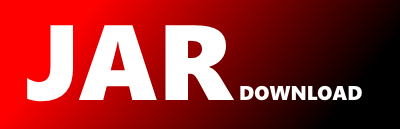
org.marid.applib.dialogs.ShellDialog Maven / Gradle / Ivy
The newest version!
/*-
* #%L
* marid-webapp
* %%
* Copyright (C) 2012 - 2018 MARID software development group
* %%
* This program and the accompanying materials are made available under the terms of the Eclipse Public License v1.0
* and Eclipse Distribution License v. 1.0 which accompanies this distribution.
* The Eclipse Public License is available at http://www.eclipse.org/legal/epl-v10.html
* and the Eclipse Distribution License is available at
* http://www.eclipse.org/org/documents/edl-v10.php.
* #L%
*/
package org.marid.applib.dialogs;
import org.eclipse.swt.SWT;
import org.eclipse.swt.layout.GridData;
import org.eclipse.swt.layout.GridLayout;
import org.eclipse.swt.widgets.*;
import org.intellij.lang.annotations.MagicConstant;
import org.marid.applib.image.AppImage;
import org.marid.applib.image.WithImages;
import org.marid.misc.Condition;
import org.marid.misc.ListenableValue;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Stream;
import static org.eclipse.swt.SWT.NONE;
import static org.marid.applib.controls.Controls.control;
public abstract class ShellDialog extends Shell implements WithImages {
public ShellDialog(Shell parent, @MagicConstant(flagsFromClass = SWT.class) int style) {
super(parent, style);
final var layout = new GridLayout(1, false);
layout.marginHeight = 10;
layout.marginWidth = 10;
layout.verticalSpacing = 10;
setLayout(layout);
}
public ShellDialog(Shell parent) {
this(parent, SWT.CLOSE | SWT.TITLE | SWT.APPLICATION_MODAL);
}
protected int formStyle() {
return NONE;
}
protected Composite form(Composite parent) {
return Stream.of(parent.getChildren())
.filter(Composite.class::isInstance)
.map(Composite.class::cast)
.filter(c -> "form".equals(c.getData("dialogControlType")))
.findFirst()
.orElseGet(() -> {
final var c = new Composite(parent, formStyle());
final var l = new GridLayout(3, false);
l.marginWidth = l.marginHeight = 10;
c.setLayout(l);
c.setLayoutData(new GridData(GridData.FILL_BOTH));
c.setData("dialogControlType", "form");
return c;
});
}
@SafeVarargs
public final C addField(String text,
AppImage image,
Function supplier,
Consumer... controlConsumers) {
return addField(this, text, image, supplier, controlConsumers);
}
@SafeVarargs
public final C addField(Composite parent,
String text,
AppImage image,
Function supplier,
Consumer... controlConsumers) {
final var form = form(parent);
final var imgButton = new Label(form, NONE);
imgButton.setImage(image(image));
final var label = new Label(form, NONE);
label.setText(text + ": ");
final var control = supplier.apply(form);
control.setLayoutData(new GridData(GridData.FILL_HORIZONTAL));
label.setData("labelFor", control);
for (final var controlConsumer : controlConsumers) {
controlConsumer.accept(control);
}
return control;
}
@SafeVarargs
public final Button addButton(String text, AppImage image, Listener listener, Consumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy