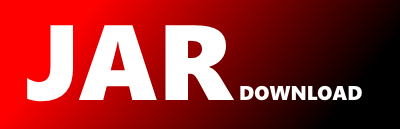
org.mapstruct.ValueMapping Maven / Gradle / Ivy
/*
* Copyright MapStruct Authors.
*
* Licensed under the Apache License version 2.0, available at http://www.apache.org/licenses/LICENSE-2.0
*/
package org.mapstruct;
import java.lang.annotation.ElementType;
import java.lang.annotation.Repeatable;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* Configures the mapping of source constant value to target constant value.
*
* Supported mappings are
*
* - Enumeration to Enumeration
*
* Example 1:
*
*
* public enum OrderType { RETAIL, B2B, C2C, EXTRA, STANDARD, NORMAL }
*
* public enum ExternalOrderType { RETAIL, B2B, SPECIAL, DEFAULT }
*
* @ValueMapping(target = "SPECIAL", source = "EXTRA"),
* @ValueMapping(target = "DEFAULT", source = "STANDARD"),
* @ValueMapping(target = "DEFAULT", source = "NORMAL")
* ExternalOrderType orderTypeToExternalOrderType(OrderType orderType);
*
* Mapping result:
* +---------------------+----------------------------+
* | OrderType | ExternalOrderType |
* +---------------------+----------------------------+
* | null | null |
* | OrderType.EXTRA | ExternalOrderType.SPECIAL |
* | OrderType.STANDARD | ExternalOrderType.DEFAULT |
* | OrderType.NORMAL | ExternalOrderType.DEFAULT |
* | OrderType.RETAIL | ExternalOrderType.RETAIL |
* | OrderType.B2B | ExternalOrderType.B2B |
* +---------------------+----------------------------+
*
*
* Example 2:
*
*
* @ValueMapping( source = MappingConstants.NULL, target = "DEFAULT" ),
* @ValueMapping( source = "STANDARD", target = MappingConstants.NULL ),
* @ValueMapping( source = MappingConstants.ANY_REMAINING, target = "SPECIAL" )
* ExternalOrderType orderTypeToExternalOrderType(OrderType orderType);
*
* Mapping result:
* +---------------------+----------------------------+
* | OrderType | ExternalOrderType |
* +---------------------+----------------------------+
* | null | ExternalOrderType.DEFAULT |
* | OrderType.STANDARD | null |
* | OrderType.RETAIL | ExternalOrderType.RETAIL |
* | OrderType.B2B | ExternalOrderType.B2B |
* | OrderType.NORMAL | ExternalOrderType.SPECIAL |
* | OrderType.EXTRA | ExternalOrderType.SPECIAL |
* +---------------------+----------------------------+
*
*
* Example 3:
*
* MapStruct will WARN on incomplete mappings. However, if for some reason no match is found, an
* {@link java.lang.IllegalStateException} will be thrown. This compile-time error can be avoided by
* using {@link MappingConstants#THROW_EXCEPTION} for {@link ValueMapping#target()}. It will result an
* {@link java.lang.IllegalArgumentException} at runtime.
*
* @ValueMapping( source = "STANDARD", target = "DEFAULT" ),
* @ValueMapping( source = "C2C", target = MappingConstants.THROW_EXCEPTION )
* ExternalOrderType orderTypeToExternalOrderType(OrderType orderType);
*
* Mapping result:
* {@link java.lang.IllegalArgumentException} with the error message:
* Unexpected enum constant: C2C
*
*
* @author Sjaak Derksen
*/
@Repeatable(ValueMappings.class)
@Retention(RetentionPolicy.CLASS)
@Target({ElementType.METHOD, ElementType.ANNOTATION_TYPE})
public @interface ValueMapping {
/**
* The source value constant to use for this mapping.
*
*
* Valid values:
*
* - enum constant name
* - {@link MappingConstants#NULL}
* - {@link MappingConstants#ANY_REMAINING}
* - {@link MappingConstants#ANY_UNMAPPED}
*
*
* NOTE:When using <ANY_REMAINING>, MapStruct will perform the normal name based mapping, in which
* source is mapped to target based on enum identifier equality. Using <ANY_UNMAPPED> will not apply name
* based mapping.
*
* @return The source value.
*/
String source();
/**
* The target value constant to use for this mapping.
*
*
* Valid values:
*
* - enum constant name
* - {@link MappingConstants#NULL}
* - {@link MappingConstants#THROW_EXCEPTION}
*
*
* @return The target value.
*/
String target();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy