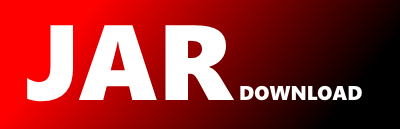
org.marvelution.jira.plugins.jenkins.model.SiteStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jira-jenkins-plugin Show documentation
Show all versions of jira-jenkins-plugin Show documentation
JIRA Plugin to integrate Jenkins CI
/*
* Copyright (c) 2012-present Marvelution B.V.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.marvelution.jira.plugins.jenkins.model;
import javax.xml.bind.annotation.*;
import static java.util.Objects.requireNonNull;
/**
* Site Status model
*
* @author Mark Rekveld
* @since 1.1.0
*/
@XmlRootElement(name = "site-status")
@XmlAccessorType(XmlAccessType.FIELD)
public class SiteStatus {
@XmlElement
private Status status;
@XmlElement
private boolean pluginInstalled;
@XmlElement
private Site site;
/**
* Package JAX-B Constructor
*/
/* package */ SiteStatus() {
}
private SiteStatus(Status status, Site site) {
this(status, site, false);
}
private SiteStatus(Status status, Site site, boolean pluginInstalled) {
this.status = status;
this.site = site;
this.pluginInstalled = pluginInstalled;
}
/**
* Returns the {@link #status}
*/
public Status getStatus() {
return status;
}
/**
* Returns the {@link #site}
*/
public Site getSite() {
return site;
}
/**
* Returns a flag indicating if the plugin is installed on the remote site
*
* @since 2.0.0
*/
public boolean isPluginInstalled() {
return pluginInstalled;
}
/**
* Sets the plugin installed flag
*/
public void setPluginInstalled(boolean pluginInstalled) {
this.pluginInstalled = pluginInstalled;
}
/**
* Creates a new {@link SiteStatus} for the specified {@link Status} and {@link Site}
*/
public static SiteStatus forStatus(Status status, Site site) {
return new SiteStatus(requireNonNull(status), requireNonNull(site));
}
/**
* Creates a new {@link Status#ONLINE} {@link SiteStatus} that has the plugin installed
*/
public static SiteStatus online(Site site) {
return new SiteStatus(Status.ONLINE, site, true);
}
/**
* Creates a new {@link Status#ONLINE} {@link SiteStatus} that doesn't has the plugin installed
*/
public static SiteStatus onlineNoPlugin(Site site) {
return forStatus(Status.ONLINE, site);
}
/**
* Creates a new {@link Status#OFFLINE} {@link SiteStatus} with assumes no plugin installed
*/
public static SiteStatus offline(Site site) {
return forStatus(Status.OFFLINE, site);
}
/**
* Creates a new {@link Status#NOT_ACCESSIBLE} {@link SiteStatus} with assumes no plugin installed
*/
public static SiteStatus notAccessible(Site site) {
return forStatus(Status.NOT_ACCESSIBLE, site);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy