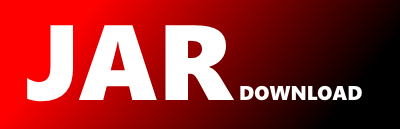
org.marketcetera.marketdata.csv.CSVQuantum Maven / Gradle / Ivy
package org.marketcetera.marketdata.csv;
import java.util.Arrays;
import org.marketcetera.marketdata.MarketDataRequest;
import org.marketcetera.util.misc.ClassVersion;
/* $License$ */
/**
* Represents a single item of data extracted from a CSV market data file and the meta-data necessary
* to interpret it.
*
* Objects of this type are passed to {@link CSVFeedEventTranslator#toEvent(Object, String)} implementations.
*
* @author Colin DuPlantis
* @since 2.1.0
* @version $Id: CSVQuantum.java 16154 2012-07-14 16:34:05Z colin $
*/
@ClassVersion("$Id: CSVQuantum.java 16154 2012-07-14 16:34:05Z colin $")
public class CSVQuantum
{
/**
* Gets a CSVQuantum
object with the given properties.
*
* @param inLine a String[]
value containing the distinct tokens from a single line in a CSV file
* @param inRequest a MarketDataRequest
value containing the original market data request
* @param inReplayRate a double
value containing the replay rate at which to process the events
* @return a CSVQuantum
value
*/
static CSVQuantum getQuantum(String[] inLine,
MarketDataRequest inRequest,
double inReplayRate)
{
return new CSVQuantum(inLine,
inRequest,
inReplayRate);
}
/**
* Get the line value.
*
*
This method retrieves the discrete elements of a single line of a market data file
*
* @return a String[]
value
*/
public String[] getLine()
{
return line;
}
/**
* Get the request value.
*
* @return a MarketDataRequest
value
*/
public MarketDataRequest getRequest()
{
return request;
}
/**
* Get the replayRate value.
*
* @return a double
value
*/
public double getReplayRate()
{
return replayRate;
}
/* (non-Javadoc)
* @see java.lang.Object#toString()
*/
@Override
public String toString()
{
return Arrays.toString(line);
}
/**
* Create a new CSVQuantum instance.
*
* @param inLine a String[]
value
* @param inRequest a MarketDataRequest
value
* @param inReplayRate a double
value
*/
private CSVQuantum(String[] inLine,
MarketDataRequest inRequest,
double inReplayRate)
{
line = inLine;
request = inRequest;
replayRate = inReplayRate;
}
/**
* a single line from the file
*/
private final String[] line;
/**
* the original market data request
*/
private final MarketDataRequest request;
/**
* rate at which to replay the events
*/
private final double replayRate;
}