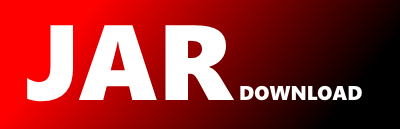
org.mandas.docker.client.messages.ImmutableMemoryStats Maven / Gradle / Ivy
package org.mandas.docker.client.messages;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import org.mandas.docker.Nullable;
/**
* Immutable implementation of {@link MemoryStats}.
*
* Use the builder to create immutable instances:
* {@code ImmutableMemoryStats.builder()}.
*/
@SuppressWarnings({"all"})
final class ImmutableMemoryStats implements MemoryStats {
private final @Nullable MemoryStats.Stats stats;
private final @Nullable Long maxUsage;
private final @Nullable Long usage;
private final @Nullable Long failcnt;
private final @Nullable Long limit;
private ImmutableMemoryStats(
@Nullable MemoryStats.Stats stats,
@Nullable Long maxUsage,
@Nullable Long usage,
@Nullable Long failcnt,
@Nullable Long limit) {
this.stats = stats;
this.maxUsage = maxUsage;
this.usage = usage;
this.failcnt = failcnt;
this.limit = limit;
}
/**
* @return The value of the {@code stats} attribute
*/
@JsonProperty("stats")
@Override
public @Nullable MemoryStats.Stats stats() {
return stats;
}
/**
* @return The value of the {@code maxUsage} attribute
*/
@JsonProperty("max_usage")
@Override
public @Nullable Long maxUsage() {
return maxUsage;
}
/**
* @return The value of the {@code usage} attribute
*/
@JsonProperty("usage")
@Override
public @Nullable Long usage() {
return usage;
}
/**
* @return The value of the {@code failcnt} attribute
*/
@JsonProperty("failcnt")
@Override
public @Nullable Long failcnt() {
return failcnt;
}
/**
* @return The value of the {@code limit} attribute
*/
@JsonProperty("limit")
@Override
public @Nullable Long limit() {
return limit;
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats#stats() stats} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for stats (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats withStats(@Nullable MemoryStats.Stats value) {
if (this.stats == value) return this;
return new ImmutableMemoryStats(value, this.maxUsage, this.usage, this.failcnt, this.limit);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats#maxUsage() maxUsage} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for maxUsage (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats withMaxUsage(@Nullable Long value) {
if (Objects.equals(this.maxUsage, value)) return this;
return new ImmutableMemoryStats(this.stats, value, this.usage, this.failcnt, this.limit);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats#usage() usage} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for usage (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats withUsage(@Nullable Long value) {
if (Objects.equals(this.usage, value)) return this;
return new ImmutableMemoryStats(this.stats, this.maxUsage, value, this.failcnt, this.limit);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats#failcnt() failcnt} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for failcnt (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats withFailcnt(@Nullable Long value) {
if (Objects.equals(this.failcnt, value)) return this;
return new ImmutableMemoryStats(this.stats, this.maxUsage, this.usage, value, this.limit);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats#limit() limit} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for limit (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats withLimit(@Nullable Long value) {
if (Objects.equals(this.limit, value)) return this;
return new ImmutableMemoryStats(this.stats, this.maxUsage, this.usage, this.failcnt, value);
}
/**
* This instance is equal to all instances of {@code ImmutableMemoryStats} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableMemoryStats
&& equalTo(0, (ImmutableMemoryStats) another);
}
private boolean equalTo(int synthetic, ImmutableMemoryStats another) {
return Objects.equals(stats, another.stats)
&& Objects.equals(maxUsage, another.maxUsage)
&& Objects.equals(usage, another.usage)
&& Objects.equals(failcnt, another.failcnt)
&& Objects.equals(limit, another.limit);
}
/**
* Computes a hash code from attributes: {@code stats}, {@code maxUsage}, {@code usage}, {@code failcnt}, {@code limit}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(stats);
h += (h << 5) + Objects.hashCode(maxUsage);
h += (h << 5) + Objects.hashCode(usage);
h += (h << 5) + Objects.hashCode(failcnt);
h += (h << 5) + Objects.hashCode(limit);
return h;
}
/**
* Prints the immutable value {@code MemoryStats} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "MemoryStats{"
+ "stats=" + stats
+ ", maxUsage=" + maxUsage
+ ", usage=" + usage
+ ", failcnt=" + failcnt
+ ", limit=" + limit
+ "}";
}
/**
* Creates an immutable copy of a {@link MemoryStats} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable MemoryStats instance
*/
public static ImmutableMemoryStats copyOf(MemoryStats instance) {
if (instance instanceof ImmutableMemoryStats) {
return (ImmutableMemoryStats) instance;
}
return ImmutableMemoryStats.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableMemoryStats ImmutableMemoryStats}.
*
* ImmutableMemoryStats.builder()
* .stats(org.mandas.docker.client.messages.MemoryStats.Stats | null) // nullable {@link MemoryStats#stats() stats}
* .maxUsage(Long | null) // nullable {@link MemoryStats#maxUsage() maxUsage}
* .usage(Long | null) // nullable {@link MemoryStats#usage() usage}
* .failcnt(Long | null) // nullable {@link MemoryStats#failcnt() failcnt}
* .limit(Long | null) // nullable {@link MemoryStats#limit() limit}
* .build();
*
* @return A new ImmutableMemoryStats builder
*/
public static ImmutableMemoryStats.Builder builder() {
return new ImmutableMemoryStats.Builder();
}
/**
* Builds instances of type {@link ImmutableMemoryStats ImmutableMemoryStats}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder {
private MemoryStats.Stats stats;
private Long maxUsage;
private Long usage;
private Long failcnt;
private Long limit;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code MemoryStats} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(MemoryStats instance) {
Objects.requireNonNull(instance, "instance");
@Nullable MemoryStats.Stats statsValue = instance.stats();
if (statsValue != null) {
stats(statsValue);
}
@Nullable Long maxUsageValue = instance.maxUsage();
if (maxUsageValue != null) {
maxUsage(maxUsageValue);
}
@Nullable Long usageValue = instance.usage();
if (usageValue != null) {
usage(usageValue);
}
@Nullable Long failcntValue = instance.failcnt();
if (failcntValue != null) {
failcnt(failcntValue);
}
@Nullable Long limitValue = instance.limit();
if (limitValue != null) {
limit(limitValue);
}
return this;
}
/**
* Initializes the value for the {@link MemoryStats#stats() stats} attribute.
* @param stats The value for stats (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("stats")
public final Builder stats(@Nullable MemoryStats.Stats stats) {
this.stats = stats;
return this;
}
/**
* Initializes the value for the {@link MemoryStats#maxUsage() maxUsage} attribute.
* @param maxUsage The value for maxUsage (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("max_usage")
public final Builder maxUsage(@Nullable Long maxUsage) {
this.maxUsage = maxUsage;
return this;
}
/**
* Initializes the value for the {@link MemoryStats#usage() usage} attribute.
* @param usage The value for usage (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("usage")
public final Builder usage(@Nullable Long usage) {
this.usage = usage;
return this;
}
/**
* Initializes the value for the {@link MemoryStats#failcnt() failcnt} attribute.
* @param failcnt The value for failcnt (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("failcnt")
public final Builder failcnt(@Nullable Long failcnt) {
this.failcnt = failcnt;
return this;
}
/**
* Initializes the value for the {@link MemoryStats#limit() limit} attribute.
* @param limit The value for limit (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("limit")
public final Builder limit(@Nullable Long limit) {
this.limit = limit;
return this;
}
/**
* Builds a new {@link ImmutableMemoryStats ImmutableMemoryStats}.
* @return An immutable instance of MemoryStats
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableMemoryStats build() {
return new ImmutableMemoryStats(stats, maxUsage, usage, failcnt, limit);
}
}
/**
* Immutable implementation of {@link MemoryStats.Stats}.
*
* Use the builder to create immutable instances:
* {@code ImmutableMemoryStats.Stats.builder()}.
*/
static final class Stats implements MemoryStats.Stats {
private final Long activeFile;
private final Long totalActiveFile;
private final Long inactiveFile;
private final Long totalInactiveFile;
private final Long cache;
private final Long totalCache;
private final Long activeAnon;
private final Long totalActiveAnon;
private final Long inactiveAnon;
private final Long totalInactiveAnon;
private final BigInteger hierarchicalMemoryLimit;
private final Long mappedFile;
private final Long totalMappedFile;
private final Long pgmajfault;
private final Long totalPgmajfault;
private final Long pgpgin;
private final Long totalPgpgin;
private final Long pgpgout;
private final Long totalPgpgout;
private final Long pgfault;
private final Long totalPgfault;
private final Long rss;
private final Long totalRss;
private final Long rssHuge;
private final Long totalRssHuge;
private final Long unevictable;
private final Long totalUnevictable;
private final @Nullable Long totalWriteback;
private final @Nullable Long writeback;
private Stats(
Long activeFile,
Long totalActiveFile,
Long inactiveFile,
Long totalInactiveFile,
Long cache,
Long totalCache,
Long activeAnon,
Long totalActiveAnon,
Long inactiveAnon,
Long totalInactiveAnon,
BigInteger hierarchicalMemoryLimit,
Long mappedFile,
Long totalMappedFile,
Long pgmajfault,
Long totalPgmajfault,
Long pgpgin,
Long totalPgpgin,
Long pgpgout,
Long totalPgpgout,
Long pgfault,
Long totalPgfault,
Long rss,
Long totalRss,
Long rssHuge,
Long totalRssHuge,
Long unevictable,
Long totalUnevictable,
@Nullable Long totalWriteback,
@Nullable Long writeback) {
this.activeFile = activeFile;
this.totalActiveFile = totalActiveFile;
this.inactiveFile = inactiveFile;
this.totalInactiveFile = totalInactiveFile;
this.cache = cache;
this.totalCache = totalCache;
this.activeAnon = activeAnon;
this.totalActiveAnon = totalActiveAnon;
this.inactiveAnon = inactiveAnon;
this.totalInactiveAnon = totalInactiveAnon;
this.hierarchicalMemoryLimit = hierarchicalMemoryLimit;
this.mappedFile = mappedFile;
this.totalMappedFile = totalMappedFile;
this.pgmajfault = pgmajfault;
this.totalPgmajfault = totalPgmajfault;
this.pgpgin = pgpgin;
this.totalPgpgin = totalPgpgin;
this.pgpgout = pgpgout;
this.totalPgpgout = totalPgpgout;
this.pgfault = pgfault;
this.totalPgfault = totalPgfault;
this.rss = rss;
this.totalRss = totalRss;
this.rssHuge = rssHuge;
this.totalRssHuge = totalRssHuge;
this.unevictable = unevictable;
this.totalUnevictable = totalUnevictable;
this.totalWriteback = totalWriteback;
this.writeback = writeback;
}
/**
* @return The value of the {@code activeFile} attribute
*/
@JsonProperty("active_file")
@Override
public Long activeFile() {
return activeFile;
}
/**
* @return The value of the {@code totalActiveFile} attribute
*/
@JsonProperty("total_active_file")
@Override
public Long totalActiveFile() {
return totalActiveFile;
}
/**
* @return The value of the {@code inactiveFile} attribute
*/
@JsonProperty("inactive_file")
@Override
public Long inactiveFile() {
return inactiveFile;
}
/**
* @return The value of the {@code totalInactiveFile} attribute
*/
@JsonProperty("total_inactive_file")
@Override
public Long totalInactiveFile() {
return totalInactiveFile;
}
/**
* @return The value of the {@code cache} attribute
*/
@JsonProperty("cache")
@Override
public Long cache() {
return cache;
}
/**
* @return The value of the {@code totalCache} attribute
*/
@JsonProperty("total_cache")
@Override
public Long totalCache() {
return totalCache;
}
/**
* @return The value of the {@code activeAnon} attribute
*/
@JsonProperty("active_anon")
@Override
public Long activeAnon() {
return activeAnon;
}
/**
* @return The value of the {@code totalActiveAnon} attribute
*/
@JsonProperty("total_active_anon")
@Override
public Long totalActiveAnon() {
return totalActiveAnon;
}
/**
* @return The value of the {@code inactiveAnon} attribute
*/
@JsonProperty("inactive_anon")
@Override
public Long inactiveAnon() {
return inactiveAnon;
}
/**
* @return The value of the {@code totalInactiveAnon} attribute
*/
@JsonProperty("total_inactive_anon")
@Override
public Long totalInactiveAnon() {
return totalInactiveAnon;
}
/**
* @return The value of the {@code hierarchicalMemoryLimit} attribute
*/
@JsonProperty("hierarchical_memory_limit")
@Override
public BigInteger hierarchicalMemoryLimit() {
return hierarchicalMemoryLimit;
}
/**
* @return The value of the {@code mappedFile} attribute
*/
@JsonProperty("mapped_file")
@Override
public Long mappedFile() {
return mappedFile;
}
/**
* @return The value of the {@code totalMappedFile} attribute
*/
@JsonProperty("total_mapped_file")
@Override
public Long totalMappedFile() {
return totalMappedFile;
}
/**
* @return The value of the {@code pgmajfault} attribute
*/
@JsonProperty("pgmajfault")
@Override
public Long pgmajfault() {
return pgmajfault;
}
/**
* @return The value of the {@code totalPgmajfault} attribute
*/
@JsonProperty("total_pgmajfault")
@Override
public Long totalPgmajfault() {
return totalPgmajfault;
}
/**
* @return The value of the {@code pgpgin} attribute
*/
@JsonProperty("pgpgin")
@Override
public Long pgpgin() {
return pgpgin;
}
/**
* @return The value of the {@code totalPgpgin} attribute
*/
@JsonProperty("total_pgpgin")
@Override
public Long totalPgpgin() {
return totalPgpgin;
}
/**
* @return The value of the {@code pgpgout} attribute
*/
@JsonProperty("pgpgout")
@Override
public Long pgpgout() {
return pgpgout;
}
/**
* @return The value of the {@code totalPgpgout} attribute
*/
@JsonProperty("total_pgpgout")
@Override
public Long totalPgpgout() {
return totalPgpgout;
}
/**
* @return The value of the {@code pgfault} attribute
*/
@JsonProperty("pgfault")
@Override
public Long pgfault() {
return pgfault;
}
/**
* @return The value of the {@code totalPgfault} attribute
*/
@JsonProperty("total_pgfault")
@Override
public Long totalPgfault() {
return totalPgfault;
}
/**
* @return The value of the {@code rss} attribute
*/
@JsonProperty("rss")
@Override
public Long rss() {
return rss;
}
/**
* @return The value of the {@code totalRss} attribute
*/
@JsonProperty("total_rss")
@Override
public Long totalRss() {
return totalRss;
}
/**
* @return The value of the {@code rssHuge} attribute
*/
@JsonProperty("rss_huge")
@Override
public Long rssHuge() {
return rssHuge;
}
/**
* @return The value of the {@code totalRssHuge} attribute
*/
@JsonProperty("total_rss_huge")
@Override
public Long totalRssHuge() {
return totalRssHuge;
}
/**
* @return The value of the {@code unevictable} attribute
*/
@JsonProperty("unevictable")
@Override
public Long unevictable() {
return unevictable;
}
/**
* @return The value of the {@code totalUnevictable} attribute
*/
@JsonProperty("total_unevictable")
@Override
public Long totalUnevictable() {
return totalUnevictable;
}
/**
* @return The value of the {@code totalWriteback} attribute
*/
@JsonProperty("total_writeback")
@Override
public @Nullable Long totalWriteback() {
return totalWriteback;
}
/**
* @return The value of the {@code writeback} attribute
*/
@JsonProperty("writeback")
@Override
public @Nullable Long writeback() {
return writeback;
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#activeFile() activeFile} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for activeFile
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withActiveFile(Long value) {
Long newValue = Objects.requireNonNull(value, "activeFile");
if (this.activeFile.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
newValue,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalActiveFile() totalActiveFile} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalActiveFile
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalActiveFile(Long value) {
Long newValue = Objects.requireNonNull(value, "totalActiveFile");
if (this.totalActiveFile.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
newValue,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#inactiveFile() inactiveFile} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for inactiveFile
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withInactiveFile(Long value) {
Long newValue = Objects.requireNonNull(value, "inactiveFile");
if (this.inactiveFile.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
newValue,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalInactiveFile() totalInactiveFile} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalInactiveFile
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalInactiveFile(Long value) {
Long newValue = Objects.requireNonNull(value, "totalInactiveFile");
if (this.totalInactiveFile.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
newValue,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#cache() cache} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for cache
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withCache(Long value) {
Long newValue = Objects.requireNonNull(value, "cache");
if (this.cache.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
newValue,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalCache() totalCache} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalCache
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalCache(Long value) {
Long newValue = Objects.requireNonNull(value, "totalCache");
if (this.totalCache.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
newValue,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#activeAnon() activeAnon} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for activeAnon
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withActiveAnon(Long value) {
Long newValue = Objects.requireNonNull(value, "activeAnon");
if (this.activeAnon.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
newValue,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalActiveAnon() totalActiveAnon} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalActiveAnon
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalActiveAnon(Long value) {
Long newValue = Objects.requireNonNull(value, "totalActiveAnon");
if (this.totalActiveAnon.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
newValue,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#inactiveAnon() inactiveAnon} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for inactiveAnon
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withInactiveAnon(Long value) {
Long newValue = Objects.requireNonNull(value, "inactiveAnon");
if (this.inactiveAnon.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
newValue,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalInactiveAnon() totalInactiveAnon} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalInactiveAnon
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalInactiveAnon(Long value) {
Long newValue = Objects.requireNonNull(value, "totalInactiveAnon");
if (this.totalInactiveAnon.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
newValue,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#hierarchicalMemoryLimit() hierarchicalMemoryLimit} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for hierarchicalMemoryLimit
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withHierarchicalMemoryLimit(BigInteger value) {
BigInteger newValue = Objects.requireNonNull(value, "hierarchicalMemoryLimit");
if (this.hierarchicalMemoryLimit.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
newValue,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#mappedFile() mappedFile} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for mappedFile
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withMappedFile(Long value) {
Long newValue = Objects.requireNonNull(value, "mappedFile");
if (this.mappedFile.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
newValue,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalMappedFile() totalMappedFile} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalMappedFile
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalMappedFile(Long value) {
Long newValue = Objects.requireNonNull(value, "totalMappedFile");
if (this.totalMappedFile.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
newValue,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#pgmajfault() pgmajfault} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for pgmajfault
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withPgmajfault(Long value) {
Long newValue = Objects.requireNonNull(value, "pgmajfault");
if (this.pgmajfault.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
newValue,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalPgmajfault() totalPgmajfault} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalPgmajfault
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalPgmajfault(Long value) {
Long newValue = Objects.requireNonNull(value, "totalPgmajfault");
if (this.totalPgmajfault.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
newValue,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#pgpgin() pgpgin} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for pgpgin
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withPgpgin(Long value) {
Long newValue = Objects.requireNonNull(value, "pgpgin");
if (this.pgpgin.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
newValue,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalPgpgin() totalPgpgin} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalPgpgin
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalPgpgin(Long value) {
Long newValue = Objects.requireNonNull(value, "totalPgpgin");
if (this.totalPgpgin.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
newValue,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#pgpgout() pgpgout} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for pgpgout
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withPgpgout(Long value) {
Long newValue = Objects.requireNonNull(value, "pgpgout");
if (this.pgpgout.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
newValue,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalPgpgout() totalPgpgout} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalPgpgout
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalPgpgout(Long value) {
Long newValue = Objects.requireNonNull(value, "totalPgpgout");
if (this.totalPgpgout.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
newValue,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#pgfault() pgfault} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for pgfault
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withPgfault(Long value) {
Long newValue = Objects.requireNonNull(value, "pgfault");
if (this.pgfault.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
newValue,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalPgfault() totalPgfault} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalPgfault
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalPgfault(Long value) {
Long newValue = Objects.requireNonNull(value, "totalPgfault");
if (this.totalPgfault.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
newValue,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#rss() rss} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for rss
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withRss(Long value) {
Long newValue = Objects.requireNonNull(value, "rss");
if (this.rss.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
newValue,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalRss() totalRss} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalRss
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalRss(Long value) {
Long newValue = Objects.requireNonNull(value, "totalRss");
if (this.totalRss.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
newValue,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#rssHuge() rssHuge} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for rssHuge
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withRssHuge(Long value) {
Long newValue = Objects.requireNonNull(value, "rssHuge");
if (this.rssHuge.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
newValue,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalRssHuge() totalRssHuge} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalRssHuge
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalRssHuge(Long value) {
Long newValue = Objects.requireNonNull(value, "totalRssHuge");
if (this.totalRssHuge.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
newValue,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#unevictable() unevictable} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for unevictable
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withUnevictable(Long value) {
Long newValue = Objects.requireNonNull(value, "unevictable");
if (this.unevictable.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
newValue,
this.totalUnevictable,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalUnevictable() totalUnevictable} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalUnevictable
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalUnevictable(Long value) {
Long newValue = Objects.requireNonNull(value, "totalUnevictable");
if (this.totalUnevictable.equals(newValue)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
newValue,
this.totalWriteback,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#totalWriteback() totalWriteback} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for totalWriteback (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withTotalWriteback(@Nullable Long value) {
if (Objects.equals(this.totalWriteback, value)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
value,
this.writeback);
}
/**
* Copy the current immutable object by setting a value for the {@link MemoryStats.Stats#writeback() writeback} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for writeback (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableMemoryStats.Stats withWriteback(@Nullable Long value) {
if (Objects.equals(this.writeback, value)) return this;
return new ImmutableMemoryStats.Stats(
this.activeFile,
this.totalActiveFile,
this.inactiveFile,
this.totalInactiveFile,
this.cache,
this.totalCache,
this.activeAnon,
this.totalActiveAnon,
this.inactiveAnon,
this.totalInactiveAnon,
this.hierarchicalMemoryLimit,
this.mappedFile,
this.totalMappedFile,
this.pgmajfault,
this.totalPgmajfault,
this.pgpgin,
this.totalPgpgin,
this.pgpgout,
this.totalPgpgout,
this.pgfault,
this.totalPgfault,
this.rss,
this.totalRss,
this.rssHuge,
this.totalRssHuge,
this.unevictable,
this.totalUnevictable,
this.totalWriteback,
value);
}
/**
* This instance is equal to all instances of {@code Stats} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableMemoryStats.Stats
&& equalTo(0, (ImmutableMemoryStats.Stats) another);
}
private boolean equalTo(int synthetic, ImmutableMemoryStats.Stats another) {
return activeFile.equals(another.activeFile)
&& totalActiveFile.equals(another.totalActiveFile)
&& inactiveFile.equals(another.inactiveFile)
&& totalInactiveFile.equals(another.totalInactiveFile)
&& cache.equals(another.cache)
&& totalCache.equals(another.totalCache)
&& activeAnon.equals(another.activeAnon)
&& totalActiveAnon.equals(another.totalActiveAnon)
&& inactiveAnon.equals(another.inactiveAnon)
&& totalInactiveAnon.equals(another.totalInactiveAnon)
&& hierarchicalMemoryLimit.equals(another.hierarchicalMemoryLimit)
&& mappedFile.equals(another.mappedFile)
&& totalMappedFile.equals(another.totalMappedFile)
&& pgmajfault.equals(another.pgmajfault)
&& totalPgmajfault.equals(another.totalPgmajfault)
&& pgpgin.equals(another.pgpgin)
&& totalPgpgin.equals(another.totalPgpgin)
&& pgpgout.equals(another.pgpgout)
&& totalPgpgout.equals(another.totalPgpgout)
&& pgfault.equals(another.pgfault)
&& totalPgfault.equals(another.totalPgfault)
&& rss.equals(another.rss)
&& totalRss.equals(another.totalRss)
&& rssHuge.equals(another.rssHuge)
&& totalRssHuge.equals(another.totalRssHuge)
&& unevictable.equals(another.unevictable)
&& totalUnevictable.equals(another.totalUnevictable)
&& Objects.equals(totalWriteback, another.totalWriteback)
&& Objects.equals(writeback, another.writeback);
}
/**
* Computes a hash code from attributes: {@code activeFile}, {@code totalActiveFile}, {@code inactiveFile}, {@code totalInactiveFile}, {@code cache}, {@code totalCache}, {@code activeAnon}, {@code totalActiveAnon}, {@code inactiveAnon}, {@code totalInactiveAnon}, {@code hierarchicalMemoryLimit}, {@code mappedFile}, {@code totalMappedFile}, {@code pgmajfault}, {@code totalPgmajfault}, {@code pgpgin}, {@code totalPgpgin}, {@code pgpgout}, {@code totalPgpgout}, {@code pgfault}, {@code totalPgfault}, {@code rss}, {@code totalRss}, {@code rssHuge}, {@code totalRssHuge}, {@code unevictable}, {@code totalUnevictable}, {@code totalWriteback}, {@code writeback}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + activeFile.hashCode();
h += (h << 5) + totalActiveFile.hashCode();
h += (h << 5) + inactiveFile.hashCode();
h += (h << 5) + totalInactiveFile.hashCode();
h += (h << 5) + cache.hashCode();
h += (h << 5) + totalCache.hashCode();
h += (h << 5) + activeAnon.hashCode();
h += (h << 5) + totalActiveAnon.hashCode();
h += (h << 5) + inactiveAnon.hashCode();
h += (h << 5) + totalInactiveAnon.hashCode();
h += (h << 5) + hierarchicalMemoryLimit.hashCode();
h += (h << 5) + mappedFile.hashCode();
h += (h << 5) + totalMappedFile.hashCode();
h += (h << 5) + pgmajfault.hashCode();
h += (h << 5) + totalPgmajfault.hashCode();
h += (h << 5) + pgpgin.hashCode();
h += (h << 5) + totalPgpgin.hashCode();
h += (h << 5) + pgpgout.hashCode();
h += (h << 5) + totalPgpgout.hashCode();
h += (h << 5) + pgfault.hashCode();
h += (h << 5) + totalPgfault.hashCode();
h += (h << 5) + rss.hashCode();
h += (h << 5) + totalRss.hashCode();
h += (h << 5) + rssHuge.hashCode();
h += (h << 5) + totalRssHuge.hashCode();
h += (h << 5) + unevictable.hashCode();
h += (h << 5) + totalUnevictable.hashCode();
h += (h << 5) + Objects.hashCode(totalWriteback);
h += (h << 5) + Objects.hashCode(writeback);
return h;
}
/**
* Prints the immutable value {@code Stats} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "Stats{"
+ "activeFile=" + activeFile
+ ", totalActiveFile=" + totalActiveFile
+ ", inactiveFile=" + inactiveFile
+ ", totalInactiveFile=" + totalInactiveFile
+ ", cache=" + cache
+ ", totalCache=" + totalCache
+ ", activeAnon=" + activeAnon
+ ", totalActiveAnon=" + totalActiveAnon
+ ", inactiveAnon=" + inactiveAnon
+ ", totalInactiveAnon=" + totalInactiveAnon
+ ", hierarchicalMemoryLimit=" + hierarchicalMemoryLimit
+ ", mappedFile=" + mappedFile
+ ", totalMappedFile=" + totalMappedFile
+ ", pgmajfault=" + pgmajfault
+ ", totalPgmajfault=" + totalPgmajfault
+ ", pgpgin=" + pgpgin
+ ", totalPgpgin=" + totalPgpgin
+ ", pgpgout=" + pgpgout
+ ", totalPgpgout=" + totalPgpgout
+ ", pgfault=" + pgfault
+ ", totalPgfault=" + totalPgfault
+ ", rss=" + rss
+ ", totalRss=" + totalRss
+ ", rssHuge=" + rssHuge
+ ", totalRssHuge=" + totalRssHuge
+ ", unevictable=" + unevictable
+ ", totalUnevictable=" + totalUnevictable
+ ", totalWriteback=" + totalWriteback
+ ", writeback=" + writeback
+ "}";
}
/**
* Creates an immutable copy of a {@link MemoryStats.Stats} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Stats instance
*/
public static ImmutableMemoryStats.Stats copyOf(MemoryStats.Stats instance) {
if (instance instanceof ImmutableMemoryStats.Stats) {
return (ImmutableMemoryStats.Stats) instance;
}
return ImmutableMemoryStats.Stats.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableMemoryStats.Stats Stats}.
*
* ImmutableMemoryStats.Stats.builder()
* .activeFile(Long) // required {@link MemoryStats.Stats#activeFile() activeFile}
* .totalActiveFile(Long) // required {@link MemoryStats.Stats#totalActiveFile() totalActiveFile}
* .inactiveFile(Long) // required {@link MemoryStats.Stats#inactiveFile() inactiveFile}
* .totalInactiveFile(Long) // required {@link MemoryStats.Stats#totalInactiveFile() totalInactiveFile}
* .cache(Long) // required {@link MemoryStats.Stats#cache() cache}
* .totalCache(Long) // required {@link MemoryStats.Stats#totalCache() totalCache}
* .activeAnon(Long) // required {@link MemoryStats.Stats#activeAnon() activeAnon}
* .totalActiveAnon(Long) // required {@link MemoryStats.Stats#totalActiveAnon() totalActiveAnon}
* .inactiveAnon(Long) // required {@link MemoryStats.Stats#inactiveAnon() inactiveAnon}
* .totalInactiveAnon(Long) // required {@link MemoryStats.Stats#totalInactiveAnon() totalInactiveAnon}
* .hierarchicalMemoryLimit(java.math.BigInteger) // required {@link MemoryStats.Stats#hierarchicalMemoryLimit() hierarchicalMemoryLimit}
* .mappedFile(Long) // required {@link MemoryStats.Stats#mappedFile() mappedFile}
* .totalMappedFile(Long) // required {@link MemoryStats.Stats#totalMappedFile() totalMappedFile}
* .pgmajfault(Long) // required {@link MemoryStats.Stats#pgmajfault() pgmajfault}
* .totalPgmajfault(Long) // required {@link MemoryStats.Stats#totalPgmajfault() totalPgmajfault}
* .pgpgin(Long) // required {@link MemoryStats.Stats#pgpgin() pgpgin}
* .totalPgpgin(Long) // required {@link MemoryStats.Stats#totalPgpgin() totalPgpgin}
* .pgpgout(Long) // required {@link MemoryStats.Stats#pgpgout() pgpgout}
* .totalPgpgout(Long) // required {@link MemoryStats.Stats#totalPgpgout() totalPgpgout}
* .pgfault(Long) // required {@link MemoryStats.Stats#pgfault() pgfault}
* .totalPgfault(Long) // required {@link MemoryStats.Stats#totalPgfault() totalPgfault}
* .rss(Long) // required {@link MemoryStats.Stats#rss() rss}
* .totalRss(Long) // required {@link MemoryStats.Stats#totalRss() totalRss}
* .rssHuge(Long) // required {@link MemoryStats.Stats#rssHuge() rssHuge}
* .totalRssHuge(Long) // required {@link MemoryStats.Stats#totalRssHuge() totalRssHuge}
* .unevictable(Long) // required {@link MemoryStats.Stats#unevictable() unevictable}
* .totalUnevictable(Long) // required {@link MemoryStats.Stats#totalUnevictable() totalUnevictable}
* .totalWriteback(Long | null) // nullable {@link MemoryStats.Stats#totalWriteback() totalWriteback}
* .writeback(Long | null) // nullable {@link MemoryStats.Stats#writeback() writeback}
* .build();
*
* @return A new Stats builder
*/
public static ImmutableMemoryStats.Stats.Builder builder() {
return new ImmutableMemoryStats.Stats.Builder();
}
/**
* Builds instances of type {@link ImmutableMemoryStats.Stats Stats}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder {
private static final long INIT_BIT_ACTIVE_FILE = 0x1L;
private static final long INIT_BIT_TOTAL_ACTIVE_FILE = 0x2L;
private static final long INIT_BIT_INACTIVE_FILE = 0x4L;
private static final long INIT_BIT_TOTAL_INACTIVE_FILE = 0x8L;
private static final long INIT_BIT_CACHE = 0x10L;
private static final long INIT_BIT_TOTAL_CACHE = 0x20L;
private static final long INIT_BIT_ACTIVE_ANON = 0x40L;
private static final long INIT_BIT_TOTAL_ACTIVE_ANON = 0x80L;
private static final long INIT_BIT_INACTIVE_ANON = 0x100L;
private static final long INIT_BIT_TOTAL_INACTIVE_ANON = 0x200L;
private static final long INIT_BIT_HIERARCHICAL_MEMORY_LIMIT = 0x400L;
private static final long INIT_BIT_MAPPED_FILE = 0x800L;
private static final long INIT_BIT_TOTAL_MAPPED_FILE = 0x1000L;
private static final long INIT_BIT_PGMAJFAULT = 0x2000L;
private static final long INIT_BIT_TOTAL_PGMAJFAULT = 0x4000L;
private static final long INIT_BIT_PGPGIN = 0x8000L;
private static final long INIT_BIT_TOTAL_PGPGIN = 0x10000L;
private static final long INIT_BIT_PGPGOUT = 0x20000L;
private static final long INIT_BIT_TOTAL_PGPGOUT = 0x40000L;
private static final long INIT_BIT_PGFAULT = 0x80000L;
private static final long INIT_BIT_TOTAL_PGFAULT = 0x100000L;
private static final long INIT_BIT_RSS = 0x200000L;
private static final long INIT_BIT_TOTAL_RSS = 0x400000L;
private static final long INIT_BIT_RSS_HUGE = 0x800000L;
private static final long INIT_BIT_TOTAL_RSS_HUGE = 0x1000000L;
private static final long INIT_BIT_UNEVICTABLE = 0x2000000L;
private static final long INIT_BIT_TOTAL_UNEVICTABLE = 0x4000000L;
private long initBits = 0x7ffffffL;
private Long activeFile;
private Long totalActiveFile;
private Long inactiveFile;
private Long totalInactiveFile;
private Long cache;
private Long totalCache;
private Long activeAnon;
private Long totalActiveAnon;
private Long inactiveAnon;
private Long totalInactiveAnon;
private BigInteger hierarchicalMemoryLimit;
private Long mappedFile;
private Long totalMappedFile;
private Long pgmajfault;
private Long totalPgmajfault;
private Long pgpgin;
private Long totalPgpgin;
private Long pgpgout;
private Long totalPgpgout;
private Long pgfault;
private Long totalPgfault;
private Long rss;
private Long totalRss;
private Long rssHuge;
private Long totalRssHuge;
private Long unevictable;
private Long totalUnevictable;
private Long totalWriteback;
private Long writeback;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Stats} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(MemoryStats.Stats instance) {
Objects.requireNonNull(instance, "instance");
this.activeFile(instance.activeFile());
this.totalActiveFile(instance.totalActiveFile());
this.inactiveFile(instance.inactiveFile());
this.totalInactiveFile(instance.totalInactiveFile());
this.cache(instance.cache());
this.totalCache(instance.totalCache());
this.activeAnon(instance.activeAnon());
this.totalActiveAnon(instance.totalActiveAnon());
this.inactiveAnon(instance.inactiveAnon());
this.totalInactiveAnon(instance.totalInactiveAnon());
this.hierarchicalMemoryLimit(instance.hierarchicalMemoryLimit());
this.mappedFile(instance.mappedFile());
this.totalMappedFile(instance.totalMappedFile());
this.pgmajfault(instance.pgmajfault());
this.totalPgmajfault(instance.totalPgmajfault());
this.pgpgin(instance.pgpgin());
this.totalPgpgin(instance.totalPgpgin());
this.pgpgout(instance.pgpgout());
this.totalPgpgout(instance.totalPgpgout());
this.pgfault(instance.pgfault());
this.totalPgfault(instance.totalPgfault());
this.rss(instance.rss());
this.totalRss(instance.totalRss());
this.rssHuge(instance.rssHuge());
this.totalRssHuge(instance.totalRssHuge());
this.unevictable(instance.unevictable());
this.totalUnevictable(instance.totalUnevictable());
@Nullable Long totalWritebackValue = instance.totalWriteback();
if (totalWritebackValue != null) {
totalWriteback(totalWritebackValue);
}
@Nullable Long writebackValue = instance.writeback();
if (writebackValue != null) {
writeback(writebackValue);
}
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#activeFile() activeFile} attribute.
* @param activeFile The value for activeFile
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("active_file")
public final Builder activeFile(Long activeFile) {
this.activeFile = Objects.requireNonNull(activeFile, "activeFile");
initBits &= ~INIT_BIT_ACTIVE_FILE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalActiveFile() totalActiveFile} attribute.
* @param totalActiveFile The value for totalActiveFile
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_active_file")
public final Builder totalActiveFile(Long totalActiveFile) {
this.totalActiveFile = Objects.requireNonNull(totalActiveFile, "totalActiveFile");
initBits &= ~INIT_BIT_TOTAL_ACTIVE_FILE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#inactiveFile() inactiveFile} attribute.
* @param inactiveFile The value for inactiveFile
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("inactive_file")
public final Builder inactiveFile(Long inactiveFile) {
this.inactiveFile = Objects.requireNonNull(inactiveFile, "inactiveFile");
initBits &= ~INIT_BIT_INACTIVE_FILE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalInactiveFile() totalInactiveFile} attribute.
* @param totalInactiveFile The value for totalInactiveFile
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_inactive_file")
public final Builder totalInactiveFile(Long totalInactiveFile) {
this.totalInactiveFile = Objects.requireNonNull(totalInactiveFile, "totalInactiveFile");
initBits &= ~INIT_BIT_TOTAL_INACTIVE_FILE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#cache() cache} attribute.
* @param cache The value for cache
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("cache")
public final Builder cache(Long cache) {
this.cache = Objects.requireNonNull(cache, "cache");
initBits &= ~INIT_BIT_CACHE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalCache() totalCache} attribute.
* @param totalCache The value for totalCache
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_cache")
public final Builder totalCache(Long totalCache) {
this.totalCache = Objects.requireNonNull(totalCache, "totalCache");
initBits &= ~INIT_BIT_TOTAL_CACHE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#activeAnon() activeAnon} attribute.
* @param activeAnon The value for activeAnon
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("active_anon")
public final Builder activeAnon(Long activeAnon) {
this.activeAnon = Objects.requireNonNull(activeAnon, "activeAnon");
initBits &= ~INIT_BIT_ACTIVE_ANON;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalActiveAnon() totalActiveAnon} attribute.
* @param totalActiveAnon The value for totalActiveAnon
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_active_anon")
public final Builder totalActiveAnon(Long totalActiveAnon) {
this.totalActiveAnon = Objects.requireNonNull(totalActiveAnon, "totalActiveAnon");
initBits &= ~INIT_BIT_TOTAL_ACTIVE_ANON;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#inactiveAnon() inactiveAnon} attribute.
* @param inactiveAnon The value for inactiveAnon
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("inactive_anon")
public final Builder inactiveAnon(Long inactiveAnon) {
this.inactiveAnon = Objects.requireNonNull(inactiveAnon, "inactiveAnon");
initBits &= ~INIT_BIT_INACTIVE_ANON;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalInactiveAnon() totalInactiveAnon} attribute.
* @param totalInactiveAnon The value for totalInactiveAnon
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_inactive_anon")
public final Builder totalInactiveAnon(Long totalInactiveAnon) {
this.totalInactiveAnon = Objects.requireNonNull(totalInactiveAnon, "totalInactiveAnon");
initBits &= ~INIT_BIT_TOTAL_INACTIVE_ANON;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#hierarchicalMemoryLimit() hierarchicalMemoryLimit} attribute.
* @param hierarchicalMemoryLimit The value for hierarchicalMemoryLimit
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("hierarchical_memory_limit")
public final Builder hierarchicalMemoryLimit(BigInteger hierarchicalMemoryLimit) {
this.hierarchicalMemoryLimit = Objects.requireNonNull(hierarchicalMemoryLimit, "hierarchicalMemoryLimit");
initBits &= ~INIT_BIT_HIERARCHICAL_MEMORY_LIMIT;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#mappedFile() mappedFile} attribute.
* @param mappedFile The value for mappedFile
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("mapped_file")
public final Builder mappedFile(Long mappedFile) {
this.mappedFile = Objects.requireNonNull(mappedFile, "mappedFile");
initBits &= ~INIT_BIT_MAPPED_FILE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalMappedFile() totalMappedFile} attribute.
* @param totalMappedFile The value for totalMappedFile
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_mapped_file")
public final Builder totalMappedFile(Long totalMappedFile) {
this.totalMappedFile = Objects.requireNonNull(totalMappedFile, "totalMappedFile");
initBits &= ~INIT_BIT_TOTAL_MAPPED_FILE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#pgmajfault() pgmajfault} attribute.
* @param pgmajfault The value for pgmajfault
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("pgmajfault")
public final Builder pgmajfault(Long pgmajfault) {
this.pgmajfault = Objects.requireNonNull(pgmajfault, "pgmajfault");
initBits &= ~INIT_BIT_PGMAJFAULT;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalPgmajfault() totalPgmajfault} attribute.
* @param totalPgmajfault The value for totalPgmajfault
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_pgmajfault")
public final Builder totalPgmajfault(Long totalPgmajfault) {
this.totalPgmajfault = Objects.requireNonNull(totalPgmajfault, "totalPgmajfault");
initBits &= ~INIT_BIT_TOTAL_PGMAJFAULT;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#pgpgin() pgpgin} attribute.
* @param pgpgin The value for pgpgin
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("pgpgin")
public final Builder pgpgin(Long pgpgin) {
this.pgpgin = Objects.requireNonNull(pgpgin, "pgpgin");
initBits &= ~INIT_BIT_PGPGIN;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalPgpgin() totalPgpgin} attribute.
* @param totalPgpgin The value for totalPgpgin
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_pgpgin")
public final Builder totalPgpgin(Long totalPgpgin) {
this.totalPgpgin = Objects.requireNonNull(totalPgpgin, "totalPgpgin");
initBits &= ~INIT_BIT_TOTAL_PGPGIN;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#pgpgout() pgpgout} attribute.
* @param pgpgout The value for pgpgout
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("pgpgout")
public final Builder pgpgout(Long pgpgout) {
this.pgpgout = Objects.requireNonNull(pgpgout, "pgpgout");
initBits &= ~INIT_BIT_PGPGOUT;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalPgpgout() totalPgpgout} attribute.
* @param totalPgpgout The value for totalPgpgout
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_pgpgout")
public final Builder totalPgpgout(Long totalPgpgout) {
this.totalPgpgout = Objects.requireNonNull(totalPgpgout, "totalPgpgout");
initBits &= ~INIT_BIT_TOTAL_PGPGOUT;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#pgfault() pgfault} attribute.
* @param pgfault The value for pgfault
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("pgfault")
public final Builder pgfault(Long pgfault) {
this.pgfault = Objects.requireNonNull(pgfault, "pgfault");
initBits &= ~INIT_BIT_PGFAULT;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalPgfault() totalPgfault} attribute.
* @param totalPgfault The value for totalPgfault
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_pgfault")
public final Builder totalPgfault(Long totalPgfault) {
this.totalPgfault = Objects.requireNonNull(totalPgfault, "totalPgfault");
initBits &= ~INIT_BIT_TOTAL_PGFAULT;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#rss() rss} attribute.
* @param rss The value for rss
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("rss")
public final Builder rss(Long rss) {
this.rss = Objects.requireNonNull(rss, "rss");
initBits &= ~INIT_BIT_RSS;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalRss() totalRss} attribute.
* @param totalRss The value for totalRss
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_rss")
public final Builder totalRss(Long totalRss) {
this.totalRss = Objects.requireNonNull(totalRss, "totalRss");
initBits &= ~INIT_BIT_TOTAL_RSS;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#rssHuge() rssHuge} attribute.
* @param rssHuge The value for rssHuge
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("rss_huge")
public final Builder rssHuge(Long rssHuge) {
this.rssHuge = Objects.requireNonNull(rssHuge, "rssHuge");
initBits &= ~INIT_BIT_RSS_HUGE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalRssHuge() totalRssHuge} attribute.
* @param totalRssHuge The value for totalRssHuge
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_rss_huge")
public final Builder totalRssHuge(Long totalRssHuge) {
this.totalRssHuge = Objects.requireNonNull(totalRssHuge, "totalRssHuge");
initBits &= ~INIT_BIT_TOTAL_RSS_HUGE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#unevictable() unevictable} attribute.
* @param unevictable The value for unevictable
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("unevictable")
public final Builder unevictable(Long unevictable) {
this.unevictable = Objects.requireNonNull(unevictable, "unevictable");
initBits &= ~INIT_BIT_UNEVICTABLE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalUnevictable() totalUnevictable} attribute.
* @param totalUnevictable The value for totalUnevictable
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_unevictable")
public final Builder totalUnevictable(Long totalUnevictable) {
this.totalUnevictable = Objects.requireNonNull(totalUnevictable, "totalUnevictable");
initBits &= ~INIT_BIT_TOTAL_UNEVICTABLE;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#totalWriteback() totalWriteback} attribute.
* @param totalWriteback The value for totalWriteback (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("total_writeback")
public final Builder totalWriteback(@Nullable Long totalWriteback) {
this.totalWriteback = totalWriteback;
return this;
}
/**
* Initializes the value for the {@link MemoryStats.Stats#writeback() writeback} attribute.
* @param writeback The value for writeback (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("writeback")
public final Builder writeback(@Nullable Long writeback) {
this.writeback = writeback;
return this;
}
/**
* Builds a new {@link ImmutableMemoryStats.Stats Stats}.
* @return An immutable instance of Stats
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableMemoryStats.Stats build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableMemoryStats.Stats(
activeFile,
totalActiveFile,
inactiveFile,
totalInactiveFile,
cache,
totalCache,
activeAnon,
totalActiveAnon,
inactiveAnon,
totalInactiveAnon,
hierarchicalMemoryLimit,
mappedFile,
totalMappedFile,
pgmajfault,
totalPgmajfault,
pgpgin,
totalPgpgin,
pgpgout,
totalPgpgout,
pgfault,
totalPgfault,
rss,
totalRss,
rssHuge,
totalRssHuge,
unevictable,
totalUnevictable,
totalWriteback,
writeback);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_ACTIVE_FILE) != 0) attributes.add("activeFile");
if ((initBits & INIT_BIT_TOTAL_ACTIVE_FILE) != 0) attributes.add("totalActiveFile");
if ((initBits & INIT_BIT_INACTIVE_FILE) != 0) attributes.add("inactiveFile");
if ((initBits & INIT_BIT_TOTAL_INACTIVE_FILE) != 0) attributes.add("totalInactiveFile");
if ((initBits & INIT_BIT_CACHE) != 0) attributes.add("cache");
if ((initBits & INIT_BIT_TOTAL_CACHE) != 0) attributes.add("totalCache");
if ((initBits & INIT_BIT_ACTIVE_ANON) != 0) attributes.add("activeAnon");
if ((initBits & INIT_BIT_TOTAL_ACTIVE_ANON) != 0) attributes.add("totalActiveAnon");
if ((initBits & INIT_BIT_INACTIVE_ANON) != 0) attributes.add("inactiveAnon");
if ((initBits & INIT_BIT_TOTAL_INACTIVE_ANON) != 0) attributes.add("totalInactiveAnon");
if ((initBits & INIT_BIT_HIERARCHICAL_MEMORY_LIMIT) != 0) attributes.add("hierarchicalMemoryLimit");
if ((initBits & INIT_BIT_MAPPED_FILE) != 0) attributes.add("mappedFile");
if ((initBits & INIT_BIT_TOTAL_MAPPED_FILE) != 0) attributes.add("totalMappedFile");
if ((initBits & INIT_BIT_PGMAJFAULT) != 0) attributes.add("pgmajfault");
if ((initBits & INIT_BIT_TOTAL_PGMAJFAULT) != 0) attributes.add("totalPgmajfault");
if ((initBits & INIT_BIT_PGPGIN) != 0) attributes.add("pgpgin");
if ((initBits & INIT_BIT_TOTAL_PGPGIN) != 0) attributes.add("totalPgpgin");
if ((initBits & INIT_BIT_PGPGOUT) != 0) attributes.add("pgpgout");
if ((initBits & INIT_BIT_TOTAL_PGPGOUT) != 0) attributes.add("totalPgpgout");
if ((initBits & INIT_BIT_PGFAULT) != 0) attributes.add("pgfault");
if ((initBits & INIT_BIT_TOTAL_PGFAULT) != 0) attributes.add("totalPgfault");
if ((initBits & INIT_BIT_RSS) != 0) attributes.add("rss");
if ((initBits & INIT_BIT_TOTAL_RSS) != 0) attributes.add("totalRss");
if ((initBits & INIT_BIT_RSS_HUGE) != 0) attributes.add("rssHuge");
if ((initBits & INIT_BIT_TOTAL_RSS_HUGE) != 0) attributes.add("totalRssHuge");
if ((initBits & INIT_BIT_UNEVICTABLE) != 0) attributes.add("unevictable");
if ((initBits & INIT_BIT_TOTAL_UNEVICTABLE) != 0) attributes.add("totalUnevictable");
return "Cannot build Stats, some of required attributes are not set " + attributes;
}
}
}
}