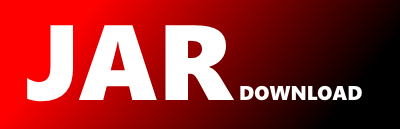
org.mandas.docker.client.messages.ImmutableNetworkSettings Maven / Gradle / Ivy
package org.mandas.docker.client.messages;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import org.mandas.docker.Nullable;
/**
* Immutable implementation of {@link NetworkSettings}.
*
* Use the builder to create immutable instances:
* {@code ImmutableNetworkSettings.builder()}.
*/
@SuppressWarnings({"all", "deprecation", "removal"})
final class ImmutableNetworkSettings implements NetworkSettings {
private final @Nullable String ipAddress;
private final @Nullable Integer ipPrefixLen;
private final @Nullable String gateway;
private final @Nullable String bridge;
private final @Nullable Map> portMapping;
private transient final @Nullable Map> ports;
private final @Nullable Map> nullValuedPorts;
private final @Nullable String macAddress;
private final @Nullable Map networks;
private final @Nullable String endpointId;
private final @Nullable String sandboxId;
private final @Nullable String sandboxKey;
private final @Nullable Boolean hairpinMode;
private final @Nullable String linkLocalIPv6Address;
private final @Nullable Integer linkLocalIPv6PrefixLen;
private final @Nullable String globalIPv6Address;
private final @Nullable Integer globalIPv6PrefixLen;
private final @Nullable String ipv6Gateway;
private ImmutableNetworkSettings(
@Nullable String ipAddress,
@Nullable Integer ipPrefixLen,
@Nullable String gateway,
@Nullable String bridge,
@Nullable Map> portMapping,
@Nullable Map> nullValuedPorts,
@Nullable String macAddress,
@Nullable Map networks,
@Nullable String endpointId,
@Nullable String sandboxId,
@Nullable String sandboxKey,
@Nullable Boolean hairpinMode,
@Nullable String linkLocalIPv6Address,
@Nullable Integer linkLocalIPv6PrefixLen,
@Nullable String globalIPv6Address,
@Nullable Integer globalIPv6PrefixLen,
@Nullable String ipv6Gateway) {
this.ipAddress = ipAddress;
this.ipPrefixLen = ipPrefixLen;
this.gateway = gateway;
this.bridge = bridge;
this.portMapping = portMapping;
this.nullValuedPorts = nullValuedPorts;
this.macAddress = macAddress;
this.networks = networks;
this.endpointId = endpointId;
this.sandboxId = sandboxId;
this.sandboxKey = sandboxKey;
this.hairpinMode = hairpinMode;
this.linkLocalIPv6Address = linkLocalIPv6Address;
this.linkLocalIPv6PrefixLen = linkLocalIPv6PrefixLen;
this.globalIPv6Address = globalIPv6Address;
this.globalIPv6PrefixLen = globalIPv6PrefixLen;
this.ipv6Gateway = ipv6Gateway;
this.ports = NetworkSettings.super.ports();
}
/**
* @return The value of the {@code ipAddress} attribute
*/
@JsonProperty("IPAddress")
@Deprecated
@Override
public @Nullable String ipAddress() {
return ipAddress;
}
/**
* @return The value of the {@code ipPrefixLen} attribute
*/
@JsonProperty("IPPrefixLen")
@Deprecated
@Override
public @Nullable Integer ipPrefixLen() {
return ipPrefixLen;
}
/**
* @return The value of the {@code gateway} attribute
*/
@JsonProperty("Gateway")
@Override
public @Nullable String gateway() {
return gateway;
}
/**
* @return The value of the {@code bridge} attribute
*/
@JsonProperty("Bridge")
@Override
public @Nullable String bridge() {
return bridge;
}
/**
* @return The value of the {@code portMapping} attribute
*/
@JsonProperty("PortMapping")
@Override
public @Nullable Map> portMapping() {
return portMapping;
}
/**
* @return The computed-at-construction value of the {@code ports} attribute
*/
@JsonProperty("ports")
@JsonIgnore
@Override
public @Nullable Map> ports() {
return ports;
}
/**
* @return The value of the {@code nullValuedPorts} attribute
*/
@JsonProperty("Ports")
@Override
public @Nullable Map> nullValuedPorts() {
return nullValuedPorts;
}
/**
* @return The value of the {@code macAddress} attribute
*/
@JsonProperty("MacAddress")
@Deprecated
@Override
public @Nullable String macAddress() {
return macAddress;
}
/**
* @return The value of the {@code networks} attribute
*/
@JsonProperty("Networks")
@Override
public @Nullable Map networks() {
return networks;
}
/**
* @return The value of the {@code endpointId} attribute
*/
@JsonProperty("EndpointID")
@Override
public @Nullable String endpointId() {
return endpointId;
}
/**
* @return The value of the {@code sandboxId} attribute
*/
@JsonProperty("SandboxID")
@Override
public @Nullable String sandboxId() {
return sandboxId;
}
/**
* @return The value of the {@code sandboxKey} attribute
*/
@JsonProperty("SandboxKey")
@Override
public @Nullable String sandboxKey() {
return sandboxKey;
}
/**
* @return The value of the {@code hairpinMode} attribute
*/
@JsonProperty("HairpinMode")
@Deprecated
@Override
public @Nullable Boolean hairpinMode() {
return hairpinMode;
}
/**
* @return The value of the {@code linkLocalIPv6Address} attribute
*/
@JsonProperty("LinkLocalIPv6Address")
@Deprecated
@Override
public @Nullable String linkLocalIPv6Address() {
return linkLocalIPv6Address;
}
/**
* @return The value of the {@code linkLocalIPv6PrefixLen} attribute
*/
@JsonProperty("LinkLocalIPv6PrefixLen")
@Deprecated
@Override
public @Nullable Integer linkLocalIPv6PrefixLen() {
return linkLocalIPv6PrefixLen;
}
/**
* @return The value of the {@code globalIPv6Address} attribute
*/
@JsonProperty("GlobalIPv6Address")
@Override
public @Nullable String globalIPv6Address() {
return globalIPv6Address;
}
/**
* @return The value of the {@code globalIPv6PrefixLen} attribute
*/
@JsonProperty("GlobalIPv6PrefixLen")
@Override
public @Nullable Integer globalIPv6PrefixLen() {
return globalIPv6PrefixLen;
}
/**
* @return The value of the {@code ipv6Gateway} attribute
*/
@JsonProperty("IPv6Gateway")
@Override
public @Nullable String ipv6Gateway() {
return ipv6Gateway;
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#ipAddress() ipAddress} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for ipAddress (can be {@code null})
* @return A modified copy of the {@code this} object
*/
@Deprecated
public final ImmutableNetworkSettings withIpAddress(@Nullable String value) {
if (Objects.equals(this.ipAddress, value)) return this;
return new ImmutableNetworkSettings(
value,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#ipPrefixLen() ipPrefixLen} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for ipPrefixLen (can be {@code null})
* @return A modified copy of the {@code this} object
*/
@Deprecated
public final ImmutableNetworkSettings withIpPrefixLen(@Nullable Integer value) {
if (Objects.equals(this.ipPrefixLen, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
value,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#gateway() gateway} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for gateway (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkSettings withGateway(@Nullable String value) {
if (Objects.equals(this.gateway, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
value,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#bridge() bridge} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for bridge (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkSettings withBridge(@Nullable String value) {
if (Objects.equals(this.bridge, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
value,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by replacing the {@link NetworkSettings#portMapping() portMapping} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the portMapping map
* @return A modified copy of {@code this} object
*/
public final ImmutableNetworkSettings withPortMapping(@Nullable Map> entries) {
if (this.portMapping == entries) return this;
@Nullable Map> newValue = entries == null ? null : createUnmodifiableMap(true, false, entries);
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
newValue,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by replacing the {@link NetworkSettings#nullValuedPorts() nullValuedPorts} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the nullValuedPorts map
* @return A modified copy of {@code this} object
*/
public final ImmutableNetworkSettings withNullValuedPorts(@Nullable Map> entries) {
if (this.nullValuedPorts == entries) return this;
@Nullable Map> newValue = entries == null ? null : createUnmodifiableMap(false, false, entries);
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
newValue,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#macAddress() macAddress} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for macAddress (can be {@code null})
* @return A modified copy of the {@code this} object
*/
@Deprecated
public final ImmutableNetworkSettings withMacAddress(@Nullable String value) {
if (Objects.equals(this.macAddress, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
value,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by replacing the {@link NetworkSettings#networks() networks} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the networks map
* @return A modified copy of {@code this} object
*/
public final ImmutableNetworkSettings withNetworks(@Nullable Map entries) {
if (this.networks == entries) return this;
@Nullable Map newValue = entries == null ? null : createUnmodifiableMap(true, false, entries);
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
newValue,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#endpointId() endpointId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for endpointId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkSettings withEndpointId(@Nullable String value) {
if (Objects.equals(this.endpointId, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
value,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#sandboxId() sandboxId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for sandboxId (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkSettings withSandboxId(@Nullable String value) {
if (Objects.equals(this.sandboxId, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
value,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#sandboxKey() sandboxKey} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for sandboxKey (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkSettings withSandboxKey(@Nullable String value) {
if (Objects.equals(this.sandboxKey, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
value,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#hairpinMode() hairpinMode} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for hairpinMode (can be {@code null})
* @return A modified copy of the {@code this} object
*/
@Deprecated
public final ImmutableNetworkSettings withHairpinMode(@Nullable Boolean value) {
if (Objects.equals(this.hairpinMode, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
value,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#linkLocalIPv6Address() linkLocalIPv6Address} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for linkLocalIPv6Address (can be {@code null})
* @return A modified copy of the {@code this} object
*/
@Deprecated
public final ImmutableNetworkSettings withLinkLocalIPv6Address(@Nullable String value) {
if (Objects.equals(this.linkLocalIPv6Address, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
value,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#linkLocalIPv6PrefixLen() linkLocalIPv6PrefixLen} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for linkLocalIPv6PrefixLen (can be {@code null})
* @return A modified copy of the {@code this} object
*/
@Deprecated
public final ImmutableNetworkSettings withLinkLocalIPv6PrefixLen(@Nullable Integer value) {
if (Objects.equals(this.linkLocalIPv6PrefixLen, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
value,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#globalIPv6Address() globalIPv6Address} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for globalIPv6Address (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkSettings withGlobalIPv6Address(@Nullable String value) {
if (Objects.equals(this.globalIPv6Address, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
value,
this.globalIPv6PrefixLen,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#globalIPv6PrefixLen() globalIPv6PrefixLen} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for globalIPv6PrefixLen (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkSettings withGlobalIPv6PrefixLen(@Nullable Integer value) {
if (Objects.equals(this.globalIPv6PrefixLen, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
value,
this.ipv6Gateway);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkSettings#ipv6Gateway() ipv6Gateway} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for ipv6Gateway (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkSettings withIpv6Gateway(@Nullable String value) {
if (Objects.equals(this.ipv6Gateway, value)) return this;
return new ImmutableNetworkSettings(
this.ipAddress,
this.ipPrefixLen,
this.gateway,
this.bridge,
this.portMapping,
this.nullValuedPorts,
this.macAddress,
this.networks,
this.endpointId,
this.sandboxId,
this.sandboxKey,
this.hairpinMode,
this.linkLocalIPv6Address,
this.linkLocalIPv6PrefixLen,
this.globalIPv6Address,
this.globalIPv6PrefixLen,
value);
}
/**
* This instance is equal to all instances of {@code ImmutableNetworkSettings} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ImmutableNetworkSettings
&& equalTo(0, (ImmutableNetworkSettings) another);
}
private boolean equalTo(int synthetic, ImmutableNetworkSettings another) {
return Objects.equals(ipAddress, another.ipAddress)
&& Objects.equals(ipPrefixLen, another.ipPrefixLen)
&& Objects.equals(gateway, another.gateway)
&& Objects.equals(bridge, another.bridge)
&& Objects.equals(portMapping, another.portMapping)
&& Objects.equals(ports, another.ports)
&& Objects.equals(nullValuedPorts, another.nullValuedPorts)
&& Objects.equals(macAddress, another.macAddress)
&& Objects.equals(networks, another.networks)
&& Objects.equals(endpointId, another.endpointId)
&& Objects.equals(sandboxId, another.sandboxId)
&& Objects.equals(sandboxKey, another.sandboxKey)
&& Objects.equals(hairpinMode, another.hairpinMode)
&& Objects.equals(linkLocalIPv6Address, another.linkLocalIPv6Address)
&& Objects.equals(linkLocalIPv6PrefixLen, another.linkLocalIPv6PrefixLen)
&& Objects.equals(globalIPv6Address, another.globalIPv6Address)
&& Objects.equals(globalIPv6PrefixLen, another.globalIPv6PrefixLen)
&& Objects.equals(ipv6Gateway, another.ipv6Gateway);
}
/**
* Computes a hash code from attributes: {@code ipAddress}, {@code ipPrefixLen}, {@code gateway}, {@code bridge}, {@code portMapping}, {@code ports}, {@code nullValuedPorts}, {@code macAddress}, {@code networks}, {@code endpointId}, {@code sandboxId}, {@code sandboxKey}, {@code hairpinMode}, {@code linkLocalIPv6Address}, {@code linkLocalIPv6PrefixLen}, {@code globalIPv6Address}, {@code globalIPv6PrefixLen}, {@code ipv6Gateway}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Objects.hashCode(ipAddress);
h += (h << 5) + Objects.hashCode(ipPrefixLen);
h += (h << 5) + Objects.hashCode(gateway);
h += (h << 5) + Objects.hashCode(bridge);
h += (h << 5) + Objects.hashCode(portMapping);
h += (h << 5) + Objects.hashCode(ports);
h += (h << 5) + Objects.hashCode(nullValuedPorts);
h += (h << 5) + Objects.hashCode(macAddress);
h += (h << 5) + Objects.hashCode(networks);
h += (h << 5) + Objects.hashCode(endpointId);
h += (h << 5) + Objects.hashCode(sandboxId);
h += (h << 5) + Objects.hashCode(sandboxKey);
h += (h << 5) + Objects.hashCode(hairpinMode);
h += (h << 5) + Objects.hashCode(linkLocalIPv6Address);
h += (h << 5) + Objects.hashCode(linkLocalIPv6PrefixLen);
h += (h << 5) + Objects.hashCode(globalIPv6Address);
h += (h << 5) + Objects.hashCode(globalIPv6PrefixLen);
h += (h << 5) + Objects.hashCode(ipv6Gateway);
return h;
}
/**
* Prints the immutable value {@code NetworkSettings} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "NetworkSettings{"
+ "ipAddress=" + ipAddress
+ ", ipPrefixLen=" + ipPrefixLen
+ ", gateway=" + gateway
+ ", bridge=" + bridge
+ ", portMapping=" + portMapping
+ ", ports=" + ports
+ ", nullValuedPorts=" + nullValuedPorts
+ ", macAddress=" + macAddress
+ ", networks=" + networks
+ ", endpointId=" + endpointId
+ ", sandboxId=" + sandboxId
+ ", sandboxKey=" + sandboxKey
+ ", hairpinMode=" + hairpinMode
+ ", linkLocalIPv6Address=" + linkLocalIPv6Address
+ ", linkLocalIPv6PrefixLen=" + linkLocalIPv6PrefixLen
+ ", globalIPv6Address=" + globalIPv6Address
+ ", globalIPv6PrefixLen=" + globalIPv6PrefixLen
+ ", ipv6Gateway=" + ipv6Gateway
+ "}";
}
/**
* Creates an immutable copy of a {@link NetworkSettings} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable NetworkSettings instance
*/
public static ImmutableNetworkSettings copyOf(NetworkSettings instance) {
if (instance instanceof ImmutableNetworkSettings) {
return (ImmutableNetworkSettings) instance;
}
return ImmutableNetworkSettings.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableNetworkSettings ImmutableNetworkSettings}.
*
* ImmutableNetworkSettings.builder()
* .ipAddress(String | null) // nullable {@link NetworkSettings#ipAddress() ipAddress}
* .ipPrefixLen(Integer | null) // nullable {@link NetworkSettings#ipPrefixLen() ipPrefixLen}
* .gateway(String | null) // nullable {@link NetworkSettings#gateway() gateway}
* .bridge(String | null) // nullable {@link NetworkSettings#bridge() bridge}
* .portMapping(Map<String, Map<String, String>> | null) // nullable {@link NetworkSettings#portMapping() portMapping}
* .nullValuedPorts(Map<String, List<org.mandas.docker.client.messages.PortBinding>> | null) // nullable {@link NetworkSettings#nullValuedPorts() nullValuedPorts}
* .macAddress(String | null) // nullable {@link NetworkSettings#macAddress() macAddress}
* .networks(Map<String, org.mandas.docker.client.messages.AttachedNetwork> | null) // nullable {@link NetworkSettings#networks() networks}
* .endpointId(String | null) // nullable {@link NetworkSettings#endpointId() endpointId}
* .sandboxId(String | null) // nullable {@link NetworkSettings#sandboxId() sandboxId}
* .sandboxKey(String | null) // nullable {@link NetworkSettings#sandboxKey() sandboxKey}
* .hairpinMode(Boolean | null) // nullable {@link NetworkSettings#hairpinMode() hairpinMode}
* .linkLocalIPv6Address(String | null) // nullable {@link NetworkSettings#linkLocalIPv6Address() linkLocalIPv6Address}
* .linkLocalIPv6PrefixLen(Integer | null) // nullable {@link NetworkSettings#linkLocalIPv6PrefixLen() linkLocalIPv6PrefixLen}
* .globalIPv6Address(String | null) // nullable {@link NetworkSettings#globalIPv6Address() globalIPv6Address}
* .globalIPv6PrefixLen(Integer | null) // nullable {@link NetworkSettings#globalIPv6PrefixLen() globalIPv6PrefixLen}
* .ipv6Gateway(String | null) // nullable {@link NetworkSettings#ipv6Gateway() ipv6Gateway}
* .build();
*
* @return A new ImmutableNetworkSettings builder
*/
public static ImmutableNetworkSettings.Builder builder() {
return new ImmutableNetworkSettings.Builder();
}
/**
* Builds instances of type {@link ImmutableNetworkSettings ImmutableNetworkSettings}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
static final class Builder implements NetworkSettings.Builder {
private String ipAddress;
private Integer ipPrefixLen;
private String gateway;
private String bridge;
private Map> portMapping = null;
private Map> nullValuedPorts = null;
private String macAddress;
private Map networks = null;
private String endpointId;
private String sandboxId;
private String sandboxKey;
private Boolean hairpinMode;
private String linkLocalIPv6Address;
private Integer linkLocalIPv6PrefixLen;
private String globalIPv6Address;
private Integer globalIPv6PrefixLen;
private String ipv6Gateway;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code NetworkSettings} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(NetworkSettings instance) {
Objects.requireNonNull(instance, "instance");
@Nullable String ipAddressValue = instance.ipAddress();
if (ipAddressValue != null) {
ipAddress(ipAddressValue);
}
@Nullable Integer ipPrefixLenValue = instance.ipPrefixLen();
if (ipPrefixLenValue != null) {
ipPrefixLen(ipPrefixLenValue);
}
@Nullable String gatewayValue = instance.gateway();
if (gatewayValue != null) {
gateway(gatewayValue);
}
@Nullable String bridgeValue = instance.bridge();
if (bridgeValue != null) {
bridge(bridgeValue);
}
@Nullable Map> portMappingValue = instance.portMapping();
if (portMappingValue != null) {
putAllPortMapping(portMappingValue);
}
@Nullable Map> nullValuedPortsValue = instance.nullValuedPorts();
if (nullValuedPortsValue != null) {
putAllNullValuedPorts(nullValuedPortsValue);
}
@Nullable String macAddressValue = instance.macAddress();
if (macAddressValue != null) {
macAddress(macAddressValue);
}
@Nullable Map networksValue = instance.networks();
if (networksValue != null) {
putAllNetworks(networksValue);
}
@Nullable String endpointIdValue = instance.endpointId();
if (endpointIdValue != null) {
endpointId(endpointIdValue);
}
@Nullable String sandboxIdValue = instance.sandboxId();
if (sandboxIdValue != null) {
sandboxId(sandboxIdValue);
}
@Nullable String sandboxKeyValue = instance.sandboxKey();
if (sandboxKeyValue != null) {
sandboxKey(sandboxKeyValue);
}
@Nullable Boolean hairpinModeValue = instance.hairpinMode();
if (hairpinModeValue != null) {
hairpinMode(hairpinModeValue);
}
@Nullable String linkLocalIPv6AddressValue = instance.linkLocalIPv6Address();
if (linkLocalIPv6AddressValue != null) {
linkLocalIPv6Address(linkLocalIPv6AddressValue);
}
@Nullable Integer linkLocalIPv6PrefixLenValue = instance.linkLocalIPv6PrefixLen();
if (linkLocalIPv6PrefixLenValue != null) {
linkLocalIPv6PrefixLen(linkLocalIPv6PrefixLenValue);
}
@Nullable String globalIPv6AddressValue = instance.globalIPv6Address();
if (globalIPv6AddressValue != null) {
globalIPv6Address(globalIPv6AddressValue);
}
@Nullable Integer globalIPv6PrefixLenValue = instance.globalIPv6PrefixLen();
if (globalIPv6PrefixLenValue != null) {
globalIPv6PrefixLen(globalIPv6PrefixLenValue);
}
@Nullable String ipv6GatewayValue = instance.ipv6Gateway();
if (ipv6GatewayValue != null) {
ipv6Gateway(ipv6GatewayValue);
}
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#ipAddress() ipAddress} attribute.
* @param ipAddress The value for ipAddress (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("IPAddress")
@Deprecated
public final Builder ipAddress(@Nullable String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#ipPrefixLen() ipPrefixLen} attribute.
* @param ipPrefixLen The value for ipPrefixLen (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("IPPrefixLen")
@Deprecated
public final Builder ipPrefixLen(@Nullable Integer ipPrefixLen) {
this.ipPrefixLen = ipPrefixLen;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#gateway() gateway} attribute.
* @param gateway The value for gateway (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Gateway")
public final Builder gateway(@Nullable String gateway) {
this.gateway = gateway;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#bridge() bridge} attribute.
* @param bridge The value for bridge (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Bridge")
public final Builder bridge(@Nullable String bridge) {
this.bridge = bridge;
return this;
}
/**
* Put one entry to the {@link NetworkSettings#portMapping() portMapping} map.
* @param key The key in the portMapping map
* @param value The associated value in the portMapping map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addPortMapping(String key, Map value) {
if (this.portMapping == null) {
this.portMapping = new LinkedHashMap>();
}
this.portMapping.put(
Objects.requireNonNull(key, "portMapping key"),
Objects.requireNonNull(value, value == null ? "portMapping value for key: " + key : null));
return this;
}
/**
* Put one entry to the {@link NetworkSettings#portMapping() portMapping} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addPortMapping(Map.Entry> entry) {
if (this.portMapping == null) {
this.portMapping = new LinkedHashMap>();
}
String k = entry.getKey();
Map v = entry.getValue();
this.portMapping.put(
Objects.requireNonNull(k, "portMapping key"),
Objects.requireNonNull(v, v == null ? "portMapping value for key: " + k : null));
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link NetworkSettings#portMapping() portMapping} map. Nulls are not permitted as keys or values, but parameter itself can be null
* @param entries The entries that will be added to the portMapping map
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("PortMapping")
public final Builder portMapping(@Nullable Map> entries) {
if (entries == null) {
this.portMapping = null;
return this;
}
this.portMapping = new LinkedHashMap>();
return putAllPortMapping(entries);
}
/**
* Put all mappings from the specified map as entries to {@link NetworkSettings#portMapping() portMapping} map. Nulls are not permitted
* @param entries The entries that will be added to the portMapping map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putAllPortMapping(Map> entries) {
if (this.portMapping == null) {
this.portMapping = new LinkedHashMap>();
}
for (Map.Entry> e : entries.entrySet()) {
String k = e.getKey();
Map v = e.getValue();
this.portMapping.put(
Objects.requireNonNull(k, "portMapping key"),
Objects.requireNonNull(v, v == null ? "portMapping value for key: " + k : null));
}
return this;
}
/**
* Put one entry to the {@link NetworkSettings#nullValuedPorts() nullValuedPorts} map.
* @param key The key in the nullValuedPorts map
* @param value The associated value in the nullValuedPorts map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addNullValuedPort(String key, List value) {
if (this.nullValuedPorts == null) {
this.nullValuedPorts = new LinkedHashMap>();
}
this.nullValuedPorts.put(key, value);
return this;
}
/**
* Put one entry to the {@link NetworkSettings#nullValuedPorts() nullValuedPorts} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addNullValuedPort(Map.Entry> entry) {
if (this.nullValuedPorts == null) {
this.nullValuedPorts = new LinkedHashMap>();
}
String k = entry.getKey();
List v = entry.getValue();
this.nullValuedPorts.put(k, v);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link NetworkSettings#nullValuedPorts() nullValuedPorts} map. Nulls are not permitted as keys or values, but parameter itself can be null
* @param entries The entries that will be added to the nullValuedPorts map
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Ports")
public final Builder nullValuedPorts(@Nullable Map> entries) {
if (entries == null) {
this.nullValuedPorts = null;
return this;
}
this.nullValuedPorts = new LinkedHashMap>();
return putAllNullValuedPorts(entries);
}
/**
* Put all mappings from the specified map as entries to {@link NetworkSettings#nullValuedPorts() nullValuedPorts} map. Nulls are not permitted
* @param entries The entries that will be added to the nullValuedPorts map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putAllNullValuedPorts(Map> entries) {
if (this.nullValuedPorts == null) {
this.nullValuedPorts = new LinkedHashMap>();
}
for (Map.Entry> e : entries.entrySet()) {
String k = e.getKey();
List v = e.getValue();
this.nullValuedPorts.put(k, v);
}
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#macAddress() macAddress} attribute.
* @param macAddress The value for macAddress (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("MacAddress")
@Deprecated
public final Builder macAddress(@Nullable String macAddress) {
this.macAddress = macAddress;
return this;
}
/**
* Put one entry to the {@link NetworkSettings#networks() networks} map.
* @param key The key in the networks map
* @param value The associated value in the networks map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addNetwork(String key, AttachedNetwork value) {
if (this.networks == null) {
this.networks = new LinkedHashMap();
}
this.networks.put(
Objects.requireNonNull(key, "networks key"),
Objects.requireNonNull(value, value == null ? "networks value for key: " + key : null));
return this;
}
/**
* Put one entry to the {@link NetworkSettings#networks() networks} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addNetwork(Map.Entry entry) {
if (this.networks == null) {
this.networks = new LinkedHashMap();
}
String k = entry.getKey();
AttachedNetwork v = entry.getValue();
this.networks.put(
Objects.requireNonNull(k, "networks key"),
Objects.requireNonNull(v, v == null ? "networks value for key: " + k : null));
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link NetworkSettings#networks() networks} map. Nulls are not permitted as keys or values, but parameter itself can be null
* @param entries The entries that will be added to the networks map
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("Networks")
public final Builder networks(@Nullable Map entries) {
if (entries == null) {
this.networks = null;
return this;
}
this.networks = new LinkedHashMap();
return putAllNetworks(entries);
}
/**
* Put all mappings from the specified map as entries to {@link NetworkSettings#networks() networks} map. Nulls are not permitted
* @param entries The entries that will be added to the networks map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putAllNetworks(Map entries) {
if (this.networks == null) {
this.networks = new LinkedHashMap();
}
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
AttachedNetwork v = e.getValue();
this.networks.put(
Objects.requireNonNull(k, "networks key"),
Objects.requireNonNull(v, v == null ? "networks value for key: " + k : null));
}
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#endpointId() endpointId} attribute.
* @param endpointId The value for endpointId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("EndpointID")
public final Builder endpointId(@Nullable String endpointId) {
this.endpointId = endpointId;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#sandboxId() sandboxId} attribute.
* @param sandboxId The value for sandboxId (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("SandboxID")
public final Builder sandboxId(@Nullable String sandboxId) {
this.sandboxId = sandboxId;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#sandboxKey() sandboxKey} attribute.
* @param sandboxKey The value for sandboxKey (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("SandboxKey")
public final Builder sandboxKey(@Nullable String sandboxKey) {
this.sandboxKey = sandboxKey;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#hairpinMode() hairpinMode} attribute.
* @param hairpinMode The value for hairpinMode (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("HairpinMode")
@Deprecated
public final Builder hairpinMode(@Nullable Boolean hairpinMode) {
this.hairpinMode = hairpinMode;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#linkLocalIPv6Address() linkLocalIPv6Address} attribute.
* @param linkLocalIPv6Address The value for linkLocalIPv6Address (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("LinkLocalIPv6Address")
@Deprecated
public final Builder linkLocalIPv6Address(@Nullable String linkLocalIPv6Address) {
this.linkLocalIPv6Address = linkLocalIPv6Address;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#linkLocalIPv6PrefixLen() linkLocalIPv6PrefixLen} attribute.
* @param linkLocalIPv6PrefixLen The value for linkLocalIPv6PrefixLen (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("LinkLocalIPv6PrefixLen")
@Deprecated
public final Builder linkLocalIPv6PrefixLen(@Nullable Integer linkLocalIPv6PrefixLen) {
this.linkLocalIPv6PrefixLen = linkLocalIPv6PrefixLen;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#globalIPv6Address() globalIPv6Address} attribute.
* @param globalIPv6Address The value for globalIPv6Address (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("GlobalIPv6Address")
public final Builder globalIPv6Address(@Nullable String globalIPv6Address) {
this.globalIPv6Address = globalIPv6Address;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#globalIPv6PrefixLen() globalIPv6PrefixLen} attribute.
* @param globalIPv6PrefixLen The value for globalIPv6PrefixLen (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("GlobalIPv6PrefixLen")
public final Builder globalIPv6PrefixLen(@Nullable Integer globalIPv6PrefixLen) {
this.globalIPv6PrefixLen = globalIPv6PrefixLen;
return this;
}
/**
* Initializes the value for the {@link NetworkSettings#ipv6Gateway() ipv6Gateway} attribute.
* @param ipv6Gateway The value for ipv6Gateway (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@JsonProperty("IPv6Gateway")
public final Builder ipv6Gateway(@Nullable String ipv6Gateway) {
this.ipv6Gateway = ipv6Gateway;
return this;
}
/**
* Builds a new {@link ImmutableNetworkSettings ImmutableNetworkSettings}.
* @return An immutable instance of NetworkSettings
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableNetworkSettings build() {
return new ImmutableNetworkSettings(
ipAddress,
ipPrefixLen,
gateway,
bridge,
portMapping == null ? null : createUnmodifiableMap(false, false, portMapping),
nullValuedPorts == null ? null : createUnmodifiableMap(false, false, nullValuedPorts),
macAddress,
networks == null ? null : createUnmodifiableMap(false, false, networks),
endpointId,
sandboxId,
sandboxKey,
hairpinMode,
linkLocalIPv6Address,
linkLocalIPv6PrefixLen,
globalIPv6Address,
globalIPv6PrefixLen,
ipv6Gateway);
}
}
private static Map createUnmodifiableMap(boolean checkNulls, boolean skipNulls, Map extends K, ? extends V> map) {
switch (map.size()) {
case 0: return Collections.emptyMap();
case 1: {
Map.Entry extends K, ? extends V> e = map.entrySet().iterator().next();
K k = e.getKey();
V v = e.getValue();
if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, v == null ? "value for key: " + k : null);
}
if (skipNulls && (k == null || v == null)) {
return Collections.emptyMap();
}
return Collections.singletonMap(k, v);
}
default: {
Map linkedMap = new LinkedHashMap<>(map.size() * 4 / 3 + 1);
if (skipNulls || checkNulls) {
for (Map.Entry extends K, ? extends V> e : map.entrySet()) {
K k = e.getKey();
V v = e.getValue();
if (skipNulls) {
if (k == null || v == null) continue;
} else if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, v == null ? "value for key: " + k : null);
}
linkedMap.put(k, v);
}
} else {
linkedMap.putAll(map);
}
return Collections.unmodifiableMap(linkedMap);
}
}
}
}