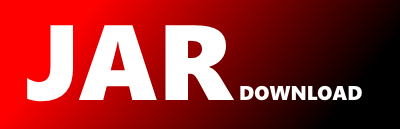
org.marketcetera.trade.client.TradeClient Maven / Gradle / Ivy
package org.marketcetera.trade.client;
import java.math.BigDecimal;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Map;
import org.marketcetera.core.BaseClient;
import org.marketcetera.core.position.PositionKey;
import org.marketcetera.event.HasFIXMessage;
import org.marketcetera.fix.ActiveFixSession;
import org.marketcetera.persist.CollectionPageResponse;
import org.marketcetera.persist.PageRequest;
import org.marketcetera.trade.AverageFillPrice;
import org.marketcetera.trade.BrokerID;
import org.marketcetera.trade.ExecutionReport;
import org.marketcetera.trade.ExecutionReportSummary;
import org.marketcetera.trade.Instrument;
import org.marketcetera.trade.Option;
import org.marketcetera.trade.Order;
import org.marketcetera.trade.OrderID;
import org.marketcetera.trade.OrderSummary;
import org.marketcetera.trade.Report;
import org.marketcetera.trade.ReportID;
import org.marketcetera.trade.Suggestion;
import org.marketcetera.trade.SuggestionListener;
import org.marketcetera.trade.TradeMessagePublisher;
/* $License$ */
/**
* Provides trading-related services.
*
* @author Colin DuPlantis
* @version $Id$
* @since $Release$
*/
public interface TradeClient
extends BaseClient,TradeMessagePublisher
{
/**
* Get the currently available FIX initiator sessions.
*
* @return a List<ActiveFixSession>
value
*/
List readAvailableFixInitiatorSessions();
/**
* Get open orders.
*
* @return a Collection<OrderSummary>
value
*/
Collection getOpenOrders();
/**
* Get open orders.
*
* @param inPageRequest a PageRequest
value
* @return a CollectionPageResponset<OrderSummary>
value
*/
CollectionPageResponse getOpenOrders(PageRequest inPageRequest);
/**
* Submit the given orders.
*
* @param inOrders a List<Order>
value
* @return a List<SendOrderResponse>
value
*/
List sendOrders(List inOrders);
/**
* Submit a trade suggestion.
*
* @param inSuggestion a Suggestion
value
*/
void sendOrderSuggestion(Suggestion inSuggestion);
/**
* Submit the given order.
*
* @param inOrder an Order
value
* @return a SendOrderResponse
value
*/
SendOrderResponse sendOrder(Order inOrder);
/**
* Returns the position of the supplied instrument based on reports generated and received on or before the supplied date in UTC.
*
* @param inDate a Date
value
* @param inInstrument an Instrument
value
* @return a BigDecimal
value
*/
BigDecimal getPositionAsOf(Date inDate,
Instrument inInstrument);
/**
* Returns all positions based on reports generated and received on or before the supplied date in UTC.
*
* @param inDate a Date
value
* @return a Map<PositionKey<? extends Instrument>,BigDecimal>
value
*/
Map,BigDecimal> getAllPositionsAsOf(Date inDate);
/**
* Returns all positions of options with the given root symbols based on reports generated and received on or before the supplied date in UTC.
*
* @param inDate a Date
value
* @param inRootSymbols a String[]
value
* @return a Map<PositionKey<Optiont>,BigDecimal>
value
*/
Map,BigDecimal> getOptionPositionsAsOf(Date inDate,
String... inRootSymbols);
/**
* Add the given report to the system data flow.
*
* Reports added this way will be added to the system data bus. Reports will be
* persisted and become part of the system record. The report will be owned by the
* user logged in to the client.
*
*
This will affect reported positions
.
*
* @param inReport a HasFIXMessage
value
* @param inBrokerID a BrokerID
value
*/
void addReport(HasFIXMessage inReport,
BrokerID inBrokerID);
/**
* Removes the given report from the persistent report store.
*
* Reports removed this way will not be added to the system data bus and no clients
* will receive this report.
*
*
This will affect reported positions
.
*
* @param inReportId a ReportID
value
*/
void deleteReport(ReportID inReportId);
/**
* Resolves the given symbol to an Instrument
.
*
* @param inSymbol a String
value
* @return an Instrument
value
*/
Instrument resolveSymbol(String inSymbol);
/**
* Find the root order ID for the order chain of the given order ID.
*
* @param inOrderID an OrderID
value
* @return an OrderID
value
*/
OrderID findRootOrderIdFor(OrderID inOrderID);
/**
* Get the option roots for the given underlying.
*
* @param inUnderlying a String
value
* @return a Collection<String>
value
*/
Collection getOptionRoots(String inUnderlying);
/**
* Get the underlying for the given root.
*
* @param inOptionRoot a String
value
* @return a String
value
*/
String getUnderlying(String inOptionRoot);
/**
* Get the most recent execution report for the order chain represented by the given order id.
*
* The given OrderID
can be either from any order in the chain or the root order id for the chain.
* In either case, the most recent execution report for the entire chain will be returned, not the given order necessarily.
*
*
If no execution report exists for any order in the given chain, the call will return null.
*
* @param inOrderId an OrderID
value
* @return an ExecutionReport
value or null
*/
ExecutionReport getLatestExecutionReportForOrderChain(OrderID inOrderId);
/**
* Get reports with the given page request.
*
* @param inPageRequest a PageRequest
value
* @return a CollectionPageResponse<Report>
value
*/
CollectionPageResponse getReports(PageRequest inPageRequest);
/**
* Get fills with the given page request.
*
* @param inPageRequest a PageRequest
value
* @return a CollectionPageResponse<ExecutionReportSummary>
value
*/
CollectionPageResponse getFills(PageRequest inPageRequest);
/**
* Get average price fills values.
*
* @param inPageRequest a PageRequest
value
* @return a CollectionPageResponse<AveragePriceFill>
value
*/
CollectionPageResponse getAveragePriceFills(PageRequest inPageRequest);
/**
* Add the given suggestion listener.
*
* @param inSuggestionListener a SuggestionListener
value
*/
void addSuggestionListener(SuggestionListener inSuggestionListener);
/**
* Remove the given suggestion listener.
*
* @param inSuggestionListener a SuggestionListener
value
*/
void removeSuggestionListener(SuggestionListener inSuggestionListener);
}