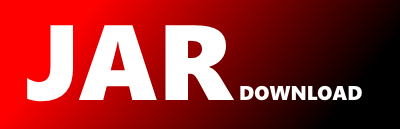
org.mandas.docker.client.messages.ImmutableCpuStats Maven / Gradle / Ivy
package org.mandas.docker.client.messages;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import org.mandas.docker.Nullable;
/**
* Immutable implementation of {@link CpuStats}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCpuStats.builder()}.
*/
@SuppressWarnings({"all"})
final class ImmutableCpuStats implements CpuStats {
private final CpuStats.CpuUsage cpuUsage;
private final @Nullable Long systemCpuUsage;
private final CpuStats.ThrottlingData throttlingData;
private ImmutableCpuStats(
CpuStats.CpuUsage cpuUsage,
@Nullable Long systemCpuUsage,
CpuStats.ThrottlingData throttlingData) {
this.cpuUsage = cpuUsage;
this.systemCpuUsage = systemCpuUsage;
this.throttlingData = throttlingData;
}
/**
* @return The value of the {@code cpuUsage} attribute
*/
@JsonProperty("cpu_usage")
@Override
public CpuStats.CpuUsage cpuUsage() {
return cpuUsage;
}
/**
* @return The value of the {@code systemCpuUsage} attribute
*/
@JsonProperty("system_cpu_usage")
@Override
public @Nullable Long systemCpuUsage() {
return systemCpuUsage;
}
/**
* @return The value of the {@code throttlingData} attribute
*/
@JsonProperty("throttling_data")
@Override
public CpuStats.ThrottlingData throttlingData() {
return throttlingData;
}
/**
* Copy the current immutable object by setting a value for the {@link CpuStats#cpuUsage() cpuUsage} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for cpuUsage
* @return A modified copy of the {@code this} object
*/
public final ImmutableCpuStats withCpuUsage(CpuStats.CpuUsage value) {
if (this.cpuUsage == value) return this;
CpuStats.CpuUsage newValue = Objects.requireNonNull(value, "cpuUsage");
return new ImmutableCpuStats(newValue, this.systemCpuUsage, this.throttlingData);
}
/**
* Copy the current immutable object by setting a value for the {@link CpuStats#systemCpuUsage() systemCpuUsage} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for systemCpuUsage (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCpuStats withSystemCpuUsage(@Nullable Long value) {
if (Objects.equals(this.systemCpuUsage, value)) return this;
return new ImmutableCpuStats(this.cpuUsage, value, this.throttlingData);
}
/**
* Copy the current immutable object by setting a value for the {@link CpuStats#throttlingData() throttlingData} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for throttlingData
* @return A modified copy of the {@code this} object
*/
public final ImmutableCpuStats withThrottlingData(CpuStats.ThrottlingData value) {
if (this.throttlingData == value) return this;
CpuStats.ThrottlingData newValue = Objects.requireNonNull(value, "throttlingData");
return new ImmutableCpuStats(this.cpuUsage, this.systemCpuUsage, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableCpuStats} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCpuStats
&& equalTo(0, (ImmutableCpuStats) another);
}
private boolean equalTo(int synthetic, ImmutableCpuStats another) {
return cpuUsage.equals(another.cpuUsage)
&& Objects.equals(systemCpuUsage, another.systemCpuUsage)
&& throttlingData.equals(another.throttlingData);
}
/**
* Computes a hash code from attributes: {@code cpuUsage}, {@code systemCpuUsage}, {@code throttlingData}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + cpuUsage.hashCode();
h += (h << 5) + Objects.hashCode(systemCpuUsage);
h += (h << 5) + throttlingData.hashCode();
return h;
}
/**
* Prints the immutable value {@code CpuStats} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "CpuStats{"
+ "cpuUsage=" + cpuUsage
+ ", systemCpuUsage=" + systemCpuUsage
+ ", throttlingData=" + throttlingData
+ "}";
}
/**
* Creates an immutable copy of a {@link CpuStats} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CpuStats instance
*/
public static ImmutableCpuStats copyOf(CpuStats instance) {
if (instance instanceof ImmutableCpuStats) {
return (ImmutableCpuStats) instance;
}
return ImmutableCpuStats.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCpuStats ImmutableCpuStats}.
*
* ImmutableCpuStats.builder()
* .cpuUsage(org.mandas.docker.client.messages.CpuStats.CpuUsage) // required {@link CpuStats#cpuUsage() cpuUsage}
* .systemCpuUsage(Long | null) // nullable {@link CpuStats#systemCpuUsage() systemCpuUsage}
* .throttlingData(org.mandas.docker.client.messages.CpuStats.ThrottlingData) // required {@link CpuStats#throttlingData() throttlingData}
* .build();
*
* @return A new ImmutableCpuStats builder
*/
public static ImmutableCpuStats.Builder builder() {
return new ImmutableCpuStats.Builder();
}
/**
* Builds instances of type {@link ImmutableCpuStats ImmutableCpuStats}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder {
private static final long INIT_BIT_CPU_USAGE = 0x1L;
private static final long INIT_BIT_THROTTLING_DATA = 0x2L;
private long initBits = 0x3L;
private CpuStats.CpuUsage cpuUsage;
private Long systemCpuUsage;
private CpuStats.ThrottlingData throttlingData;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code CpuStats} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CpuStats instance) {
Objects.requireNonNull(instance, "instance");
this.cpuUsage(instance.cpuUsage());
@Nullable Long systemCpuUsageValue = instance.systemCpuUsage();
if (systemCpuUsageValue != null) {
systemCpuUsage(systemCpuUsageValue);
}
this.throttlingData(instance.throttlingData());
return this;
}
/**
* Initializes the value for the {@link CpuStats#cpuUsage() cpuUsage} attribute.
* @param cpuUsage The value for cpuUsage
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("cpu_usage")
public final Builder cpuUsage(CpuStats.CpuUsage cpuUsage) {
this.cpuUsage = Objects.requireNonNull(cpuUsage, "cpuUsage");
initBits &= ~INIT_BIT_CPU_USAGE;
return this;
}
/**
* Initializes the value for the {@link CpuStats#systemCpuUsage() systemCpuUsage} attribute.
* @param systemCpuUsage The value for systemCpuUsage (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("system_cpu_usage")
public final Builder systemCpuUsage(@Nullable Long systemCpuUsage) {
this.systemCpuUsage = systemCpuUsage;
return this;
}
/**
* Initializes the value for the {@link CpuStats#throttlingData() throttlingData} attribute.
* @param throttlingData The value for throttlingData
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("throttling_data")
public final Builder throttlingData(CpuStats.ThrottlingData throttlingData) {
this.throttlingData = Objects.requireNonNull(throttlingData, "throttlingData");
initBits &= ~INIT_BIT_THROTTLING_DATA;
return this;
}
/**
* Builds a new {@link ImmutableCpuStats ImmutableCpuStats}.
* @return An immutable instance of CpuStats
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCpuStats build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableCpuStats(cpuUsage, systemCpuUsage, throttlingData);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_CPU_USAGE) != 0) attributes.add("cpuUsage");
if ((initBits & INIT_BIT_THROTTLING_DATA) != 0) attributes.add("throttlingData");
return "Cannot build CpuStats, some of required attributes are not set " + attributes;
}
}
/**
* Immutable implementation of {@link CpuStats.CpuUsage}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCpuStats.CpuUsage.builder()}.
*/
static final class CpuUsage implements CpuStats.CpuUsage {
private final Long totalUsage;
private final @Nullable List percpuUsage;
private final Long usageInKernelmode;
private final Long usageInUsermode;
private CpuUsage(
Long totalUsage,
@Nullable List percpuUsage,
Long usageInKernelmode,
Long usageInUsermode) {
this.totalUsage = totalUsage;
this.percpuUsage = percpuUsage;
this.usageInKernelmode = usageInKernelmode;
this.usageInUsermode = usageInUsermode;
}
/**
* @return The value of the {@code totalUsage} attribute
*/
@JsonProperty("total_usage")
@Override
public Long totalUsage() {
return totalUsage;
}
/**
* @return The value of the {@code percpuUsage} attribute
*/
@JsonProperty("percpu_usage")
@Override
public @Nullable List percpuUsage() {
return percpuUsage;
}
/**
* @return The value of the {@code usageInKernelmode} attribute
*/
@JsonProperty("usage_in_kernelmode")
@Override
public Long usageInKernelmode() {
return usageInKernelmode;
}
/**
* @return The value of the {@code usageInUsermode} attribute
*/
@JsonProperty("usage_in_usermode")
@Override
public Long usageInUsermode() {
return usageInUsermode;
}
/**
* Copy the current immutable object by setting a value for the {@link CpuStats.CpuUsage#totalUsage() totalUsage} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalUsage
* @return A modified copy of the {@code this} object
*/
public final ImmutableCpuStats.CpuUsage withTotalUsage(Long value) {
Long newValue = Objects.requireNonNull(value, "totalUsage");
if (this.totalUsage.equals(newValue)) return this;
return new ImmutableCpuStats.CpuUsage(newValue, this.percpuUsage, this.usageInKernelmode, this.usageInUsermode);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CpuStats.CpuUsage#percpuUsage() percpuUsage}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCpuStats.CpuUsage withPercpuUsage(@Nullable long... elements) {
if (elements == null) {
return new ImmutableCpuStats.CpuUsage(this.totalUsage, null, this.usageInKernelmode, this.usageInUsermode);
}
ArrayList wrappedList = new ArrayList<>(elements.length);
for (long element : elements) {
wrappedList.add(element);
}
List newValue = createUnmodifiableList(false, wrappedList);
return new ImmutableCpuStats.CpuUsage(this.totalUsage, newValue, this.usageInKernelmode, this.usageInUsermode);
}
/**
* Copy the current immutable object with elements that replace the content of {@link CpuStats.CpuUsage#percpuUsage() percpuUsage}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of percpuUsage elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableCpuStats.CpuUsage withPercpuUsage(@Nullable Iterable elements) {
if (this.percpuUsage == elements) return this;
@Nullable List newValue = elements == null ? null : createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableCpuStats.CpuUsage(this.totalUsage, newValue, this.usageInKernelmode, this.usageInUsermode);
}
/**
* Copy the current immutable object by setting a value for the {@link CpuStats.CpuUsage#usageInKernelmode() usageInKernelmode} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for usageInKernelmode
* @return A modified copy of the {@code this} object
*/
public final ImmutableCpuStats.CpuUsage withUsageInKernelmode(Long value) {
Long newValue = Objects.requireNonNull(value, "usageInKernelmode");
if (this.usageInKernelmode.equals(newValue)) return this;
return new ImmutableCpuStats.CpuUsage(this.totalUsage, this.percpuUsage, newValue, this.usageInUsermode);
}
/**
* Copy the current immutable object by setting a value for the {@link CpuStats.CpuUsage#usageInUsermode() usageInUsermode} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for usageInUsermode
* @return A modified copy of the {@code this} object
*/
public final ImmutableCpuStats.CpuUsage withUsageInUsermode(Long value) {
Long newValue = Objects.requireNonNull(value, "usageInUsermode");
if (this.usageInUsermode.equals(newValue)) return this;
return new ImmutableCpuStats.CpuUsage(this.totalUsage, this.percpuUsage, this.usageInKernelmode, newValue);
}
/**
* This instance is equal to all instances of {@code CpuUsage} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCpuStats.CpuUsage
&& equalTo(0, (ImmutableCpuStats.CpuUsage) another);
}
private boolean equalTo(int synthetic, ImmutableCpuStats.CpuUsage another) {
return totalUsage.equals(another.totalUsage)
&& Objects.equals(percpuUsage, another.percpuUsage)
&& usageInKernelmode.equals(another.usageInKernelmode)
&& usageInUsermode.equals(another.usageInUsermode);
}
/**
* Computes a hash code from attributes: {@code totalUsage}, {@code percpuUsage}, {@code usageInKernelmode}, {@code usageInUsermode}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + totalUsage.hashCode();
h += (h << 5) + Objects.hashCode(percpuUsage);
h += (h << 5) + usageInKernelmode.hashCode();
h += (h << 5) + usageInUsermode.hashCode();
return h;
}
/**
* Prints the immutable value {@code CpuUsage} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "CpuUsage{"
+ "totalUsage=" + totalUsage
+ ", percpuUsage=" + percpuUsage
+ ", usageInKernelmode=" + usageInKernelmode
+ ", usageInUsermode=" + usageInUsermode
+ "}";
}
/**
* Creates an immutable copy of a {@link CpuStats.CpuUsage} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CpuUsage instance
*/
public static ImmutableCpuStats.CpuUsage copyOf(CpuStats.CpuUsage instance) {
if (instance instanceof ImmutableCpuStats.CpuUsage) {
return (ImmutableCpuStats.CpuUsage) instance;
}
return ImmutableCpuStats.CpuUsage.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCpuStats.CpuUsage CpuUsage}.
*
* ImmutableCpuStats.CpuUsage.builder()
* .totalUsage(Long) // required {@link CpuStats.CpuUsage#totalUsage() totalUsage}
* .percpuUsage(List<Long> | null) // nullable {@link CpuStats.CpuUsage#percpuUsage() percpuUsage}
* .usageInKernelmode(Long) // required {@link CpuStats.CpuUsage#usageInKernelmode() usageInKernelmode}
* .usageInUsermode(Long) // required {@link CpuStats.CpuUsage#usageInUsermode() usageInUsermode}
* .build();
*
* @return A new CpuUsage builder
*/
public static ImmutableCpuStats.CpuUsage.Builder builder() {
return new ImmutableCpuStats.CpuUsage.Builder();
}
/**
* Builds instances of type {@link ImmutableCpuStats.CpuUsage CpuUsage}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder {
private static final long INIT_BIT_TOTAL_USAGE = 0x1L;
private static final long INIT_BIT_USAGE_IN_KERNELMODE = 0x2L;
private static final long INIT_BIT_USAGE_IN_USERMODE = 0x4L;
private long initBits = 0x7L;
private Long totalUsage;
private List percpuUsage = null;
private Long usageInKernelmode;
private Long usageInUsermode;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code CpuUsage} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CpuStats.CpuUsage instance) {
Objects.requireNonNull(instance, "instance");
this.totalUsage(instance.totalUsage());
@Nullable List percpuUsageValue = instance.percpuUsage();
if (percpuUsageValue != null) {
addAllPercpuUsage(percpuUsageValue);
}
this.usageInKernelmode(instance.usageInKernelmode());
this.usageInUsermode(instance.usageInUsermode());
return this;
}
/**
* Initializes the value for the {@link CpuStats.CpuUsage#totalUsage() totalUsage} attribute.
* @param totalUsage The value for totalUsage
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_usage")
public final Builder totalUsage(Long totalUsage) {
this.totalUsage = Objects.requireNonNull(totalUsage, "totalUsage");
initBits &= ~INIT_BIT_TOTAL_USAGE;
return this;
}
/**
* Adds one element to {@link CpuStats.CpuUsage#percpuUsage() percpuUsage} list.
* @param element A percpuUsage element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder percpuUsage(long element) {
if (this.percpuUsage == null) {
this.percpuUsage = new ArrayList();
}
this.percpuUsage.add(element);
return this;
}
/**
* Adds elements to {@link CpuStats.CpuUsage#percpuUsage() percpuUsage} list.
* @param elements An array of percpuUsage elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder percpuUsage(long... elements) {
if (this.percpuUsage == null) {
this.percpuUsage = new ArrayList();
}
for (long element : elements) {
this.percpuUsage.add(element);
}
return this;
}
/**
* Sets or replaces all elements for {@link CpuStats.CpuUsage#percpuUsage() percpuUsage} list.
* @param elements An iterable of percpuUsage elements
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("percpu_usage")
public final Builder percpuUsage(@Nullable Iterable elements) {
if (elements == null) {
this.percpuUsage = null;
return this;
}
this.percpuUsage = new ArrayList();
return addAllPercpuUsage(elements);
}
/**
* Adds elements to {@link CpuStats.CpuUsage#percpuUsage() percpuUsage} list.
* @param elements An iterable of percpuUsage elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllPercpuUsage(Iterable elements) {
Objects.requireNonNull(elements, "percpuUsage element");
if (this.percpuUsage == null) {
this.percpuUsage = new ArrayList();
}
for (Long element : elements) {
this.percpuUsage.add(Objects.requireNonNull(element, "percpuUsage element"));
}
return this;
}
/**
* Initializes the value for the {@link CpuStats.CpuUsage#usageInKernelmode() usageInKernelmode} attribute.
* @param usageInKernelmode The value for usageInKernelmode
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("usage_in_kernelmode")
public final Builder usageInKernelmode(Long usageInKernelmode) {
this.usageInKernelmode = Objects.requireNonNull(usageInKernelmode, "usageInKernelmode");
initBits &= ~INIT_BIT_USAGE_IN_KERNELMODE;
return this;
}
/**
* Initializes the value for the {@link CpuStats.CpuUsage#usageInUsermode() usageInUsermode} attribute.
* @param usageInUsermode The value for usageInUsermode
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("usage_in_usermode")
public final Builder usageInUsermode(Long usageInUsermode) {
this.usageInUsermode = Objects.requireNonNull(usageInUsermode, "usageInUsermode");
initBits &= ~INIT_BIT_USAGE_IN_USERMODE;
return this;
}
/**
* Builds a new {@link ImmutableCpuStats.CpuUsage CpuUsage}.
* @return An immutable instance of CpuUsage
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCpuStats.CpuUsage build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableCpuStats.CpuUsage(
totalUsage,
percpuUsage == null ? null : createUnmodifiableList(true, percpuUsage),
usageInKernelmode,
usageInUsermode);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_TOTAL_USAGE) != 0) attributes.add("totalUsage");
if ((initBits & INIT_BIT_USAGE_IN_KERNELMODE) != 0) attributes.add("usageInKernelmode");
if ((initBits & INIT_BIT_USAGE_IN_USERMODE) != 0) attributes.add("usageInUsermode");
return "Cannot build CpuUsage, some of required attributes are not set " + attributes;
}
}
}
/**
* Immutable implementation of {@link CpuStats.ThrottlingData}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCpuStats.ThrottlingData.builder()}.
*/
static final class ThrottlingData implements CpuStats.ThrottlingData {
private final Long periods;
private final Long throttledPeriods;
private final Long throttledTime;
private ThrottlingData(Long periods, Long throttledPeriods, Long throttledTime) {
this.periods = periods;
this.throttledPeriods = throttledPeriods;
this.throttledTime = throttledTime;
}
/**
* @return The value of the {@code periods} attribute
*/
@JsonProperty("periods")
@Override
public Long periods() {
return periods;
}
/**
* @return The value of the {@code throttledPeriods} attribute
*/
@JsonProperty("throttled_periods")
@Override
public Long throttledPeriods() {
return throttledPeriods;
}
/**
* @return The value of the {@code throttledTime} attribute
*/
@JsonProperty("throttled_time")
@Override
public Long throttledTime() {
return throttledTime;
}
/**
* Copy the current immutable object by setting a value for the {@link CpuStats.ThrottlingData#periods() periods} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for periods
* @return A modified copy of the {@code this} object
*/
public final ImmutableCpuStats.ThrottlingData withPeriods(Long value) {
Long newValue = Objects.requireNonNull(value, "periods");
if (this.periods.equals(newValue)) return this;
return new ImmutableCpuStats.ThrottlingData(newValue, this.throttledPeriods, this.throttledTime);
}
/**
* Copy the current immutable object by setting a value for the {@link CpuStats.ThrottlingData#throttledPeriods() throttledPeriods} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for throttledPeriods
* @return A modified copy of the {@code this} object
*/
public final ImmutableCpuStats.ThrottlingData withThrottledPeriods(Long value) {
Long newValue = Objects.requireNonNull(value, "throttledPeriods");
if (this.throttledPeriods.equals(newValue)) return this;
return new ImmutableCpuStats.ThrottlingData(this.periods, newValue, this.throttledTime);
}
/**
* Copy the current immutable object by setting a value for the {@link CpuStats.ThrottlingData#throttledTime() throttledTime} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for throttledTime
* @return A modified copy of the {@code this} object
*/
public final ImmutableCpuStats.ThrottlingData withThrottledTime(Long value) {
Long newValue = Objects.requireNonNull(value, "throttledTime");
if (this.throttledTime.equals(newValue)) return this;
return new ImmutableCpuStats.ThrottlingData(this.periods, this.throttledPeriods, newValue);
}
/**
* This instance is equal to all instances of {@code ThrottlingData} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableCpuStats.ThrottlingData
&& equalTo(0, (ImmutableCpuStats.ThrottlingData) another);
}
private boolean equalTo(int synthetic, ImmutableCpuStats.ThrottlingData another) {
return periods.equals(another.periods)
&& throttledPeriods.equals(another.throttledPeriods)
&& throttledTime.equals(another.throttledTime);
}
/**
* Computes a hash code from attributes: {@code periods}, {@code throttledPeriods}, {@code throttledTime}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + periods.hashCode();
h += (h << 5) + throttledPeriods.hashCode();
h += (h << 5) + throttledTime.hashCode();
return h;
}
/**
* Prints the immutable value {@code ThrottlingData} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "ThrottlingData{"
+ "periods=" + periods
+ ", throttledPeriods=" + throttledPeriods
+ ", throttledTime=" + throttledTime
+ "}";
}
/**
* Creates an immutable copy of a {@link CpuStats.ThrottlingData} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ThrottlingData instance
*/
public static ImmutableCpuStats.ThrottlingData copyOf(CpuStats.ThrottlingData instance) {
if (instance instanceof ImmutableCpuStats.ThrottlingData) {
return (ImmutableCpuStats.ThrottlingData) instance;
}
return ImmutableCpuStats.ThrottlingData.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCpuStats.ThrottlingData ThrottlingData}.
*
* ImmutableCpuStats.ThrottlingData.builder()
* .periods(Long) // required {@link CpuStats.ThrottlingData#periods() periods}
* .throttledPeriods(Long) // required {@link CpuStats.ThrottlingData#throttledPeriods() throttledPeriods}
* .throttledTime(Long) // required {@link CpuStats.ThrottlingData#throttledTime() throttledTime}
* .build();
*
* @return A new ThrottlingData builder
*/
public static ImmutableCpuStats.ThrottlingData.Builder builder() {
return new ImmutableCpuStats.ThrottlingData.Builder();
}
/**
* Builds instances of type {@link ImmutableCpuStats.ThrottlingData ThrottlingData}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder {
private static final long INIT_BIT_PERIODS = 0x1L;
private static final long INIT_BIT_THROTTLED_PERIODS = 0x2L;
private static final long INIT_BIT_THROTTLED_TIME = 0x4L;
private long initBits = 0x7L;
private Long periods;
private Long throttledPeriods;
private Long throttledTime;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ThrottlingData} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(CpuStats.ThrottlingData instance) {
Objects.requireNonNull(instance, "instance");
this.periods(instance.periods());
this.throttledPeriods(instance.throttledPeriods());
this.throttledTime(instance.throttledTime());
return this;
}
/**
* Initializes the value for the {@link CpuStats.ThrottlingData#periods() periods} attribute.
* @param periods The value for periods
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("periods")
public final Builder periods(Long periods) {
this.periods = Objects.requireNonNull(periods, "periods");
initBits &= ~INIT_BIT_PERIODS;
return this;
}
/**
* Initializes the value for the {@link CpuStats.ThrottlingData#throttledPeriods() throttledPeriods} attribute.
* @param throttledPeriods The value for throttledPeriods
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("throttled_periods")
public final Builder throttledPeriods(Long throttledPeriods) {
this.throttledPeriods = Objects.requireNonNull(throttledPeriods, "throttledPeriods");
initBits &= ~INIT_BIT_THROTTLED_PERIODS;
return this;
}
/**
* Initializes the value for the {@link CpuStats.ThrottlingData#throttledTime() throttledTime} attribute.
* @param throttledTime The value for throttledTime
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("throttled_time")
public final Builder throttledTime(Long throttledTime) {
this.throttledTime = Objects.requireNonNull(throttledTime, "throttledTime");
initBits &= ~INIT_BIT_THROTTLED_TIME;
return this;
}
/**
* Builds a new {@link ImmutableCpuStats.ThrottlingData ThrottlingData}.
* @return An immutable instance of ThrottlingData
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCpuStats.ThrottlingData build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableCpuStats.ThrottlingData(periods, throttledPeriods, throttledTime);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_PERIODS) != 0) attributes.add("periods");
if ((initBits & INIT_BIT_THROTTLED_PERIODS) != 0) attributes.add("throttledPeriods");
if ((initBits & INIT_BIT_THROTTLED_TIME) != 0) attributes.add("throttledTime");
return "Cannot build ThrottlingData, some of required attributes are not set " + attributes;
}
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}