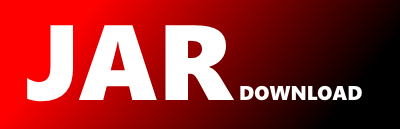
org.mapfish.print.config.Template Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of print-lib Show documentation
Show all versions of print-lib Show documentation
Library for generating PDFs and images from online webmapping services
package org.mapfish.print.config;
import com.google.common.base.Optional;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import org.geotools.styling.Style;
import org.json.JSONException;
import org.json.JSONWriter;
import org.mapfish.print.attribute.Attribute;
import org.mapfish.print.attribute.InternalAttribute;
import org.mapfish.print.config.access.AccessAssertion;
import org.mapfish.print.config.access.AlwaysAllowAssertion;
import org.mapfish.print.config.access.RoleAccessAssertion;
import org.mapfish.print.map.style.StyleParser;
import org.mapfish.print.processor.Processor;
import org.mapfish.print.processor.ProcessorDependencyGraph;
import org.mapfish.print.processor.ProcessorDependencyGraphFactory;
import org.mapfish.print.processor.map.CreateMapProcessor;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.client.ClientHttpRequestFactory;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Nonnull;
/**
* Represents a report template configuration.
*/
public class Template implements ConfigurationObject, HasConfiguration {
private static final org.slf4j.Logger LOGGER = LoggerFactory.getLogger(Template.class);
@Autowired
private ProcessorDependencyGraphFactory processorGraphFactory;
@Autowired
private ClientHttpRequestFactory httpRequestFactory;
@Autowired
private StyleParser styleParser;
private String reportTemplate;
private Map attributes = Maps.newHashMap();
private List processors = Lists.newArrayList();
private boolean mapExport;
private String jdbcUrl;
private String jdbcUser;
private String jdbcPassword;
private volatile ProcessorDependencyGraph processorGraph;
private Map styles = new HashMap<>();
private Configuration configuration;
private AccessAssertion accessAssertion = AlwaysAllowAssertion.INSTANCE;
private PDFConfig pdfConfig = new PDFConfig();
private String tableDataKey;
private String outputFilename;
public final String getOutputFilename() {
return this.outputFilename;
}
/**
* The default output file name of the report (takes precedence over {@link
* org.mapfish.print.config.Configuration#setOutputFilename(String)}). This can be overridden by the
* outputFilename parameter in the request JSON.
*
* This can be a string and can also have a date section in the string that will be filled when the report
* is created for example a section with ${<dateFormatString>} will be replaced with the current
* date formatted in the way defined by the <dateFormatString> string. The format rules are the
* rules in
*
* java.text.SimpleDateFormat (do a google search if the link above is broken).
*
*
* Example: outputFilename: print-${dd-MM-yyyy}
should output:
* print-22-11-2014.pdf
*
*
* Note: the suffix will be appended to the end of the name.
*
*
* @param outputFilename default output file name of the report.
*/
public final void setOutputFilename(final String outputFilename) {
this.outputFilename = outputFilename;
}
/**
* Get the merged configuration between this template and the configuration's template. The settings in
* the template take priority over the configurations settings but if not set in the template then the
* default will be the configuration's options.
*/
public PDFConfig getPdfConfig() {
return this.pdfConfig.getMergedInstance(this.configuration.getPdfConfig());
}
/**
* Configure various properties related to the reports generated as PDFs.
*
* @param pdfConfig the pdf configuration
*/
public final void setPdfConfig(final PDFConfig pdfConfig) {
this.pdfConfig = pdfConfig;
}
/**
* Print out the template information that the client needs for performing a request.
*
* @param json the writer to write the information to.
*/
public final void printClientConfig(final JSONWriter json) throws JSONException {
json.key("attributes");
json.array();
for (Map.Entry entry: this.attributes.entrySet()) {
Attribute attribute = entry.getValue();
if (attribute.getClass().getAnnotation(InternalAttribute.class) == null) {
json.object();
attribute.printClientConfig(json, this);
json.endObject();
}
}
json.endArray();
}
public final Map getAttributes() {
return this.attributes;
}
/**
* Set the attributes for this template.
*
* @param attributes the attribute map
*/
public final void setAttributes(final Map attributes) {
for (Map.Entry entry: attributes.entrySet()) {
Object attribute = entry.getValue();
if (!(attribute instanceof Attribute)) {
final String msg =
"Attribute: '" + entry.getKey() + "' is not an attribute. It is a: " + attribute;
LOGGER.error("Error setting the Attributes: " + msg);
throw new IllegalArgumentException(msg);
} else {
((Attribute) attribute).setConfigName(entry.getKey());
}
}
this.attributes = attributes;
}
public final String getReportTemplate() {
return this.reportTemplate;
}
public final void setReportTemplate(final String reportTemplate) {
this.reportTemplate = reportTemplate;
}
public final List getProcessors() {
return this.processors;
}
/**
* Set the normal processors.
*
* @param processors the processors to set.
*/
public final void setProcessors(final List processors) {
assertProcessors(processors);
this.processors = processors;
}
private void assertProcessors(final List processorsToCheck) {
for (Processor entry: processorsToCheck) {
if (!(entry instanceof Processor)) {
final String msg = "Processor: " + entry + " is not a processor.";
LOGGER.error("Error setting the Attributes: " + msg);
throw new IllegalArgumentException(msg);
}
}
}
/**
* Set the key of the data that is the datasource for the main table in the report.
*
* @param tableData the key of the data that is the datasource for the main table in the report.
*/
public final void setTableData(final String tableData) {
this.tableDataKey = tableData;
}
public final String getTableDataKey() {
return this.tableDataKey;
}
public final String getJdbcUrl() {
return this.jdbcUrl;
}
public final void setJdbcUrl(final String jdbcUrl) {
this.jdbcUrl = jdbcUrl;
}
public final String getJdbcUser() {
return this.jdbcUser;
}
public final void setJdbcUser(final String jdbcUser) {
this.jdbcUser = jdbcUser;
}
public final String getJdbcPassword() {
return this.jdbcPassword;
}
public final void setJdbcPassword(final String jdbcPassword) {
this.jdbcPassword = jdbcPassword;
}
/**
* Get the processor graph to use for executing all the processors for the template.
*
* @return the processor graph.
*/
public final ProcessorDependencyGraph getProcessorGraph() {
if (this.processorGraph == null) {
synchronized (this) {
if (this.processorGraph == null) {
final Map> attcls = new HashMap<>();
for (String attributeName: this.attributes.keySet()) {
attcls.put(attributeName, this.attributes.get(attributeName).getValueType());
}
this.processorGraph = this.processorGraphFactory.build(this.processors, attcls);
}
}
}
return this.processorGraph;
}
/**
* Set the named styles defined in the configuration for this.
*
* @param styles set the styles specific for this template.
*/
public final void setStyles(final Map styles) {
this.styles = styles;
}
/**
* Look for a style in the named styles provided in the configuration.
*
* @param styleName the name of the style to look for.
*/
@SuppressWarnings("unchecked")
@Nonnull
public final Optional