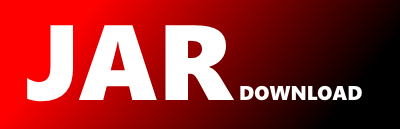
org.marid.ide.project.ProjectProfile Maven / Gradle / Ivy
/*
* Copyright (c) 2016 Dmitry Ovchinnikov
* Marid, the free data acquisition and visualization software
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of
* the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.marid.ide.project;
import org.apache.maven.model.Model;
import org.apache.maven.model.io.xpp3.MavenXpp3Reader;
import org.apache.maven.model.io.xpp3.MavenXpp3Writer;
import org.codehaus.plexus.util.xml.pull.XmlPullParserException;
import org.jmlspecs.annotation.Immutable;
import org.marid.logging.LogSupport;
import javax.annotation.Nonnull;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.file.Files;
import java.nio.file.NoSuchFileException;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.logging.Logger;
import static java.util.Arrays.asList;
import static org.apache.commons.lang3.SystemUtils.USER_HOME;
/**
* @author Dmitry Ovchinnikov
*/
@Immutable
public class ProjectProfile implements LogSupport {
private final Model model;
private final Path path;
private final Path pomFile;
private final Path src;
private final Path target;
private final Path srcMain;
private final Path srcTest;
private final Path srcMainJava;
private final Path srcMainResources;
private final Path srcTestJava;
private final Path srcTestResources;
private final Path repository;
private final Logger logger;
public ProjectProfile(String name) {
path = Paths.get(USER_HOME, "marid", "profiles", name);
pomFile = path.resolve("pom.xml");
src = path.resolve("src");
target = path.resolve("target");
srcMain = src.resolve("main");
srcTest = src.resolve("test");
srcMainJava = srcMain.resolve("java");
srcMainResources = srcMain.resolve("resources");
srcTestJava = srcTest.resolve("java");
srcTestResources = srcTest.resolve("resources");
repository = path.resolve(".repo");
logger = Logger.getLogger(getName());
model = loadModel();
model.setModelVersion("4.0.0");
}
private Model loadModel() {
try (final InputStream is = Files.newInputStream(pomFile)) {
final MavenXpp3Reader reader = new MavenXpp3Reader();
return reader.read(is);
} catch (NoSuchFileException x) {
log(FINE, "There is no {0} file", x.getFile());
} catch (IOException x) {
log(WARNING, "Unable to read pom.xml", x);
} catch (XmlPullParserException x) {
log(WARNING, "Unable to parse pom.xml", x);
}
return new Model();
}
public Model getModel() {
return model;
}
public Path getPath() {
return path;
}
public Path getPomFile() {
return pomFile;
}
public Path getRepository() {
return repository;
}
public Path getTarget() {
return target;
}
public String getName() {
return path.getFileName().toString();
}
@Nonnull
@Override
public Logger logger() {
return logger;
}
private void createFileStructure() {
try {
for (final Path dir : asList(srcMainJava, srcMainResources, srcTestJava, srcTestResources)) {
Files.createDirectories(dir);
}
} catch (Exception x) {
log(WARNING, "Unable to create file structure", x);
}
}
private void savePomFile() {
try (final OutputStream os = Files.newOutputStream(pomFile)) {
final MavenXpp3Writer writer = new MavenXpp3Writer();
writer.write(os, model);
} catch (IOException x) {
log(WARNING, "Unable to save {0}", x, pomFile);
}
}
public void save() {
createFileStructure();
savePomFile();
}
@Override
public boolean equals(Object obj) {
return obj instanceof ProjectProfile && (((ProjectProfile) obj).getName().equals(this.getName()));
}
@Override
public int hashCode() {
return getName().hashCode();
}
@Override
public String toString() {
return getName();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy