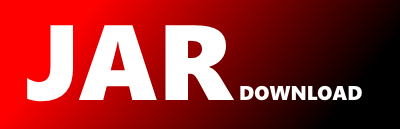
org.marvelution.jira.plugins.jenkins.scheduler.JenkinsScheduler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jira-jenkins-plugin Show documentation
Show all versions of jira-jenkins-plugin Show documentation
JIRA Plugin to integrate Jenkins CI
/*
* Copyright (c) 2012-present Marvelution B.V.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.marvelution.jira.plugins.jenkins.scheduler;
import java.util.Date;
import java.util.concurrent.TimeUnit;
import javax.inject.Inject;
import javax.inject.Named;
import com.atlassian.plugin.spring.scanner.annotation.export.ExportAsService;
import com.atlassian.plugin.spring.scanner.annotation.imports.ComponentImport;
import com.atlassian.sal.api.lifecycle.LifecycleAware;
import com.atlassian.scheduler.SchedulerService;
import com.atlassian.scheduler.SchedulerServiceException;
import com.atlassian.scheduler.config.JobConfig;
import com.atlassian.scheduler.config.JobId;
import com.atlassian.scheduler.config.RunMode;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import static org.marvelution.jira.plugins.jenkins.scheduler.JenkinsSynchronizationJob.JOB_KEY;
import static com.atlassian.scheduler.config.Schedule.forInterval;
import static java.lang.System.currentTimeMillis;
/**
* Jenkins Scheduler implementation
*
* @author Mark Rekveld
* @since 1.0.0
*/
@Named
@ExportAsService(value = LifecycleAware.class)
public class JenkinsScheduler implements LifecycleAware {
private static final Logger LOGGER = LoggerFactory.getLogger(JenkinsScheduler.class);
private static final String PROPERTY_KEY = "jenkins.connector.scheduler.interval";
private static final long DEFAULT_INTERVAL = TimeUnit.HOURS.toMillis(1);
private static final JobId JOB_ID = JobId.of("jenkins.sync.schedule");
private final SchedulerService schedulerService;
private final JenkinsSynchronizationJob synchronizationJob;
@Inject
public JenkinsScheduler(@ComponentImport SchedulerService schedulerService, JenkinsSynchronizationJob synchronizationJob) {
this.schedulerService = schedulerService;
this.synchronizationJob = synchronizationJob;
}
@Override
public void onStart() {
LOGGER.info("Starting the Jenkins Scheduler");
String property = System.getProperty(PROPERTY_KEY, Long.toString(DEFAULT_INTERVAL));
long interval;
try {
interval = Long.parseLong(property);
} catch (Exception e) {
interval = DEFAULT_INTERVAL;
}
if (!schedulerService.getRegisteredJobRunnerKeys().contains(JOB_KEY)) {
LOGGER.debug("Registering Jenkins JobRunner {}", JOB_KEY);
schedulerService.registerJobRunner(JOB_KEY, synchronizationJob);
}
JobConfig jobConfig = JobConfig.forJobRunnerKey(JOB_KEY)
.withRunMode(RunMode.RUN_ONCE_PER_CLUSTER)
.withSchedule(forInterval(interval, new Date(currentTimeMillis() + TimeUnit.MINUTES.toMillis(1))));
try {
LOGGER.debug("Scheduling the Jenkins Synchronization Job {} with {}", JOB_ID, jobConfig);
schedulerService.scheduleJob(JOB_ID, jobConfig);
} catch (SchedulerServiceException e) {
LOGGER.error(
"Failed to schedule the Jenkins Synchronization Job. Builds can only be synchronized manually until this is fixed!", e);
}
}
@Override
public void onStop() {
LOGGER.info("Stopping the Jenkins Scheduler");
schedulerService.unregisterJobRunner(JOB_KEY);
schedulerService.unscheduleJob(JOB_ID);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy