nullable {@link ContainerConfig#attachStdin() attachStdin}
* .attachStdout(Boolean | null) // nullable {@link ContainerConfig#attachStdout() attachStdout}
* .attachStderr(Boolean | null) // nullable {@link ContainerConfig#attachStderr() attachStderr}
* .portSpecs(List<String> | null) // nullable {@link ContainerConfig#portSpecs() portSpecs}
* .exposedPorts(Set<String> | null) // nullable {@link ContainerConfig#exposedPorts() exposedPorts}
* .tty(Boolean | null) // nullable {@link ContainerConfig#tty() tty}
* .openStdin(Boolean | null) // nullable {@link ContainerConfig#openStdin() openStdin}
* .stdinOnce(Boolean | null) // nullable {@link ContainerConfig#stdinOnce() stdinOnce}
* .env(List<String> | null) // nullable {@link ContainerConfig#env() env}
* .cmd(List<String> | null) // nullable {@link ContainerConfig#cmd() cmd}
* .image(String | null) // nullable {@link ContainerConfig#image() image}
* .volumes(Set<String> | null) // nullable {@link ContainerConfig#volumes() volumes}
* .workingDir(String | null) // nullable {@link ContainerConfig#workingDir() workingDir}
* .entrypoint(List<String> | null) // nullable {@link ContainerConfig#entrypoint() entrypoint}
* .networkDisabled(Boolean | null) // nullable {@link ContainerConfig#networkDisabled() networkDisabled}
* .onBuild(List<String> | null) // nullable {@link ContainerConfig#onBuild() onBuild}
* .labels(Map<String, String> | null) // nullable {@link ContainerConfig#labels() labels}
* .macAddress(String | null) // nullable {@link ContainerConfig#macAddress() macAddress}
* .hostConfig(org.mandas.docker.client.messages.HostConfig | null) // nullable {@link ContainerConfig#hostConfig() hostConfig}
* .stopSignal(String | null) // nullable {@link ContainerConfig#stopSignal() stopSignal}
* .healthcheck(org.mandas.docker.client.messages.Healthcheck | null) // nullable {@link ContainerConfig#healthcheck() healthcheck}
* .networkingConfig(org.mandas.docker.client.messages.NetworkingConfig | null) // nullable {@link ContainerConfig#networkingConfig() networkingConfig}
* .build();
*
* @return A new ImmutableContainerConfig builder
*/
public static ImmutableContainerConfig.Builder builder() {
return new ImmutableContainerConfig.Builder();
}
/**
* Builds instances of type {@link ImmutableContainerConfig ImmutableContainerConfig}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder implements ContainerConfig.Builder {
private String hostname;
private String domainname;
private String user;
private Boolean attachStdin;
private Boolean attachStdout;
private Boolean attachStderr;
private List portSpecs = null;
private List exposedPorts = null;
private Boolean tty;
private Boolean openStdin;
private Boolean stdinOnce;
private List env = null;
private List cmd = null;
private String image;
private List volumes = null;
private String workingDir;
private List entrypoint = null;
private Boolean networkDisabled;
private List onBuild = null;
private Map labels = null;
private String macAddress;
private HostConfig hostConfig;
private String stopSignal;
private Healthcheck healthcheck;
private NetworkingConfig networkingConfig;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ContainerConfig} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ContainerConfig instance) {
Objects.requireNonNull(instance, "instance");
@Nullable String hostnameValue = instance.hostname();
if (hostnameValue != null) {
hostname(hostnameValue);
}
@Nullable String domainnameValue = instance.domainname();
if (domainnameValue != null) {
domainname(domainnameValue);
}
@Nullable String userValue = instance.user();
if (userValue != null) {
user(userValue);
}
@Nullable Boolean attachStdinValue = instance.attachStdin();
if (attachStdinValue != null) {
attachStdin(attachStdinValue);
}
@Nullable Boolean attachStdoutValue = instance.attachStdout();
if (attachStdoutValue != null) {
attachStdout(attachStdoutValue);
}
@Nullable Boolean attachStderrValue = instance.attachStderr();
if (attachStderrValue != null) {
attachStderr(attachStderrValue);
}
@Nullable List portSpecsValue = instance.portSpecs();
if (portSpecsValue != null) {
addAllPortSpecs(portSpecsValue);
}
@Nullable Set exposedPortsValue = instance.exposedPorts();
if (exposedPortsValue != null) {
addAllExposedPorts(exposedPortsValue);
}
@Nullable Boolean ttyValue = instance.tty();
if (ttyValue != null) {
tty(ttyValue);
}
@Nullable Boolean openStdinValue = instance.openStdin();
if (openStdinValue != null) {
openStdin(openStdinValue);
}
@Nullable Boolean stdinOnceValue = instance.stdinOnce();
if (stdinOnceValue != null) {
stdinOnce(stdinOnceValue);
}
@Nullable List envValue = instance.env();
if (envValue != null) {
addAllEnv(envValue);
}
@Nullable List cmdValue = instance.cmd();
if (cmdValue != null) {
addAllCmd(cmdValue);
}
@Nullable String imageValue = instance.image();
if (imageValue != null) {
image(imageValue);
}
@Nullable Set volumesValue = instance.volumes();
if (volumesValue != null) {
addAllVolumes(volumesValue);
}
@Nullable String workingDirValue = instance.workingDir();
if (workingDirValue != null) {
workingDir(workingDirValue);
}
@Nullable List entrypointValue = instance.entrypoint();
if (entrypointValue != null) {
addAllEntrypoint(entrypointValue);
}
@Nullable Boolean networkDisabledValue = instance.networkDisabled();
if (networkDisabledValue != null) {
networkDisabled(networkDisabledValue);
}
@Nullable List onBuildValue = instance.onBuild();
if (onBuildValue != null) {
addAllOnBuild(onBuildValue);
}
@Nullable Map labelsValue = instance.labels();
if (labelsValue != null) {
putAllLabels(labelsValue);
}
@Nullable String macAddressValue = instance.macAddress();
if (macAddressValue != null) {
macAddress(macAddressValue);
}
@Nullable HostConfig hostConfigValue = instance.hostConfig();
if (hostConfigValue != null) {
hostConfig(hostConfigValue);
}
@Nullable String stopSignalValue = instance.stopSignal();
if (stopSignalValue != null) {
stopSignal(stopSignalValue);
}
@Nullable Healthcheck healthcheckValue = instance.healthcheck();
if (healthcheckValue != null) {
healthcheck(healthcheckValue);
}
@Nullable NetworkingConfig networkingConfigValue = instance.networkingConfig();
if (networkingConfigValue != null) {
networkingConfig(networkingConfigValue);
}
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#hostname() hostname} attribute.
* @param hostname The value for hostname (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Hostname")
public final Builder hostname(@Nullable String hostname) {
this.hostname = hostname;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#domainname() domainname} attribute.
* @param domainname The value for domainname (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Domainname")
public final Builder domainname(@Nullable String domainname) {
this.domainname = domainname;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#user() user} attribute.
* @param user The value for user (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("User")
public final Builder user(@Nullable String user) {
this.user = user;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#attachStdin() attachStdin} attribute.
* @param attachStdin The value for attachStdin (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("AttachStdin")
public final Builder attachStdin(@Nullable Boolean attachStdin) {
this.attachStdin = attachStdin;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#attachStdout() attachStdout} attribute.
* @param attachStdout The value for attachStdout (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("AttachStdout")
public final Builder attachStdout(@Nullable Boolean attachStdout) {
this.attachStdout = attachStdout;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#attachStderr() attachStderr} attribute.
* @param attachStderr The value for attachStderr (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("AttachStderr")
public final Builder attachStderr(@Nullable Boolean attachStderr) {
this.attachStderr = attachStderr;
return this;
}
/**
* Adds one element to {@link ContainerConfig#portSpecs() portSpecs} list.
* @param element A portSpecs element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder portSpec(String element) {
if (this.portSpecs == null) {
this.portSpecs = new ArrayList();
}
this.portSpecs.add(Objects.requireNonNull(element, "portSpecs element"));
return this;
}
/**
* Adds elements to {@link ContainerConfig#portSpecs() portSpecs} list.
* @param elements An array of portSpecs elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder portSpecs(String... elements) {
if (this.portSpecs == null) {
this.portSpecs = new ArrayList();
}
for (String element : elements) {
this.portSpecs.add(Objects.requireNonNull(element, "portSpecs element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ContainerConfig#portSpecs() portSpecs} list.
* @param elements An iterable of portSpecs elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("PortSpecs")
public final Builder portSpecs(@Nullable Iterable elements) {
if (elements == null) {
this.portSpecs = null;
return this;
}
this.portSpecs = new ArrayList();
return addAllPortSpecs(elements);
}
/**
* Adds elements to {@link ContainerConfig#portSpecs() portSpecs} list.
* @param elements An iterable of portSpecs elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllPortSpecs(Iterable elements) {
Objects.requireNonNull(elements, "portSpecs element");
if (this.portSpecs == null) {
this.portSpecs = new ArrayList();
}
for (String element : elements) {
this.portSpecs.add(Objects.requireNonNull(element, "portSpecs element"));
}
return this;
}
/**
* Adds one element to {@link ContainerConfig#exposedPorts() exposedPorts} set.
* @param element A exposedPorts element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder exposedPort(String element) {
if (this.exposedPorts == null) {
this.exposedPorts = new ArrayList();
}
this.exposedPorts.add(Objects.requireNonNull(element, "exposedPorts element"));
return this;
}
/**
* Adds elements to {@link ContainerConfig#exposedPorts() exposedPorts} set.
* @param elements An array of exposedPorts elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder exposedPorts(String... elements) {
if (this.exposedPorts == null) {
this.exposedPorts = new ArrayList();
}
for (String element : elements) {
this.exposedPorts.add(Objects.requireNonNull(element, "exposedPorts element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ContainerConfig#exposedPorts() exposedPorts} set.
* @param elements An iterable of exposedPorts elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("ExposedPorts")
@JsonSerialize(using = ObjectMapperProvider.SetSerializer.class)
@JsonDeserialize(using = ObjectMapperProvider.SetDeserializer.class)
public final Builder exposedPorts(@Nullable Iterable elements) {
if (elements == null) {
this.exposedPorts = null;
return this;
}
this.exposedPorts = new ArrayList();
return addAllExposedPorts(elements);
}
/**
* Adds elements to {@link ContainerConfig#exposedPorts() exposedPorts} set.
* @param elements An iterable of exposedPorts elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllExposedPorts(Iterable elements) {
Objects.requireNonNull(elements, "exposedPorts element");
if (this.exposedPorts == null) {
this.exposedPorts = new ArrayList();
}
for (String element : elements) {
this.exposedPorts.add(Objects.requireNonNull(element, "exposedPorts element"));
}
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#tty() tty} attribute.
* @param tty The value for tty (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Tty")
public final Builder tty(@Nullable Boolean tty) {
this.tty = tty;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#openStdin() openStdin} attribute.
* @param openStdin The value for openStdin (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("OpenStdin")
public final Builder openStdin(@Nullable Boolean openStdin) {
this.openStdin = openStdin;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#stdinOnce() stdinOnce} attribute.
* @param stdinOnce The value for stdinOnce (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("StdinOnce")
public final Builder stdinOnce(@Nullable Boolean stdinOnce) {
this.stdinOnce = stdinOnce;
return this;
}
/**
* Adds one element to {@link ContainerConfig#env() env} list.
* @param element A env element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder env(String element) {
if (this.env == null) {
this.env = new ArrayList();
}
this.env.add(Objects.requireNonNull(element, "env element"));
return this;
}
/**
* Adds elements to {@link ContainerConfig#env() env} list.
* @param elements An array of env elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder env(String... elements) {
if (this.env == null) {
this.env = new ArrayList();
}
for (String element : elements) {
this.env.add(Objects.requireNonNull(element, "env element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ContainerConfig#env() env} list.
* @param elements An iterable of env elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Env")
public final Builder env(@Nullable Iterable elements) {
if (elements == null) {
this.env = null;
return this;
}
this.env = new ArrayList();
return addAllEnv(elements);
}
/**
* Adds elements to {@link ContainerConfig#env() env} list.
* @param elements An iterable of env elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllEnv(Iterable elements) {
Objects.requireNonNull(elements, "env element");
if (this.env == null) {
this.env = new ArrayList();
}
for (String element : elements) {
this.env.add(Objects.requireNonNull(element, "env element"));
}
return this;
}
/**
* Adds one element to {@link ContainerConfig#cmd() cmd} list.
* @param element A cmd element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder cmd(String element) {
if (this.cmd == null) {
this.cmd = new ArrayList();
}
this.cmd.add(Objects.requireNonNull(element, "cmd element"));
return this;
}
/**
* Adds elements to {@link ContainerConfig#cmd() cmd} list.
* @param elements An array of cmd elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder cmd(String... elements) {
if (this.cmd == null) {
this.cmd = new ArrayList();
}
for (String element : elements) {
this.cmd.add(Objects.requireNonNull(element, "cmd element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ContainerConfig#cmd() cmd} list.
* @param elements An iterable of cmd elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Cmd")
public final Builder cmd(@Nullable Iterable elements) {
if (elements == null) {
this.cmd = null;
return this;
}
this.cmd = new ArrayList();
return addAllCmd(elements);
}
/**
* Adds elements to {@link ContainerConfig#cmd() cmd} list.
* @param elements An iterable of cmd elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllCmd(Iterable elements) {
Objects.requireNonNull(elements, "cmd element");
if (this.cmd == null) {
this.cmd = new ArrayList();
}
for (String element : elements) {
this.cmd.add(Objects.requireNonNull(element, "cmd element"));
}
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#image() image} attribute.
* @param image The value for image (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Image")
public final Builder image(@Nullable String image) {
this.image = image;
return this;
}
/**
* Adds one element to {@link ContainerConfig#volumes() volumes} set.
* @param element A volumes element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder volume(String element) {
if (this.volumes == null) {
this.volumes = new ArrayList();
}
this.volumes.add(Objects.requireNonNull(element, "volumes element"));
return this;
}
/**
* Adds elements to {@link ContainerConfig#volumes() volumes} set.
* @param elements An array of volumes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder volumes(String... elements) {
if (this.volumes == null) {
this.volumes = new ArrayList();
}
for (String element : elements) {
this.volumes.add(Objects.requireNonNull(element, "volumes element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ContainerConfig#volumes() volumes} set.
* @param elements An iterable of volumes elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Volumes")
@JsonSerialize(using = ObjectMapperProvider.SetSerializer.class)
@JsonDeserialize(using = ObjectMapperProvider.SetDeserializer.class)
public final Builder volumes(@Nullable Iterable elements) {
if (elements == null) {
this.volumes = null;
return this;
}
this.volumes = new ArrayList();
return addAllVolumes(elements);
}
/**
* Adds elements to {@link ContainerConfig#volumes() volumes} set.
* @param elements An iterable of volumes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllVolumes(Iterable elements) {
Objects.requireNonNull(elements, "volumes element");
if (this.volumes == null) {
this.volumes = new ArrayList();
}
for (String element : elements) {
this.volumes.add(Objects.requireNonNull(element, "volumes element"));
}
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#workingDir() workingDir} attribute.
* @param workingDir The value for workingDir (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("WorkingDir")
public final Builder workingDir(@Nullable String workingDir) {
this.workingDir = workingDir;
return this;
}
/**
* Adds one element to {@link ContainerConfig#entrypoint() entrypoint} list.
* @param element A entrypoint element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder entrypoint(String element) {
if (this.entrypoint == null) {
this.entrypoint = new ArrayList();
}
this.entrypoint.add(Objects.requireNonNull(element, "entrypoint element"));
return this;
}
/**
* Adds elements to {@link ContainerConfig#entrypoint() entrypoint} list.
* @param elements An array of entrypoint elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder entrypoint(String... elements) {
if (this.entrypoint == null) {
this.entrypoint = new ArrayList();
}
for (String element : elements) {
this.entrypoint.add(Objects.requireNonNull(element, "entrypoint element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ContainerConfig#entrypoint() entrypoint} list.
* @param elements An iterable of entrypoint elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Entrypoint")
public final Builder entrypoint(@Nullable Iterable elements) {
if (elements == null) {
this.entrypoint = null;
return this;
}
this.entrypoint = new ArrayList();
return addAllEntrypoint(elements);
}
/**
* Adds elements to {@link ContainerConfig#entrypoint() entrypoint} list.
* @param elements An iterable of entrypoint elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllEntrypoint(Iterable elements) {
Objects.requireNonNull(elements, "entrypoint element");
if (this.entrypoint == null) {
this.entrypoint = new ArrayList();
}
for (String element : elements) {
this.entrypoint.add(Objects.requireNonNull(element, "entrypoint element"));
}
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#networkDisabled() networkDisabled} attribute.
* @param networkDisabled The value for networkDisabled (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("NetworkDisabled")
public final Builder networkDisabled(@Nullable Boolean networkDisabled) {
this.networkDisabled = networkDisabled;
return this;
}
/**
* Adds one element to {@link ContainerConfig#onBuild() onBuild} list.
* @param element A onBuild element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder onBuild(String element) {
if (this.onBuild == null) {
this.onBuild = new ArrayList();
}
this.onBuild.add(Objects.requireNonNull(element, "onBuild element"));
return this;
}
/**
* Adds elements to {@link ContainerConfig#onBuild() onBuild} list.
* @param elements An array of onBuild elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder onBuild(String... elements) {
if (this.onBuild == null) {
this.onBuild = new ArrayList();
}
for (String element : elements) {
this.onBuild.add(Objects.requireNonNull(element, "onBuild element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ContainerConfig#onBuild() onBuild} list.
* @param elements An iterable of onBuild elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("OnBuild")
public final Builder onBuild(@Nullable Iterable elements) {
if (elements == null) {
this.onBuild = null;
return this;
}
this.onBuild = new ArrayList();
return addAllOnBuild(elements);
}
/**
* Adds elements to {@link ContainerConfig#onBuild() onBuild} list.
* @param elements An iterable of onBuild elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllOnBuild(Iterable elements) {
Objects.requireNonNull(elements, "onBuild element");
if (this.onBuild == null) {
this.onBuild = new ArrayList();
}
for (String element : elements) {
this.onBuild.add(Objects.requireNonNull(element, "onBuild element"));
}
return this;
}
/**
* Put one entry to the {@link ContainerConfig#labels() labels} map.
* @param key The key in the labels map
* @param value The associated value in the labels map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addLabel(String key, String value) {
if (this.labels == null) {
this.labels = new LinkedHashMap();
}
this.labels.put(
Objects.requireNonNull(key, "labels key"),
Objects.requireNonNull(value, value == null ? "labels value for key: " + key : null));
return this;
}
/**
* Put one entry to the {@link ContainerConfig#labels() labels} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addLabel(Map.Entry entry) {
if (this.labels == null) {
this.labels = new LinkedHashMap();
}
String k = entry.getKey();
String v = entry.getValue();
this.labels.put(
Objects.requireNonNull(k, "labels key"),
Objects.requireNonNull(v, v == null ? "labels value for key: " + k : null));
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link ContainerConfig#labels() labels} map. Nulls are not permitted as keys or values, but parameter itself can be null
* @param entries The entries that will be added to the labels map
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Labels")
public final Builder labels(@Nullable Map entries) {
if (entries == null) {
this.labels = null;
return this;
}
this.labels = new LinkedHashMap();
return putAllLabels(entries);
}
/**
* Put all mappings from the specified map as entries to {@link ContainerConfig#labels() labels} map. Nulls are not permitted
* @param entries The entries that will be added to the labels map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putAllLabels(Map entries) {
if (this.labels == null) {
this.labels = new LinkedHashMap();
}
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
String v = e.getValue();
this.labels.put(
Objects.requireNonNull(k, "labels key"),
Objects.requireNonNull(v, v == null ? "labels value for key: " + k : null));
}
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#macAddress() macAddress} attribute.
* @param macAddress The value for macAddress (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("MacAddress")
@Deprecated
public final Builder macAddress(@Nullable String macAddress) {
this.macAddress = macAddress;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#hostConfig() hostConfig} attribute.
* @param hostConfig The value for hostConfig (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("HostConfig")
public final Builder hostConfig(@Nullable HostConfig hostConfig) {
this.hostConfig = hostConfig;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#stopSignal() stopSignal} attribute.
* @param stopSignal The value for stopSignal (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("StopSignal")
public final Builder stopSignal(@Nullable String stopSignal) {
this.stopSignal = stopSignal;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#healthcheck() healthcheck} attribute.
* @param healthcheck The value for healthcheck (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Healthcheck")
public final Builder healthcheck(@Nullable Healthcheck healthcheck) {
this.healthcheck = healthcheck;
return this;
}
/**
* Initializes the value for the {@link ContainerConfig#networkingConfig() networkingConfig} attribute.
* @param networkingConfig The value for networkingConfig (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("NetworkingConfig")
public final Builder networkingConfig(@Nullable NetworkingConfig networkingConfig) {
this.networkingConfig = networkingConfig;
return this;
}
/**
* Builds a new {@link ImmutableContainerConfig ImmutableContainerConfig}.
* @return An immutable instance of ContainerConfig
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableContainerConfig build() {
return new ImmutableContainerConfig(
hostname,
domainname,
user,
attachStdin,
attachStdout,
attachStderr,
portSpecs == null ? null : createUnmodifiableList(true, portSpecs),
exposedPorts == null ? null : createUnmodifiableSet(exposedPorts),
tty,
openStdin,
stdinOnce,
env == null ? null : createUnmodifiableList(true, env),
cmd == null ? null : createUnmodifiableList(true, cmd),
image,
volumes == null ? null : createUnmodifiableSet(volumes),
workingDir,
entrypoint == null ? null : createUnmodifiableList(true, entrypoint),
networkDisabled,
onBuild == null ? null : createUnmodifiableList(true, onBuild),
labels == null ? null : createUnmodifiableMap(false, false, labels),
macAddress,
hostConfig,
stopSignal,
healthcheck,
networkingConfig);
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
/** Unmodifiable set constructed from list to avoid rehashing. */
private static Set createUnmodifiableSet(List list) {
switch(list.size()) {
case 0: return Collections.emptySet();
case 1: return Collections.singleton(list.get(0));
default:
Set set = new LinkedHashSet<>(list.size() * 4 / 3 + 1);
set.addAll(list);
return Collections.unmodifiableSet(set);
}
}
private static Map createUnmodifiableMap(boolean checkNulls, boolean skipNulls, Map extends K, ? extends V> map) {
switch (map.size()) {
case 0: return Collections.emptyMap();
case 1: {
Map.Entry extends K, ? extends V> e = map.entrySet().iterator().next();
K k = e.getKey();
V v = e.getValue();
if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, v == null ? "value for key: " + k : null);
}
if (skipNulls && (k == null || v == null)) {
return Collections.emptyMap();
}
return Collections.singletonMap(k, v);
}
default: {
Map linkedMap = new LinkedHashMap<>(map.size() * 4 / 3 + 1);
if (skipNulls || checkNulls) {
for (java.util.Map.Entry extends K, ? extends V> e : map.entrySet()) {
K k = e.getKey();
V v = e.getValue();
if (skipNulls) {
if (k == null || v == null) continue;
} else if (checkNulls) {
java.util.Objects.requireNonNull(k, "key");
java.util.Objects.requireNonNull(v, v == null ? "value for key: " + k : null);
}
linkedMap.put(k, v);
}
} else {
linkedMap.putAll(map);
}
return java.util.Collections.unmodifiableMap(linkedMap);
}
}
}
}