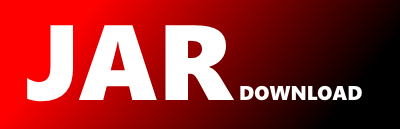
org.mandas.docker.client.messages.ImmutableContainerState Maven / Gradle / Ivy
package org.mandas.docker.client.messages;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.Date;
import java.util.List;
import java.util.Objects;
import org.mandas.docker.Nullable;
/**
* Immutable implementation of {@link ContainerState}.
*
* Use the builder to create immutable instances:
* {@code ImmutableContainerState.builder()}.
*/
@SuppressWarnings({"all"})
final class ImmutableContainerState implements ContainerState {
private final @Nullable String status;
private final boolean running;
private final boolean paused;
private final @Nullable Boolean restarting;
private final Integer pid;
private final Long exitCode;
private final Date startedAt;
private final Date finishedAt;
private final @Nullable String error;
private final @Nullable Boolean oomKilled;
private final @Nullable ContainerState.Health health;
private ImmutableContainerState(
@Nullable String status,
boolean running,
boolean paused,
@Nullable Boolean restarting,
Integer pid,
Long exitCode,
Date startedAt,
Date finishedAt,
@Nullable String error,
@Nullable Boolean oomKilled,
@Nullable ContainerState.Health health) {
this.status = status;
this.running = running;
this.paused = paused;
this.restarting = restarting;
this.pid = pid;
this.exitCode = exitCode;
this.startedAt = startedAt;
this.finishedAt = finishedAt;
this.error = error;
this.oomKilled = oomKilled;
this.health = health;
}
/**
* @return The value of the {@code status} attribute
*/
@JsonProperty("Status")
@Override
public @Nullable String status() {
return status;
}
/**
* @return The value of the {@code running} attribute
*/
@JsonProperty("Running")
@Override
public boolean running() {
return running;
}
/**
* @return The value of the {@code paused} attribute
*/
@JsonProperty("Paused")
@Override
public boolean paused() {
return paused;
}
/**
* @return The value of the {@code restarting} attribute
*/
@JsonProperty("Restarting")
@Override
public @Nullable Boolean restarting() {
return restarting;
}
/**
* @return The value of the {@code pid} attribute
*/
@JsonProperty("Pid")
@Override
public Integer pid() {
return pid;
}
/**
* @return The value of the {@code exitCode} attribute
*/
@JsonProperty("ExitCode")
@Override
public Long exitCode() {
return exitCode;
}
/**
* @return The value of the {@code startedAt} attribute
*/
@JsonProperty("StartedAt")
@Override
public Date startedAt() {
return startedAt;
}
/**
* @return The value of the {@code finishedAt} attribute
*/
@JsonProperty("FinishedAt")
@Override
public Date finishedAt() {
return finishedAt;
}
/**
* @return The value of the {@code error} attribute
*/
@JsonProperty("Error")
@Override
public @Nullable String error() {
return error;
}
/**
* @return The value of the {@code oomKilled} attribute
*/
@JsonProperty("OOMKilled")
@Override
public @Nullable Boolean oomKilled() {
return oomKilled;
}
/**
* @return The value of the {@code health} attribute
*/
@JsonProperty("Health")
@Override
public @Nullable ContainerState.Health health() {
return health;
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#status() status} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for status (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withStatus(@Nullable String value) {
if (Objects.equals(this.status, value)) return this;
return new ImmutableContainerState(
value,
this.running,
this.paused,
this.restarting,
this.pid,
this.exitCode,
this.startedAt,
this.finishedAt,
this.error,
this.oomKilled,
this.health);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#running() running} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for running
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withRunning(boolean value) {
if (this.running == value) return this;
return new ImmutableContainerState(
this.status,
value,
this.paused,
this.restarting,
this.pid,
this.exitCode,
this.startedAt,
this.finishedAt,
this.error,
this.oomKilled,
this.health);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#paused() paused} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for paused
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withPaused(boolean value) {
if (this.paused == value) return this;
return new ImmutableContainerState(
this.status,
this.running,
value,
this.restarting,
this.pid,
this.exitCode,
this.startedAt,
this.finishedAt,
this.error,
this.oomKilled,
this.health);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#restarting() restarting} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for restarting (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withRestarting(@Nullable Boolean value) {
if (Objects.equals(this.restarting, value)) return this;
return new ImmutableContainerState(
this.status,
this.running,
this.paused,
value,
this.pid,
this.exitCode,
this.startedAt,
this.finishedAt,
this.error,
this.oomKilled,
this.health);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#pid() pid} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for pid
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withPid(Integer value) {
Integer newValue = Objects.requireNonNull(value, "pid");
if (this.pid.equals(newValue)) return this;
return new ImmutableContainerState(
this.status,
this.running,
this.paused,
this.restarting,
newValue,
this.exitCode,
this.startedAt,
this.finishedAt,
this.error,
this.oomKilled,
this.health);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#exitCode() exitCode} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for exitCode
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withExitCode(Long value) {
Long newValue = Objects.requireNonNull(value, "exitCode");
if (this.exitCode.equals(newValue)) return this;
return new ImmutableContainerState(
this.status,
this.running,
this.paused,
this.restarting,
this.pid,
newValue,
this.startedAt,
this.finishedAt,
this.error,
this.oomKilled,
this.health);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#startedAt() startedAt} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for startedAt
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withStartedAt(Date value) {
if (this.startedAt == value) return this;
Date newValue = Objects.requireNonNull(value, "startedAt");
return new ImmutableContainerState(
this.status,
this.running,
this.paused,
this.restarting,
this.pid,
this.exitCode,
newValue,
this.finishedAt,
this.error,
this.oomKilled,
this.health);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#finishedAt() finishedAt} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for finishedAt
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withFinishedAt(Date value) {
if (this.finishedAt == value) return this;
Date newValue = Objects.requireNonNull(value, "finishedAt");
return new ImmutableContainerState(
this.status,
this.running,
this.paused,
this.restarting,
this.pid,
this.exitCode,
this.startedAt,
newValue,
this.error,
this.oomKilled,
this.health);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#error() error} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for error (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withError(@Nullable String value) {
if (Objects.equals(this.error, value)) return this;
return new ImmutableContainerState(
this.status,
this.running,
this.paused,
this.restarting,
this.pid,
this.exitCode,
this.startedAt,
this.finishedAt,
value,
this.oomKilled,
this.health);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#oomKilled() oomKilled} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for oomKilled (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withOomKilled(@Nullable Boolean value) {
if (Objects.equals(this.oomKilled, value)) return this;
return new ImmutableContainerState(
this.status,
this.running,
this.paused,
this.restarting,
this.pid,
this.exitCode,
this.startedAt,
this.finishedAt,
this.error,
value,
this.health);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState#health() health} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for health (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState withHealth(@Nullable ContainerState.Health value) {
if (this.health == value) return this;
return new ImmutableContainerState(
this.status,
this.running,
this.paused,
this.restarting,
this.pid,
this.exitCode,
this.startedAt,
this.finishedAt,
this.error,
this.oomKilled,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableContainerState} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableContainerState
&& equalTo(0, (ImmutableContainerState) another);
}
private boolean equalTo(int synthetic, ImmutableContainerState another) {
return Objects.equals(status, another.status)
&& running == another.running
&& paused == another.paused
&& Objects.equals(restarting, another.restarting)
&& pid.equals(another.pid)
&& exitCode.equals(another.exitCode)
&& startedAt.equals(another.startedAt)
&& finishedAt.equals(another.finishedAt)
&& Objects.equals(error, another.error)
&& Objects.equals(oomKilled, another.oomKilled)
&& Objects.equals(health, another.health);
}
/**
* Computes a hash code from attributes: {@code status}, {@code running}, {@code paused}, {@code restarting}, {@code pid}, {@code exitCode}, {@code startedAt}, {@code finishedAt}, {@code error}, {@code oomKilled}, {@code health}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(status);
h += (h << 5) + Boolean.hashCode(running);
h += (h << 5) + Boolean.hashCode(paused);
h += (h << 5) + Objects.hashCode(restarting);
h += (h << 5) + pid.hashCode();
h += (h << 5) + exitCode.hashCode();
h += (h << 5) + startedAt.hashCode();
h += (h << 5) + finishedAt.hashCode();
h += (h << 5) + Objects.hashCode(error);
h += (h << 5) + Objects.hashCode(oomKilled);
h += (h << 5) + Objects.hashCode(health);
return h;
}
/**
* Prints the immutable value {@code ContainerState} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "ContainerState{"
+ "status=" + status
+ ", running=" + running
+ ", paused=" + paused
+ ", restarting=" + restarting
+ ", pid=" + pid
+ ", exitCode=" + exitCode
+ ", startedAt=" + startedAt
+ ", finishedAt=" + finishedAt
+ ", error=" + error
+ ", oomKilled=" + oomKilled
+ ", health=" + health
+ "}";
}
/**
* Creates an immutable copy of a {@link ContainerState} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ContainerState instance
*/
public static ImmutableContainerState copyOf(ContainerState instance) {
if (instance instanceof ImmutableContainerState) {
return (ImmutableContainerState) instance;
}
return ImmutableContainerState.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableContainerState ImmutableContainerState}.
*
* ImmutableContainerState.builder()
* .status(String | null) // nullable {@link ContainerState#status() status}
* .running(boolean) // required {@link ContainerState#running() running}
* .paused(boolean) // required {@link ContainerState#paused() paused}
* .restarting(Boolean | null) // nullable {@link ContainerState#restarting() restarting}
* .pid(Integer) // required {@link ContainerState#pid() pid}
* .exitCode(Long) // required {@link ContainerState#exitCode() exitCode}
* .startedAt(Date) // required {@link ContainerState#startedAt() startedAt}
* .finishedAt(Date) // required {@link ContainerState#finishedAt() finishedAt}
* .error(String | null) // nullable {@link ContainerState#error() error}
* .oomKilled(Boolean | null) // nullable {@link ContainerState#oomKilled() oomKilled}
* .health(org.mandas.docker.client.messages.ContainerState.Health | null) // nullable {@link ContainerState#health() health}
* .build();
*
* @return A new ImmutableContainerState builder
*/
public static ImmutableContainerState.Builder builder() {
return new ImmutableContainerState.Builder();
}
/**
* Builds instances of type {@link ImmutableContainerState ImmutableContainerState}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder {
private static final long INIT_BIT_RUNNING = 0x1L;
private static final long INIT_BIT_PAUSED = 0x2L;
private static final long INIT_BIT_PID = 0x4L;
private static final long INIT_BIT_EXIT_CODE = 0x8L;
private static final long INIT_BIT_STARTED_AT = 0x10L;
private static final long INIT_BIT_FINISHED_AT = 0x20L;
private long initBits = 0x3fL;
private String status;
private boolean running;
private boolean paused;
private Boolean restarting;
private Integer pid;
private Long exitCode;
private Date startedAt;
private Date finishedAt;
private String error;
private Boolean oomKilled;
private ContainerState.Health health;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ContainerState} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ContainerState instance) {
Objects.requireNonNull(instance, "instance");
@Nullable String statusValue = instance.status();
if (statusValue != null) {
status(statusValue);
}
this.running(instance.running());
this.paused(instance.paused());
@Nullable Boolean restartingValue = instance.restarting();
if (restartingValue != null) {
restarting(restartingValue);
}
this.pid(instance.pid());
this.exitCode(instance.exitCode());
this.startedAt(instance.startedAt());
this.finishedAt(instance.finishedAt());
@Nullable String errorValue = instance.error();
if (errorValue != null) {
error(errorValue);
}
@Nullable Boolean oomKilledValue = instance.oomKilled();
if (oomKilledValue != null) {
oomKilled(oomKilledValue);
}
@Nullable ContainerState.Health healthValue = instance.health();
if (healthValue != null) {
health(healthValue);
}
return this;
}
/**
* Initializes the value for the {@link ContainerState#status() status} attribute.
* @param status The value for status (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Status")
public final Builder status(@Nullable String status) {
this.status = status;
return this;
}
/**
* Initializes the value for the {@link ContainerState#running() running} attribute.
* @param running The value for running
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Running")
public final Builder running(boolean running) {
this.running = running;
initBits &= ~INIT_BIT_RUNNING;
return this;
}
/**
* Initializes the value for the {@link ContainerState#paused() paused} attribute.
* @param paused The value for paused
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Paused")
public final Builder paused(boolean paused) {
this.paused = paused;
initBits &= ~INIT_BIT_PAUSED;
return this;
}
/**
* Initializes the value for the {@link ContainerState#restarting() restarting} attribute.
* @param restarting The value for restarting (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Restarting")
public final Builder restarting(@Nullable Boolean restarting) {
this.restarting = restarting;
return this;
}
/**
* Initializes the value for the {@link ContainerState#pid() pid} attribute.
* @param pid The value for pid
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Pid")
public final Builder pid(Integer pid) {
this.pid = Objects.requireNonNull(pid, "pid");
initBits &= ~INIT_BIT_PID;
return this;
}
/**
* Initializes the value for the {@link ContainerState#exitCode() exitCode} attribute.
* @param exitCode The value for exitCode
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("ExitCode")
public final Builder exitCode(Long exitCode) {
this.exitCode = Objects.requireNonNull(exitCode, "exitCode");
initBits &= ~INIT_BIT_EXIT_CODE;
return this;
}
/**
* Initializes the value for the {@link ContainerState#startedAt() startedAt} attribute.
* @param startedAt The value for startedAt
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("StartedAt")
public final Builder startedAt(Date startedAt) {
this.startedAt = Objects.requireNonNull(startedAt, "startedAt");
initBits &= ~INIT_BIT_STARTED_AT;
return this;
}
/**
* Initializes the value for the {@link ContainerState#finishedAt() finishedAt} attribute.
* @param finishedAt The value for finishedAt
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("FinishedAt")
public final Builder finishedAt(Date finishedAt) {
this.finishedAt = Objects.requireNonNull(finishedAt, "finishedAt");
initBits &= ~INIT_BIT_FINISHED_AT;
return this;
}
/**
* Initializes the value for the {@link ContainerState#error() error} attribute.
* @param error The value for error (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Error")
public final Builder error(@Nullable String error) {
this.error = error;
return this;
}
/**
* Initializes the value for the {@link ContainerState#oomKilled() oomKilled} attribute.
* @param oomKilled The value for oomKilled (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("OOMKilled")
public final Builder oomKilled(@Nullable Boolean oomKilled) {
this.oomKilled = oomKilled;
return this;
}
/**
* Initializes the value for the {@link ContainerState#health() health} attribute.
* @param health The value for health (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Health")
public final Builder health(@Nullable ContainerState.Health health) {
this.health = health;
return this;
}
/**
* Builds a new {@link ImmutableContainerState ImmutableContainerState}.
* @return An immutable instance of ContainerState
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableContainerState build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableContainerState(
status,
running,
paused,
restarting,
pid,
exitCode,
startedAt,
finishedAt,
error,
oomKilled,
health);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_RUNNING) != 0) attributes.add("running");
if ((initBits & INIT_BIT_PAUSED) != 0) attributes.add("paused");
if ((initBits & INIT_BIT_PID) != 0) attributes.add("pid");
if ((initBits & INIT_BIT_EXIT_CODE) != 0) attributes.add("exitCode");
if ((initBits & INIT_BIT_STARTED_AT) != 0) attributes.add("startedAt");
if ((initBits & INIT_BIT_FINISHED_AT) != 0) attributes.add("finishedAt");
return "Cannot build ContainerState, some of required attributes are not set " + attributes;
}
}
/**
* Immutable implementation of {@link ContainerState.HealthLog}.
*
* Use the builder to create immutable instances:
* {@code ImmutableContainerState.HealthLog.builder()}.
*/
static final class HealthLog implements ContainerState.HealthLog {
private final Date start;
private final Date end;
private final Long exitCode;
private final String output;
private HealthLog(Date start, Date end, Long exitCode, String output) {
this.start = start;
this.end = end;
this.exitCode = exitCode;
this.output = output;
}
/**
* @return The value of the {@code start} attribute
*/
@JsonProperty("Start")
@Override
public Date start() {
return start;
}
/**
* @return The value of the {@code end} attribute
*/
@JsonProperty("End")
@Override
public Date end() {
return end;
}
/**
* @return The value of the {@code exitCode} attribute
*/
@JsonProperty("ExitCode")
@Override
public Long exitCode() {
return exitCode;
}
/**
* @return The value of the {@code output} attribute
*/
@JsonProperty("Output")
@Override
public String output() {
return output;
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState.HealthLog#start() start} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for start
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState.HealthLog withStart(Date value) {
if (this.start == value) return this;
Date newValue = Objects.requireNonNull(value, "start");
return new ImmutableContainerState.HealthLog(newValue, this.end, this.exitCode, this.output);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState.HealthLog#end() end} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for end
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState.HealthLog withEnd(Date value) {
if (this.end == value) return this;
Date newValue = Objects.requireNonNull(value, "end");
return new ImmutableContainerState.HealthLog(this.start, newValue, this.exitCode, this.output);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState.HealthLog#exitCode() exitCode} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for exitCode
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState.HealthLog withExitCode(Long value) {
Long newValue = Objects.requireNonNull(value, "exitCode");
if (this.exitCode.equals(newValue)) return this;
return new ImmutableContainerState.HealthLog(this.start, this.end, newValue, this.output);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState.HealthLog#output() output} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for output
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState.HealthLog withOutput(String value) {
String newValue = Objects.requireNonNull(value, "output");
if (this.output.equals(newValue)) return this;
return new ImmutableContainerState.HealthLog(this.start, this.end, this.exitCode, newValue);
}
/**
* This instance is equal to all instances of {@code HealthLog} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableContainerState.HealthLog
&& equalTo(0, (ImmutableContainerState.HealthLog) another);
}
private boolean equalTo(int synthetic, ImmutableContainerState.HealthLog another) {
return start.equals(another.start)
&& end.equals(another.end)
&& exitCode.equals(another.exitCode)
&& output.equals(another.output);
}
/**
* Computes a hash code from attributes: {@code start}, {@code end}, {@code exitCode}, {@code output}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + start.hashCode();
h += (h << 5) + end.hashCode();
h += (h << 5) + exitCode.hashCode();
h += (h << 5) + output.hashCode();
return h;
}
/**
* Prints the immutable value {@code HealthLog} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "HealthLog{"
+ "start=" + start
+ ", end=" + end
+ ", exitCode=" + exitCode
+ ", output=" + output
+ "}";
}
/**
* Creates an immutable copy of a {@link ContainerState.HealthLog} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable HealthLog instance
*/
public static ImmutableContainerState.HealthLog copyOf(ContainerState.HealthLog instance) {
if (instance instanceof ImmutableContainerState.HealthLog) {
return (ImmutableContainerState.HealthLog) instance;
}
return ImmutableContainerState.HealthLog.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableContainerState.HealthLog HealthLog}.
*
* ImmutableContainerState.HealthLog.builder()
* .start(Date) // required {@link ContainerState.HealthLog#start() start}
* .end(Date) // required {@link ContainerState.HealthLog#end() end}
* .exitCode(Long) // required {@link ContainerState.HealthLog#exitCode() exitCode}
* .output(String) // required {@link ContainerState.HealthLog#output() output}
* .build();
*
* @return A new HealthLog builder
*/
public static ImmutableContainerState.HealthLog.Builder builder() {
return new ImmutableContainerState.HealthLog.Builder();
}
/**
* Builds instances of type {@link ImmutableContainerState.HealthLog HealthLog}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder {
private static final long INIT_BIT_START = 0x1L;
private static final long INIT_BIT_END = 0x2L;
private static final long INIT_BIT_EXIT_CODE = 0x4L;
private static final long INIT_BIT_OUTPUT = 0x8L;
private long initBits = 0xfL;
private Date start;
private Date end;
private Long exitCode;
private String output;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code HealthLog} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ContainerState.HealthLog instance) {
Objects.requireNonNull(instance, "instance");
this.start(instance.start());
this.end(instance.end());
this.exitCode(instance.exitCode());
this.output(instance.output());
return this;
}
/**
* Initializes the value for the {@link ContainerState.HealthLog#start() start} attribute.
* @param start The value for start
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Start")
public final Builder start(Date start) {
this.start = Objects.requireNonNull(start, "start");
initBits &= ~INIT_BIT_START;
return this;
}
/**
* Initializes the value for the {@link ContainerState.HealthLog#end() end} attribute.
* @param end The value for end
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("End")
public final Builder end(Date end) {
this.end = Objects.requireNonNull(end, "end");
initBits &= ~INIT_BIT_END;
return this;
}
/**
* Initializes the value for the {@link ContainerState.HealthLog#exitCode() exitCode} attribute.
* @param exitCode The value for exitCode
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("ExitCode")
public final Builder exitCode(Long exitCode) {
this.exitCode = Objects.requireNonNull(exitCode, "exitCode");
initBits &= ~INIT_BIT_EXIT_CODE;
return this;
}
/**
* Initializes the value for the {@link ContainerState.HealthLog#output() output} attribute.
* @param output The value for output
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Output")
public final Builder output(String output) {
this.output = Objects.requireNonNull(output, "output");
initBits &= ~INIT_BIT_OUTPUT;
return this;
}
/**
* Builds a new {@link ImmutableContainerState.HealthLog HealthLog}.
* @return An immutable instance of HealthLog
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableContainerState.HealthLog build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableContainerState.HealthLog(start, end, exitCode, output);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_START) != 0) attributes.add("start");
if ((initBits & INIT_BIT_END) != 0) attributes.add("end");
if ((initBits & INIT_BIT_EXIT_CODE) != 0) attributes.add("exitCode");
if ((initBits & INIT_BIT_OUTPUT) != 0) attributes.add("output");
return "Cannot build HealthLog, some of required attributes are not set " + attributes;
}
}
}
/**
* Immutable implementation of {@link ContainerState.Health}.
*
* Use the builder to create immutable instances:
* {@code ImmutableContainerState.Health.builder()}.
*/
static final class Health implements ContainerState.Health {
private final String status;
private final Integer failingStreak;
private final List log;
private Health(
String status,
Integer failingStreak,
List log) {
this.status = status;
this.failingStreak = failingStreak;
this.log = log;
}
/**
* @return The value of the {@code status} attribute
*/
@JsonProperty("Status")
@Override
public String status() {
return status;
}
/**
* @return The value of the {@code failingStreak} attribute
*/
@JsonProperty("FailingStreak")
@Override
public Integer failingStreak() {
return failingStreak;
}
/**
* @return The value of the {@code log} attribute
*/
@JsonProperty("Log")
@Override
public List log() {
return log;
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState.Health#status() status} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for status
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState.Health withStatus(String value) {
String newValue = Objects.requireNonNull(value, "status");
if (this.status.equals(newValue)) return this;
return new ImmutableContainerState.Health(newValue, this.failingStreak, this.log);
}
/**
* Copy the current immutable object by setting a value for the {@link ContainerState.Health#failingStreak() failingStreak} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for failingStreak
* @return A modified copy of the {@code this} object
*/
public final ImmutableContainerState.Health withFailingStreak(Integer value) {
Integer newValue = Objects.requireNonNull(value, "failingStreak");
if (this.failingStreak.equals(newValue)) return this;
return new ImmutableContainerState.Health(this.status, newValue, this.log);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerState.Health#log() log}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerState.Health withLog(ContainerState.HealthLog... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableContainerState.Health(this.status, this.failingStreak, newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ContainerState.Health#log() log}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of log elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableContainerState.Health withLog(Iterable extends ContainerState.HealthLog> elements) {
if (this.log == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableContainerState.Health(this.status, this.failingStreak, newValue);
}
/**
* This instance is equal to all instances of {@code Health} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableContainerState.Health
&& equalTo(0, (ImmutableContainerState.Health) another);
}
private boolean equalTo(int synthetic, ImmutableContainerState.Health another) {
return status.equals(another.status)
&& failingStreak.equals(another.failingStreak)
&& log.equals(another.log);
}
/**
* Computes a hash code from attributes: {@code status}, {@code failingStreak}, {@code log}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + status.hashCode();
h += (h << 5) + failingStreak.hashCode();
h += (h << 5) + log.hashCode();
return h;
}
/**
* Prints the immutable value {@code Health} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "Health{"
+ "status=" + status
+ ", failingStreak=" + failingStreak
+ ", log=" + log
+ "}";
}
/**
* Creates an immutable copy of a {@link ContainerState.Health} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Health instance
*/
public static ImmutableContainerState.Health copyOf(ContainerState.Health instance) {
if (instance instanceof ImmutableContainerState.Health) {
return (ImmutableContainerState.Health) instance;
}
return ImmutableContainerState.Health.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableContainerState.Health Health}.
*
* ImmutableContainerState.Health.builder()
* .status(String) // required {@link ContainerState.Health#status() status}
* .failingStreak(Integer) // required {@link ContainerState.Health#failingStreak() failingStreak}
* .log|addAllLog(org.mandas.docker.client.messages.ContainerState.HealthLog) // {@link ContainerState.Health#log() log} elements
* .build();
*
* @return A new Health builder
*/
public static ImmutableContainerState.Health.Builder builder() {
return new ImmutableContainerState.Health.Builder();
}
/**
* Builds instances of type {@link ImmutableContainerState.Health Health}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder {
private static final long INIT_BIT_STATUS = 0x1L;
private static final long INIT_BIT_FAILING_STREAK = 0x2L;
private long initBits = 0x3L;
private String status;
private Integer failingStreak;
private List log = new ArrayList();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Health} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ContainerState.Health instance) {
Objects.requireNonNull(instance, "instance");
this.status(instance.status());
this.failingStreak(instance.failingStreak());
addAllLog(instance.log());
return this;
}
/**
* Initializes the value for the {@link ContainerState.Health#status() status} attribute.
* @param status The value for status
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Status")
public final Builder status(String status) {
this.status = Objects.requireNonNull(status, "status");
initBits &= ~INIT_BIT_STATUS;
return this;
}
/**
* Initializes the value for the {@link ContainerState.Health#failingStreak() failingStreak} attribute.
* @param failingStreak The value for failingStreak
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("FailingStreak")
public final Builder failingStreak(Integer failingStreak) {
this.failingStreak = Objects.requireNonNull(failingStreak, "failingStreak");
initBits &= ~INIT_BIT_FAILING_STREAK;
return this;
}
/**
* Adds one element to {@link ContainerState.Health#log() log} list.
* @param element A log element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder log(ContainerState.HealthLog element) {
this.log.add(Objects.requireNonNull(element, "log element"));
return this;
}
/**
* Adds elements to {@link ContainerState.Health#log() log} list.
* @param elements An array of log elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder log(ContainerState.HealthLog... elements) {
for (ContainerState.HealthLog element : elements) {
this.log.add(Objects.requireNonNull(element, "log element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link ContainerState.Health#log() log} list.
* @param elements An iterable of log elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Log")
public final Builder log(Iterable extends ContainerState.HealthLog> elements) {
this.log.clear();
return addAllLog(elements);
}
/**
* Adds elements to {@link ContainerState.Health#log() log} list.
* @param elements An iterable of log elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllLog(Iterable extends ContainerState.HealthLog> elements) {
for (ContainerState.HealthLog element : elements) {
this.log.add(Objects.requireNonNull(element, "log element"));
}
return this;
}
/**
* Builds a new {@link ImmutableContainerState.Health Health}.
* @return An immutable instance of Health
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableContainerState.Health build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableContainerState.Health(status, failingStreak, createUnmodifiableList(true, log));
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_STATUS) != 0) attributes.add("status");
if ((initBits & INIT_BIT_FAILING_STREAK) != 0) attributes.add("failingStreak");
return "Cannot build Health, some of required attributes are not set " + attributes;
}
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}