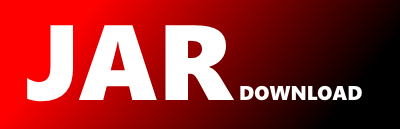
org.oscim.theme.XmlRenderThemeStyleLayer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vtm Show documentation
Show all versions of vtm Show documentation
OpenGL vector map library written in Java - running on Android, iOS, Desktop and within the browser.
/*
* Copyright 2014 Ludwig M Brinckmann
* Copyright 2016 devemux86
*
* This program is free software: you can redistribute it and/or modify it under the
* terms of the GNU Lesser General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A
* PARTICULAR PURPOSE. See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License along with
* this program. If not, see .
*/
package org.oscim.theme;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* An individual layer in the render theme menu system.
* A layer can have translations, categories that will always be enabled when the layer is selected,
* as well as optional overlays.
*/
public class XmlRenderThemeStyleLayer implements Serializable {
private static final long serialVersionUID = 1L;
private final Set categories;
private final String defaultLanguage;
private final boolean enabled;
private final String id;
private final List overlays;
private final Map titles;
private final boolean visible;
XmlRenderThemeStyleLayer(String id, boolean visible, boolean enabled, String defaultLanguage) {
this.id = id;
this.titles = new HashMap<>();
this.categories = new LinkedHashSet<>();
this.visible = visible;
this.defaultLanguage = defaultLanguage;
this.enabled = enabled;
this.overlays = new ArrayList<>();
}
public void addCategory(String category) {
this.categories.add(category);
}
public void addOverlay(XmlRenderThemeStyleLayer overlay) {
this.overlays.add(overlay);
}
public void addTranslation(String language, String name) {
this.titles.put(language, name);
}
public Set getCategories() {
return this.categories;
}
public String getId() {
return this.id;
}
public List getOverlays() {
return this.overlays;
}
public String getTitle(String language) {
String result = this.titles.get(language);
if (result == null) {
return this.titles.get(this.defaultLanguage);
}
return result;
}
public Map getTitles() {
return this.titles;
}
public boolean isEnabled() {
return this.enabled;
}
public boolean isVisible() {
return this.visible;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy