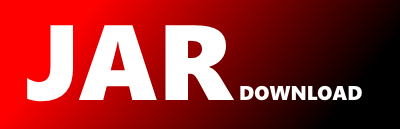
org.marid.xml.XmlBind Maven / Gradle / Ivy
/*
* Copyright (c) 2016 Dmitry Ovchinnikov
* Marid, the free data acquisition and visualization software
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of
* the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*/
package org.marid.xml;
import org.marid.cache.MaridClassValue;
import org.marid.function.SafeBiFunction;
import org.marid.function.SafeTriConsumer;
import org.marid.misc.Calls;
import org.marid.misc.Casts;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import java.util.function.Consumer;
import static javax.xml.bind.JAXBContext.newInstance;
import static org.marid.misc.Calls.call;
/**
* @author Dmitry Ovchinnikov
*/
public class XmlBind {
private static final ClassValue CONTEXT_CV = new MaridClassValue<>(c -> call(() -> newInstance(c)));
public static final Consumer DEFAULT_OUTPUT = marshaller -> Calls.call(() -> {
marshaller.setProperty(Marshaller.JAXB_ENCODING, "UTF-8");
return null;
});
public static final Consumer FORMATTED_OUTPUT = DEFAULT_OUTPUT.andThen(marshaller -> Calls.call(() -> {
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
return null;
}));
public static final Consumer DEFAULT_INPUT = unmarshaller -> {
};
public static void save(Class> type,
R bean,
T output,
Consumer marshallerConsumer,
SafeTriConsumer marshalTask) {
final JAXBContext context = CONTEXT_CV.get(type);
final Marshaller marshaller = call(context::createMarshaller);
marshallerConsumer.accept(marshaller);
marshalTask.accept(marshaller, bean, output);
}
public static R load(Class> type,
T input,
Consumer unmarshallerConsumer,
SafeBiFunction unmarshalTask) {
final JAXBContext context = CONTEXT_CV.get(type);
final Unmarshaller unmarshaller = call(context::createUnmarshaller);
unmarshallerConsumer.accept(unmarshaller);
return Casts.cast(unmarshalTask.apply(unmarshaller, input));
}
public static void save(R bean,
T output,
Consumer marshallerConsumer,
SafeTriConsumer marshalTask) {
save(bean.getClass(), bean, output, marshallerConsumer, marshalTask);
}
public static void save(R bean, T output, SafeTriConsumer marshalTask) {
save(bean, output, FORMATTED_OUTPUT, marshalTask);
}
public static R loadBean(Class type,
T input,
Consumer unmarshallerConsumer,
SafeBiFunction unmarshalTask) {
return load(type, input, unmarshallerConsumer, unmarshalTask);
}
public static R load(Class type, T input, SafeBiFunction unmarshalTask) {
return loadBean(type, input, DEFAULT_INPUT, unmarshalTask);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy