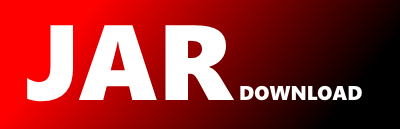
org.maptalks.geojson.json.GeoJSONFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geojson4j Show documentation
Show all versions of geojson4j Show documentation
GeoJSON Serilization and mutual conversion with JSON .
The newest version!
package org.maptalks.geojson.json;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import org.maptalks.geojson.*;
import java.util.HashMap;
import java.util.Map;
public class GeoJSONFactory {
static Map geoJsonTypeMap = new HashMap();
static {
geoJsonTypeMap.put(GeoJSONTypes.TYPE_POINT,Point.class);
geoJsonTypeMap.put(GeoJSONTypes.TYPE_LINESTRING,LineString.class);
geoJsonTypeMap.put(GeoJSONTypes.TYPE_POLYGON,Polygon.class);
geoJsonTypeMap.put(GeoJSONTypes.TYPE_MULTIPOINT,MultiPoint.class);
geoJsonTypeMap.put(GeoJSONTypes.TYPE_MULTILINESTRING,MultiLineString.class);
geoJsonTypeMap.put(GeoJSONTypes.TYPE_MULTIPOLYGON,MultiPolygon.class);
geoJsonTypeMap.put(GeoJSONTypes.TYPE_GEOMETRYCOLLECTION,GeometryCollection.class);
}
public static GeoJSON create(String json) {
JSONObject node = JSON.parseObject(json);
return create(node);
}
/**
* 将json解析为FeatureCollection数组
* @param json
* @return
*/
public static FeatureCollection[] createFeatureCollectionArray(String json) {
JSONArray node = JSON.parseArray(json);
int size = node.size();
FeatureCollection[] result = new FeatureCollection[size];
for (int i = 0; i < size; i++) {
if (node.get(i) == null) {
result[i] = null;
continue;
}
result[i] = ((FeatureCollection) create(((JSONObject) node.get(i))));
}
return result;
}
/**
* 将json解析为Feature数组
* @param json
* @return
*/
public static Feature[] createFeatureArray(String json) {
JSONArray node = JSON.parseArray(json);
int size = node.size();
Feature[] result = new Feature[size];
for (int i = 0; i < size; i++) {
if (node.get(i) == null) {
result[i] = null;
continue;
}
GeoJSON geojson = create(((JSONObject) node.get(i)));
if (geojson instanceof Geometry) {
result[i] = new Feature(((Geometry) geojson));
} else {
result[i] = ((Feature) geojson);
}
}
return result;
}
/**
* 将json解析为Geometry数组
* @param json
* @return
*/
public static Geometry[] createGeometryArray(String json) {
JSONArray node = JSON.parseArray(json);
int size = node.size();
Geometry[] result = new Geometry[size];
for (int i = 0; i < size; i++) {
if (node.get(i) == null) {
result[i] = null;
continue;
}
GeoJSON geojson = create(((JSONObject) node.get(i)));
if (geojson instanceof Feature) {
result[i] = ((Feature) geojson).getGeometry();
} else {
result[i] = ((Geometry) geojson);
}
}
return result;
}
private static GeoJSON create(JSONObject node) {
String type = node.getString("type");
if ("FeatureCollection".equals(type)) {
return readFeatureCollection(node);
} else if ("Feature".equals(type)) {
return readFeature(node);
} else {
return readGeometry(node, type);
}
}
private static FeatureCollection readFeatureCollection(JSONObject node) {
JSONArray jFeatures = node.getJSONArray("features");
if (jFeatures == null) {
//return a empty FeatureCollection
FeatureCollection result = new FeatureCollection();
result.setFeatures(new Feature[0]);
return result;
}
int size = jFeatures.size();
Feature[] features = new Feature[size];
for (int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy