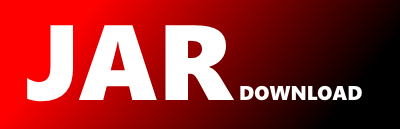
org.marvelution.jira.plugins.jenkins.services.JobService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jira-jenkins-plugin Show documentation
Show all versions of jira-jenkins-plugin Show documentation
JIRA Plugin to integrate Jenkins CI
/*
* Copyright (c) 2012-present Marvelution B.V.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.marvelution.jira.plugins.jenkins.services;
import java.net.URI;
import java.util.List;
import java.util.Set;
import org.marvelution.jira.plugins.jenkins.model.Build;
import org.marvelution.jira.plugins.jenkins.model.Job;
import org.marvelution.jira.plugins.jenkins.model.JobSyncStatus;
import org.marvelution.jira.plugins.jenkins.model.Site;
/**
* {@link Job} services interface
*
* @author Mark Rekveld
* @since 1.0.0
*/
public interface JobService {
/**
* Synchronize a single {@link Job} by the given jobId
*
* @param jobId the id of the {@link Job} to synchronize
*/
void sync(int jobId);
/**
* Synchronize all the Jobs and there Builds from the given {@link Site}
*
* @param site the {@link Site} to synchronize
*/
void syncAllFromSite(Site site);
/**
* Get the current synchronization status of the job given
*
* @param job the {@link Job} to get the {@link JobSyncStatus} for
* @return the {@link JobSyncStatus}, may be {@code null} in case of no current status
*/
JobSyncStatus getSyncStatus(Job job);
/**
* Get all the {@link Job} objects related to the given {@link Site}
*
* @param site the {@link Site} to to get the Jobs for
* @return the {@link List} of {@link Job}s
*/
List getAllBySite(Site site);
/**
* Get all the {@link Job} objects related to the given {@link Site}
*
* @param site the {@link Site} to to get the Jobs for
* @param includeDeleted flag to include deleted jobs or not
* @return the {@link List} of {@link Job}s
*/
List getAllBySite(Site site, boolean includeDeleted);
/**
* Get all the {@link Job}s configured
*
* @return {@link List} of all the {@link Job} objects available
*/
List getAll();
/**
* Get all the {@link Job}s configured
*
* @param includeDeleted flag to include deleted jobs or not
* @return {@link List} of all the {@link Job} objects available
*/
List getAll(boolean includeDeleted);
/**
* Get a {@link Job} by its ID
*
* @param jobId the ID of the {@link Job} to get
* @return the {@link Job} may be {@code null}
*/
Job get(int jobId);
/**
* Get a {@link Job} by its name
*
* @param name the name of the job to get
* @return a {@link List} of {@link Job}s, may be {@code empty} but not {@code null}
*/
List get(String name);
/**
* Get the {@link URI} for the {@link Job} given
*
* @param job the {@link Job} to get the URL for
* @return the {@link URI}
* @see #getJobBuildUrl(Job, Build)
*/
URI getJobUrl(Job job);
/**
* Get the {@link URI} for the {@link Job} given. If the {@link Build} is not {@code null} then the build url
* part will be added to the URI
*
* @param job the {@link Job} to get the URL for
* @param build optionally the {@link Build} to add to the URL
* @return the {@link URI}
*/
URI getJobBuildUrl(Job job, Build build);
/**
* Enable/Disable the synchronization of a job
*
* @param jobId the ID of the {@link Job} to update the synchronization state of
* @param enabled the synchronization state ({@code true} to enable synchronization and {@code false} to disable it)
*/
void enable(int jobId, boolean enabled);
/**
* Save the given {@link Job}
*
* @param job the {@link Job} to sav
* @return the saved {@link Job}
*/
Job save(Job job);
/**
* Delete the given {@link Job}
*
* @param job the {@link Job} to delete
*/
void delete(Job job);
/**
* Delete all the Jobs and there Builds that came from the given {@link Site}
*
* @param site the {@link Site} to delete all the builds from
*/
void deleteAllFromSite(Site site);
/**
* Mark the given {@link Job} as deleted
*
* @param job the {@link Job} to be marked as deleted
*/
void markAsDelete(Job job);
/**
* Mark all the Jobs and there Builds that came from the given {@link Site} as deleted
*
* @param site the {@link Site} to mark all the jobs and builds from
*/
void markAllFromSiteAsDelete(Site site);
/**
* Get all the issue keys that are related to the given {@link Job}
*
* @param job the {@link Job} to get the issue keys for
* @return Collection of Issue Keys, may be {@code empty}, but not {@code null}
*/
Set getRelatedIssuesKeys(Job job);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy