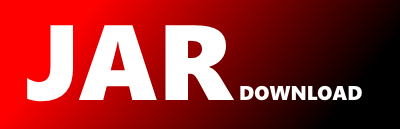
org.oscim.utils.QuadTree Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vtm Show documentation
Show all versions of vtm Show documentation
OpenGL vector map library written in Java - running on Android, iOS, Desktop and within the browser.
package org.oscim.utils;
import org.oscim.core.Box;
import org.oscim.core.Point;
import org.oscim.utils.pool.Pool;
import org.oscim.utils.quadtree.BoxTree;
import org.oscim.utils.quadtree.BoxTree.BoxItem;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.List;
/**
* Quad-tree with fixed extents.
* This implementation uses int bounding-boxes internally,
* so items extents should be greater than 1. FIXME tests this case
*/
public class QuadTree extends BoxTree, T> implements SpatialIndex {
static final Logger log = LoggerFactory.getLogger(QuadTree.class);
public QuadTree(int extents, int maxDepth) {
super(extents, maxDepth);
}
final Pool> boxPool = new Pool>() {
@Override
protected BoxItem createItem() {
return new BoxItem();
}
};
private BoxItem getBox(Box box) {
BoxItem it = boxPool.get();
it.x1 = (int) box.xmin;
it.y1 = (int) box.ymin;
it.x2 = (int) box.xmax;
it.y2 = (int) box.ymax;
return it;
}
@Override
public void insert(Box box, T item) {
insert(new BoxItem(box, item));
}
@Override
public boolean remove(Box box, T item) {
BoxItem bbox = getBox(box);
boolean ok = remove(bbox, item);
boxPool.release(bbox);
return ok;
}
static class CollectCb implements SearchCb {
@SuppressWarnings("unchecked")
@Override
public boolean call(T item, Object context) {
List l = (List) context;
l.add(item);
return true;
}
}
final CollectCb collectCb = new CollectCb();
@Override
public List search(Box bbox, List results) {
BoxItem box = getBox(bbox);
search(box, collectCb, results);
boxPool.release(box);
return results;
}
@Override
public boolean search(Box bbox, SearchCb cb, Object context) {
BoxItem box = getBox(bbox);
boolean finished = search(box, cb, context);
boxPool.release(box);
return finished;
}
@Override
public List searchKNearestNeighbors(Point center, int k, double maxDistance, List results) {
// TODO
return results;
}
@Override
public void searchKNearestNeighbors(Point center, int k, double maxDistance, SearchCb cb, Object context) {
// TODO
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy