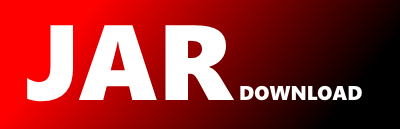
org.mapfish.print.attribute.DataSourceAttribute Maven / Gradle / Ivy
Show all versions of print-lib Show documentation
package org.mapfish.print.attribute;
import org.json.JSONException;
import org.json.JSONWriter;
import org.mapfish.print.PrintException;
import org.mapfish.print.config.Configuration;
import org.mapfish.print.config.Template;
import org.mapfish.print.output.Values;
import org.mapfish.print.wrapper.PArray;
import org.mapfish.print.wrapper.PObject;
import org.mapfish.print.wrapper.yaml.PYamlArray;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
/**
*
* This attribute represents a collection of attributes which can be used as the data source of a Jasper
* report's table/detail section.
*
*
* For example consider the case where the report should contain multiple tables or charts but the number of
* reports may change depending on the request. In this case the client will post a datasource attribute json
* object containing an array of all the table attribute objects. The {@link
* org.mapfish.print.processor.jasper.DataSourceProcessor} will process the datasource attribute and create a
* Jasper datasource that contains all the tables.
*
*
* This datasource must be used in tandem with the
* {@link org.mapfish.print.processor.jasper.DataSourceProcessor}
* processor (see !createDataSource processor).
*
*
* The json data of this attribute is special since it represents an array of attributes, each element in the
* array must contain all of the attributes required to satisfy the processors in the {@link
* org.mapfish.print.processor.jasper.DataSourceProcessor}.
*
*
* Example configuration:
*
*
* datasource: !datasource
* table: !table
* map: !map
* width: 200
* height: 100
*
*
* Example request data:
*
*
* datasource: [
* {
* table: {
* ... // normal table attribute data
* },
* map: {
* ... // normal map attribute data
* }
* }, {
* table: {
* ... // normal table attribute data
* },
* map: {
* ... // normal map attribute data
* }
* }
* ]
*
* [[examples=verboseExample,datasource_dynamic_tables,datasource_many_dynamictables_legend,
* datasource_multiple_maps,customDynamicReport,report]]
*/
public final class DataSourceAttribute implements Attribute {
private static final Logger LOGGER = LoggerFactory.getLogger(DataSourceAttribute.class);
private Map attributes = new HashMap<>();
private String configName;
private PYamlArray defaults;
/**
* Default values for this attribute. Example:
*
* attributes:
* datasource: !datasource
* attributes:
* name: !string {}
* count: !integer {}
* default:
* - name: "name"
* - count: 3
*
* @param defaultData The default values.
*/
public void setDefault(final List